起初我看到别的博主发了一个这样的图
于是,我给chatgpt提出要求:
我想写一个html网页,上面是一个圆圆的时钟,下面写倒计时,距离新年还有多少天,多少小时,多少分钟,时钟有时针分针秒针,而且能够实时转动
第一步:新建一个新年时钟倒计时.html,里面填充内容如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>New Year Countdown</title>
<style>
.clock {
width: 200px;
height: 200px;
border: 2px solid black;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div id="clock" class="clock"></div>
<div id="countdown"></div>
<script>
function updateClock() {
const clock = document.getElementById("clock");
const currentTime = new Date();
const seconds = currentTime.getSeconds();
const minutes = currentTime.getMinutes();
const hours = currentTime.getHours();
const secondsAngle = (seconds / 60) * 360;
const minutesAngle = ((minutes * 60 + seconds) / 3600) * 360;
const hoursAngle = ((hours * 3600 + minutes * 60 + seconds) / 43200) * 360;
clock.style.transform = `rotate(${secondsAngle}deg)`;
clock.innerHTML = `${hours.toString().padStart(2, '0')}:${minutes.toString().padStart(2, '0')}:${seconds.toString().padStart(2, '0')}`;
setTimeout(updateClock, 1000);
}
function updateCountdown() {
const countdown = document.getElementById("countdown");
const now = new Date();
const newYear = new Date(now.getFullYear() + 1, 0, 1);
const timeLeft = newYear - now;
const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24));
const hours = Math.floor((timeLeft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((timeLeft % (1000 * 60 * 60)) / (1000 * 60));
countdown.innerHTML = `Time remaining until New Year: ${days} days, ${hours} hours, ${minutes} minutes`;
setTimeout(updateCountdown, 1000);
}
updateClock();
updateCountdown();
</script>
</body>
</html>
第二步:点击调试开始运行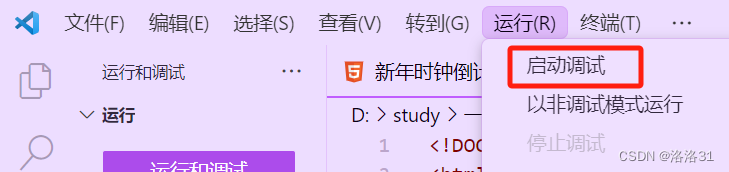
运行成果如图
不会做动图,凑合看。
总之就是一秒转一点,我说gpt烙铁,你别把我笑死。(无奈扶额)
重新调教了n次chatgpt,屡次调节效果如图
1.0版本
分针时针秒针该转不该转的都在转
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>新年时钟倒计时</title>
<style>
.clock {
width: 200px;
height: 200px;
border: 2px solid black;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
position: relative;
animation: rotate 60s infinite linear;
}
.hour-hand, .minute-hand, .second-hand {
position: absolute;
background-color: black;
transform-origin: bottom center;
}
.hour-hand {
width: 4px;
height: 75px;
top: 50%;
left: 50%;
margin: -75px -2px 0;
animation: rotate 43200s infinite linear;
}
.minute-hand {
width: 2px;
height: 95px;
top: 50%;
left: 50%;
margin: -95px -1px 0;
animation: rotate 3600s infinite linear;
}
.second-hand {
width: 1px;
height: 105px;
top: 50%;
left: 50%;
margin: -105px;
animation: rotate 60s infinite linear;
}
@keyframes rotate {
0% {
transform: rotate(0);
}
100% {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="clock">
<div class="hour-hand"></div>
<div class="minute-hand"></div>
<div class="second-hand"></div>
</div>
<div id="countdown"></div>
<script>
function updateCountdown() {
const countdown = document.getElementById("countdown");
const now = new Date();
const newYear = new Date(2024, 1, 10); // 设置新年日期为2024年2月10日
const timeLeft = newYear - now;
const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24));
const hours = Math.floor((timeLeft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((timeLeft % (10