<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=sc, initial-scale=1.0">
<title>Document</title>
<style>
.box1 .item{
display: inline-block;
border: 1px solid red;
width: 50px;
height: 20px;
}
</style>
</head>
<body>
<div class="box1">
<h2 class="page-header">点击切换颜色</h2>
<div class="item"></div>
<div class="item"></div>
<div class="item"></div>
</div>
<script>
//获取div元素
let items=document.getElementsByClassName('item');
//遍历并绑定事件
for (let i=0;i<items.length;i++){
items[i].onclick=function(){
//修改当前背景颜色
// this.style.background='pink'
items[i].style.background='pink';
}
}
/* {
let i=0;
items[i].onclick=function(){
//修改当前背景颜色
// this.style.background='pink'
items[i].style.background='pink';
}
}
{
let i=1;
items[i].onclick=function(){
//修改当前背景颜色
// this.style.background='pink'
items[i].style.background='pink';
}
}
{
let i=2;
items[i].onclick=function(){
//修改当前背景颜色
// this.style.background='pink'
items[i].style.background='pink';
}
}*/
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>变量的简化写法Document</title>
</head>
<body>
<script>
//ES6 允许在大括号里面,直接写入变量和函数,作为对象的属性和方法
//这样更为简便
let name='叶挺';
let change=function(){
console.log('我一定会加油进入IT行业的');
}
const me={
name,
change,
improve(){
console.log('努力总会看到效果的');
}
}
console.log(me);
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>变量的简化写法Document</title>
</head>
<body>
<script>
//ES6 允许在大括号里面,直接写入变量和函数,作为对象的属性和方法
//这样更为简便
let name='叶挺';
let change=function(){
console.log('我一定会加油进入IT行业的');
}
const me={
name,
change,
improve(){
console.log('努力总会看到效果的');
}
}
console.log(me);
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>参数的默认值</title>
</head>
<body>
<script>
//ES6运行给函数参数赋值初始值
//1.形参初始值 具有默认的参数,一般位置要靠后(潜规则)
// function add(a,b){
// return a+b;
// }
// let result=add(1,2)
// console.log(result,typeof result);
//2.与解构赋值结合
function connect({host='hh',username,password,port}){
console.log(host);
}
connect({
host:'atgigu.com',
username:'root',
password:'root',
prot:3306
})
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>箭头函数</title>
</head>
<body>
<script>
//ES6允许使用【箭头函数】
//声明一个函数
// let fun=function(){
// } 等于下面式子
// let fun=(a,b)=>{
// return a+b;
// }
// //调用函数
// // let result=fun(1,4);
// // console.log(result);
// //this是静态的,this始终指向声明时作用域下的this的值
// function get1(){
// console.log(this.name);
// }
// let get2=()=>{
// console.log(this.name);
// }
// //设置全局作用域对象的 name 属性
// window.name='高凡';
// const conpany={
// name:"gaofan"
// }
//直接调用
// get1();
// get2();
//call调用
// get1.call(conpany);
// get2.call(conpany);无论调用谁都是声明时哪个this的值
//.不能作为构造函数实例化对象
// let Person=(name,age)=>{
// this.name=name;
// this.age=age;
// }
// let me=new Person('xiao',30);
// console.log(me);
//3.不能使用arguments变量
//4.箭头函数的简写
//1.省略小括号,当形参只有一个的时候
// let add=n=>{
// return n+n;
// }
//2.省略大括号,当代码只有一跳语句的时候,此时return也必须省略
//而且语句执行完的值就是return
let add=n=>n*n;
console.log(add(8))
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>实践</title>
<style>
#ad{
width: 100px;
height: 100px;
background-color: #58a;
}
</style>
</head>
<body>
<div id="ad"></div>
<script>
//需求1 点击2s div变颜色
//获取元素
let ad=document.getElementById('ad');
ad.addEventListener("click",function(){
//定时器
setTimeout(()=>{
//修改背景颜色
this.style.background='pink';
},2000)
});
//需求2 从数组中返回偶数的元素
const arr=[1,3,4,5,6,100,25];
// const result=arr.filter(function(item){
// if (item%2===0) {
// return true;
// } else {
// return false;
// }
// });
const result=arr.filter(item=>item%2===0);
console.log(result);
//箭头函数适合与this无关的回调,定时器,数组的方法回调
//箭头函数不适合this有关的回调,事件回调,对象的方法
// {
// name:'您好',
// getName()=>{
// this.name;
// }
// }
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>实践</title>
<style>
#ad{
width: 100px;
height: 100px;
background-color: #58a;
}
</style>
</head>
<body>
<div id="ad"></div>
<script>
//需求1 点击2s div变颜色
//获取元素
let ad=document.getElementById('ad');
ad.addEventListener("click",function(){
//定时器
setTimeout(()=>{
//修改背景颜色
this.style.background='pink';
},2000)
});
//需求2 从数组中返回偶数的元素
const arr=[1,3,4,5,6,100,25];
// const result=arr.filter(function(item){
// if (item%2===0) {
// return true;
// } else {
// return false;
// }
// });
const result=arr.filter(item=>item%2===0);
console.log(result);
//箭头函数适合与this无关的回调,定时器,数组的方法回调
//箭头函数不适合this有关的回调,事件回调,对象的方法
// {
// name:'您好',
// getName()=>{
// this.name;
// }
// }
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>const 定义常量</title>
</head>
<body>
<script>
//声明常量
const school='江苏电子';
/*
注意事项*
1.一定要有初始值
const school;会报错
2.一般常量要大写(潜规则)
3.常量的值不能修改
school="大大";报错
4.块级作用域
{
const scll="adad"
}
console.log(scll)报错
5.对与数组和对象元素的修改,不算做对常量的修改,不会报错
*/
const TEAM=['YT','CJH','PJJ','JT'];
TEAM.push('XJ');
console.log(TEAM);
</script>
</body>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>sperd扩展运算符应用</title>
</head>
<body>
<div></div>
<div></div>
<div></div>
<script>
//1.数组的合并
// const py=['cjh','pjj','zyl','yyh'];
// const qr=['yjw','lst','wmy'];
// //老方法const qrpy=py.concat(qr);
// const qrpy=[...py,...qr];
// console.log(qrpy);
//2.数组的克隆
// const py=['cjh','pjj','zyl','yyh'];
// const hpy=[...py];
// console.log(hpy);
//3.将伪数组转为真正的数组 //伪数组:伪数组 (ArrayLike) ,又称类数组。是一个类似数组的对象,是一种按照索引存储数据且具有 length 属性的对象
const divs=document.querySelectorAll('div');
const divArr=[...divs];
console.log(divArr);
</script>
</body>
</html>
</html>
ES6-11第一天
最新推荐文章于 2024-11-11 11:05:35 发布
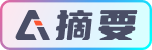