前言
为了更好的学习smtp协议,利用python的smtplib,pyqt5模块开发了一个自动登录qq邮箱可以向所有qq发送文本及文件邮件的程序。
SMTP协议简介
SMTP 的全称是“Simple Mail Transfer Protocol”,即简单邮件传输协议。它是一组用于从源地址到目的地址传输邮件的规范,通过它来控制邮件的中转方式。SMTP 协议属于 TCP/IP 协议簇,它帮助每台计算机在发送或中转信件时找到下一个目的地。SMTP 服务器就是遵循 SMTP 协议的发送邮件服务器。
环境准备
1.首先要在qq邮箱开启smtp服务,点击设置-》账户如下图
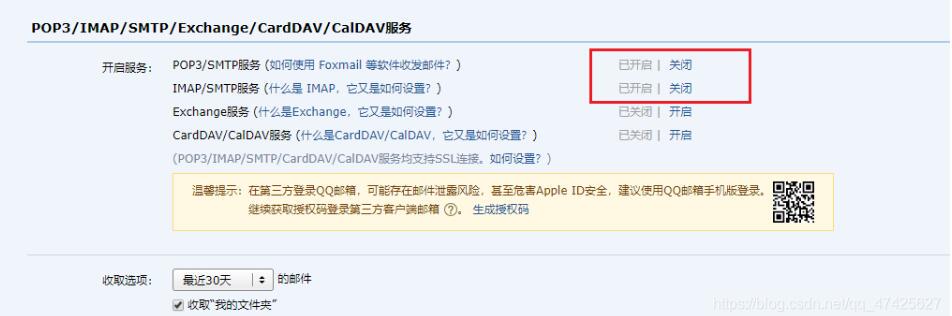
然后验证密保,获取授权码,smtp登录的密码会使用到授权码。
主要代码实现
smtp登录qq,发送邮件
主要使用smtplib模块实现登录qq邮箱发送邮件,可以发送文本邮件也可以发送文件。
import smtplib
from email.header import Header
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from smtp.Mail import MailConfig
class Smtp:
def __init__(self):
self.config=MailConfig();
self.stmp = smtplib.SMTP_SSL(self.config.host.encode(), self.config.port)
print("smtp server connect success!")
self.sender='mocked<'+self.config.user+'>' #qq邮箱发送者须为此格式
def loginSmtp(self):
self.stmp.login(self.config.user, self.config.password)
print("smtp login success!")
#发送文本信息
def sendMessage(self,msg,title,receiver):
message = MIMEText(msg, 'plain', 'utf-8') # 发送的内容
message['From'] = self.sender
message['To'] = 'test@qq.com'
subject = title # 邮件主题
message['Subject'] = Header(subject, 'utf-8') # 邮件标题
try:
self.stmp.sendmail(self.sender, receiver, message.as_string())
except Exception as e:
print('邮件发送失败--' + str(e))
print('邮件发送成功')
#发送文本及文件
def sendMsgAndFile(self,msg,title,filePath,fileName,receiver):
# 创建一个带附件的实例
message = MIMEMultipart()
message['From'] = self.sender
message['To'] = Header("测试", 'utf-8')
subject = title
message['Subject'] = Header(subject, 'utf-8')
# 邮件正文内容
message.attach(MIMEText(msg, 'plain', 'utf-8'))
# 构造附件1,传送当前目录下的 test.txt 文件
att1 = MIMEText(open(filePath, 'rb').read(), 'base64', 'utf-8')
att1["Content-Type"] = 'application/octet-stream'
# 这里的filename可以任意写,写什么名字,邮件中显示什么名字
att1["Content-Disposition"] = 'attachment; filename='+fileName
message.attach(att1)
try:
self.stmp.sendmail(self.sender, receiver, message.as_string())
print("邮件发送成功")
except smtplib.SMTPException:
print("Error: 无法发送邮件")
pyqt5实现图形界面
import os
import sys
from PyQt5 import QtCore, QtGui, QtWidgets
from PyQt5.QtWidgets import QFileDialog, QMessageBox
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
sys.path.insert(0, BASE_DIR)
from smtp.SmtpUtil import Smtp
from utils import StringUtils
class Ui_MainWindow(object):
def setupUi(self, MainWindow):
MainWindow.setObjectName("MainWindow")
MainWindow.resize(583, 481)
icon = QtGui.QIcon()
icon.addPixmap(QtGui.QPixmap("../res/smtpIcon.png"), QtGui.QIcon.Normal, QtGui.QIcon.Off)
MainWindow.setWindowIcon(icon)
self.centralwidget = QtWidgets.QWidget(MainWindow)
self.centralwidget.setObjectName("centralwidget")
self.verticalLayout_6 = QtWidgets.QVBoxLayout(self.centralwidget)
self.verticalLayout_6.setObjectName("verticalLayout_6")
self.horizontalLayout = QtWidgets.QHBoxLayout()
self.horizontalLayout.setObjectName("horizontalLayout")
self.label = QtWidgets.QLabel(self.centralwidget)
self.label.setObjectName("label")
self.horizontalLayout.addWidget(self.label)
self.lineEdit = QtWidgets.QLineEdit(self.centralwidget)
self.lineEdit.setObjectName("lineEdit")
self.horizontalLayout.addWidget(self.lineEdit)
self.verticalLayout_6.addLayout(self.horizontalLayout)
self.horizontalLayout_2 = QtWidgets.QHBoxLayout()
self.horizontalLayout_2.setObjectName("horizontalLayout_2")
self.label_2 = QtWidgets.QLabel(self.centralwidget)
self.label_2.setObjectName("label_2")
self.horizontalLayout_2.addWidget(self.label_2)
self.lineEdit_2 = QtWidgets.QLineEdit(self.centralwidget)
self.lineEdit_2.setObjectName("lineEdit_2")
self.horizontalLayout_2.addWidget(self.lineEdit_2)
self.verticalLayout_6.addLayout(self.horizontalLayout_2)
self.verticalLayout_4 = QtWidgets.QVBoxLayout()
self.verticalLayout_4.setObjectName("verticalLayout_4")
self.label_3 = QtWidgets.QLabel(self.centralwidget)
self.label_3.setObjectName("label_3")
self.verticalLayout_4.addWidget(self.label_3)
self.verticalLayout_6.addLayout(self.verticalLayout_4)
self.verticalLayout = QtWidgets.QVBoxLayout()
self.verticalLayout.setObjectName("verticalLayout")
self.plainTextEdit = QtWidgets.QPlainTextEdit(self.centralwidget)
self.plainTextEdit.setObjectName("plainTextEdit")
self.verticalLayout.addWidget(self.plainTextEdit)
self.verticalLayout_6.addLayout(self.verticalLayout)
self.horizontalLayout_3 = QtWidgets.QHBoxLayout()
self.horizontalLayout_3.setObjectName("horizontalLayout_3")
self.pushButton = QtWidgets.QPushButton(self.centralwidget)
self.pushButton.setObjectName("pushButton")
self.horizontalLayout_3.addWidget(self.pushButton)
self.pushButton_2 = QtWidgets.QPushButton(self.centralwidget)
self.pushButton_2.setAutoRepeat(False)
self.pushButton_2.setAutoRepeatDelay(200)
self.pushButton_2.setObjectName("pushButton_2")
self.horizontalLayout_3.addWidget(self.pushButton_2)
self.verticalLayout_5 = QtWidgets.QVBoxLayout()
self.verticalLayout_5.setObjectName("verticalLayout_5")
self.horizontalLayout_3.addLayout(self.verticalLayout_5)
self.horizontalLayout_4 = QtWidgets.QHBoxLayout()
self.horizontalLayout_4.setObjectName("horizontalLayout_4")
self.horizontalLayout_3.addLayout(self.horizontalLayout_4)
self.verticalLayout_6.addLayout(self.horizontalLayout_3)
MainWindow.setCentralWidget(self.centralwidget)
self.menubar = QtWidgets.QMenuBar(MainWindow)
self.menubar.setGeometry(QtCore.QRect(0, 0, 583, 18))
self.menubar.setObjectName("menubar")
MainWindow.setMenuBar(self.menubar)
self.statusbar = QtWidgets.QStatusBar(MainWindow)
self.statusbar.setObjectName("statusbar")
MainWindow.setStatusBar(self.statusbar)
self.retranslateUi(MainWindow)
QtCore.QMetaObject.connectSlotsByName(MainWindow)
#点击动作事件触发
self.pushButton.clicked.connect(self.addFile)
self.pushButton_2.clicked.connect(self.send)
def retranslateUi(self, MainWindow):
_translate = QtCore.QCoreApplication.translate
MainWindow.setWindowTitle(_translate("MainWindow", "smtp邮件发送"))
self.label.setText(_translate("MainWindow", "收件人地址:"))
self.label_2.setText(_translate("MainWindow", "邮件主题:"))
self.label_3.setText(_translate("MainWindow", "内容:"))
self.plainTextEdit.setPlainText(_translate("MainWindow", "..."))
self.pushButton.setText(_translate("MainWindow", "添加文件"))
self.pushButton_2.setText(_translate("MainWindow", "确认发送"))
self.fileName = ''
def send(self):
receiver=self.lineEdit.text()
title=self.lineEdit_2.text()
content=self.plainTextEdit.toPlainText()
print(receiver+""+title+" "+content)
if StringUtils.isNull(receiver) or StringUtils.isNull(title):
QMessageBox.information(self.MainWindow, '警告', '邮件接收者和邮件主题不能为空!',
QMessageBox.Ok | QMessageBox.Close,
QMessageBox.Close)
smtp = Smtp()
smtp.loginSmtp()
if StringUtils.isNull(self.fileName):
smtp.sendMessage(content,title,receiver)
else:
smtp.sendMsgAndFile(content,title,self.filePath,self.fileName,receiver)
def addFile(self):
file=QFileDialog.getOpenFileName()
self.filePath=file[0]
self.fileName=os.path.basename(self.filePath)
self.plainTextEdit.appendPlainText("附件:"+self.filePath)
print(self.filePath)
print(self.fileName)
if __name__=='__main__':
import sys
app = QtWidgets.QApplication(sys.argv)
widget = QtWidgets.QMainWindow()
ui = Ui_MainWindow()
ui.setupUi(widget)
widget.show()
sys.exit(app.exec_())
功能效果展示
程序界面:
填写收件人邮箱地址及相应信息点击确认发送即可发送邮件
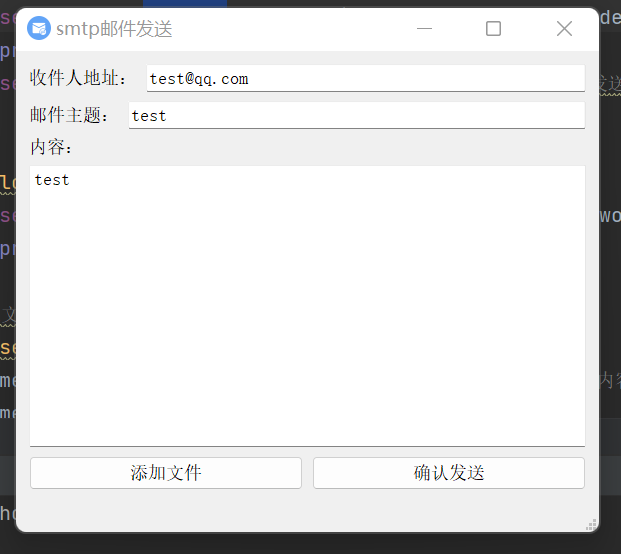
成功发送文本邮件:
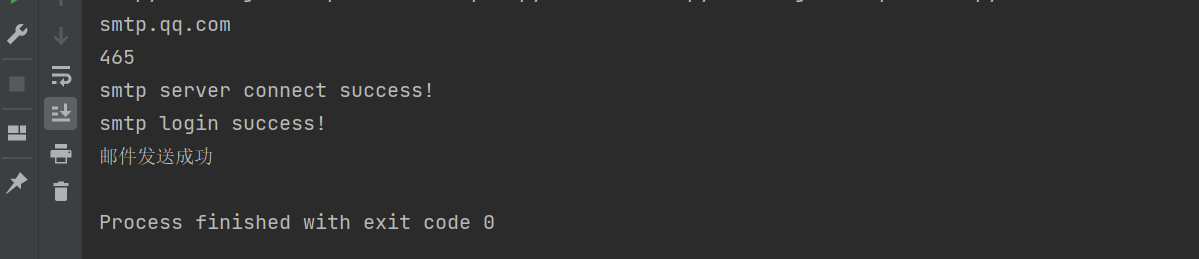
邮箱收到文本及附件:
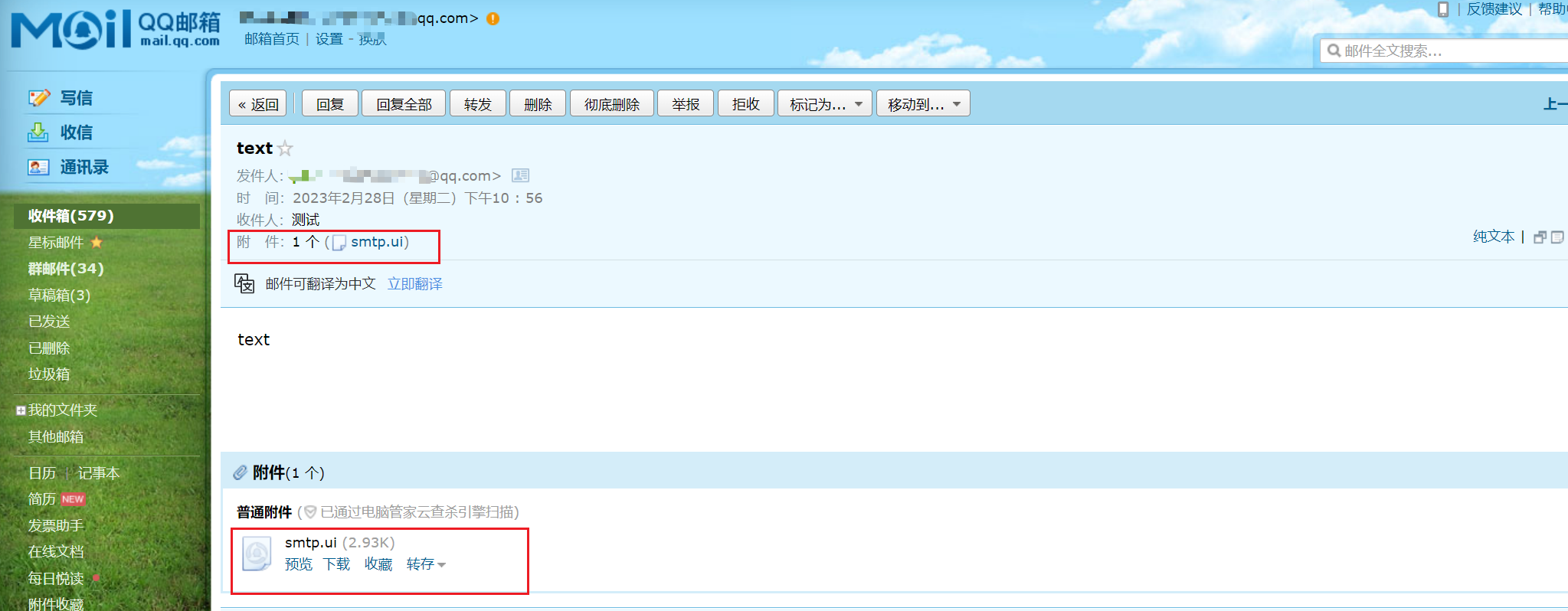