1 整合MyBatis Demo
1)新建springboot工程
2)添加web,msql,mybatis依赖
<dependencies>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.0.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/druid -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.17</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
org.mybatis.spring.boot mybatis‐spring‐boot‐starter 1.3.1 MyBatis包中自动包含jdbc的依赖,所以不用手动引入jdbc 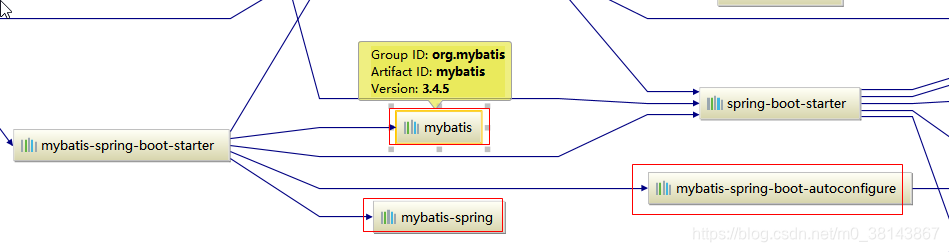
3)添加数据库以及druid数据源配置
spring.datasource.password=xxx
spring.datasource.username=xxx
spring.datasource.url=jdbc:mysql://1ip:3306/mybatis
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
spring.datasource.schema=classpath:department.sql,classpath:employee.sql
spring.datasource.initialization-mode=always
#数据源其他配置
spring.datasource.initialSize=5
spring.datasource.minIdle=5
spring.datasource.maxActive=20
spring.datasource.maxWait=60000
spring.datasource.timeBetweenEvictionRunsMillis=60000
spring.datasource.minEvictableIdleTimeMillis=300000
spring.datasource.validationQuery=SELECT 1 FROM DUAL
spring.datasource.testWhileIdle=true
spring.datasource.testOnBorrow=false
spring.datasource.testOnReturn=false
spring.datasource.poolPreparedStatements=true
#配置监控统计拦截的filters,去掉后监控界面sql无法统计,'wall’用于防火墙
spring.datasource.filters=stat,wall,log4j
spring.datasource.maxPoolPreparedStatementPerConnectionSize=20
spring.datasource.useGlobalDataSourceStat=true
spring.datasource.connectionProperties=druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
4)类路径下新建 department.sql,employee.sql
5)编写druid配置类
@Configuration
public class DruidConfig {
@ConfigurationProperties(prefix="spring.datasource")
@Bean
public DataSource druid(){
return new DruidDataSource();
}
@Bean
public ServletRegistrationBean getServletRegistrationBean(){
ServletRegistrationBean druidServletRegistrationBean=new ServletRegistrationBean(
new StatViewServlet(),"/druid/*");
Map<String,String> initParams=new HashMap<>();
initParams.put("loginUsername","admin");//登录用户密码
initParams.put("loginPassword","123456");
initParams.put("allow","");//默认就是允许所有访问
initParams.put("deny","192.168.15.21");//拒绝访问
druidServletRegistrationBean.setInitParameters(initParams);
return druidServletRegistrationBean;
}
@Bean
public FilterRegistrationBean getFilterRegistrationBean(){
FilterRegistrationBean filterRegistrationBean=new FilterRegistrationBean();
filterRegistrationBean.setFilter(new StatViewFilter());
Map<String,String> initParams=new HashMap<>();
initParams.put("exclusions","*.js,*.css,/druid/*"); //不做拦截
filterRegistrationBean.setInitParameters(initParams);
filterRegistrationBean.setUrlPatterns(Arrays.asList("/*"));
return filterRegistrationBean;
}
}
6)新建Department ,Employee文件
7)mybatis两种方式
自动配置MybatisAutoConfiguration MybatisProperties
a)注解模式:只需要编写 DepartmentMapper
1)编写 DepartmentMapper 文件
@Mapper
public interface DepartmentMapper {
@Select("select * from department where id=#{id}")
public Department getDeptById(Integer id);
@Delete("delete from department where id=#{id}")
public void deleteDeptById(Integer id);
//useGeneratedKeys = true,是否使用自动生成的主键 keyProperty = "id" Department 的id作为主键字段
@Options(useGeneratedKeys = true,keyProperty = "id")
@Insert("insert into department(departmentName) values(#{departmentName})")
public int insertDept(Department dept);
@Update("update department set departmentName=#{departmentName} where id=#{id}")
public void updateDept(Department dept);
}
2)编写DeptmentController
@RestController
public class DeptmentController {
@Autowired
private DepartmentMapper departmentMapper;
@GetMapping("/dept/{id}")
public Department getDepartmentById(@PathVariable("id") Integer id){
return departmentMapper.getDeptById(id);
}
@GetMapping("/dept")
public Department insertDept(Department dept){
departmentMapper.insertDept(dept);
return dept;
}
}
3)测试 数据添加成功
4)修改数据库字段为驼峰命名之后 发生异常
5)修改DepartmentMapper 中字段为department_name
查询结果departmentName字段并没有装配
6)自定义MyBatis的配置规则;给容器中添加一个ConfigurationCustomizer
@org.springframework.context.annotation.Configuration
public class MyBatisConfig {
@Bean
public ConfigurationCustomizer configurationCustomizer(){
return new ConfigurationCustomizer(){
@Override
public void customize(Configuration configuration) {
configuration.setMapUnderscoreToCamelCase(true);//开启驼峰命名
}
};
}
}
测试查询结果
7)为了避免每个xxxMapper类都加@Mapper注解;可以在启动类上配置扫描的mapper文件的包
b) 配置文件模式
1)新建EmployeeMapper
public interface EmployeeMapper {
public void insertEmp(Employee employee);
public Employee getEmployeeById(Integer id);
}
2)在类路径下新建mybatis文件夹,在mybatis下新建mybatis-config.xml配置文件并进行编辑(参考https://github.com/mybatis/spring-boot-starter/blob/master/mybatis-spring-boot-samples/mybatis-spring-boot-sample-xml/src/main/resources/mybatis-config.xml)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
#设置驼峰命名匹配
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
</configuration>
3)在mybatis文件夹下新建mapper文件,在mapper文件下新建EmployeeMapper.xml文件并进行编辑(参考https://github.com/mybatis/spring-boot-starter/blob/master/mybatis-spring-boot-samples/mybatis-spring-boot-sample-xml/src/main/resources/sample/mybatis/mapper/HotelMapper.xml)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.tang.webmybatis.mapper.EmployeeMapper">
<select id="getEmployee" resultType="com.tang.webmybatis.bean.Employee" parameterType="java.lang.Integer">
select * from employee where id = #{id}
</select>
<insert id="insertEmp">
insert into employee(lastName,email,gender,d_id) VALUES (#{lastName},#{email},#{gender},#{d_id})
</insert>
</mapper>
4)添加mybatis配置
#指定全局配置文件的位置
mybatis.config‐location=classpath:mybatis/mybatis-config.xml
#指定sql映射文件的位置
mybatis.mapper‐locations=classpath:mybatis/mapper/*.xml
打印sql日志配置
logging.level.com.xxx.mapper(具体的mapper包名com.tang.webcache.mapper)=debug
或者logging.level.root=debug可以查看sql查询语句
5)测试
更多使用参照
http://www.mybatis.org/spring-boot-starter/mybatis-spring-boot-autoconfigure/