任务目标
- 需求
-使用网络编程,IO流,序列化流,多线程实现C\S结构的学生信息管理系统
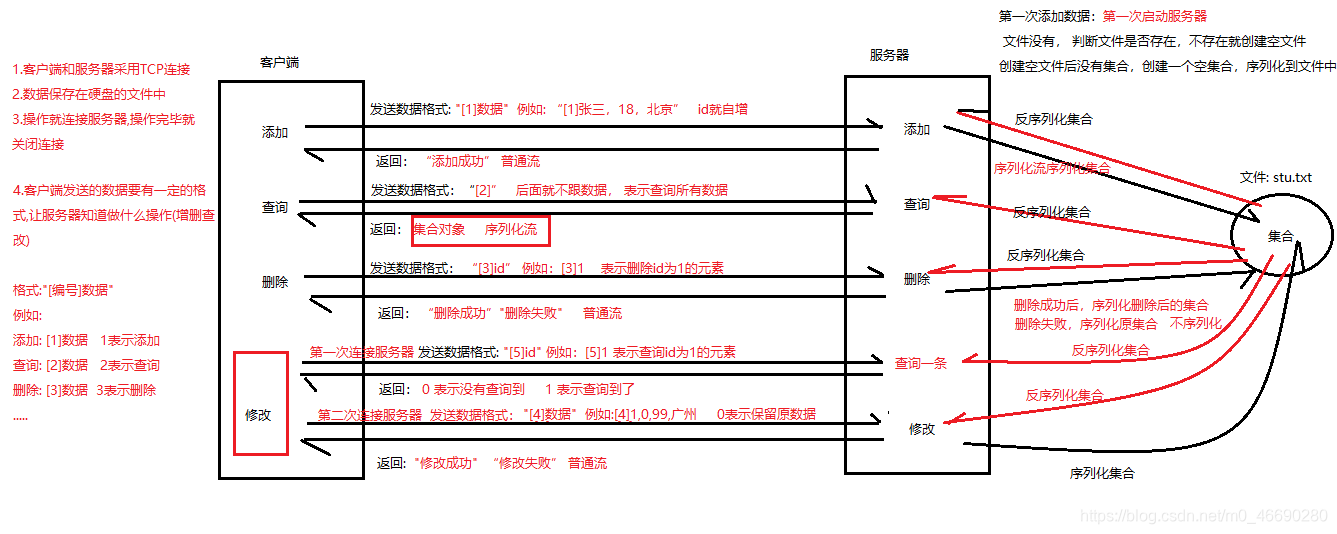
思路分析:
1.对象需要用到序列化,创建的学生类实现Serializable接口
2.保存在文件,第一次用文件有没有,没有需创建,同时如果防止没有数据的时候读取报错,需要创建一个空集合,序列化到文件中.
3.服务器和客户端约定好一种格式 比如添加[1]数据 表示添加 传输的格式[1]张三,18,北京
4.id需要自增,需用到静态代码块,读取文件,拿到list的长度来编号
5.客户端发送请求送,服务器需先从文件中序列化集合出来,操作完后重写上去(数据覆盖)
6.修改比较特殊,先判断id是否存在,存在的情况需再次链接数据库发送格式数据,进行修改
服务器端
1.服务器的菜单
public class Server {
public static void main(String[] args) {
try {
ServerSocket ss = new ServerSocket(8888);
while (true){
Socket socket = ss.accept();
InputStream is = socket.getInputStream();
byte[] bys = new byte[1024];
int len = is.read(bys);
String message = new String(bys,0,len);
char op = message.charAt(1);
switch (op){
case '1':
break;
case '2':
break;
case '3':
break;
case '4':
break;
case '5':
break;
default:
System.out.println("格式错误!");
socket.close();
break;
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
2.服务器的添加功能
private static void addStudent(Socket socket,String message) {
try {
FileInputStream fis = new FileInputStream("day25\\stu.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
String[] arr = message.substring(3).split(",");
String name = arr[0];
int age = Integer.parseInt(arr[1]);
String address = arr[2];
Student stu = new Student(++Utils.id,name,age,address);
list.add(stu);
FileOutputStream fos = new FileOutputStream("day25\\stu.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
OutputStream os = socket.getOutputStream();
os.write("添加成功".getBytes());
fos.close();
fis.close();
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
3.服务器第一次启动创建文件,并写入一个空集合对象到文件中
public class Server {
public static void main(String[] args) {
try {
File file = new File("day25\\stu.txt");
if (!file.exists()){
file.createNewFile();
}
FileInputStream fis = new FileInputStream(file);
ObjectInputStream ois = new ObjectInputStream(fis);
ois.close();
} catch (Exception e) {
try {
FileOutputStream fos = new FileOutputStream("day25\\stu.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
ArrayList<Student> list = new ArrayList<>();
oos.writeObject(list);
oos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
4.服务器的查询功能
private static void selectStudent(Socket socket, String message) {
try {
FileInputStream fis = new FileInputStream("day25\\stu.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
OutputStream os = socket.getOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(os);
oos.writeObject(list);
ois.close();
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
5.服务器的删除功能
private static void deleteStudent(Socket socket, String message) {
String msg = message.substring(3);
int id = Integer.parseInt(msg);
try {
FileInputStream fis = new FileInputStream("day25\\stu.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
boolean flag = false;
for (int i = 0; i < list.size(); i++) {
Student stu = list.get(i);
if (stu.getId() == id){
list.remove(i);
flag = true;
FileOutputStream fos = new FileOutputStream("day25\\stu.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
break;
}
}
OutputStream os = socket.getOutputStream();
if (flag == true){
os.write("删除成功".getBytes());
}else {
os.write("删除失败".getBytes());
}
ois.close();
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
6.服务器查询一条数据
private static void selectById(Socket socket, String message) {
String msg = message.substring(3);
int id = Integer.parseInt(msg);
try {
FileInputStream fis = new FileInputStream("day25\\stu.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
boolean flag = false;
for (int i = 0; i < list.size(); i++) {
Student stu = list.get(i);
if (stu.getId() == id) {
flag = true;
break;
}
}
OutputStream os = socket.getOutputStream();
if (flag == false){
os.write(0);
}else {
os.write(1);
}
ois.close();
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
7.服务器修改功能
private static void updateStudent(Socket socket, String message) {
String msg = message.substring(3);
String[] arr = msg.split(",");
int id = Integer.parseInt(arr[0]);
String name = arr[1];
int age = Integer.parseInt(arr[2]);
String address = arr[3];
try {
FileInputStream fis = new FileInputStream("day25\\stu.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
boolean flag = false;
for (int i = 0; i < list.size(); i++) {
Student stu = list.get(i);
if (stu.getId() == id){
if (!"0".equals(name)){
stu.setName(name);
}
if (!"0".equals(arr[2])){
stu.setAge(age);
}
if (!"0".equals(address)){
stu.setName(address);
}
flag = true;
break;
}
}
System.out.println(list);
FileOutputStream fos = new FileOutputStream("day25\\stu.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
oos.close();
OutputStream os = socket.getOutputStream();
if (flag == true){
os.write("修改成功".getBytes());
}else{
os.write("修改失败".getBytes());
}
socket.close();
ois.close();
} catch (Exception e) {
e.printStackTrace();
}
}
客户端
1.客户端的菜单
public class Client {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (true){
System.out.println("******************************************************");
System.out.println("1.添加学员\t2.查询学员\t3.删除学员\t4.修改学员\t5.退出");
System.out.println("******************************************************");
System.out.println("请输入功能序号:");
int op = sc.nextInt();
switch (op){
case 1:
break;
case 2:
break;
case 3:
break;
case 4:
break;
case 5:
System.out.println("谢谢使用,欢迎下次再来");
System.exit(0);
}
}
}
}
2.客户端的添加功能
private static void addStudent() {
Scanner sc = new Scanner(System.in);
System.out.println("请输入学员姓名:");
String name = sc.next();
System.out.println("请输入学员年龄:");
int age = sc.nextInt();
System.out.println("请输入学员地址:");
String address = sc.next();
String msg = "[1]"+name+","+age+","+address;
try {
Socket socket = new Socket("127.0.0.1",8888);
OutputStream os = socket.getOutputStream();
os.write(msg.getBytes());
InputStream is = socket.getInputStream();
byte[] bys = new byte[1024];
int len = is.read(bys);
System.out.println(new String(bys,0,len));
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
3.客户端的查询功能
private static void selectStudent() {
String message = "[2]";
try {
Socket socket = new Socket("127.0.0.1",8888);
OutputStream os = socket.getOutputStream();
os.write(message.getBytes());
InputStream is = socket.getInputStream();
ObjectInputStream ois = new ObjectInputStream(is);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
if (list.size() == 0){
System.out.println("没有数据");
}else{
for (Student stu : list) {
System.out.println(stu);
}
}
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
4.客户端的删除功能
private static void deleteStudent() {
Scanner sc = new Scanner(System.in);
System.out.println("请输入学员id:");
int id = sc.nextInt();
String message = "[3]"+id;
try {
Socket socket = new Socket("127.0.0.1",8888);
OutputStream os = socket.getOutputStream();
os.write(message.getBytes());
InputStream is = socket.getInputStream();
byte[] bys = new byte[1024];
int len = is.read(bys);
System.out.println(new String(bys,0,len));
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
5.客户端的修改功能
private static void updateStudent() {
Scanner sc = new Scanner(System.in);
System.out.println("请输入学员id:");
int id = sc.nextInt();
String message = "[5]"+id;
try {
Socket socket = new Socket("127.0.0.1",8888);
OutputStream os = socket.getOutputStream();
os.write(message.getBytes());
InputStream is = socket.getInputStream();
int len = is.read();
if (len == 0){
System.out.println("没有改id,修改失败");
socket.close();
return;
}else{
socket.close();
try {
System.out.println("请输入需要修改的姓名,0表示不修改:");
String name = sc.next();
System.out.println("请输入需要修改的年龄,0表示不修改:");
int age = sc.nextInt();
System.out.println("请输入需要修改的地址,0表示不修改:");
String address = sc.next();
String msg = "[4]"+id+","+name+","+age+","+address;
Socket socket2 = new Socket("127.0.0.1",8888);
OutputStream os2 = socket2.getOutputStream();
os2.write(msg.getBytes());
InputStream is2 = socket2.getInputStream();
byte[] bys = new byte[1024];
int len2 = is2.read(bys);
System.out.println(new String(bys,0,len2));
socket2.close();
} catch (Exception e) {
e.printStackTrace();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
6.服务器多线程
- 如果有多个客户端连接服务器,那么服务器接收请求后,应该开辟多条线程来执行不同客户端的任务
while (true){
Socket socket = ss.accept();
new Thread(()->{
}).start();
}
工具类
1.学生类
public class Student implements Serializable {
private int id;
private String name;
private int age;
private String address;
public Student() {
}
public Student(int id, String name, int age, String address) {
this.id = id;
this.name = name;
this.age = age;
this.address = address;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
", address='" + address + '\'' +
'}';
}
}
最后的实现代码
目录
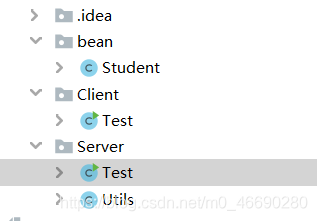
Student
public class Student implements Serializable {
private int id;
private String name;
private int age;
private String adress;
public Student(int id, String name, int age, String adress) {
this.id = id;
this.name = name;
this.age = age;
this.adress = adress;
}
public Student() {
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getAdress() {
return adress;
}
public void setAdress(String adress) {
this.adress = adress;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
", adress='" + adress + '\'' +
'}';
}
}
Client
public class Test {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (true) {
System.out.println("**********************************************");
System.out.println("1.添加学员\t2.查询学员\t3.删除学员\t4.修改学员\t5.退出系统");
System.out.println("**********************************************");
int op = sc.nextInt();
switch (op) {
case 1:addStudent(sc);
break;
case 2:findStudent();
break;
case 3:deleteStudent(sc);
break;
case 4:updateStudent(sc);
break;
case 5:
System.out.println("谢谢使用");
System.exit(0);
}
}
}
private static void updateStudent(Scanner sc) {
try {
Socket socket = new Socket("localhost", 8888);
OutputStream os = socket.getOutputStream();
System.out.println("请输入要修改的学生id");
int id = sc.nextInt();
os.write(("[5]" + id).getBytes());
InputStream is = socket.getInputStream();
int read = is.read();
if (read==0){
System.out.println("没有查询到要修改的id");
socket.close();
return;
}else {
socket.close();
try {
System.out.println("请输入要修改的学生姓名,如输入0视为保存原值");
String name = sc.next();
System.out.println("请输入要修改的学生年龄,如输入0视为保存原值");
int age = sc.nextInt();
System.out.println("请输入要修改的学生地址,如输入0视为保存原值");
String adress = sc.next();
String s="[4]"+id+","+name+","+age+","+adress;
Socket socket2 = new Socket("localhost", 8888);
OutputStream os2 = socket2.getOutputStream();
os2.write(s.getBytes());
socket2.shutdownOutput();
InputStream is2 = socket2.getInputStream();
byte[]bytes=new byte[1024];
int read2 = is2.read(bytes);
System.out.println(new String(bytes,0,read2));
socket2.close();
}catch (Exception e){
e.printStackTrace();
}
}
}catch (Exception e){e.printStackTrace();}
}
private static void deleteStudent(Scanner sc) {
try {
Socket socket = new Socket("localhost", 8888);
OutputStream os = socket.getOutputStream();
System.out.println("请输入要删除的学生id");
int id = sc.nextInt();
os.write(("[3]"+id).getBytes());
socket.shutdownOutput();
InputStream is = socket.getInputStream();
byte[]bytes=new byte[1024];
int read = is.read(bytes);
System.out.println(new String(bytes,0,read));
socket.close();
}catch (Exception e){
}
}
private static void findStudent() {
try {
Socket socket = new Socket("localhost", 8888);
OutputStream os = socket.getOutputStream();
os.write("[2]".getBytes());
InputStream is = socket.getInputStream();
ObjectInputStream ois = new ObjectInputStream(is);
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
for (Student student : list) {
System.out.println(student);
}
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static void addStudent(Scanner sc) {
System.out.println("请输入要添加的学员姓名");
String name = sc.next();
System.out.println("请输入要添加的学员年龄");
int age = sc.nextInt();
System.out.println("请输入要添加的学员地址");
String adress = sc.next();
String message="[1]"+name+","+age+","+adress;
try {
Socket socket=new Socket("localhost",8888);
socket.getOutputStream().write(message.getBytes());
byte[]bys=new byte[1024];
int len = socket.getInputStream().read(bys);
System.out.println(new String(bys,0,len));
socket.close();
}catch (Exception e){}
}
}
Server
public class Test {
public static void main(String[] args) {
try {
File file = new File("stu.txt");
if (!file.exists()) {
file.createNewFile();
}
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(file));
ois.close();
} catch (Exception e) {
try {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("stu.txt"));
ArrayList<Student> list = new ArrayList<>();
oos.writeObject(list);
oos.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
try {
ServerSocket serverSocket = new ServerSocket(8888);
while (true) {
Socket accept = serverSocket.accept();
new Thread(() -> {
try {
byte[] bytes = new byte[1024];
int len = accept.getInputStream().read(bytes);
String message = new String(bytes, 0, len);
char op = message.charAt(1);
switch (op) {
case '1':
addStudent(accept, message);
break;
case '2':
findStudent(accept);
break;
case '3':
deleteStudent(accept, message);
break;
case '4':
updateStudent(accept, message);
break;
case '5':
findOneStudent(accept, message);
break;
default://关闭
System.out.println("格式错误");
accept.close();
break;
}
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static void updateStudent(Socket accept, String message) {
try {
String[] split = message.substring(3).split(",");
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("stu.txt"));
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
Boolean flag = false;
for (int i = 0; i < list.size(); i++) {
Student student = list.get(i);
if (student.getId() == Integer.parseInt(split[0])) {
if (!"0".equals(split[1])) {
student.setName(split[1]);
}
if (!"0".equals(split[2])) {
student.setAge(Integer.parseInt(split[2]));
}
if (!"0".equals(split[3])) {
student.setAdress(split[3]);
}
flag = true;
break;
}
}
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("stu.txt"));
oos.writeObject(list);
oos.close();
OutputStream os = accept.getOutputStream();
if (flag == true) {
os.write("修改成功".getBytes());
} else {
os.write("修改失败".getBytes());
}
accept.shutdownOutput();
accept.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static void findOneStudent(Socket accept, String message) {
try {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("stu.txt"));
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
Integer c = Integer.parseInt(message.substring(3));
OutputStream os = accept.getOutputStream();
Boolean flag = false;
for (Student student : list) {
if (student.getId() == c) {
flag = true;
break;
}
}
if (flag == true) {
os.write(1);
} else {
os.write(0);
}
ois.close();
accept.close();
} catch (Exception e) {
}
}
private static void deleteStudent(Socket accept, String message) {
try {
String substring = message.substring(3);
int id = Integer.parseInt(substring);
ObjectInputStream os = new ObjectInputStream(new FileInputStream("stu.txt"));
ArrayList<Student> list = (ArrayList<Student>) os.readObject();
boolean flag = false;
for (int i = 0; i < list.size(); i++) {
Student student = list.get(i);
if (student.getId() == id) {
list.remove(i);
flag = true;
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("stu.txt"));
oos.writeObject(list);
break;
}
}
OutputStream outputStream = accept.getOutputStream();
if (flag == true) {
outputStream.write("删除成功".getBytes());
} else {
outputStream.write("找不到要删除的ID,删除失败".getBytes());
}
accept.shutdownOutput();
os.close();
accept.close();
} catch (Exception e) {
}
}
private static void findStudent(Socket accept) {
try {
ObjectInputStream os = new ObjectInputStream(new FileInputStream("stu.txt"));
ArrayList<Student> list = (ArrayList<Student>) os.readObject();
ObjectOutputStream oos = new ObjectOutputStream(accept.getOutputStream());
oos.writeObject(list);
accept.shutdownOutput();
accept.close();
} catch (Exception e) {
}
}
private static void addStudent(Socket accept, String message) {
try {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("stu.txt"));
ArrayList<Student> list = (ArrayList<Student>) ois.readObject();
String[] split = message.substring(3).split(",");
Student student = new Student(++Utils.id, split[0], Integer.parseInt(split[1]), split[2]);
list.add(student);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("stu.txt"));
oos.writeObject(list);
OutputStream os = accept.getOutputStream();
os.write("添加成功".getBytes());
os.close();
accept.close();
} catch (Exception e) {
}
}
}
Utils
public class Utils {
public static int id=0;
static {
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("stu.txt"))) {
ArrayList<Student>list = (ArrayList<Student>) ois.readObject();
if (list.size()==0){
id=0;
}else {
id = list.get(list.size() - 1).getId();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}