加载测试专用属性
我们可以在配置文件中配置所有的属性,但是假定我的需求是我在做测试的时候,我需要临时的去加一些数据或者改一些属性的话,应该怎么去做呢?其实可以用我们前面的profile去给它加属性,但是现在的需求特别简单,就是只在这个测试中有效,并不影响到所有的测试,我想加一个临时属性。
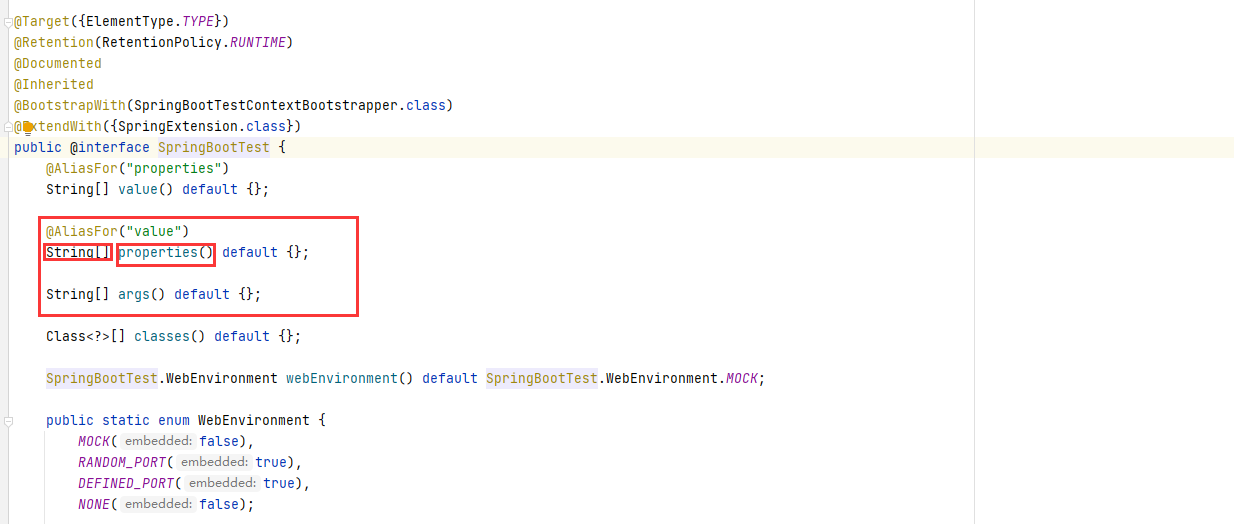
- 在启动测试环境时可以通过properties参数设置测试环境专用的属性
properties属性可以为当前测试用例添加临时的属性配置,不用在配置文件中写了,这样比多环境开发中的测试环境影响范围更小,仅对当前测试类有效。
//这里的配置能够覆盖配置文件的信息
@SpringBootTest(properties = {"test.prop=testValue1"})
class PropertiesAndArgsTest {
@Value("${test.prop}")
private String msg;
@Test
void contextLoads() {
System.out.println(msg);
}
}
- 在启动测试环境时可以通过args参数设置测试环境专用的传入参数
args属性可以为当前测试用例添加临时的命令行参数。
//同样可以覆盖配置文件信息
@SpringBootTest(args={"--test.prop=testValue2"})
public class PropertiesAndArgsTest {
@Value("${test.prop}")
private String msg;
@Test
void testProperties(){
System.out.println(msg);
}
}
当 args参数与 properties参数设置共存时, args属性配置优先于properties属性配置加载。
加载测试专用配置
我们加载临时的一些配置数据,但是如果临时加了一些bean能不能用呢?
- 1.创建专用的测试环境配置类
package com.zqf.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/*
仅用于演示当前实验效果,实际开发不能这么注入String类型的数据
*/
@Configuration
public class MsgConfig {
@Bean //String类型的bean,Strng本身就是个包装类
public String msg(){
return "bean msg";
}
}
当前测试用例实际上是模拟了一套spring运行的环境,那在这ConfigurationTest类,我们这套环境不仅要加载上面的这些信息也就是源码的信息,还要加载MsgConfig。
- 2.使用@Import注解实现导入测试环境专用的配置类
package com.zqf;
import com.zqf.config.MsgConfig;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.context.annotation.Import;
@SpringBootTest
@Import({MsgConfig.class}) //@Import(MsConfig.class)这个注解应该是把类加载到spring 容器里面啊
public class ConfigurationTest {
@Autowired
private String msg;
@Test
void testConfiguration(){
System.out.println(msg);
}
}
Web环境模拟测试
在我们的测试用例,我们可以对数据层业务层进行测试,那表现层能不能测呢?
启动web环境
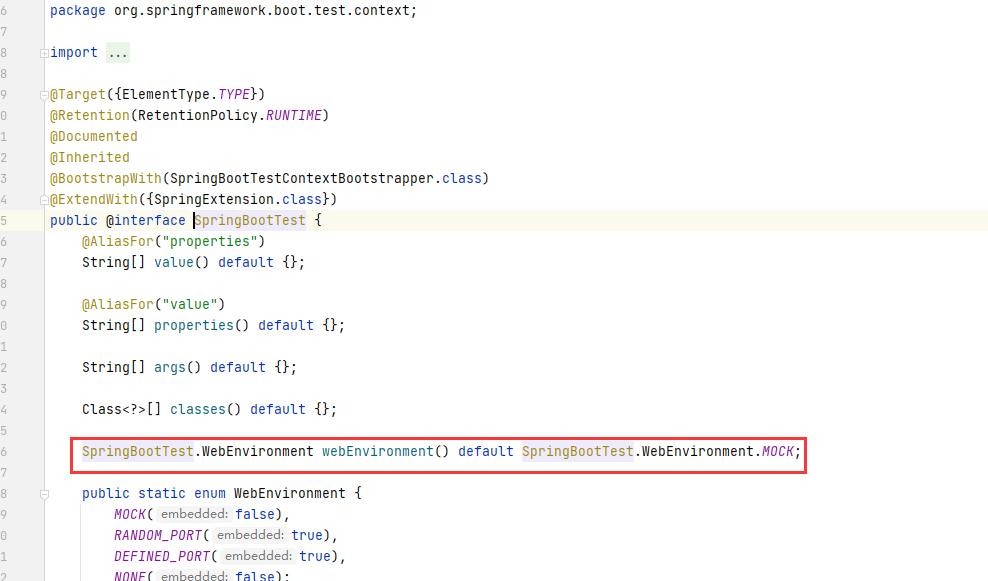
利用@SpringBootTest注解中webEnvironment属性设置在测试用例中启动web环境。
package com.zqf;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class WebTest {
@Test
void testRandomPort() {
System.out.println("123");
}
}
发送虚拟请求
- 创建Controller
package com.zqf.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/books")
public class BookController {
@GetMapping
public String getById() {
System.out.println("getById is running .....");
return "springboot";
}
}
在测试类里面是模拟的调用,在这里边我们要用spring给我们提供的虚拟调用的东西来做这件事儿,步骤比较固定,需要开启虚拟调用这套模式:在类名上添加@AutoConfigureMockMvc,这相当于一个开关。虚拟调用的对象叫MockMvc。
发起的mvc的虚拟调用,它并不是一个真正的请求调用,它是虚拟出来了一套Web环境,并在这套虚拟的环境中发了这么一个请求调用。
- 虚拟请求测试
package com.zqf;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
//1.使用 webEnvironment 属性开启web环境
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
//2.开启虚拟mvc调用
@AutoConfigureMockMvc
public class WebTest {
@Test
void testRandomPort() {
}
@Test
//注入虚拟mvc调用对象
public void testWeb(@Autowired MockMvc mvc) throws Exception {
//3.创建虚拟请求,当前访问/books
MockHttpServletRequestBuilder builder = MockMvcRequestBuilders.get("/books");
//执行请求
ResultActions actions = mvc.perform(builder);
}
}