计算器
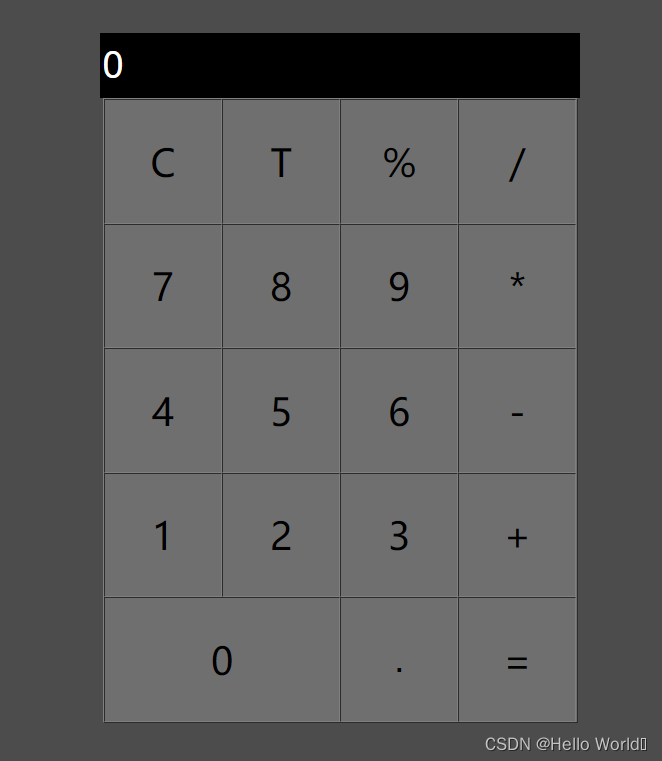
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
body {
background-color: #4c4c4c;
}
input {
margin-top: 3%;
width: 25%;
height: 50px;
font-size: 30px;
color: white;
border-style: none;
background-color: black;
}
table {
background-color: #6f6f6f;
}
tr {
text-align: center;
font-size: 30px;
height: 20%;
}
td {
cursor: pointer;
width: 25%;
}
td:hover {
background-color: #efefef;
}
button {
width: 100%;
height: 100%;
background-color: #6f6f6f;
border: none;
font-size: 30px;
}
button:hover {
background-color: #efefef;
}
</style>
</head>
<body>
<center>
<input type="text" id="showResult" value="0" readonly>
<table width="25%" height="500px" border="1" cellspacing="0" align="center">
<tr>
<td id="clear">C</td>
<td id="del">T</td>
<td id="per">%</td>
<td class="ysf">/</td>
</tr>
<tr>
<td class="num">7</td>
<td class="num">8</td>
<td class="num">9</td>
<td class="ysf">*</td>
</tr>
<tr>
<td class="num">4</td>
<td class="num">5</td>
<td class="num">6</td>
<td class="ysf">-</td>
</tr>
<tr>
<td class="num">1</td>
<td class="num">2</td>
<td class="num">3</td>
<td class="ysf">+</td>
</tr>
<tr>
<td colspan="2" class="num">0</td>
<td><button id="point">.</button></td>
<td id="result">=</td>
</tr>
</table>
</center>
</body>
<script>
var numValue1 = '';
var numValue2 = '';
var opr = '';
var showResult = document.getElementById("showResult");
var nums = document.getElementsByClassName("num");
for (let i = 0; i < nums.length; i++) {
nums[i].onclick = function() {
numValue1 += this.innerHTML;
showResult.value = numValue1;
}
}
var point = document.getElementById("point");
point.onclick = function() {
if (numValue1.indexOf(".") == -1) {
numValue1 += this.innerHTML;
showResult.value = numValue1;
} else {
alert("一个数只能有一个小数点 不能重复");
}
};
var oprs = document.getElementsByClassName("ysf");
for (let i = 0; i < oprs.length; i++) {
oprs[i].onclick = function() {
opr = this.innerHTML;
if (numValue2 == "") {
numValue2 = numValue1;
numValue1 = "";
} else if (numValue1 != "") {
resultFun();
}
}
}
document.getElementById("result").onclick = function() {
resultFun();
}
document.getElementById("clear").onclick = function() {
numValue1 = "";
numValue2 = "";
opr = "";
showResult.value = "0";
}
document.getElementById("del").onclick = function() {
if (numValue1.length > 1) {
numValue1 = numValue1.substring(0, numValue1.length - 1);
showResult.value = numValue1;
} else {
showResult.value = "0";
}
}
document.getElementById("per").onclick = function() {
numValue1 = numValue1 * 0.01;
showResult.value = numValue1;
}
function resultFun() {
var one = Number(numValue2);
var two = Number(numValue1);
var res = null;
switch (opr) {
case "+":
res = one + two;
break;
case "-":
res = one - two;
break;
case "*":
res = one * two;
break;
case "/":
if (two == 0) {
alert("除数不能为0 请重新输入")
numValue1 = "";
numValue2 = "";
opr = '';
res = 0;
} else {
res = one / two;
}
break;
}
numValue2 = res.toFixed(6);
numValue1 = "";
showResult.value = numValue2 * 1;
};
</script>
</html>
倒计时
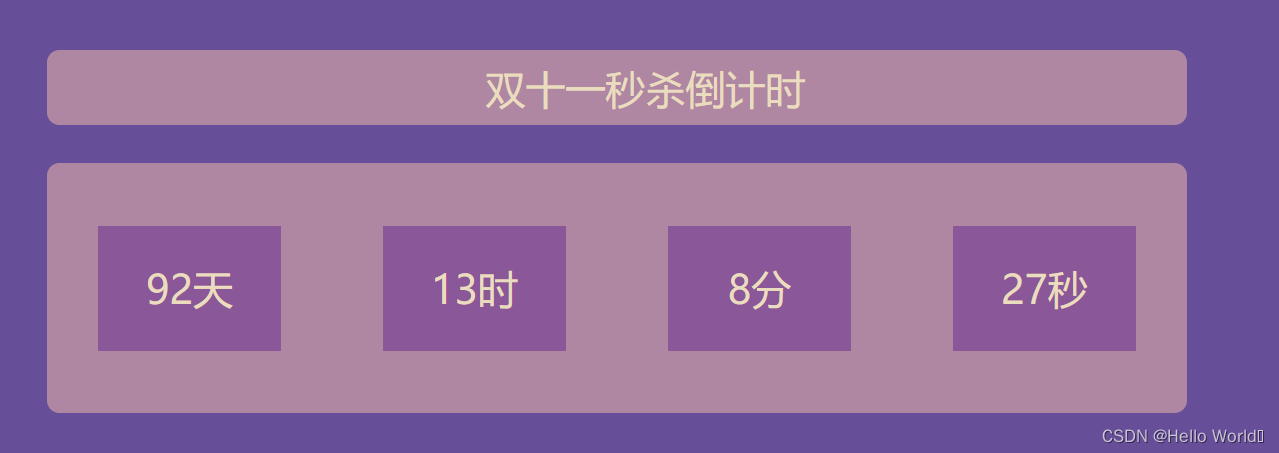
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
body {
background-color: #664E98;
}
#d1 {
margin-left: 20%;
margin-top: 2%;
border-radius: 10px;
width: 60%;
height: 200px;
background-color: #AF87A3;
display: flex;
justify-content: space-around;
align-items: center;
}
.clock {
width: 16%;
height: 50%;
background-color: #8a5898;
display: flex;
justify-content: center;
align-items: center;
}
#d2 {
margin-left: 20%;
margin-top: 13%;
border-radius: 10px;
width: 60%;
height: 60px;
background-color: #AF87A3;
display: flex;
align-items: center;
justify-content: center;
}
#msText {
color: #EADCBE;
font-size: 200%;
margin-left: 5%;
}
#sTian{
color: #EADCBE;
font-size: 200%;
}
#sShi{
color: #EADCBE;
font-size: 200%;
}
#sFen{
color: #EADCBE;
font-size: 200%;
}
#sMiao{
color: #EADCBE;
font-size: 200%;
}
</style>
</head>
<body>
<div id="d2">
<span id="msText">双十一秒杀倒计时</span>
</div>
<div id="d1">
<div class="clock" id="tian">
<span id="sTian"></span>
</div>
<div class="clock" id="shi">
<span id="sShi"></span>
</div>
<div class="clock" id="fen">
<span id="sFen"></span>
</div>
<div class="clock" id="miao">
<span id="sMiao"></span>
</div>
</div>
</body>
<script>
setInterval(function() {
var now = new Date().getTime();
var end = new Date("2022-11-11 00:00:00").getTime();
var time = end - now;
if (time < 0) {
return;
}
var days = parseInt(time / 1000 / 60 / 60 / 24);
var hours = parseInt(time / 1000 / 60 / 60 % 24);
var minutes = parseInt(time / 1000 / 60 % 60);
var seconds = parseInt(time / 1000 % 60);
document.getElementById("sTian").innerHTML = days + "天";
document.getElementById("sShi").innerHTML = hours + "时";
document.getElementById("sFen").innerHTML = minutes + "分";
document.getElementById("sMiao").innerHTML = seconds + "秒";
}, 1000);
</script>
</html>
省市区三级联动

<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<select name="" id="province" onchange="selectProvince()">
<option value="">--请选择省份--</option>
<option value="">陕西</option>
<option value="">河南</option>
</select>
<select name="" id="city" onchange="selectCity()">
<option value="">--请选择城市--</option>
</select>
<select name="" id="district">
<option value="">--请选择区--</option>
</select>
</body>
<script>
var provinces = document.getElementById("province");
var citys = document.getElementById("city");
var districts = document.getElementById("district");
var cityArr = [
[],
["西安", "商洛"],
["郑州", "洛阳"]
];
function selectProvince() {
citys.length = 1;
districts.length = 1;
provinceIndex = provinces.selectedIndex;
var cityNames = cityArr[provinceIndex];
for (let i = 0; i < cityNames.length; i++) {
var option = document.createElement("option");
option.innerText = cityNames[i];
citys.appendChild(option);
}
}
var districtArr1 = [
[],
["长安区", "高新区"],
["商州区", "洛南县"]
];
var districtArr2 = [
[],
["中原区", "二七区"],
["老城区", "西工区"]
];
function selectCity() {
districts.length = 1;
if (provinceIndex == 1) {
var cityIndex = citys.selectedIndex;
var districtNames1 = districtArr1[cityIndex];
for (let i = 0; i < districtNames1.length; i++) {
var option = document.createElement("option");
option.innerText = districtNames1[i];
districts.appendChild(option);
}
} else if (provinceIndex == 2) {
var cityIndex = citys.selectedIndex;
var districtNames2 = districtArr2[cityIndex];
for (let i = 0; i < districtArr2.length; i++) {
var option = document.createElement("option");
option.innerText = districtNames2[i];
districts.appendChild(option);
}
}
}
</script>
</html>
手机验证码倒计时
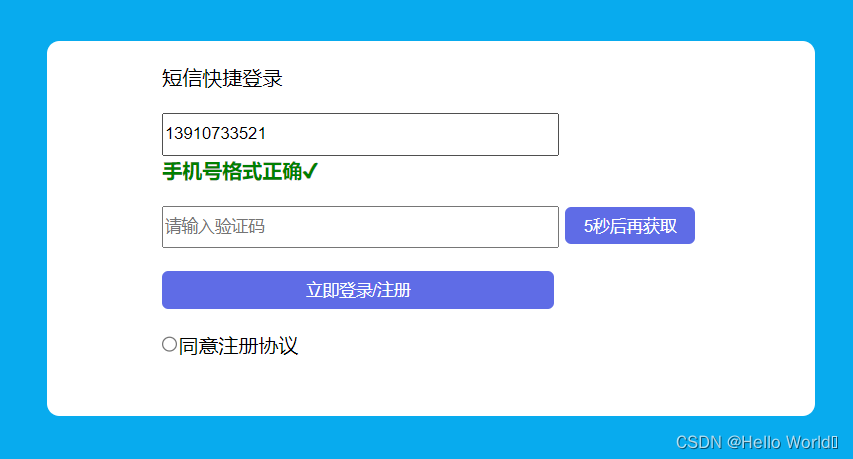
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
* {
margin: 0;
padding: 0;
}
body {
background-color: #08ABEE;
}
#d1 {
margin-left: 30%;
margin-top: 10%;
border-radius: 10px;
width: 40%;
height: 300px;
background-color: white;
display: flex;
flex-direction: column;
justify-content: flex-start;
}
.c1 {
margin-top: 3%;
margin-left: 15%;
}
#tel {
width: 60%;
height: 30px;
}
#i2 {
width: 60%;
height: 30px;
}
#b1 {
width: 20%;
height: 30px;
}
#b2 {
width: 60%;
height: 30px;
}
button {
color: white;
border: 0;
border-radius: 5px;
background-color: #5F6CE6;
}
</style>
</head>
<body>
<div id="d1">
<div id="f1" class="c1">
短信快捷登录
</div>
<div class="c1">
<form action="#" method="GET">
<input type="text" id="tel" name="phone" value="" placeholder="请输入手机号(11位)" onblur="checkTel()">
</form>
<span id="telText"></span>
</div>
<div class="c1">
<input type="" id="i2" name="yzm" placeholder="请输入验证码">
<button id="b1">获取验证码</button>
</div>
<div class="c1">
<button id="b2">立即登录/注册</button>
</div>
<div class="c1">
<input type="radio" name="" id="ty"><label for="ty">同意注册协议</label>
</div>
</div>
</body>
<script type="text/javascript">
function checkTel() {
var regx = /^1[0-9]{10}$/;
var value = document.getElementsByName("phone")[0].value;
var flag = regx.test(value);
var telText = document.getElementById("telText");
if (flag) {
telText.innerHTML = "<b style='color:green'>手机号格式正确✔</b>";
} else {
telText.innerHTML = "<b style='color:red'>手机号格式错误✘</b>";
}
return flag;
}
var butYzm = document.getElementById("b1");
var time = 5;
var timer = null;
butYzm.addEventListener("click", function() {
this.disabled = true;
if (checkTel()) {
timer = setInterval(function() {
if (time == 0) {
clearInterval(timer);
butYzm.disabled = false;
butYzm.innerHTML = "获取验证码";
time = 5;
} else {
butYzm.innerHTML = time + "秒后再获取";
time--;
}
}, 1000);
} else {
alert("请输入手机号");
}
});
</script>
</html>