一、内容简介:
- File类
- 文件字节输入输出流
- 文件字符输入输出流
- 缓冲流
- 随机流
- 数组流
- 数据流
- 对象流
- 序列化与对象克隆
- 使用Scanner解析文件
- 文件锁
二、输入输出流基础
1.File类
知识点
- 创建File类的构造方法:
File(String Filename);
File(String directoryPath,String filename)
File(File dir,String filename)
- File类的常用方法
public String getName()
:获取文件名字public boolean canRead()
:判断文件是否可读public boolean canWrite()
:判断文件是否可被写入public boolean exists()
:判断文件是否存在public long length()
:获取文件长度public String getAbsolutePath()
:获取文件的绝对路径public String getParent()
:获取文件的父目录public boolean isFile()
:判断是否是一个文件public boolean isDirectory()
:判断是否是一个目录public boolean isHidden()
:判断文件是否是隐藏文件public long lastModified()
:获取文件最后修改时间(毫秒数)
代码
package edu.moth12;
import java.io.File;
public class test {
public static void main(String[] args) {
File file = new File("D:\\java");
getAllFile(file);
}
public static void getAllFile(File dir){
File[] files = dir.listFiles();
for (File f:
files) {
if(f.isDirectory())getAllFile(f);
else{
String s = f.toString();
boolean b = s.endsWith(".java");
if(b) System.out.println(f);
}
}
}
}
- 运行结果:
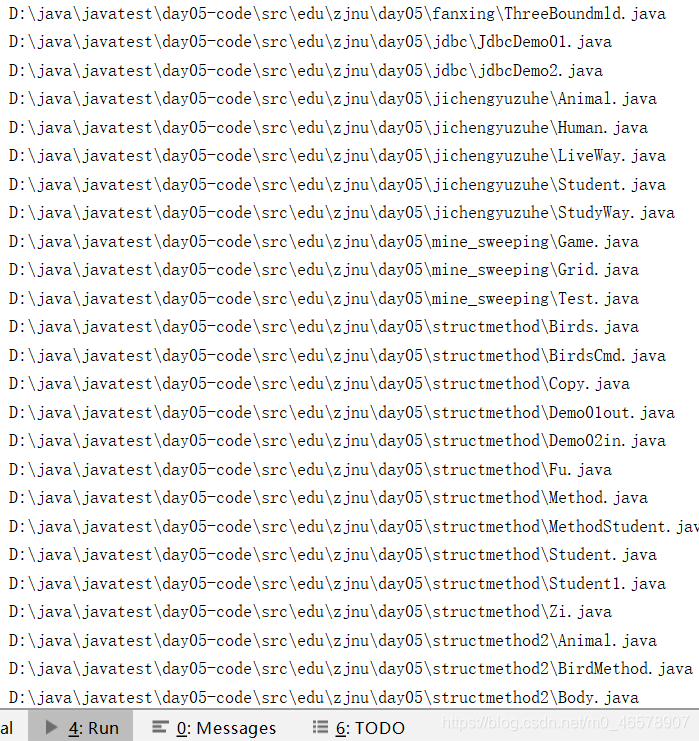
2. 文件字节输入输出流
知识点
代码
package edu.moth12.In_OutStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class FileInput_Stream {
public static void main(String[] args) {
int n = -1;
byte [] a = new byte[100];
try{
File f = new File("D:\\data.txt");
InputStream in = new FileInputStream(f);
while((n = in.read(a,0,100)) != -1){
String s = new String(a,0,n);
System.out.print(s);
}
in.close();
}catch(IOException e){
System.out.println("File read error" + e);
}
}
}
- 运行结果
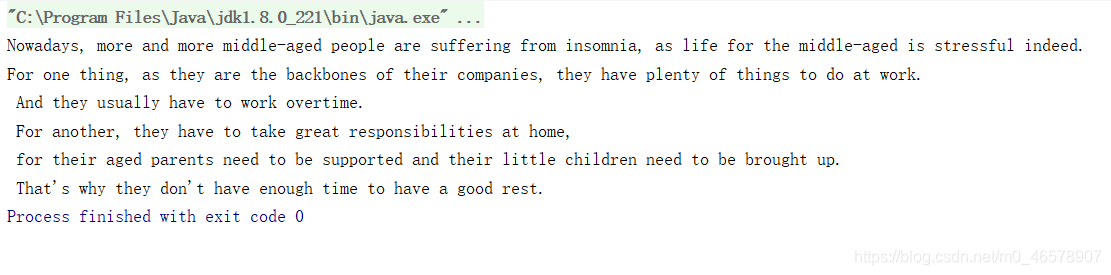
package edu.moth12.In_OutStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FileOutput_Stream {
public static void main(String[] args) {
byte [] a = "新年快乐".getBytes();
byte [] b = "Happy new year".getBytes();
File file = new File("data.txt");
try{
OutputStream out = new FileOutputStream(file);
System.out.println(file.getName()+"的大小:"+file.length()+"字节");
out.write(a);
out.close();
out = new FileOutputStream(file,true);
System.out.println(file.getName()+"的大小:"+file.length()+"字节");
out.write(b,0,b.length);
System.out.println(file.getName()+"的大小:"+file.length()+"字节");
out.close();
}catch (IOException e){
System.out.println("Error:"+e);
}
}
}
- 运行结果
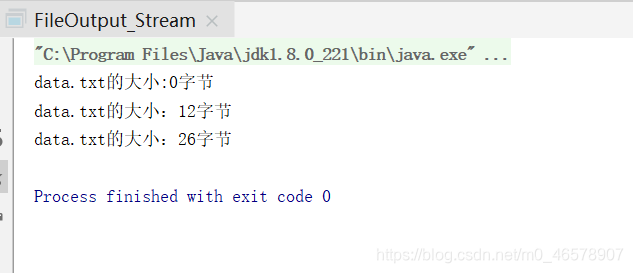
3. 文件字符输入、输出流
知识点
代码
package edu.moth12.In_OutStream;
import java.io.*;
public class FileReader_Writer {
public static void main(String[] args) {
File sourceFile = new File("D:\\a.txt");
File targetFile = new File("D:\\b.txt");
char c[] = new char[19];
try {
Writer out = new FileWriter(targetFile, true);
Reader in = new FileReader(sourceFile);
int n = -1;
while ((n = in.read(c)) != -1) {
out.write(c, 0, n);
}
out.flush();
out.close();
} catch (IOException e) {
System.out.println("Error:" + e);
}
}
}
- 运行结果
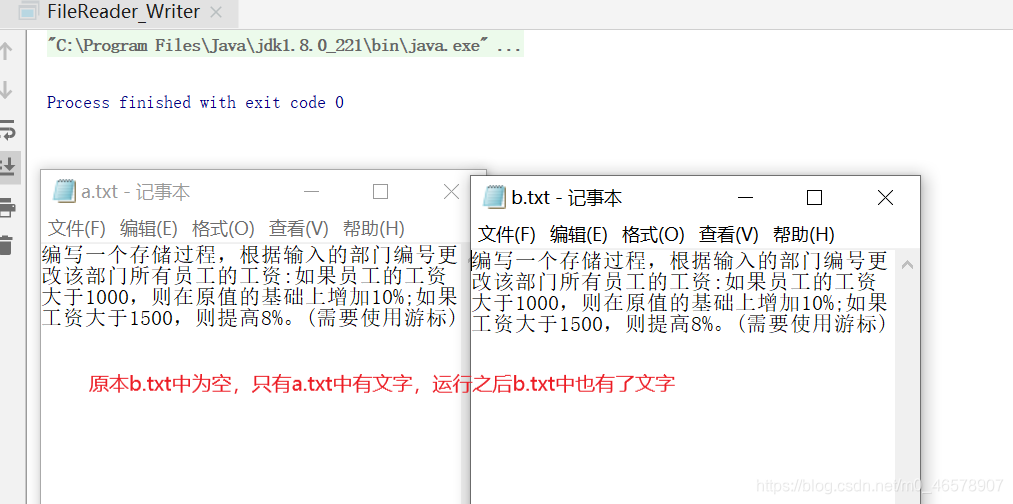
4. 缓冲流
知识点
代码
package edu.moth12.In_OutStream;
import java.io.*;
import java.util.StringTokenizer;
public class Buffered_Reader {
public static void main(String[] args) {
File fRead = new File("D:\\a.txt");
File fWrite = new File("D:\\b.txt");
try{
Writer out = new FileWriter(fWrite);
BufferedWriter bufferedWriter = new BufferedWriter(out);
Reader in = new FileReader(fRead);
BufferedReader bufferedReader = new BufferedReader(in);
String str = null;
while((str = bufferedReader.readLine())!=null){
StringTokenizer fenxi = new StringTokenizer(str);
int count = fenxi.countTokens();
str = str+"句子中的单词个数:"+count;
bufferedWriter.write(str);
bufferedWriter.newLine();
}
bufferedWriter.close();
out.close();
in = new FileReader(fWrite);
bufferedReader = new BufferedReader(in);
String s = null;
System.out.println(fWrite.getName()+"内容:");
while ((s = bufferedReader.readLine())!=null){
System.out.println(s);
}
bufferedReader.close();
in.close();
}catch (IOException e){
System.out.println(e.toString());
}
}
}
- 运行结果
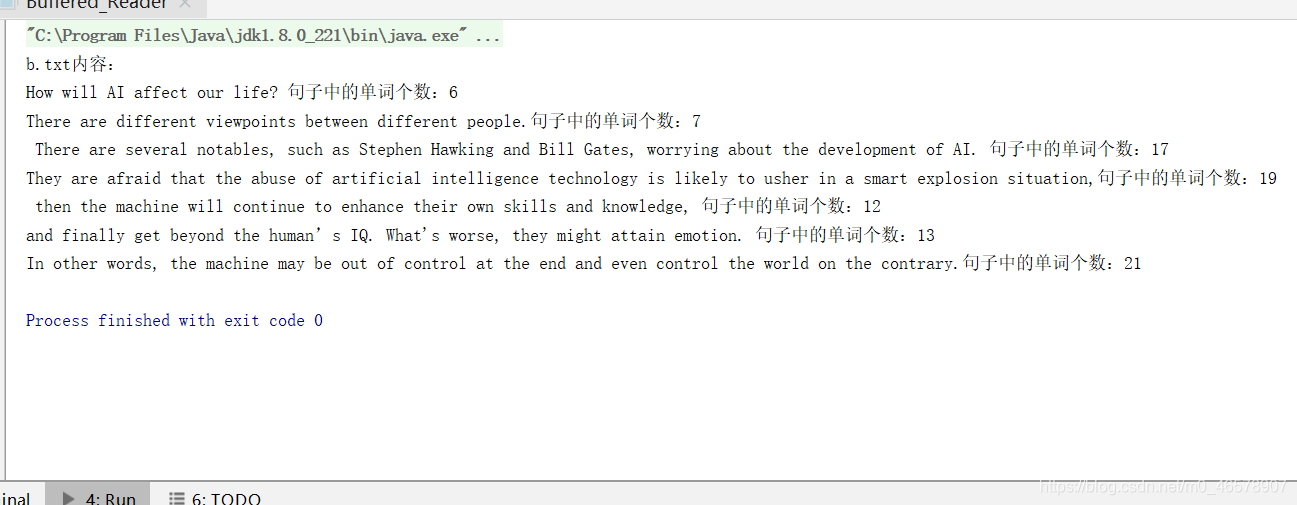
5. 随机流
知识点
代码
package edu.moth12.In_OutStream;
import java.io.IOException;
import java.io.RandomAccessFile;
public class Random_01 {
public static void main(String[] args) {
RandomAccessFile inAndOut = null;
int data[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
try {
inAndOut = new RandomAccessFile("D:\\a.txt","rw");
for (int i = data.length; i >= 0; i--) {
inAndOut.seek(i*4);
System.out.printf("\t%d",inAndOut.readInt());
}
inAndOut.close();
}catch (IOException e){
}
}
}

6. 数组流
知识点
代码
package edu.moth12.In_OutStream;
import java.io.*;
public class ByteArrayOutput_Stream {
public static void main(String[] args) {
try{
ByteArrayOutputStream outByte = new ByteArrayOutputStream();
byte [] byteContent = "mid-autumn festival ".getBytes();
outByte.write(byteContent);
ByteArrayInputStream inByte = new ByteArrayInputStream(outByte.toByteArray());
byte backByte [] = new byte[outByte.toByteArray().length];
inByte.read(backByte);
System.out.println(new String(backByte));
CharArrayWriter outChar = new CharArrayWriter();
char [] charContent = "中秋快乐".toCharArray();
outChar.write(charContent);
CharArrayReader inChar = new CharArrayReader(outChar.toCharArray());
char backChar [] = new char[outChar.toCharArray().length];
inChar.read(backChar);
System.out.println(new String(backChar));
}catch (IOException e){
}
}
}
7. 数据流
知识点
代码
8.对象流
知识点
代码
9. 序列化与克隆对象
知识点
代码
10. 使用Scanner解析文本
知识点
代码
11. 文件锁
知识点
代码