基于 JavaSE + MySQL+ JDBC 的学生信息管理系统
一、技术栈
二、功能分析
2.1 登录菜单
2.2 主菜单
- 添加学生信息
- 删除学生信息
- 修改学生信息(指定字段)
- 修改学生信息(所有信息)
- 查找学生信息
- 显示学生信息(全体学生)
- 清空学生信息
- 导出学生信息
- 有两种导出方式,一种是
自定义导出
,另一种是默认导出
- 注销账户
- 识别当前管理者信息,只能
注销当前登录的管理者信息
,注销成功后退出登录状态,返回到未登录界面
- 退出登录
三、数据库设计
3.1 学生表(student)设计
字段名 | 说明 | 类型 | 主键 | 外键 | 非空 | 唯一 | 自增 |
---|
id | 学生编号 | int(10) | 是 | 否 | 是 | 是 | 是 |
student_id | 学号 | varchar(11) | 否 | 否 | 是 | 是 | 否 |
student_name | 姓名 | varchar(20) | 否 | 否 | 是 | 否 | 否 |
student_sex | 性别 | varchar(4) | 否 | 否 | 是 | 否 | 否 |
student_age | 年龄 | int(5) | 否 | 否 | 是 | 否 | 否 |
student_phone | 电话 | varchar(15) | 否 | 否 | 否 | 是 | 否 |
student_location | 住址 | varchar(45) | 否 | 否 | 是 | 否 | 否 |
student_card | 身份证号码 | varchar(18) | 否 | 否 | 是 | 是 | 否 |
student_english | 英语成绩 | double | 否 | 否 | 是 | 否 | 否 |
student_math | math成绩 | double | 否 | 否 | 是 | 否 | 否 |
student_java | java成绩 | double | 否 | 否 | 是 | 否 | 否 |
3.2管理员表(admin)设计
字段名 | 说明 | 类型 | 主键 | 外键 | 非空 | 唯一 | 自增 |
---|
id | 管理员编号 | int(10) | 是 | 否 | 是 | 是 | 是 |
admin_id | 管理员账号 | varchar(11) | 否 | 否 | 是 | 是 | 否 |
admin_password | 管理员密码 | varchar(32) | 否 | 否 | 是 | 否 | 否 |
admin_name | 管理员姓名 | varchar(20) | 否 | 否 | 是 | 否 | 否 |
admin_card | 管理员身份证号 | varchar(18) | 否 | 否 | 是 | 是 | 否 |
admin_phone | 管理员手机号 | varchar(15) | 否 | 否 | 是 | 是 | 否 |
四、涉及到的类和接口
4.1 接口示例:
4.1.1 AdminDAO接口:用来规范 针对于 admin 表的一些常用操作
package com.student.dao;
import com.student.bean.Admin;
import java.sql.Connection;
public interface AdminDAO {
int register(Connection connection, Admin admin);
Admin login(Connection connection, String adminId, String password);
String recover(Connection connection, String card, String phone);
int unsubscribe(Connection connection, String card, String phone);
boolean checkAdminIsExistById(Connection connection, String adminId);
boolean checkAdminIsExistByCard(Connection connection, String card);
boolean checkAdminIsExistByPhone(Connection connection, String phone);
Admin getAdminByCard(Connection connection, String card);
Admin getAdminByPhone(Connection connection, String phone);
}
4.1.2 StudentDAO:用于规范针对于student表的常用操作
package com.student.dao;
import com.student.bean.Student;
import java.sql.Connection;
import java.util.List;
public interface StudentDAO {
int insert(Connection connection, Student student);
int delete(Connection connection, String studentId);
int update(Connection connection, String studentId, String key, Object value);
int updateAll(Connection connection, String oldStudentId, Student student);
boolean checkStudentIsExistById(Connection connection, String studentId);
boolean checkStudentIsExistByCard(Connection connection, String card);
boolean checkStudentIsExistByPhone(Connection connection, String phone);
Student getStudentByStudentId(Connection connection, String studentId);
Student getStudentByPhone(Connection connection, String phone);
Student getStudentByCard(Connection connection, String card);
List<Student> getStudentAll(Connection connection);
Long getCount(Connection connection);
int clearAll(Connection connection);
}
4.2 类的示例:
4.2.1 Student类:对应数据库中的student表
package com.student.bean;
import com.student.tools.Tools;
import javax.tools.Tool;
import java.math.BigDecimal;
public class Student {
private String studentId;
private String name;
private String sex;
private int age;
private String phone;
private String location;
private String card;
private double english;
private double math;
private double java;
public Student() {
}
public Student(String studentId, String name, String sex, int age, String phone, String location, String card, double english, double math, double java) {
this.studentId = studentId;
this.name = name;
this.sex = sex;
this.age = age;
this.phone = phone;
this.location = location;
this.card = card;
this.english = english;
this.math = math;
this.java = java;
}
public String getStudentId() {
return studentId;
}
public void setStudentId(String studentId) {
this.studentId = studentId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public String getCard() {
return card;
}
public void setCard(String card) {
this.card = card;
}
public double getEnglish() {
return english;
}
public void setEnglish(double english) {
this.english = english;
}
public double getMath() {
return math;
}
public void setMath(double math) {
this.math = math;
}
public double getJava() {
return java;
}
public void setJava(double java) {
this.java = java;
}
@Override
public String toString() {
return studentId + '\t' + name + "\t\t" + sex + "\t\t" + age + "\t\t" + Tools.alignment(phone) + Tools.alignment(location) + Tools.alignment(card) + english + "\t\t" + math + "\t\t" + java;
}
}
4.2.2 Admin类:对应数据库中的admin表
package com.student.bean;
public class Admin {
private String adminId;
private String password;
private String name;
private String card;
private String phone;
public Admin() {
}
public Admin(String adminId, String password, String name, String card, String phone) {
this.adminId = adminId;
this.password = password;
this.name = name;
this.card = card;
this.phone = phone;
}
public String getAdminId() {
return adminId;
}
public void setAdminId(String adminId) {
this.adminId = adminId;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCard() {
return card;
}
public void setCard(String card) {
this.card = card;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
@Override
public String toString() {
return "Admin{" +
"adminId='" + adminId + '\'' +
", password='" + password + '\'' +
", name='" + name + '\'' +
", card='" + card + '\'' +
", phone='" + phone + '\'' +
'}';
}
}
4.2.3 Tools类:用于用户进行输入的工具类
package com.student.tools;
import org.junit.jupiter.api.Test;
import java.util.Scanner;
public class Tools {
private static Scanner input = new Scanner(System.in);
public static char readRegisterMenuSelection() {
char c;
while (true) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '1' && c != '2' && c != '3' && c != '4' && c != '5') {
System.out.println("选择错误,请重新输入:");
} else break;
}
return c;
}
public static char readMainMenuSelection() {
char c;
while (true) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '0' && c != '1' && c != '2' && c != '3' && c != '4' && c != '5' && c != '6' && c != '7' && c != '8' && c != '9') {
System.out.println("选择错误,请重写输入:");
} else break;
}
return c;
}
public static void readReturn() {
System.out.println("按回车键继续....");
readKeyBoard(100, true);
}
public static int readInt() {
int n;
while (true) {
String str = readKeyBoard(2, false);
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.println("数字输入错误,请重新输入:");
}
}
return n;
}
public static String readString() {
String str = readKeyBoard(18, false);
return str;
}
public static String alignment(String str){
int length = 18;
if(str.length() < 18){
while(str.length() < length){
str += " ";
}
}
return str;
}
public static char readConfirmSelection() {
char c;
while (true) {
String str = readKeyBoard(1, false).toUpperCase();
c = str.charAt(0);
if (c == 'Y' || c == 'N') {
break;
} else {
System.out.println("选择错误,请重写输入:");
}
}
return c;
}
private static String readKeyBoard(int limit, boolean blankReturn) {
String line = "";
while (input.hasNextLine()) {
line = input.nextLine();
if (line.length() == 0) {
if (blankReturn) {
return line;
} else {
continue;
}
}
if (line.length() < 1 || line.length() > limit) {
System.out.println("输入长度(不大于" + limit + ")错误,请重新输入:");
continue;
}
break;
}
return line;
}
}
4.2.4 JDBCUtiles类:封装的数据库连接通用操作
package com.student.dao.util;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.commons.dbutils.DbUtils;
import org.junit.Test;
import javax.sql.DataSource;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
public class JDBCUtils {
private static DataSource source1;
static{
try {
Properties properties = new Properties();
InputStream resourceAsStream = ClassLoader.getSystemClassLoader().getResourceAsStream("student.properties");
properties.load(resourceAsStream);
source1 = DruidDataSourceFactory.createDataSource(properties);
} catch (Exception e) {
e.printStackTrace();
}
}
public static Connection getConnection() throws SQLException {
Connection connection = source1.getConnection();
return connection;
}
public static Connection getConnection1() throws Exception {
InputStream resourceAsStream = ClassLoader.getSystemClassLoader().getResourceAsStream("jdbc.properties");
Properties properties = new Properties();
properties.load(resourceAsStream);
String url = properties.getProperty("url");
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driverClass = properties.getProperty("driverClass");
Class.forName(driverClass);
Connection connection = DriverManager.getConnection(url, user, password);
return connection;
}
public static void closeResource(Connection connection, Statement ps, ResultSet resultSet) {
DbUtils.closeQuietly(connection);
DbUtils.closeQuietly(ps);
DbUtils.closeQuietly(resultSet);
}
}
4.2.5 BaseDAO类:封装了针对于数据表的通用的操作
package com.student.dao;
import com.student.bean.Student;
import com.student.dao.util.JDBCUtils;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import javax.management.Query;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public abstract class BaseDAO<T> {
private Class<T> clazz = null;
{
Type genericSuperclass = this.getClass().getGenericSuperclass();
ParameterizedType parameterizedType = (ParameterizedType) genericSuperclass;
Type[] actualTypeArguments = parameterizedType.getActualTypeArguments();
clazz = (Class<T>) actualTypeArguments[0];
}
public int update(Connection connection, String sql, Object... args) {
try {
QueryRunner queryRunner = new QueryRunner();
int update = queryRunner.update(connection, sql, args);
return update;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public T getInstance(Connection connection, String sql, Object... args) {
try {
QueryRunner queryRunner = new QueryRunner();
BeanHandler<T> studentBeanHandler = new BeanHandler<>(clazz);
T t = queryRunner.query(connection, sql, studentBeanHandler, args);
return t;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public List<T> getForList(Connection connection, String sql, Object... args) {
try {
QueryRunner queryRunner = new QueryRunner();
BeanListHandler<T> studentBeanListHandler = new BeanListHandler<T>(clazz);
List<T> list = queryRunner.query(connection, sql, studentBeanListHandler, args);
return list;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public <E> E getValue(Connection connection, String sql, Object... args) {
try {
QueryRunner queryRunner = new QueryRunner();
ScalarHandler scalarHandler = new ScalarHandler();
Object value = queryRunner.query(connection, sql, scalarHandler, args);
return (E) value;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
}
4.2.6 AdminDAO接口的实现类:
package com.student.dao;
import com.student.bean.Admin;
import java.sql.Connection;
public class AdminDAOImpl extends BaseDAO<Admin> implements AdminDAO {
@Override
public int register(Connection connection, Admin admin) {
String sql = "insert into admin(admin_id,admin_password,admin_name,admin_card,admin_phone) values(?,?,?,?,?)";
return this.update(connection, sql, admin.getAdminId(), admin.getPassword(), admin.getName(), admin.getCard(), admin.getPhone());
}
@Override
public Admin login(Connection connection, String adminId, String password) {
String sql = "select admin_id adminId,admin_password password,admin_name name,admin_card card,admin_phone phone from admin where admin_id = ? and admin_password = ?";
return this.getInstance(connection, sql, adminId, password);
}
@Override
public String recover(Connection connection, String card, String phone) {
String sql = "select admin_password password from admin where admin_card = ? and admin_phone = ?";
return this.getValue(connection, sql, card, phone);
}
@Override
public int unsubscribe(Connection connection, String card, String phone) {
String sql = "delete from admin where admin_card = ? and admin_phone = ?";
return this.update(connection, sql, card, phone);
}
@Override
public boolean checkAdminIsExistById(Connection connection, String adminId) {
String sql = "select count(*) from admin where admin_id = ?";
long count = this.getValue(connection, sql, adminId);
return count > 0;
}
@Override
public boolean checkAdminIsExistByCard(Connection connection, String card) {
String sql = "select count(*) from admin where admin_card = ?";
long count = this.getValue(connection, sql, card);
return count > 0;
}
@Override
public boolean checkAdminIsExistByPhone(Connection connection, String phone) {
String sql = "select count(*) from admin where admin_phone = ?";
long count = this.getValue(connection, sql, phone);
return count > 0;
}
@Override
public Admin getAdminByCard(Connection connection, String card) {
String sql = "select admin_id adminId,admin_password password,admin_name name,admin_card card,admin_phone phone from admin where admin_card = ?";
return this.getInstance(connection, sql, card);
}
@Override
public Admin getAdminByPhone(Connection connection, String phone) {
String sql = "select admin_id adminId,admin_password password,admin_name name,admin_card card,admin_phone phone from admin where admin_phone = ?";
return this.getInstance(connection, sql, phone);
}
}
4.2.7 StudentDAO接口的实现类:
package com.student.dao;
import com.student.bean.Student;
import java.sql.Connection;
import java.util.List;
public class StudentDAOImpl extends BaseDAO<Student> implements StudentDAO {
@Override
public int insert(Connection connection, com.student.bean.Student student) {
String sql = "insert into student(student_id,student_name,student_sex,student_age,student_phone," +
"student_location,student_card,student_english,student_math,student_java) values(?,?,?,?,?,?,?,?,?,?)";
return this.update(connection, sql, student.getStudentId(), student.getName(), student.getSex(),
student.getAge(), student.getPhone(), student.getLocation(),
student.getCard(), student.getEnglish(), student.getMath(), student.getJava());
}
@Override
public int delete(Connection connection, String studentId) {
String sql = "delete from student where student_id = ?";
return this.update(connection, sql, studentId);
}
@Override
public int update(Connection connection, String studentId, String key, Object value) {
String sql = "update student set " + key + "= ? where student_id = ?";
return this.update(connection, sql, value, studentId);
}
@Override
public int updateAll(Connection connection, String oldStudentId, Student student) {
String sql = "update student set student_id = ?,student_name = ?,student_sex = ?," +
"student_age = ?,student_phone = ?,student_location = ?," +
"student_card = ?,student_english = ?,student_math = ?,student_java = ? where student_id = ?";
return this.update(connection, sql, student.getStudentId(), student.getName(), student.getSex(), student.getAge(), student.getPhone(), student.getLocation(), student.getCard(),
student.getEnglish(), student.getMath(), student.getJava(), oldStudentId);
}
@Override
public boolean checkStudentIsExistById(Connection connection, String studentId) {
String sql = "select count(*) from student where student_id = ?";
long count = this.getValue(connection, sql, studentId);
return count > 0;
}
@Override
public boolean checkStudentIsExistByCard(Connection connection, String card) {
String sql = "select count(*) from student where student_card = ?";
long count = this.getValue(connection, sql, card);
return count > 0;
}
@Override
public boolean checkStudentIsExistByPhone(Connection connection, String phone) {
String sql = "select count(*) from student where student_phone = ?";
long count = this.getValue(connection, sql, phone);
return count > 0;
}
@Override
public com.student.bean.Student getStudentByStudentId(Connection connection, String studentId) {
String sql = "select student_id studentId,student_name name,student_age age,student_sex sex,student_phone phone,student_card card,student_location location," +
"student_english english,student_math math,student_java java from student where student_id = ?";
return this.getInstance(connection, sql, studentId);
}
@Override
public Student getStudentByPhone(Connection connection, String phone) {
String sql = "select student_id studentId,student_name name,student_age age,student_sex sex,student_phone phone,student_card card,student_location location," +
"student_english english,student_math math,student_java java from student where student_phone = ?";
return this.getInstance(connection, sql, phone);
}
@Override
public Student getStudentByCard(Connection connection, String card) {
String sql = "select student_id studentId,student_name name,student_age age,student_sex sex,student_phone phone,student_card card,student_location location," +
"student_english english,student_math math,student_java java from student where student_card = ?";
return this.getInstance(connection, sql, card);
}
@Override
public List<com.student.bean.Student> getStudentAll(Connection connection) {
String sql = "select student_id studentId,student_name name,student_age age,student_sex sex,student_phone phone,student_card card,student_location location," +
"student_english english,student_math math,student_java java from student";
return this.getForList(connection, sql);
}
@Override
public Long getCount(Connection connection) {
String sql = "select count(*) from student";
return this.getValue(connection, sql);
}
@Override
public int clearAll(Connection connection) {
String sql = "delete from student";
return this.update(connection, sql);
}
}
4.2.8 接收用户传递的参数和数据库中的admin表进行交互:
package com.student.service;
import com.student.bean.Admin;
import com.student.bean.Student;
import com.student.dao.AdminDAOImpl;
import com.student.dao.util.JDBCUtils;
import java.sql.Connection;
import java.sql.SQLException;
public class AdminService {
private AdminDAOImpl adminDAO = new AdminDAOImpl();
public int register(Admin admin) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.register(connection, admin);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public Admin login(String adminId, String password) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.login(connection, adminId, password);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public String recover(String card, String phone) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.recover(connection, card, phone);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public int unsubscribe(String card, String phone) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.unsubscribe(connection, card, phone);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public boolean checkAdminIsExistById(String id){
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.checkAdminIsExistById(connection,id);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public boolean checkAdminIsExistByCard(String card){
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.checkAdminIsExistByCard(connection,card);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public boolean checkAdminIsExistByPhone(String phone){
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.checkAdminIsExistByPhone(connection,phone);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public Admin getAdminByCard(String card) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.getAdminByCard(connection, card);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public Admin getAdminByPhone(String phone) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return adminDAO.getAdminByPhone(connection, phone);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
}
4.2.9 接收用户传递的参数和数据库中的student表进行交互:
package com.student.service;
import com.student.bean.Student;
import com.student.dao.StudentDAOImpl;
import com.student.dao.util.JDBCUtils;
import org.junit.jupiter.api.Test;
import java.sql.*;
import java.util.List;
import java.util.Properties;
public class StudentService {
private StudentDAOImpl studentDAO = new StudentDAOImpl();
public int addStudent(Student student) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.insert(connection, student);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public int deleteStudent(String studentId) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.delete(connection, studentId);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public int update(String studentId, String key, Object value) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.update(connection, studentId, key, value);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public int updateAll(String oldStudentId, Student student) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.updateAll(connection, oldStudentId, student);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public Object search(String studentId) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.getStudentByStudentId(connection, studentId);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public List<Student> searchAll() {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.getStudentAll(connection);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public Long getCount() {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.getCount(connection);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return null;
}
public int clearAll() {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.clearAll(connection);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return 0;
}
public boolean checkStudentIsExistById(String studentId) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.checkStudentIsExistById(connection, studentId);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public boolean checkStudentIsExistByPhone(String phone) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.checkStudentIsExistByPhone(connection, phone);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public boolean checkStudentIsExistByCard(String card) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
return studentDAO.checkStudentIsExistByCard(connection, card);
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public boolean checkExist(String value) {
Connection connection = null;
try {
connection = JDBCUtils.getConnection();
String sql = "select student_id,student_name,student_age,student_sex,student_phone,student_card,student_location," +
"student_english,student_math,student_java from student";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
ResultSet resultSet = preparedStatement.executeQuery();
ResultSetMetaData metaData = resultSet.getMetaData();
int columnCount = metaData.getColumnCount();
String columnLabel = "";
for (int i = 0; i < columnCount; i++) {
columnLabel = metaData.getColumnLabel(i + 1);
if (value.equals(columnLabel)) {
return true;
}
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JDBCUtils.closeResource(connection, null, null);
}
return false;
}
public String changeName(String name) {
if (name.length() == 2) {
name = name.substring(0, 1) + " " + name.substring(name.length() - 1);
}
return name;
}
}
4.2.10 StudentView类:用于菜单展示和用户交互
package com.student.view;
import com.student.bean.Admin;
import com.student.bean.Student;
import com.student.dao.AdminDAOImpl;
import com.student.service.AdminService;
import com.student.service.StudentService;
import com.student.tools.Tools;
import com.sun.corba.se.impl.orbutil.ObjectWriter;
import org.junit.platform.commons.util.CollectionUtils;
import javax.tools.Tool;
import java.awt.*;
import java.io.*;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.List;
public class StudentView {
private String tempAdminId = null;
private String tempAdminName = null;
private String tempAdminCard = null;
private String tempAdminPhone = null;
AdminService adminService = new AdminService();
StudentService studentService = new StudentService();
public void enterRegisterMenu() {
boolean flag = true;
while (flag) {
System.out.println("------------------------------------【学生信息管理系统】---------------------------------------");
System.out.println("\t\t\t\t\t\t\t\t\t1.登录\t\t2.注册");
System.out.println("\t\t\t\t\t\t\t\t\t3.忘记密码\t4.关于我的");
System.out.println("\t\t\t\t\t\t\t\t\t5.关闭系统");
System.out.println("-------------------------------------------------------------------------------------------");
System.out.println("请输入你的选择:");
char choice = Tools.readRegisterMenuSelection();
switch (choice) {
case '1':
login();
break;
case '2':
register();
break;
case '3':
recover();
break;
case '4':
about();
break;
case '5':
System.out.println("是否要关闭系统(Y/N)?");
char exit = Tools.readConfirmSelection();
if (exit == 'Y' || exit == 'y') {
flag = false;
System.out.println("成功关闭系统");
}
break;
}
}
}
public void enterMainMenu() {
boolean flag = true;
while (flag) {
System.out.println("------------------------------------【学生信息管理系统】---------------------------------------");
System.out.println("\t\t\t\t\t\t\t\t\t1.添加学生信息");
System.out.println("\t\t\t\t\t\t\t\t\t2.删除学生信息");
System.out.println("\t\t\t\t\t\t\t\t\t3.修改学生信息(指定部分)");
System.out.println("\t\t\t\t\t\t\t\t\t4.修改学生信息(所有信息)");
System.out.println("\t\t\t\t\t\t\t\t\t5.查找学生信息");
System.out.println("\t\t\t\t\t\t\t\t\t6.显示学生信息(全体学生)");
System.out.println("\t\t\t\t\t\t\t\t\t7.清空学生信息");
System.out.println("\t\t\t\t\t\t\t\t\t8.导出学生信息");
System.out.println("\t\t\t\t\t\t\t\t\t9.注销账户");
System.out.println("\t\t\t\t\t\t\t\t\t0.退出登录");
System.out.println("-------------------------------------------------------------------------------------------");
System.out.println("请输入你的选择:");
char choice = Tools.readMainMenuSelection();
switch (choice) {
case '1':
addStudent();
break;
case '2':
deleteStudent();
break;
case '3':
updateStudent();
break;
case '4':
updateStudentAll();
break;
case '5':
searchStudentById();
break;
case '6':
showStudentAll();
break;
case '7':
clearStudentAll();
break;
case '8':
export();
break;
case '9':
int unsubscribe = unsubscribe();
if (unsubscribe > 0) {
return;
}
break;
case '0':
System.out.println("是否要退出登录状态(Y/N)?");
char exit = Tools.readConfirmSelection();
if (exit == 'Y' || exit == 'y') {
flag = false;
System.out.println("成功退出登录");
}
break;
}
}
}
public void login() {
System.out.println("------------------------------------【登录】-------------------------------------------------");
System.out.println("请输入你的个人账号:");
String adminId = Tools.readString();
System.out.println("请输入你的个人密码:");
String password = Tools.readString();
Admin login = adminService.login(adminId, password);
if (login != null) {
System.out.println("欢迎使用学生信息管理系统!,点击回车键开始使用学生信息管理系统");
tempAdminId = login.getAdminId();
tempAdminName = login.getName();
tempAdminCard = login.getCard();
tempAdminPhone = login.getPhone();
Tools.readReturn();
enterMainMenu();
} else {
System.out.println("账号或密码有误");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void register() {
System.out.println("------------------------------------【注册】------------------------------------------------");
System.out.println("请输入你的个人账号:");
String adminId = Tools.readString();
while (adminService.checkAdminIsExistById(adminId)) {
System.out.println("你输入的账号已经存在,请重新输入:");
adminId = Tools.readString();
adminService.checkAdminIsExistById(adminId);
}
System.out.println("请输入你的个人密码:");
String password = Tools.readString();
System.out.println("请输入你的姓名:");
String name = Tools.readString();
System.out.println("请输入你的身份证号:");
String card = Tools.readString();
while (adminService.checkAdminIsExistByCard(card)) {
System.out.println("你输入的身份证号已经存在,请重新输入:");
card = Tools.readString();
adminService.checkAdminIsExistByCard(card);
}
System.out.println("请输入你的手机号:");
String phone = Tools.readString();
while (adminService.checkAdminIsExistByPhone(phone)) {
System.out.println("你输入的手机号已经存在,请重新输入:");
phone = Tools.readString();
adminService.checkAdminIsExistByPhone(phone);
}
Admin admin = new Admin(adminId, password, name, card, phone);
int register = adminService.register(admin);
if (register > 0) {
System.out.println("注册成功");
} else {
System.out.println("注册失败,请重新试一下!");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void recover() {
System.out.println("------------------------------------【找回密码】---------------------------------------------");
System.out.println("请输入你的身份证号:");
String card = Tools.readString();
System.out.println("请输入你的手机号:");
String phone = Tools.readString();
String recover = adminService.recover(card, phone);
if (recover != null) {
System.out.println("密码找回成功,你的密码为:" + recover);
} else {
System.out.println("你输入的身份证号或手机号有误!");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void about() {
try {
Desktop desktop = Desktop.getDesktop();
URI uri = new URI("https://blog.csdn.net/m0_47214030?type=blog");
desktop.browse(uri);
} catch (URISyntaxException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
System.out.println("欢迎使用学生信息管理系统【作者:☆往事随風☆】");
}
}
public void showLabel() {
System.out.println("学号\t\t姓名\t\t\t性别\t\t年龄\t\t\t电话\t\t\t\t住址\t\t\t\t\t身份证号\t\t\t英语\t\t\t数学\t\t\tjava");
}
public void addStudent() {
System.out.println("------------------------------------【添加学生信息】------------------------------------------");
boolean flag = true;
int count = 0;
while (flag) {
System.out.println("请输入学生学号:");
String studentId = Tools.readString();
while (studentService.checkStudentIsExistById(studentId)) {
System.out.println("你要添加的学号已经存在,请重新输入:");
studentId = Tools.readString();
studentService.checkStudentIsExistById(studentId);
}
System.out.println("请输入学生姓名:");
String name = Tools.readString();
name = studentService.changeName(name);
System.out.println("请输入学生性别:");
String sex = Tools.readString();
System.out.println("请输入学生年龄:");
int age = Tools.readInt();
System.out.println("请输入学生电话:");
String phone = Tools.readString();
while (studentService.checkStudentIsExistByPhone(phone)) {
System.out.println("你要添加的手机号已经存在,请重新输入:");
phone = Tools.readString();
studentService.checkStudentIsExistByPhone(phone);
}
System.out.println("请输入学生身份证号码:");
String card = Tools.readString();
while (studentService.checkStudentIsExistByCard(card)) {
System.out.println("你要添加的身份证号已经存在,请重新输入:");
card = Tools.readString();
studentService.checkStudentIsExistByCard(card);
}
System.out.println("请输入如家庭住址:");
String location = Tools.readString();
System.out.println("请输入英语成绩:");
double english = Tools.readDouble();
System.out.println("请输入数学成绩:");
double math = Tools.readDouble();
System.out.println("请输入Java成绩:");
double java = Tools.readDouble();
Student student = new Student(studentId, name, sex, age, phone, location, card, english, math, java);
int i = studentService.addStudent(student);
if (i > 0) {
count++;
System.out.println("已成功添加" + count + "条学生信息");
System.out.println("是否继续添加(Y/N)?");
char choice = Tools.readConfirmSelection();
if (choice == 'Y' || choice == 'y') {
System.out.println("-------------------------------------------------------------------------------------------");
} else {
System.out.println("成功添加" + count + "条学生信息");
break;
}
} else {
System.out.println("添加失败");
}
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void deleteStudent() {
System.out.println("------------------------------------【删除学生信息】------------------------------------------");
System.out.println("请输入要删除学生的学号:");
String studentId = Tools.readString();
Object search = studentService.search(studentId);
if (search != null) {
showLabel();
System.out.println(search);
System.out.println("确定要删除吗(Y/N):");
char choice = Tools.readConfirmSelection();
if (choice == 'y' || choice == 'Y') {
int delete = studentService.deleteStudent(studentId);
if (delete > 0) {
System.out.println("删除成功");
} else {
System.out.println("删除失败");
}
}
} else {
System.out.println("要删除的学生不存在");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void updateStudent() {
System.out.println("------------------------------------【修改学生信息】------------------------------------------");
System.out.println("请输入学生学号:");
String studentId = Tools.readString();
Object search = studentService.search(studentId);
if (search != null) {
showLabel();
System.out.println(search);
System.out.println("确定要修改该学生的部分信息(Y/N)?");
char choice = Tools.readConfirmSelection();
if (choice == 'Y' || choice == 'y') {
System.out.println("你可在以下字段中选择一个进行修改:");
System.out.println("[student_id,student_name,student_sex,student_age,student_phone,student_location,student_card,student_english,student_math,student_java]");
System.out.println("请输入要修改的字段:");
String key = Tools.readString();
while (!studentService.checkExist(key)) {
System.out.println("你输入的字段不存在,请重新输入:");
key = Tools.readString();
studentService.checkExist(key);
}
System.out.println("请输入修改后的信息:");
String value = Tools.readString();
if (key == "student_name") {
value = studentService.changeName(value);
}
int update = studentService.update(studentId, key, value);
if (update > 0) {
System.out.println("修改成功");
} else {
System.out.println("修改失败,失败原因:新修改的学号或手机号或身份证号与其他学生相同");
}
}
} else {
System.out.println("你要修改的学生不存在!");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void updateStudentAll() {
System.out.println("------------------------------------【修改学生全部信息】---------------------------------------");
System.out.println("请输入学生学号:");
String oldStudentId = Tools.readString();
Object search = studentService.search(oldStudentId);
if (search != null) {
showLabel();
System.out.println(search);
System.out.println("确定要修改该学生的全部信息(Y/N)?");
char choice = Tools.readConfirmSelection();
if (choice == 'Y' || choice == 'y') {
System.out.println("请输入修改后的学生学号:");
String studentId = Tools.readString();
System.out.println("请输入修改后的学生姓名:");
String name = Tools.readString();
name = studentService.changeName(name);
System.out.println("请输入修改后的学生性别:");
String sex = Tools.readString();
System.out.println("请输入修改后的学生年龄:");
int age = Tools.readInt();
System.out.println("请输入修改后的学生电话:");
String phone = Tools.readString();
System.out.println("请输入修改后的学生身份证号码:");
String card = Tools.readString();
System.out.println("请输入修改后的如家庭住址:");
String location = Tools.readString();
System.out.println("请输入修改后的英语成绩:");
double english = Tools.readDouble();
System.out.println("请输入修改后的数学成绩:");
double math = Tools.readDouble();
System.out.println("请输入修改后的Java成绩:");
double java = Tools.readDouble();
Student student = new Student(studentId, name, sex, age, phone, location, card, english, math, java);
int updateAll = studentService.updateAll(oldStudentId, student);
if (updateAll > 0) {
System.out.println("修改成功");
} else {
System.out.println("修改失败,失败原因:新修改的学号或手机号或身份证号与其他学生相同");
}
}
} else {
System.out.println("你要修改的学生不存在!");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void searchStudentById() {
System.out.println("------------------------------------【查询学生信息】------------------------------------------");
System.out.println("请输入要查找的学生的学号:");
String studentId = Tools.readString();
Object student = studentService.search(studentId);
if (student != null) {
System.out.println("查询结果如下:");
showLabel();
System.out.println(student);
} else {
System.out.println("该学生信息不存在");
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void showStudentAll() {
System.out.println("------------------------------------【显示全体学生信息】---------------------------------------");
Long count = studentService.getCount();
System.out.println("一共查询到" + count + "条记录");
showLabel();
List<Student> students = studentService.searchAll();
for (Student key : students) {
System.out.println(key);
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void export() {
RandomAccessFile randomAccessFile = null;
try {
System.out.println("------------------------------------【导出学生信息】------------------------------------------");
System.out.println("1.自定义路径文件名\t\t2.默认保存路径文件名(D:\\\\student.csv)");
System.out.println("3.暂不导出");
String fileURL = null;
System.out.println("请输入你的选择:");
char choice = Tools.readRegisterMenuSelection();
switch (choice) {
case '1':
System.out.println("请输入要保存的文件路径(例如:D:\\\\student.csv):");
fileURL = Tools.readString();
break;
case '2':
fileURL = "D:\\student.csv";
break;
case '3':
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
return;
}
randomAccessFile = new RandomAccessFile(new File(fileURL), "rw");
List<Student> students = studentService.searchAll();
if (students.size() > 0 && null != students) {
String title[] = new String[]{"学号,", "姓名,", "性别,", "年龄,", "手机号,", "身份证号,", "家庭住址,", "英语,", "数学,", "java,\n"};
for (String key : title) {
randomAccessFile.write(("\uFEFF" + key).getBytes());
}
for (Student student : students) {
randomAccessFile.write((student.getStudentId() + ",").getBytes());
randomAccessFile.write((student.getName() + ",").getBytes());
randomAccessFile.write((student.getSex() + ",").getBytes());
randomAccessFile.write((student.getAge() + ",").getBytes());
randomAccessFile.write((student.getPhone() + ",").getBytes());
randomAccessFile.write((student.getCard() + ",").getBytes());
randomAccessFile.write((student.getLocation() + ",").getBytes());
randomAccessFile.write((student.getEnglish() + ",").getBytes());
randomAccessFile.write((student.getMath() + ",").getBytes());
randomAccessFile.write((student.getJava() + ",").getBytes());
randomAccessFile.write("\n".getBytes());
}
System.out.println("学生信息导出完毕");
} else {
System.out.println("尚未查询到学生信息,无法进行导出操作");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (randomAccessFile != null) {
randomAccessFile.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public void clearStudentAll() {
System.out.println("------------------------------------【清空所有学生信息】---------------------------------------");
System.out.println("确定要清空所有学生信息(Y/N),执行此操作将丢失所有学生的信息:");
char choice = Tools.readConfirmSelection();
if (choice == 'Y' || choice == 'y') {
List<Student> students = studentService.searchAll();
if (students.size() > 0 && null != students) {
System.out.println("请输入你的身份证号:");
String card = Tools.readString();
boolean isExist = adminService.checkAdminIsExistByCard(card);
if (isExist) {
int clearAll = studentService.clearAll();
if (clearAll > 0) {
System.out.println("学生信息清空成功");
} else {
System.out.printf("学生信息清空失败");
}
} else {
System.out.println("你输入的身份证号不存在");
}
}else {
System.out.println("尚未存入学生信息,无法进行清空操作");
}
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
}
public int unsubscribe() {
System.out.println("------------------------------------【注销账户】----------------------------------------------");
System.out.println("当前登录的管理员账号:" + tempAdminId);
System.out.println("当前登录的管理员姓名:" + tempAdminName);
System.out.println("确定要注销账户(Y/N)?");
char choice = Tools.readConfirmSelection();
if (choice == 'Y' || choice == 'y') {
System.out.println("请输入你的身份证号:");
String card = Tools.readString();
System.out.println("请输入你的手机号:");
String phone = Tools.readString();
Admin adminByCard = adminService.getAdminByCard(card);
Admin adminByPhone = adminService.getAdminByPhone(phone);
if (adminByCard != null && adminByPhone != null) {
if (tempAdminCard.equals(adminByCard.getCard()) && tempAdminPhone.equals(adminByPhone.getPhone())) {
int unsubscribe = adminService.unsubscribe(card, phone);
if (unsubscribe > 0) {
System.out.println("账户注销成功,已成功退出登录状态");
return unsubscribe;
} else {
System.out.println("账户注销失败");
}
} else {
System.out.println("你输入的身份证号或手机号有误");
}
} else {
System.out.println("你的身份证号或手机号不存在");
}
}
System.out.println("-------------------------------------------------------------------------------------------");
Tools.readReturn();
return 0;
}
public static void main(String[] args) {
StudentView studentView = new StudentView();
studentView.enterRegisterMenu();
}
}
五、菜单页面展示
5.1 登录菜单:
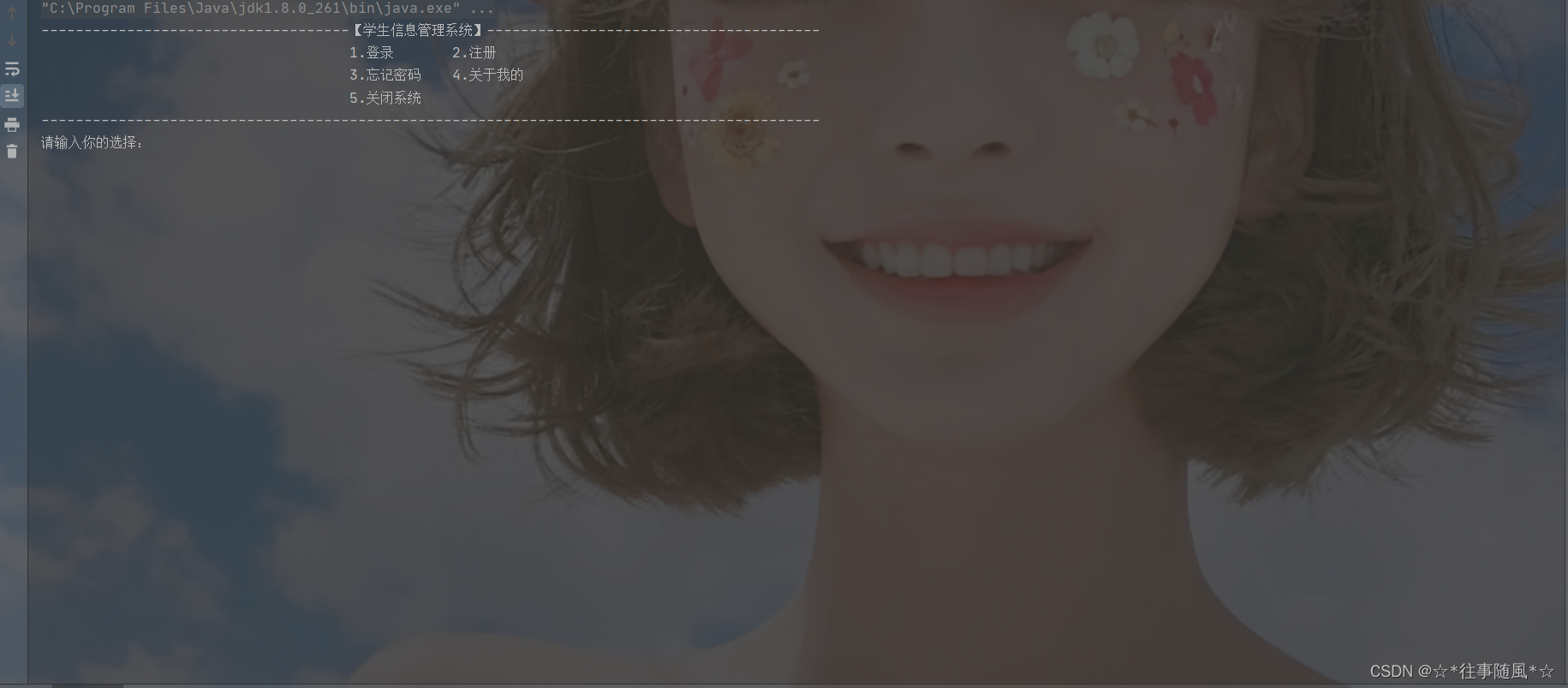
5.2 功能菜单:
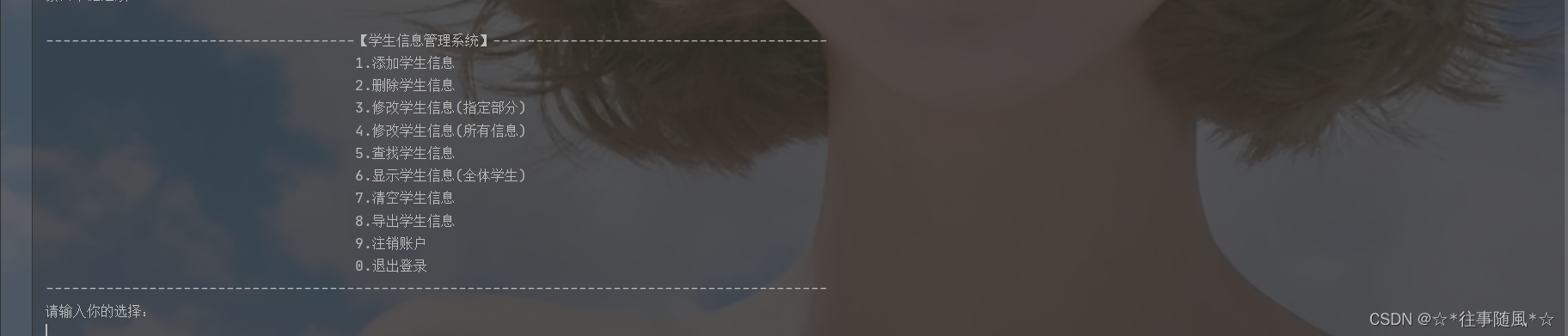
六、在线演示
功能在线演示
七、项目源码
学生信息管理系统项目源码