说明
- 加密后的内容是16进制。试着找过Base64的,但是前端加密后发现密文和正确的密文对不上,也许是不会用,能力有限,不研究了,直接用16进制的。
- 后端下载完jar包后,还需要做一些环境配置,否则运行时报错。
后端
依赖
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.70</version>
</dependency>
环境配置
- 下载后的jar包放在
jre\lib\ext
下
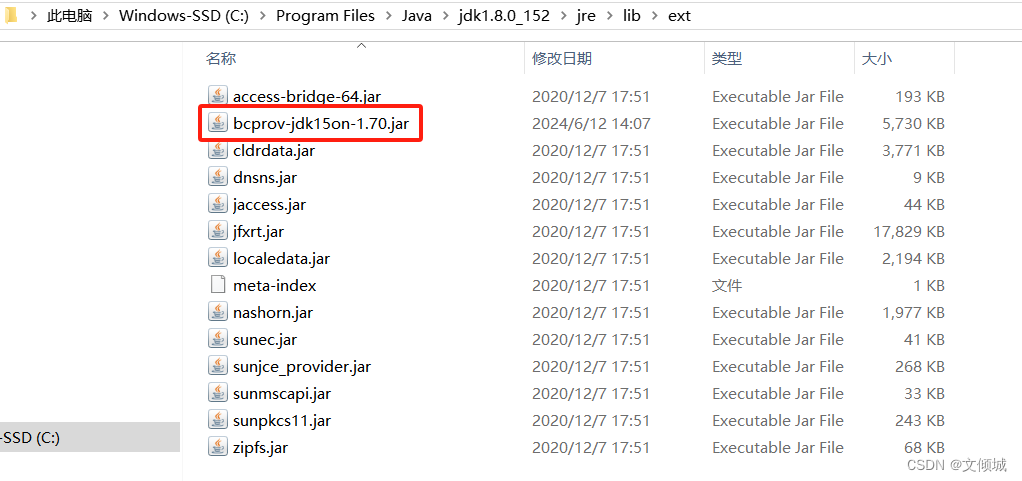
- 修改
jre\lib\security\java.security
,最后一行增加 security.provider.11=org.bouncycastle.jce.provider.BouncyCastleProvider
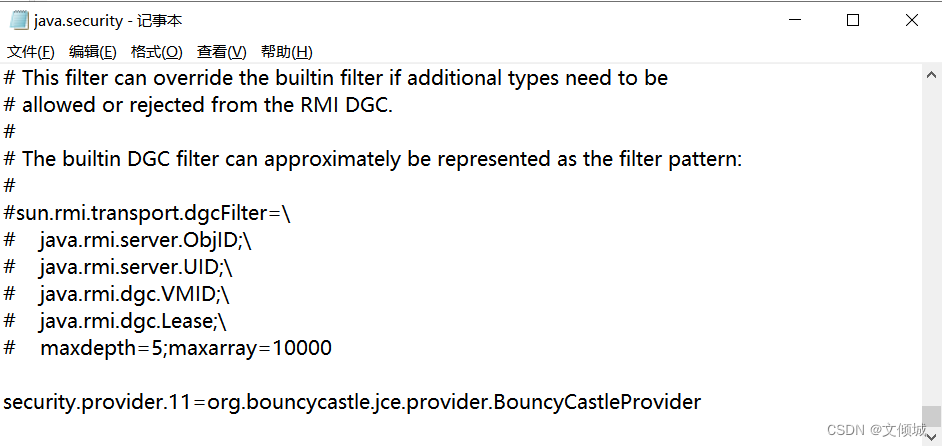
代码
package com.xxx.utils.sm4;
import java.math.BigInteger;
public class ConvertUtil
{
public static byte[] intToBytes(int num)
{
byte[] bytes = new byte[4];
bytes[0] = (byte) (0xff & (num >> 0));
bytes[1] = (byte) (0xff & (num >> 8));
bytes[2] = (byte) (0xff & (num >> 16));
bytes[3] = (byte) (0xff & (num >> 24));
return bytes;
}
public static int byteToInt(byte[] bytes)
{
int num = 0;
int temp;
temp = (0x000000ff & (bytes[0])) << 0;
num = num | temp;
temp = (0x000000ff & (bytes[1])) << 8;
num = num | temp;
temp = (0x000000ff & (bytes[2])) << 16;
num = num | temp;
temp = (0x000000ff & (bytes[3])) << 24;
num = num | temp;
return num;
}
public static byte[] longToBytes(long num)
{
byte[] bytes = new byte[8];
for (int i = 0; i < 8; i++)
{
bytes[i] = (byte) (0xff & (num >> (i * 8)));
}
return bytes;
}
public static byte[] byteConvert32Bytes(BigInteger n)
{
byte tmpd[] = (byte[])null;
if(n == null)
{
return null;
}
if(n.toByteArray().length == 33)
{
tmpd = new byte[32];
System.arraycopy(n.toByteArray(), 1, tmpd, 0, 32);
}
else if(n.toByteArray().length == 32)
{
tmpd = n.toByteArray();
}
else
{
tmpd = new byte[32];
for(int i = 0; i < 32 - n.toByteArray().length; i++)
{
tmpd[i] = 0;
}
System.arraycopy(n.toByteArray(), 0, tmpd, 32 - n.toByteArray().length, n.toByteArray().length);
}
return tmpd;
}
public static BigInteger byteConvertInteger(byte[] b)
{
if (b[0] < 0)
{
byte[] temp = new byte[b.length + 1];
temp[0] = 0;
System.arraycopy(b, 0, temp, 1, b.length);
return new BigInteger(temp);
}
return new BigInteger(b);
}
public static String getHexString(byte[] bytes)
{
return getHexString(bytes, true);
}
public static String getHexString(byte[] bytes, boolean upperCase)
{
String ret = "";
for (int i = 0; i < bytes.length; i++)
{
ret += Integer.toString((bytes[i] & 0xff) + 0x100, 16).substring(1);
}
return upperCase ? ret.toUpperCase() : ret;
}
public static void printHexString(byte[] bytes)
{
for (int i = 0; i < bytes.length; i++)
{
String hex = Integer.toHexString(bytes[i] & 0xFF);
if (hex.length() == 1)
{
hex = '0' + hex;
}
System.out.print("0x" + hex.toUpperCase() + ",");
}
System.out.println("");
}
public static byte[] hexStringToBytes(String hexString)
{
if (hexString == null || hexString.equals(""))
{
return null;
}
hexString = hexString.toUpperCase();
int length = hexString.length() / 2;
char[] hexChars = hexString.toCharArray();
byte[] d = new byte[length];
for (int i = 0; i < length; i++)
{
int pos = i * 2;
d[i] = (byte) (charToByte(hexChars[pos]) << 4 | charToByte(hexChars[pos + 1]));
}
return d;
}
public static byte charToByte(char c)
{
return (byte) "0123456789ABCDEF".indexOf(c);
}
private static final char[] DIGITS_LOWER = {'0', '1', '2', '3', '4', '5',
'6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f'};
private static final char[] DIGITS_UPPER = {'0', '1', '2', '3', '4', '5',
'6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F'};
public static char[] encodeHex(byte[] data) {
return encodeHex(data, true);
}
public static char[] encodeHex(byte[] data, boolean toLowerCase) {
return encodeHex(data, toLowerCase ? DIGITS_LOWER : DIGITS_UPPER);
}
protected static char[] encodeHex(byte[] data, char[] toDigits) {
int l = data.length;
char[] out = new char[l << 1];
for (int i = 0, j = 0; i < l; i++) {
out[j++] = toDigits[(0xF0 & data[i]) >>> 4];
out[j++] = toDigits[0x0F & data[i]];
}
return out;
}
public static String encodeHexString(byte[] data) {
return encodeHexString(data, true);
}
public static String encodeHexString(byte[] data, boolean toLowerCase) {
return encodeHexString(data, toLowerCase ? DIGITS_LOWER : DIGITS_UPPER);
}
protected static String encodeHexString(byte[] data, char[] toDigits) {
return new String(encodeHex(data, toDigits));
}
public static byte[] decodeHex(char[] data) {
int len = data.length;
if ((len & 0x01) != 0) {
throw new RuntimeException("Odd number of characters.");
}
byte[] out = new byte[len >> 1];
for (int i = 0, j = 0; j < len; i++) {
int f = toDigit(data[j], j) << 4;
j++;
f = f | toDigit(data[j], j);
j++;
out[i] = (byte) (f & 0xFF);
}
return out;
}
protected static int toDigit(char ch, int index) {
int digit = Character.digit(ch, 16);
if (digit == -1) {
throw new RuntimeException("Illegal hexadecimal character " + ch
+ " at index " + index);
}
return digit;
}
public static String StringToAsciiString(String content) {
String result = "";
int max = content.length();
for (int i = 0; i < max; i++) {
char c = content.charAt(i);
String b = Integer.toHexString(c);
result = result + b;
}
return result;
}
public static String hexStringToString(String hexString, int encodeType) {
String result = "";
int max = hexString.length() / encodeType;
for (int i = 0; i < max; i++) {
char c = (char) hexStringToAlgorism(hexString
.substring(i * encodeType, (i + 1) * encodeType));
result += c;
}
return result;
}
public static int hexStringToAlgorism(String hex) {
hex = hex.toUpperCase();
int max = hex.length();
int result = 0;
for (int i = max; i > 0; i--) {
char c = hex.charAt(i - 1);
int algorism = 0;
if (c >= '0' && c <= '9') {
algorism = c - '0';
} else {
algorism = c - 55;
}
result += Math.pow(16, max - i) * algorism;
}
return result;
}
public static String hexStringToBinary(String hex) {
hex = hex.toUpperCase();
String result = "";
int max = hex.length();
for (int i = 0; i < max; i++) {
char c = hex.charAt(i);
switch (c) {
case '0':
result += "0000";
break;
case '1':
result += "0001";
break;
case '2':
result += "0010";
break;
case '3':
result += "0011";
break;
case '4':
result += "0100";
break;
case '5':
result += "0101";
break;
case '6':
result += "0110";
break;
case '7':
result += "0111";
break;
case '8':
result += "1000";
break;
case '9':
result += "1001";
break;
case 'A':
result += "1010";
break;
case 'B':
result += "1011";
break;
case 'C':
result += "1100";
break;
case 'D':
result += "1101";
break;
case 'E':
result += "1110";
break;
case 'F':
result += "1111";
break;
}
}
return result;
}
public static String AsciiStringToString(String content) {
String result = "";
int length = content.length() / 2;
for (int i = 0; i < length; i++) {
String c = content.substring(i * 2, i * 2 + 2);
int a = hexStringToAlgorism(c);
char b = (char) a;
String d = String.valueOf(b);
result += d;
}
return result;
}
public static String algorismToHexString(int algorism, int maxLength) {
String result = "";
result = Integer.toHexString(algorism);
if (result.length() % 2 == 1) {
result = "0" + result;
}
return patchHexString(result.toUpperCase(), maxLength);
}
public static String byteToString(byte[] bytearray) {
String result = "";
char temp;
int length = bytearray.length;
for (int i = 0; i < length; i++) {
temp = (char) bytearray[i];
result += temp;
}
return result;
}
public static int binaryToAlgorism(String binary) {
int max = binary.length();
int result = 0;
for (int i = max; i > 0; i--) {
char c = binary.charAt(i - 1);
int algorism = c - '0';
result += Math.pow(2, max - i) * algorism;
}
return result;
}
public static String algorismToHEXString(int algorism) {
String result = "";
result = Integer.toHexString(algorism);
if (result.length() % 2 == 1) {
result = "0" + result;
}
result = result.toUpperCase();
return result;
}
static public String patchHexString(String str, int maxLength) {
String temp = "";
for (int i = 0; i < maxLength - str.length(); i++) {
temp = "0" + temp;
}
str = (temp + str).substring(0, maxLength);
return str;
}
public static int parseToInt(String s, int defaultInt, int radix) {
int i = 0;
try {
i = Integer.parseInt(s, radix);
} catch (NumberFormatException ex) {
i = defaultInt;
}
return i;
}
public static int parseToInt(String s, int defaultInt) {
int i = 0;
try {
i = Integer.parseInt(s);
} catch (NumberFormatException ex) {
i = defaultInt;
}
return i;
}
public static byte[] hexToByte(String hex)
throws IllegalArgumentException {
if (hex.length() % 2 != 0) {
throw new IllegalArgumentException();
}
char[] arr = hex.toCharArray();
byte[] b = new byte[hex.length() / 2];
for (int i = 0, j = 0, l = hex.length(); i < l; i++, j++) {
String swap = "" + arr[i++] + arr[i];
int byteint = Integer.parseInt(swap, 16) & 0xFF;
b[j] = new Integer(byteint).byteValue();
}
return b;
}
public static String byteToHex(byte b[]) {
if (b == null) {
throw new IllegalArgumentException(
"Argument b ( byte array ) is null! ");
}
String hs = "";
String stmp = "";
for (int n = 0; n < b.length; n++) {
stmp = Integer.toHexString(b[n] & 0xff);
if (stmp.length() == 1) {
hs = hs + "0" + stmp;
} else {
hs = hs + stmp;
}
}
return hs.toUpperCase();
}
public static String byteToHexLowerCase(byte b[]) {
if (b == null) {
throw new IllegalArgumentException(
"Argument b ( byte array ) is null! ");
}
String hs = "";
String stmp = "";
for (int n = 0; n < b.length; n++) {
stmp = Integer.toHexString(b[n] & 0xff);
if (stmp.length() == 1) {
hs = hs + "0" + stmp;
} else {
hs = hs + stmp;
}
}
return hs.toLowerCase();
}
public static byte[] subByte(byte[] input, int startIndex, int length) {
byte[] bt = new byte[length];
for (int i = 0; i < length; i++) {
bt[i] = input[i + startIndex];
}
return bt;
}
}
package com.xxx.utils.sm4;
import java.security.Key;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.SecureRandom;
import java.security.Security;
import java.util.Arrays;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.spec.SecretKeySpec;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.pqc.math.linearalgebra.ByteUtils;
public class SM4Utils {
static {
Security.addProvider(new BouncyCastleProvider());
}
public static final String ALGORITHM_NAME = "SM4";
public static final String ALGORITHM_NAME_ECB_PADDING = "SM4/ECB/PKCS5Padding";
public static final int DEFAULT_KEY_SIZE = 128;
public static final String SECRET_KEY = "xxxxxxxxxx";
static {
Security.addProvider(new BouncyCastleProvider());
}
private static Cipher generateEcbCipher(String algorithmName, int mode, byte[] key) throws Exception {
Cipher cipher = Cipher.getInstance(algorithmName, BouncyCastleProvider.PROVIDER_NAME);
Key sm4Key = new SecretKeySpec(key, ALGORITHM_NAME);
cipher.init(mode, sm4Key);
return cipher;
}
public static byte[] generateKey() throws Exception {
return generateKey(DEFAULT_KEY_SIZE);
}
public static byte[] generateKey(int keySize) throws Exception {
KeyGenerator kg = KeyGenerator.getInstance(ALGORITHM_NAME, BouncyCastleProvider.PROVIDER_NAME);
kg.init(keySize, new SecureRandom());
return kg.generateKey().getEncoded();
}
public static byte[] encryptEcb(String hexKey, byte[] paramStr) throws Exception {
byte[] keyData = ByteUtils.fromHexString(hexKey);
byte[] srcData = paramStr;
byte[] cipherArray = encrypt_Ecb_Padding(keyData, srcData);
return cipherArray;
}
public static String encryptEcbToHexString(String hexKey, byte[] paramStr) throws Exception {
return ConvertUtil.byteToHexLowerCase(encryptEcb(hexKey, paramStr));
}
public static String encryptEcbToHexString(String hexKey, String paramStr) throws Exception {
return ConvertUtil.byteToHexLowerCase(encryptEcb(hexKey, paramStr.getBytes()));
}
public static byte[] encryptEcb(byte[] paramStr) throws Exception {
byte[] keyData = ByteUtils.fromHexString(SECRET_KEY);
byte[] srcData = paramStr;
byte[] cipherArray = encrypt_Ecb_Padding(keyData, srcData);
return cipherArray;
}
public static String encryptEcbToHexString(byte[] paramStr) throws Exception {
return ConvertUtil.byteToHexLowerCase(encryptEcb(paramStr));
}
public static String encryptEcbToHexString(String paramStr) throws Exception {
return ConvertUtil.byteToHexLowerCase(encryptEcb(paramStr.getBytes()));
}
public static byte[] encrypt_Ecb_Padding(byte[] key, byte[] data) throws Exception {
Cipher cipher = generateEcbCipher(ALGORITHM_NAME_ECB_PADDING, Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data);
}
public static byte[] decryptEcb(String hexKey, byte[] cipherText) throws Exception {
byte[] keyData = ByteUtils.fromHexString(hexKey);
byte[] cipherData = cipherText;
byte[] srcData = decrypt_Ecb_Padding(keyData, cipherData);
return srcData;
}
public static String decryptEcbToString(String hexKey, byte[] cipherText) throws Exception {
return new String(decryptEcb(hexKey, cipherText));
}
public static String decryptEcbToString(String hexKey, String cipherText) throws Exception {
return new String(decryptEcb(hexKey, ConvertUtil.hexStringToBytes(cipherText)));
}
public static String decryptEcbToString(byte[] cipherText) throws Exception {
return new String(decryptEcb(SECRET_KEY, cipherText));
}
public static String decryptEcbToString(String cipherText) throws Exception {
return new String(decryptEcb(SECRET_KEY, ConvertUtil.hexStringToBytes(cipherText)));
}
public static byte[] decryptEcb(byte[] cipherText) throws Exception {
byte[] keyData = ByteUtils.fromHexString(SECRET_KEY);
byte[] cipherData = cipherText;
byte[] srcData = decrypt_Ecb_Padding(keyData, cipherData);
return srcData;
}
public static byte[] decrypt_Ecb_Padding(byte[] key, byte[] cipherText) throws Exception {
Cipher cipher = generateEcbCipher(ALGORITHM_NAME_ECB_PADDING, Cipher.DECRYPT_MODE, key);
return cipher.doFinal(cipherText);
}
public static boolean verifyEcb(String hexKey, byte[] cipherText, byte[] paramStr) throws Exception {
boolean flag = false;
byte[] keyData = ByteUtils.fromHexString(hexKey);
byte[] cipherData = cipherText;
byte[] decryptData = decrypt_Ecb_Padding(keyData, cipherData);
byte[] srcData = paramStr;
flag = Arrays.equals(decryptData, srcData);
return flag;
}
}
测试
@Test
public void testSM4() throws Exception {
try {
String data = "心有猛虎,细嗅蔷薇!";
byte[] keyByte = SM4Utils.generateKey();
String key = ConvertUtil.byteToHexLowerCase(keyByte);
System.out.println("key = " + key);
String e = SM4Utils.encryptEcbToHexString(key, data);
System.out.println("加密后:" + e);
String d = SM4Utils.decryptEcbToString(key, e);
System.out.println("解密后:" + d);
} catch (Exception e) {
e.printStackTrace();
}
}
key = 85e615189a7ed0781bd0cf390cfd0348
加密后:c7a8ee117d3eb8078e899a777e90786e7a1cdda1848c55de7bdbc3e7973d8640
解密后:心有猛虎,细嗅蔷薇!
前端
代码
const DECRYPT = 0
const CBC = 0
const ROUND = 32
const BLOCK = 16
const Sbox = [
0xd6, 0x90, 0xe9, 0xfe, 0xcc, 0xe1, 0x3d, 0xb7, 0x16, 0xb6, 0x14, 0xc2, 0x28, 0xfb, 0x2c, 0x05,
0x2b, 0x67, 0x9a, 0x76, 0x2a, 0xbe, 0x04, 0xc3, 0xaa, 0x44, 0x13, 0x26, 0x49, 0x86, 0x06, 0x99,
0x9c, 0x42, 0x50, 0xf4, 0x91, 0xef, 0x98, 0x7a, 0x33, 0x54, 0x0b, 0x43, 0xed, 0xcf, 0xac, 0x62,
0xe4, 0xb3, 0x1c, 0xa9, 0xc9, 0x08, 0xe8, 0x95, 0x80, 0xdf, 0x94, 0xfa, 0x75, 0x8f, 0x3f, 0xa6,
0x47, 0x07, 0xa7, 0xfc, 0xf3, 0x73, 0x17, 0xba, 0x83, 0x59, 0x3c, 0x19, 0xe6, 0x85, 0x4f, 0xa8,
0x68, 0x6b, 0x81, 0xb2, 0x71, 0x64, 0xda, 0x8b, 0xf8, 0xeb, 0x0f, 0x4b, 0x70, 0x56, 0x9d, 0x35,
0x1e, 0x24, 0x0e, 0x5e, 0x63, 0x58, 0xd1, 0xa2, 0x25, 0x22, 0x7c, 0x3b, 0x01, 0x21, 0x78, 0x87,
0xd4, 0x00, 0x46, 0x57, 0x9f, 0xd3, 0x27, 0x52, 0x4c, 0x36, 0x02, 0xe7, 0xa0, 0xc4, 0xc8, 0x9e,
0xea, 0xbf, 0x8a, 0xd2, 0x40, 0xc7, 0x38, 0xb5, 0xa3, 0xf7, 0xf2, 0xce, 0xf9, 0x61, 0x15, 0xa1,
0xe0, 0xae, 0x5d, 0xa4, 0x9b, 0x34, 0x1a, 0x55, 0xad, 0x93, 0x32, 0x30, 0xf5, 0x8c, 0xb1, 0xe3,
0x1d, 0xf6, 0xe2, 0x2e, 0x82, 0x66, 0xca, 0x60, 0xc0, 0x29, 0x23, 0xab, 0x0d, 0x53, 0x4e, 0x6f,
0xd5, 0xdb, 0x37, 0x45, 0xde, 0xfd, 0x8e, 0x2f, 0x03, 0xff, 0x6a, 0x72, 0x6d, 0x6c, 0x5b, 0x51,
0x8d, 0x1b, 0xaf, 0x92, 0xbb, 0xdd, 0xbc, 0x7f, 0x11, 0xd9, 0x5c, 0x41, 0x1f, 0x10, 0x5a, 0xd8,
0x0a, 0xc1, 0x31, 0x88, 0xa5, 0xcd, 0x7b, 0xbd, 0x2d, 0x74, 0xd0, 0x12, 0xb8, 0xe5, 0xb4, 0xb0,
0x89, 0x69, 0x97, 0x4a, 0x0c, 0x96, 0x77, 0x7e, 0x65, 0xb9, 0xf1, 0x09, 0xc5, 0x6e, 0xc6, 0x84,
0x18, 0xf0, 0x7d, 0xec, 0x3a, 0xdc, 0x4d, 0x20, 0x79, 0xee, 0x5f, 0x3e, 0xd7, 0xcb, 0x39, 0x48
]
const CK = [
0x00070e15, 0x1c232a31, 0x383f464d, 0x545b6269,
0x70777e85, 0x8c939aa1, 0xa8afb6bd, 0xc4cbd2d9,
0xe0e7eef5, 0xfc030a11, 0x181f262d, 0x343b4249,
0x50575e65, 0x6c737a81, 0x888f969d, 0xa4abb2b9,
0xc0c7ced5, 0xdce3eaf1, 0xf8ff060d, 0x141b2229,
0x30373e45, 0x4c535a61, 0x686f767d, 0x848b9299,
0xa0a7aeb5, 0xbcc3cad1, 0xd8dfe6ed, 0xf4fb0209,
0x10171e25, 0x2c333a41, 0x484f565d, 0x646b7279
]
function hexToArray(str) {
const arr = []
for (let i = 0, len = str.length; i < len; i += 2) {
arr.push(parseInt(str.substr(i, 2), 16))
}
return arr
}
function ArrayToHex(arr) {
return arr.map(item => {
item = item.toString(16)
return item.length === 1 ? '0' + item : item
}).join('')
}
function utf8ToArray(str) {
const arr = []
for (let i = 0, len = str.length; i < len; i++) {
const point = str.codePointAt(i)
if (point <= 0x007f) {
arr.push(point)
} else if (point <= 0x07ff) {
arr.push(0xc0 | (point >>> 6))
arr.push(0x80 | (point & 0x3f))
} else if (point <= 0xD7FF || (point >= 0xE000 && point <= 0xFFFF)) {
arr.push(0xe0 | (point >>> 12))
arr.push(0x80 | ((point >>> 6) & 0x3f))
arr.push(0x80 | (point & 0x3f))
} else if (point >= 0x010000 && point <= 0x10FFFF) {
i++
arr.push((0xf0 | (point >>> 18) & 0x1c))
arr.push((0x80 | ((point >>> 12) & 0x3f)))
arr.push((0x80 | ((point >>> 6) & 0x3f)))
arr.push((0x80 | (point & 0x3f)))
} else {
arr.push(point)
throw new Error('input is not supported')
}
}
return arr
}
function arrayToUtf8(arr) {
const str = []
for (let i = 0, len = arr.length; i < len; i++) {
if (arr[i] >= 0xf0 && arr[i] <= 0xf7) {
str.push(String.fromCodePoint(((arr[i] & 0x07) << 18) + ((arr[i + 1] & 0x3f) << 12) + ((arr[i + 2] & 0x3f) << 6) + (arr[i + 3] & 0x3f)))
i += 3
} else if (arr[i] >= 0xe0 && arr[i] <= 0xef) {
str.push(String.fromCodePoint(((arr[i] & 0x0f) << 12) + ((arr[i + 1] & 0x3f) << 6) + (arr[i + 2] & 0x3f)))
i += 2
} else if (arr[i] >= 0xc0 && arr[i] <= 0xdf) {
str.push(String.fromCodePoint(((arr[i] & 0x1f) << 6) + (arr[i + 1] & 0x3f)))
i++
} else {
str.push(String.fromCodePoint(arr[i]))
}
}
return str.join('')
}
function rotl(x, y) {
return x << y | x >>> (32 - y)
}
function byteSub(a) {
return (Sbox[a >>> 24 & 0xFF] & 0xFF) << 24 |
(Sbox[a >>> 16 & 0xFF] & 0xFF) << 16 |
(Sbox[a >>> 8 & 0xFF] & 0xFF) << 8 |
(Sbox[a & 0xFF] & 0xFF)
}
function l1(b) {
return b ^ rotl(b, 2) ^ rotl(b, 10) ^ rotl(b, 18) ^ rotl(b, 24)
}
function l2(b) {
return b ^ rotl(b, 13) ^ rotl(b, 23)
}
function sms4Crypt(input, output, roundKey) {
const x = new Array(4)
const tmp = new Array(4)
for (let i = 0; i < 4; i++) {
tmp[0] = input[0 + 4 * i] & 0xff
tmp[1] = input[1 + 4 * i] & 0xff
tmp[2] = input[2 + 4 * i] & 0xff
tmp[3] = input[3 + 4 * i] & 0xff
x[i] = tmp[0] << 24 | tmp[1] << 16 | tmp[2] << 8 | tmp[3]
}
for (let r = 0, mid; r < 32; r += 4) {
mid = x[1] ^ x[2] ^ x[3] ^ roundKey[r + 0]
x[0] ^= l1(byteSub(mid))
mid = x[2] ^ x[3] ^ x[0] ^ roundKey[r + 1]
x[1] ^= l1(byteSub(mid))
mid = x[3] ^ x[0] ^ x[1] ^ roundKey[r + 2]
x[2] ^= l1(byteSub(mid))
mid = x[0] ^ x[1] ^ x[2] ^ roundKey[r + 3]
x[3] ^= l1(byteSub(mid))
}
for (let j = 0; j < 16; j += 4) {
output[j] = x[3 - j / 4] >>> 24 & 0xff
output[j + 1] = x[3 - j / 4] >>> 16 & 0xff
output[j + 2] = x[3 - j / 4] >>> 8 & 0xff
output[j + 3] = x[3 - j / 4] & 0xff
}
}
function sms4KeyExt(key, roundKey, cryptFlag) {
const x = new Array(4)
const tmp = new Array(4)
for (let i = 0; i < 4; i++) {
tmp[0] = key[0 + 4 * i] & 0xff
tmp[1] = key[1 + 4 * i] & 0xff
tmp[2] = key[2 + 4 * i] & 0xff
tmp[3] = key[3 + 4 * i] & 0xff
x[i] = tmp[0] << 24 | tmp[1] << 16 | tmp[2] << 8 | tmp[3]
}
x[0] ^= 0xa3b1bac6
x[1] ^= 0x56aa3350
x[2] ^= 0x677d9197
x[3] ^= 0xb27022dc
for (let r = 0, mid; r < 32; r += 4) {
mid = x[1] ^ x[2] ^ x[3] ^ CK[r + 0]
roundKey[r + 0] = x[0] ^= l2(byteSub(mid))
mid = x[2] ^ x[3] ^ x[0] ^ CK[r + 1]
roundKey[r + 1] = x[1] ^= l2(byteSub(mid))
mid = x[3] ^ x[0] ^ x[1] ^ CK[r + 2]
roundKey[r + 2] = x[2] ^= l2(byteSub(mid))
mid = x[0] ^ x[1] ^ x[2] ^ CK[r + 3]
roundKey[r + 3] = x[3] ^= l2(byteSub(mid))
}
if (cryptFlag === DECRYPT) {
for (let r = 0, mid; r < 16; r++) {
mid = roundKey[r]
roundKey[r] = roundKey[31 - r]
roundKey[31 - r] = mid
}
}
}
function sm4(inArray, key, cryptFlag, {
padding = 'pkcs#5',
mode,
output = 'string'
} = {}) {
if (mode === CBC) {
}
if (typeof key === 'string') key = hexToArray(key)
if (key.length !== (128 / 8)) {
throw new Error('key is invalid')
}
if (typeof inArray === 'string') {
if (cryptFlag !== DECRYPT) {
inArray = utf8ToArray(inArray)
} else {
inArray = hexToArray(inArray)
}
} else {
inArray = [...inArray]
}
if (padding === 'pkcs#5' && cryptFlag !== DECRYPT) {
const paddingCount = BLOCK - inArray.length % BLOCK
for (let i = 0; i < paddingCount; i++) inArray.push(paddingCount)
}
const roundKey = new Array(ROUND)
sms4KeyExt(key, roundKey, cryptFlag)
const outArray = []
let restLen = inArray.length
let point = 0
while (restLen >= BLOCK) {
const input = inArray.slice(point, point + 16)
const output = new Array(16)
sms4Crypt(input, output, roundKey)
for (let i = 0; i < BLOCK; i++) {
outArray[point + i] = output[i]
}
restLen -= BLOCK
point += BLOCK
}
if (padding === 'pkcs#5' && cryptFlag === DECRYPT) {
const paddingCount = outArray[outArray.length - 1]
outArray.splice(outArray.length - paddingCount, paddingCount)
}
if (output !== 'array') {
if (cryptFlag !== DECRYPT) {
return ArrayToHex(outArray)
} else {
return arrayToUtf8(outArray)
}
} else {
return outArray
}
}
调用
sm4('内容', '与后端一致的key', 1)
在线工具测试
在线SM4加密/解密
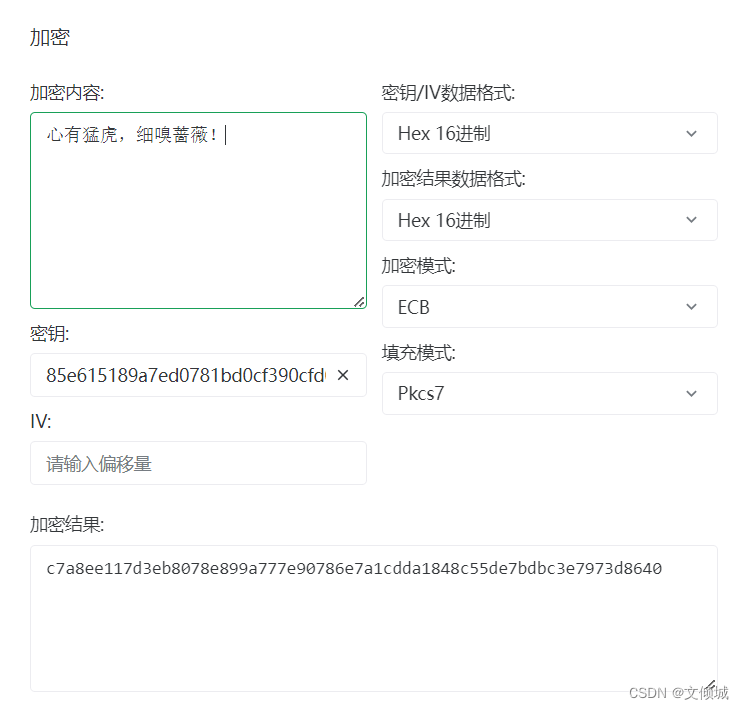