public class Test02 {
private static List<Map> collect;
private static List<Map> collect2;
public static void main(String[] args) {
test01();
// test02();
}
//使用常规的方法进行获取
private static void test02() {
Company c1= new Company("yq","1234",8888);
Company c2= new Company("ls","4567",9999);
ArrayList<Map> list = new ArrayList<Map>();
Map<String,Integer> map= new HashMap();
map.put(c1.getNo() ,c1.getQuantity());
Map<String,Integer> map2= new HashMap();
map2.put(c2.getNo() ,c2.getQuantity());
list.add(map);
list.add(map2);
list.add(map);
list.add(map2);
int countYq = 0;
int countLs = 0;
for (int i = 0; i < list.size(); i++) {
if(list.get(i).containsKey("1234")){
Integer in = (Integer)list.get(i).get("1234");
countYq += in;
}
if(list.get(i).containsKey("4567")){
Integer in = (Integer)list.get(i).get("4567");
countLs += in;
}
}
System.out.println("countYq: " + countYq);
System.out.println("countYq: "+countLs);
}
//使用Stream来获取
private static void test01() {
Company c1= new Company("yq","1234",8888);
Company c2= new Company("ls","4567",9999);
ArrayList<Map> list = new ArrayList<Map>();
Map<String,Integer> map= new HashMap();
map.put(c1.getNo() ,c1.getQuantity());
Map<String,Integer> map2= new HashMap();
map2.put(c2.getNo() ,c2.getQuantity());
list.add(map);
list.add(map2);
list.add(map);
list.add(map2);
Integer cout = 0;
Integer cout2 = 0;
Stream<Map> stream2 = list.stream();
Stream<Map> stream = list.stream();
//将过滤好的Stream转换成List集合
collect = stream.filter(e ->e.containsKey("1234")).collect(Collectors.toList());
for (int i = 0; i < collect.size(); i++) { //list的当中的每一个元素还是一个map
Integer s= (Integer)collect.get(i).get("1234");
cout += s;
}
System.out.println(cout);
collect2 = stream2.filter(e ->e.containsKey("4567")).collect(Collectors.toList());
for (int i = 0; i < collect2.size(); i++) {
Integer s= (Integer)collect2.get(i).get("4567");
cout2 += s;
}
System.out.println(cout2);
}
}
class Company{
private String name ;
private String no;
private int quantity;
public Company() {
super();
}
public Company(String name, String no, int quantity) {
super();
this.name = name;
this.no = no;
this.quantity = quantity;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNo() {
return no;
}
public void setNo(String no) {
this.no = no;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
@Override
public String toString() {
return "Company [name=" + name + ", no=" + no + ", quantity=" + quantity + "]";
}
}
作业使用Stream流来操作集合VS普通方法
最新推荐文章于 2023-07-06 17:17:25 发布
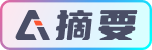