<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
*{
margin:0;
padding:0;
}
.all{
width:590px;
height:470px;
margin:100px auto;
border:1px solid black;
position:relative;
overflow:hidden;
}
.screen{
width:590px;
height:470px;
background-color:pink;
}
.all .screen ul{
list-style:none;
width:3540px;/*六张图片的宽度*/
position:absolute;
left:0;
top:0;
}
.all .screen ul li{
float:left;
}
.all .screen ol{
position:absolute;
right:10px;
bottom:10px;
list-style:none;
}
.all .screen ol li{
float:left;
width:20px;
height:20px;
background-color:white;
border:1px solid #ccc;
margin-left:10px;
cursor:pointer;
line-height:20px;
text-align:center;
}
.all .screen ol li.current{
background-color:#DB192A;
}
.all #arr{
diaplay:none;
}
.all #arr span{
display:inline-block;
position:absolute;
width:40px;
height:70px;
background-color:rgba(0, 0, 0, 0.3);
font-size:40px;
corlor:rgba(255, 255, 255, 0.7);
text-align:center;
line-height:70px;
cursor:pointer;
}
.all #arr #left{
left:0;
top:200px;
}
.all #arr #right{
right:0;
top:200px;
}
</style>
</head>
<body>
<div class="all" id="box">
<div class="screen">
<ul>
<li><img src="images/luobo1.jpg" width="590" height="470"/></li>
<li><img src="images/luobo2.jpg" width="590" height="470"/></li>
<li><img src="images/luobo3.jpg" width="590" height="470"/></li>
<li><img src="images/luobo4.jpg" width="590" height="470"/></li>
<li><img src="images/luobo5.jpg" width="590" height="470"/></li>
</ul>
<ol>
</ol>
</div>
<div id="arr"><span id="left"><</span><span id="right">></span></div>
</div>
<script>
function my$(id){
return document.getElementById(id);
}
//动画函数(匀速)-----任意一个元素移动到指定的目标位置
function animate(element, target) {
//先清理定时器
clearInterval(element.timeId);
//定时器的id存储到对象的一个属性中,为了清理定时器(只能产生一个定时器)
element.timeId = setInterval(function () {
//获取div的当前的位置
var current = element.offsetLeft;//数字类型,没有px
//div每次移动多少像素
var step = 10;
//判断向左走还是向右走
step = current < target ? step : -step;
//每次移动后的距离
current += step;
//判断当前移动后的位置是否到达目标位置
if (Math.abs(target - current) > Math.abs(step)) {
element.style.left = current + "px";
} else {
//清理定时器
clearInterval(element.timeId);
element.style.left = target + "px";
}
}, 20);
}
//获取最外面的div
var box=my$("box");
//获取screen
var screen=box.children[0];
//获取screen的宽度
var imgWidth=screen.offsetWidth;
//获取ul
var ulObj=screen.children[0];
//获取ul中所有的li
var list=ulObj.children;
//获取ol
var olObj=screen.children[1];
//焦点的div
var arr=my$("arr");
var index=0;
//创建小按钮----根据ul中的li的个数
for(var i=0;i<list.length;i++){
//创建li标签,加入ol中
var liObj=document.createElement("li");
olObj.appendChild(liObj);
liObj.innerHTML=(i+1);
//在每个ol中的li标签上添加一个自定义属性,存储索引值
liObj.setAttribute("index",i);
//注册鼠标进入事件
liObj.onmouseover=function(){
//先去掉所有的ol中的li的背景颜色
for(var j=0;j<olObj.children.length;j++){
olObj.children[j].removeAttribute("class");
}
//设置当前鼠标进来的li的背景颜色
this.className="current";
//获取鼠标进入的li的当前索引值
index=this.getAttribute("index");
//移动ul
animate(ulObj,-index*imgWidth);
};
}
//设置ol中第一个li有背景颜色
olObj.children[0].className="current";
//克隆ul中第一个li,加入到ul中的最后
ulObj.appendChild(ulObj.children[0].cloneNode(true));
//自动播放
var timeId=setInterval(clickHandle,2000);
//鼠标进入到box的div显示左右的焦点
box.onmouseover=function(){
arr.style.display="block";
//鼠标进入清楚定时器
clearInterval(timeId);
};
//鼠标离开box的div隐藏左右的焦点
box.onmouseout=function(){
arr.style.display="none";
timeId=setInterval(clickHandle,2000);
};
//右边按钮
my$("right").onclick=clickHandle;
function clickHandle(){
if(index==list.length-1){
index=0;
ulObj.style.left=0+"px";
}
index++;
animate(ulObj,-index*imgWidth);
//如果index=5说明此时显示第6个图(同第一张图一样),第一个小按钮有颜色
if(index==list.length-1){
//第五个按钮颜色被去掉
olObj.children[olObj.children.length-1].className="";
//第一个按钮颜色设置上
olObj.children[0].className="current";
}else{
//去掉所有的小按钮的背景颜色
for(var i=0;i<olObj.children.length;i++){
olObj.children[i].removeAttribute("class");
}
olObj.children[index].className="current";
}
}
//左边按钮
my$("left").onclick=function(){
if(index==0){
index=5;
ulObj.style.left=-index*imgWidth+"px";
}
index--;
animate(ulObj,-index*imgWidth);
//去掉所有小按钮的颜色
for(var i=0;i<olObj.children.length;i++){
olObj.children[i].removeAttribute("class");
}
//设置当前的索引对应的按钮的颜色
olObj.children[index].className="current";
};
</script>
</body>
</html>
javascript轮播图的实现
最新推荐文章于 2022-07-06 16:13:53 发布
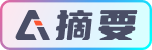