文件流概述
用来读取文件中的内容与将内容写入文件中的工具,是Java中提供好的类。
文件流分类
根据方向
- 输入(Input)流——读取文件
- 输出(Output)流——写入文件
根据内容
- 字节(Byte)流——读写二进制文件
- 字符(Char)流——读写文本文件
根据处理方式
- 节点流——针对文件建立
- 处理流——针对其他文件流建立
输入流
- 输入流是指建立在文件或者其他文件流上用来对目标文件进行读取的文件流
- 输入流只能对文件进行读取
- 读取二进制文件使用字节输入流,读取文本文件使用文本输入流(字符输入流)
字节输入流
常用字节输入流继承关系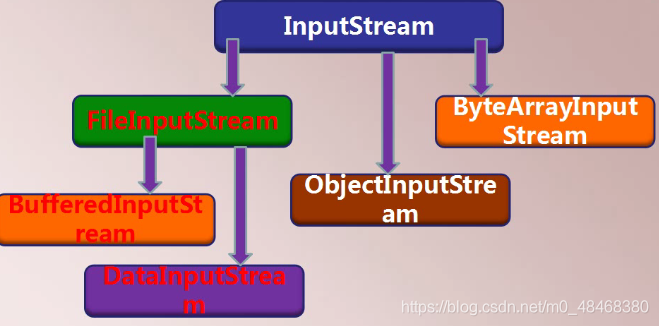
InputStream是接口
BufferedInputStream是缓冲流,先将大量数据存入缓冲区,再由缓冲区读取,减少了与磁盘的操作次数,大大提高了效率
DataInputStream可以读取多种类型的数据
字节输入流主要方法
- public abstract int read()throws IOException:对文件进行一次读取并返回读取到的字节数(无法获取内容,意义不大)
- public int read(byte[] b)throws IOException:读取字节文件并将读取的内容存入字节数组中,返回读取到的字节数(不常用,因为未知文件内容大小,无法确定字节数组的长度)
- public int read(byte[] b,int off,int len)throws IOException:读取len长度的数据,从索引off开始存入byte数组中,len不能超过一次读取最大的字节数(可以通过循环多次读取内容,在每次读取后都对数据进行处理)
- public void close()throws IOException:关闭字节输入流,释放资源
文件读写顺序:建立需要读写的文件对象——建立输入输出流——关闭输入输出流
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
/***
*
* @author Vbird
* @date 2020/8/12
*/
public class Test {
public static void main(String []args) {
/*1.创建文件对象*/
File file = new File("d:/python.jpg");
/*2.创建字节输入流*/
if(file.exists()) {//当file存在时创建输入流
InputStream in = null;
try {
in = new FileInputStream(file);//使用子类FileInputStream建立 二进制输入流
int count = in.read();//读取到的字节数,并不等于文件大小,获取文件大小用.length();
byte[] bys = new byte[10];
in.read(bys);//将读取的内容存入字节数组中
byte[] bys2= new byte[100];
in.read(bys2, 0, 10);//从0开始读取10个数据
/*利用循环读取全部内容*/
while(in.read(bys)!=-1) {//当读取到最后一个字节时往后读取则子节数为-1,内容被读取完
System.out.print("\n");
for(byte b:bys) {
System.out.print(b+" ");
}
}
while(in.read(bys2,0,10)!=-1) {
System.out.print("\n");
for(byte b:bys2) {
System.out.print(b+" ");
}
}//在未知文件长度时,按情况选择两种方法
} catch (IOException e) {//使用IOException可以解决两种异常
e.printStackTrace();
}finally {
/*3.关闭字节输入流,释放资源*/
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
字符输入流
常用字节输入流继承关系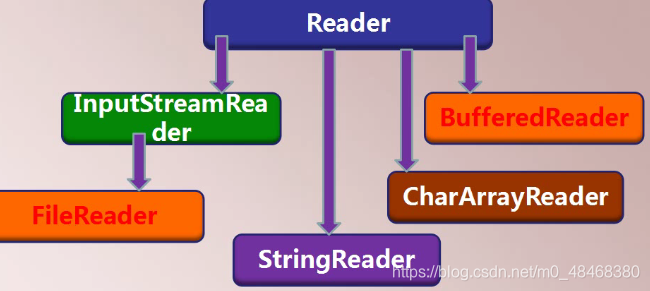
根据前缀判断各个特性,如FileReader指向文件,StringReader指向字符串等等
字符输入流主要方法
- public int read()throws IOException:进行一次读取并返回读取字节数
- public int read(char[] cbuf)throws IOException:读取字符文件并将内容存入字符数组中
- public abstract int read(char[] cbuf,int off,int len)throws IOExceptoin:读取len长度的数据,从索引off开始,存入cbuf数组中,len不能超过一次读取最大的字节数
- public void close()throws IOException:关闭字符输入流
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
/***
*
* @author Vbird
* @date 2020/8/12
*/
public class Test {
public static void main(String []args) {
/*创建文件对象*/
File file = new File("d:/one.txt");
/*创建文件流*/
FileReader read = null;
if(file.exists()) {
try {
read = new FileReader(file);
int count = read.read();//获取读取一次可以读取的字节数
/*获取文件内容*/
char[] chs = new char[100];
read.read(chs);
for(char ch:chs) {
System.out.print(ch);
}
/*利用循环获取全部内容*/
char[] chs2 = new char[2];
while((count = read.read(chs2, 0, chs2.length))!=-1) {//每次读取数组长度的数据存入数组中
String s = new String(chs2,0,count);//将读取的数据转化为字符串,不能用数组长度作参数因为最后一次读取可能数组未读满
System.out.print(s);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
read.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
输出流
- 输出流是指建立在文件或其他文件流上用来对目标文件进行写入的文件流
- 输出流只对目标文件进行写入操作
- 写入二进制文件使用字节输出流,写入文本文件使用字符输出流
字节输出流
常用的字节输出流继承关系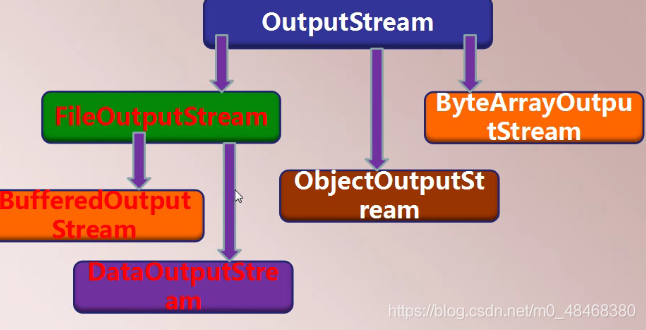
字节输出流主要方法
- public void write(int b)throws IOException:写入一个整形数
- public void write(byte[] b)throws IOException:写入字节数组
- public void write(byte[] b,int off,int len)throws IOException:在字节数组中从off开始取len长度的数据存入文件中
- public void close()throws IOException:关闭输出流
/*创建输出流*/
FileOutputStream out = null;
try {
out = new FileOutputStream("d:/启动.bat");//若没有该文件会自动创建
BufferedOutputStream buffer = new BufferedOutputStream(out);//基于out建立缓冲输出流,写入与其余一样
String str = "start=d:/one.txt";//批处理,打开one.txt
byte []bys = str.getBytes();//将字符串转为字节数组
out.write(bys);//写入字节数组
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
常用字符输出流继承结构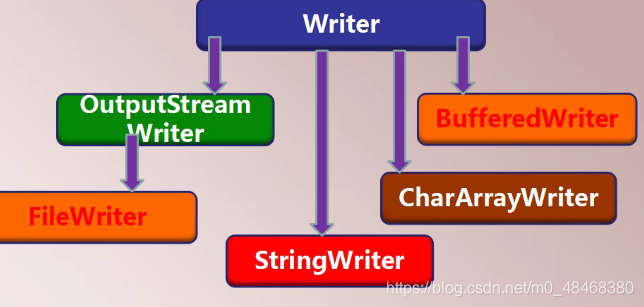
字符输出流主要方法
- public void write(int b)throws IOException:写入整形数b
- public void write(chars[] b)throwsIOException:写入字符数组
- public abstract void write(chars[] b,int off,int len)throws IOException:从字符数组off开始,将len长度的字符写入
- public void write(String str)throws IOException:写入字符串
- public void close()throws IOException:关闭流
两中输入输出流都整体相同