目录
上一篇博客:
项目结构:
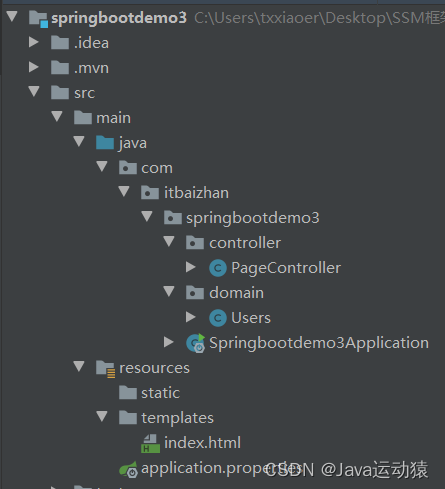
一、Thymeleaf_Thymeleaf入门
即Thymeleaf是html文件,html文件显示静态效果,jsp文件显示动态效果,而Thymeleaf文件既可以显示静态效果也可以显示动态效果。
<!--添加Thymeleaf起步依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starterthymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starterweb</artifactId>
</dependency>
3.创建视图index.html
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org"> <head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- 静态页面显示程序员,动态页面使用后端传来的msg数据代替 -->
<!-- thymeleaf支持el表达式 -->
<h2 th:text="${msg}">程序员</h2>
</body>
</html>
4.template中的html文件不能直接访问,需要编写Controller跳转到页面中
@Controller
public class PageController {
// 页面跳转
@GetMapping("/show")
public String showPage(Model model){
model.addAttribute("msg","Hello Thymeleaf");
return "index";
}
}
5.在application.properties配置文件中配置
#日志格式
logging.pattern.console=%d{HH:mm:ss.SSS}
%clr(%-5level) --- [%-15thread]
%cyan(%-50logger{50}):%msg%n
7.结果显示:
二、Thymeleaf_变量输出
语法 | 作用 |
th:text | 将model中的值作为内容放入标签中 |
th:value | 将model中的值放入input标签的value属性中 |
1.准备模型数据:
@GetMapping("/show")
public String showPage(Model model){
model.addAttribute("msg","Hello Thymeleaf");
return "index";
}
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- 静态页面下显示程序员,动态页面使用后端传来的数据 -->
<!-- thymeleaf支持el表达式 -->
<h2 th:text="${msg}">程序员</h2>
<input th:value="${msg}">
</body>
</html>
7.结果显示:
三、Thymeleaf_操作字符串
Thymeleaf提供了一些内置对象可以操作数据,内置对象可直接在模板中使用,这些对象是以#引用的,操作字符串的内置对象为strings。
方法 | 说明 |
${#strings.isEmpty(key)}
|
判断字符串是否为空,如果为空返回
true
,否则返回
false
|
${#strings.contains(msg,'T')}
|
判断字符串是否包含指定的子串,如果包含返回
true
,否则返回false
|
${#strings.startsWith(msg,'a')}
|
判断当前字符串是否以子串开头,如果是返回
true
,否则返回false
|
${#strings.endsWith(msg,'a')}
|
判断当前字符串是否以子串结尾,如果是返回
true
,否则返回false
|
${#strings.length(msg)}
|
返回字符串的长度
|
${#strings.indexOf(msg,'h')}
|
查找子串的位置,并返回该子串的下标,如果没找到则返回
-1
|
${#strings.substring(msg,2,5)}
|
截取子串,用法与
JDK
的
subString
方法相同
|
${#strings.toUpperCase(msg)}
|
字符串转大写
|
${#strings.toLowerCase(msg)
|
字符串转小写
|
1.数据模型:
@GetMapping("/show")
public String showPage(Model model){
model.addAttribute("msg","Hello Thymeleaf");
return "index";
}
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<span th:text="${#strings.isEmpty(msg)}"></span><br>
<span th:text="${#strings.contains(msg,'s')}"></span><br>
<span th:text="${#strings.length(msg)}"></span><br>
<span th:text="${#strings.toUpperCase(msg)}"></span><br>
<span th:text="${#strings.substring(msg,2,5)}"></span><br>
</body>
</html>
4.结果显示:
四、Thymeleaf_操作时间
操作时间的内置对象为dates
方法 | 说明 |
${#dates.format(key)}
|
格式化日期,默认的以浏览器默认语言为格式化标准
|
${#dates.format(key,'yyyy/MM/dd')}
|
按照自定义的格式做日期转换
|
${#dates.year(key)}
| 取年 |
${#dates.month(key)}
| 取月 |
${#dates.day(key)}
| 取日 |
1.准备数据:
@GetMapping("/show")
public String showPage(Model model){
//这里时间,年是从1990年开始算起,130即2030年,月从0开始算起,即这里的1指的是2月
model.addAttribute("date",new Date(130,1,1));
return "index";
}
2.使用内置对象操作时间
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<span th:text="${#dates.format(date)}"></span><br>
<span th:text="${#dates.format(date,'yyyy/MM/dd')}"></span><br>
<span th:text="${#dates.year(date)}"></span><br>
<span th:text="${#dates.month(date)}"></span><br>
<span th:text="${#dates.day(date)}"></span><br>
</body>
</html>
4.结果显示:
五、Thymeleaf_条件判断
1. 单分支if条件判断
语法 | 作用 |
th:if | 条件判断 |
1.准备数据模型
@GetMapping("/show")
public String showPage(Model model){
model.addAttribute("sex","女");
return "index";
}
2.进行条件判断
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<div>
<span th:if="${sex} == '女'">性别:女</span>
<span th:if="${sex} == '男'">性别:男</span>
</div>
</body>
</html>
4.结果显示:
2.多分支switch-case条件判断
语法 | 作用 |
th:switch/th:case
|
th:switch/th:case
与
Java
中的
switch
语句等效。
th:case="*"
表示
Java
中switch的
default
,即没有
case
的值为
true
时显示
th:case="*"
的内容。
|
1.准备数据模型
@GetMapping("/show")
public String showPage(Model model){
model.addAttribute("id","12");
return "index";
}
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<div th:switch="${id}">
<span th:case="1">ID为1</span>
<span th:case="2">ID为2</span>
<span th:case="3">ID为3</span>
<span th:case="*">ID为*</span>
</div>
</body>
</html>
4.结果显示:(因为传入的数12不在case案例中,所以返回case=“*”的结果)
六、Thymeleaf_迭代遍历
语法 | 作用 |
th:each | 迭代器,用于循环迭代集合 |
1.遍历集合
1.编写Users实体类
public class Users {
private String id;
private String name;
private int age;
// 省略getter/setter/构造方法
}
2.准备数据
@GetMapping("/show")
public String showPage(Model model){
List<Users> users = new ArrayList<>();
users.add(new Users("1","sxt",23));
users.add(new Users("2","baizhan",22));
users.add(new Users("3","admin",25));
model.addAttribute("users",users);
return "index";
}
3.在页面中展示数据
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<table border="1" width="50%">
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
<!-- 遍历集合的每一项起名为user -->
<tr th:each="user : ${users}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
</tr>
</table>
</body>
</html>
4.启动项目,访问http://localhost:8080/show及页面
5.结果显示:
2.使用状态变量
thymeleaf将遍历的状态变量封装到一个对象中,通过该对象的属性可以获取状态变量:
状态变量 | 含义 |
indexl | 当前迭代器的索引,从0开始 |
count | 当前迭代器对象的技术,从1开始 |
size | 被迭代对象的长度 |
odd/even | 布尔值,当前循环是否是偶数/奇数,从0开始 |
first |
布尔值,当前循环的是否是第一条,如果是返回
true
,否则返回
false
|
last |
布尔值,当前循环的是否是最后一条,如果是返回
true
,否则返回
false
|
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<table border="1" width="50%">
<!-- 冒号前的第一个对象是遍历每一项的别名,第二个对象就是封装状态变量的对象 -->
<tr th:each="user,status : ${users}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
<td th:text="${status.index}"></td>
<td th:text="${status.count}"></td>
<td th:text="${status.size}"></td>
<td th:text="${status.odd}"></td>
<td th:text="${status.even}"></td>
<td th:text="${status.first}"></td>
<td th:text="${status.last}"></td>
</tr>
</table>
</body>
</html>
4.启动项目,访问http://localhost:8080/show及页面
5.结果显示:
3.遍历Map
1.准备数据:
@GetMapping("/show")
public String showPage(Model model){
//map对象类型
Map<String,Users> map = new HashMap();
map.put("user1",new Users("1","sxt",23));
map.put("user2",new Users("2","tx",25));
map.put("user3",new Users("3","xyj",20));
model.addAttribute("map",map);
return "index";
}
2.遍历map:
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<table border="1" width="50%">
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Key</th>
</tr>
<!-- 遍历出的是一个键值对对象,map是遍历每一项的别名,key获取键,value获取值 -->
<tr th:each="map : ${map}">
<td th:text="${map.value.id}"></td>
<td th:text="${map.value.name}"></td>
<td th:text="${map.value.age}"></td>
<td th:text="${map.key}"></td>
</tr>
</table>
</body>
</html>
4.结果显示:
七、Thymeleaf_获取域中的数据
thymeleaf也可以获取request,session,application域中的数据,方法如下:
@GetMapping("/show")
public String showPage(Model model, HttpServletRequest request, HttpSession session){
//获取域中的数据
request.setAttribute("req","HttpServletRequest");
session.setAttribute("ses","HttpSession");
session.getServletContext().setAttribute("app","application");
return "index";
}
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<hr/>
request:<span th:text="${#request.getAttribute('req')}"></span>
request2:<span th:text="${#httpServletRequest.getAttribute('req')}"></span>
<hr/>
session1:<span th:text="${session.ses}"></span>
session2:<span th:text="${#httpSession.getAttribute('ses')}"></span>
<hr/>
application1:<span th:text="${application.app}"/>
application2:<span th:text="${#servletContext.getAttribute('app')}"/>
</body>
</html>
4.结果显示:
八、Thymeleaf_URL写法
<a th:href="@{http://www.baidu.com}">百度</a>
1.在路径中添加参数
@GetMapping("/show")
public String showPage(Model model, HttpServletRequest request, HttpSession session){
model.addAttribute("id","100");
model.addAttribute("name","zhangsan");
return "index";
}
@GetMapping("/show2")
@ResponseBody
public String show2(String id,String name){
return id+":"+name;
}
3.在URL中添加参数
<!DOCTYPE html>
<!-- 引入thymeleaf命名空间,方便使用thymeleaf属性 -->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>thymeleaf入门</title>
</head>
<body>
<!-- thymeleaf支持el表达式 -->
<a th:href="@{http://www.baidu.com}">百度</a>
<a th:href="@{show2?id=1&name=zhangsan}">静态参数一</a>
<a th:href="@{show2(id=1,name=zhangsan)}">静态参数二</a>
<a th:href="@{'show2?id='+${id}+'$name='+${name}}">动态参数一</a>
<a th:href="@{show2(id=${id},name=${name})}">动态参数二</a>
</body>
</html>
5.结果显示:

2. 在RESTful风格路径中添加参数
@GetMapping("/show3/{id}/{name}")
@ResponseBody
public String show3(@PathVariable String id,@PathVariable String name){
return id+":"+name;
}
2.在URL中添加参数
<a th:href="@{/show3/{id}/{name}(id=${id},name=${name})}">RESTful风格传递参数方式</a>
4.结果显示:

九、知识点整理:
1.Thymeleaf文件的后缀名是“.html ”
2.关于Thymeleaf,“在SpringBoot中推荐使用Thymeleaf编写动态页面。”
3.在Thymeleaf中,使用"th:value"属性可以将model中的值放入 <input> 的value 属性中
4.在Thymeleaf中,操作字符串的内置对象为“strings”
5.在Thymeleaf中,操作时间的内置对象为“dates ”
6.在Thymeleaf中,表示条件判断的属性为“th:if”
7.在Thymeleaf中, th:case="*" 表示“没有case的值为true时显示 th:case="*" 的内容”
8.在Thymeleaf中,遍历集合的属性为 “th:each ”
9.在Thymeleaf中,遍历集合时,迭代器索引的状态变量为“index”
10.在Thymeleaf中,遍历Map集合时,遍历出的每一项是“键值对”