《锋迷商城》系列项目
链接: 《一》 项目搭建
链接: 《二》数据库的创建
链接: 《三》业务流程设计
链接: 《四》业务流程实现:用户管理
链接: 《五》逆向工程
链接: 《六》用户认证
文章目录
十二、商品详情展示
12.1 实现流程分析
点击首页推荐的商品,轮播图商品广告,商品列表页面点击商品,就会进入到商品的详情页面
12.2 接口开发
12.2.1 商品基本信息接口
sql
-- 查询商品详情
-- 查询商品的基本信息
SELECT * FROM product where product_id=3
-- 查询商品图片
SELECT * FROM product_img where item_id=3
-- 查询商品规格 sku
SELECT * FROM product_sku where product_id=3
由于都是单表操作 使用tkMapper解决
业务层
/**
* 查询商品的基本ctId
* @return
*/
ResultVO getProductBasicInfo(String productId);
-----------------------------------------------
@Transactional(propagation = Propagation.SUPPORTS)
public ResultVO getProductBasicInfo(String productId) {
//商品基本信息
Example example = new Example(Product.class);
Example.Criteria criteria = example.createCriteria();
criteria.andEqualTo("productId", productId)
.andEqualTo("productStatus", 1);
List<Product> products = productMapper.selectByExample(example);
if (products.size() > 0) {
//商品图片
Example example1 = new Example(ProductImg.class);
Example.Criteria criteria1 = example1.createCriteria();
criteria1.andEqualTo("itemId", productId);
List<ProductImg> productImgs = productImgMapper.selectByExample(example1);
//商品规格
Example example2 = new Example(ProductSku.class);
Example.Criteria criteria2 = example2.createCriteria();
criteria2.andEqualTo("productId", productId);
List<ProductSku> productSkus = productSkuMapper.selectByExample(example2);
Map<String, Object> map = new HashMap<>();
map.put("product", products.get(0));
map.put("productImgs", productImgs);
map.put("productSkus", productSkus);
return new ResultVO(ResStatus.OK, "success", map);
} else {
return new ResultVO(ResStatus.NO, "商品不存在", null);
}
}
控制层
@RestController
@CrossOrigin
@RequestMapping("/product")
@Api(value = "提供商品所需的接口",tags = "商品管理")
public class ProductController {
@Autowired
private ProductService productService;
@ApiOperation("商品详情信息")
@GetMapping("/detail-info/{pid}")
public ResultVO getProductBasicInfo(@PathVariable("pid") String pid){
return productService.getProductBasicInfo(pid);
}
}
12.2.2 商品参数接口
业务层
/**
* 获取商品详细参数
* @param productId
* @return
*/
ResultVO getProductParamsById(String productId);
---------------------------------------------------
@Override
public ResultVO getProductParamsById(String productId) {
Example example = new Example(ProductParams.class);
Example.Criteria criteria = example.createCriteria();
criteria.andEqualTo("productId", productId);
List<ProductParams> productParams = productParamsMapper.selectByExample(example);
if(productParams.size()>0){
return new ResultVO(ResStatus.OK,"success",productParams.get(0));
}else{
return new ResultVO(ResStatus.NO,"三无产品",null);
}
}
控制层
在ProductController中添加
@ApiOperation("商品详情信息")
@GetMapping("/detail-params/{pid}")
public ResultVO getProductParams(@PathVariable("pid") String pid){
return productService.getProductParamsById(pid);
}
12.2.3 商品评价接口
数据库和DAO层实现
-
sql
SELECT u.username,u.nickname,c.* FROM product_comments c inner JOIN users u on u.user_id=c.user_id where c.product_id=3
-
ProductCommentsVO封装类
@Data @AllArgsConstructor @NoArgsConstructor public class ProductCommentsVO { private String commId; private String productId; private String productName; private String orderItemId; private Integer isAnonymous; private Integer commType; private Integer commLevel; private String commContent; private String commImgs; private Date sepcName; private Integer replyStatus; private String replyContent; private Date replyTime; private Integer isShow; //封装评论对应的用户数据 private String userId; private String username; private String nickname; private String userImg; }
-
DAO层
@Repository public interface ProductCommentsMapper extends GeneralDAO<ProductComments> { List<ProductCommentsVO> selectCommentsByProductId(String Id); }
-
mapper.xml 映射文件
<resultMap id="ProductCommentsVOMap" type="com.sjtest.fmmall.entity.ProductCommentsVO"> <id column="comm_id" jdbcType="VARCHAR" property="commId" /> <result column="product_id" jdbcType="VARCHAR" property="productId" /> <result column="product_name" jdbcType="VARCHAR" property="productName" /> <result column="order_item_id" jdbcType="VARCHAR" property="orderItemId" /> <result column="is_anonymous" jdbcType="INTEGER" property="isAnonymous" /> <result column="comm_type" jdbcType="INTEGER" property="commType" /> <result column="comm_level" jdbcType="INTEGER" property="commLevel" /> <result column="comm_content" jdbcType="VARCHAR" property="commContent" /> <result column="comm_imgs" jdbcType="VARCHAR" property="commImgs" /> <result column="sepc_name" jdbcType="TIMESTAMP" property="sepcName" /> <result column="reply_status" jdbcType="INTEGER" property="replyStatus" /> <result column="reply_content" jdbcType="VARCHAR" property="replyContent" /> <result column="reply_time" jdbcType="TIMESTAMP" property="replyTime" /> <result column="is_show" jdbcType="INTEGER" property="isShow" /> <result column="user_id" jdbcType="VARCHAR" property="userId" /> <result column="username" jdbcType="VARCHAR" property="username" /> <result column="nickname" jdbcType="VARCHAR" property="nickname" /> <result column="user_img" jdbcType="VARCHAR" property="userImg" /> </resultMap> <select id="selectCommentsByProductId" resultMap="ProductCommentsVOMap"> SELECT u.username, u.nickname, u.user_img, c.comm_id, c.product_id, c.product_name, c.order_item_id, c.user_id, c.is_anonymous, c.comm_type, c.comm_level, c.comm_content, c.comm_imgs, c.sepc_name, c.reply_status, c.reply_content, c.reply_time, c.is_show FROM product_comments c inner JOIN users u on u.user_id=c.user_id where c.product_id=#{Id} </select>
业务层实现
public interface ProductCommontsService {
ResultVO listCommontsByProductId(String productId);
}
-------------------------------------------------
@Service
public class ProductCommontsServiceImpl implements ProductCommontsService {
@Autowired
private ProductCommentsMapper productCommentsMapper;
@Override
public ResultVO listCommontsByProductId(String productId) {
List<ProductCommentsVO> productCommentsVOS = productCommentsMapper.selectCommentsByProductId(productId);
ResultVO resultVO = new ResultVO(ResStatus.OK, "success", productCommentsVOS);
return resultVO;
}
}
控制层实现
在ProductController中添加
@Autowired
private ProductCommontsService productCommontsService;
@ApiOperation("商品评价信息")
@GetMapping("/detail-commonts/{pid}")
public ResultVO getProductCommonts(@PathVariable("pid") String pid){
return productCommontsService.listCommontsByProductId(pid);
}
12.3.4商品评论分页及统计信息
流程分析
改造ProductCommentsMapper 接口
改造ProductCommentsMapper.xml 映射文件
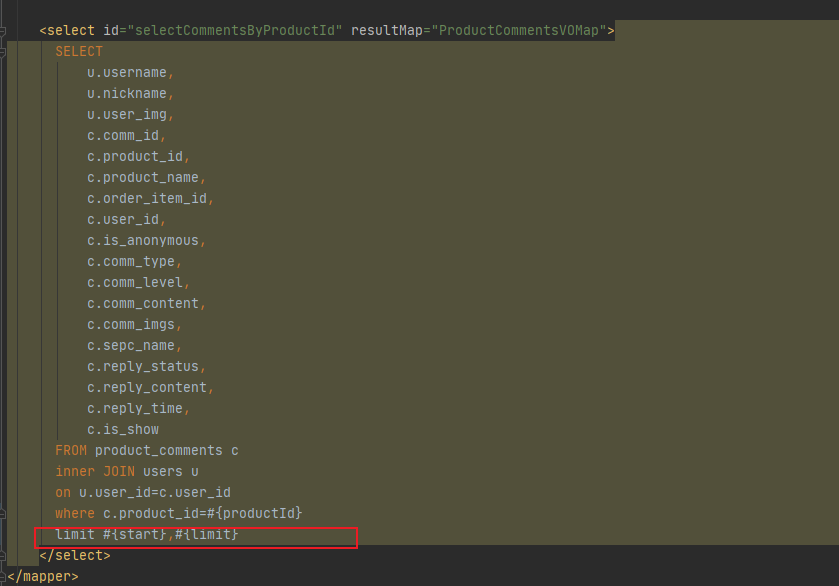
改造业务层
public interface ProductCommontsService {
ResultVO listCommontsByProductId(String productId,int pageNum,int limit);
}
/**
* 显示商品评论
* @param productId
* @return
*/
ResultVO getCommentsCountByProductId(String productId);
---------------------------------------------------
@Service
public class ProductCommontsServiceImpl implements ProductCommontsService {
@Autowired
private ProductCommentsMapper productCommentsMapper;
@Override
public ResultVO listCommontsByProductId(String productId,int pageNum,int limit) {
// List<ProductCommentsVO> productCommentsVOS = productCommentsMapper.selectCommentsByProductId(productId);
// 根据商品id查询总记录数
Example example = new Example(ProductComments.class);
Example.Criteria criteria = example.createCriteria();
criteria.andEqualTo("productId",productId);
int count = productCommentsMapper.selectCountByExample(example);
// 计算总页数
int pageCount = count % limit == 0 ? count / limit : count / limit + 1;
//查询当前页的数据
int start = (pageNum - 1) * limit;
List<ProductCommentsVO> list = productCommentsMapper.selectCommentsByProductId(productId, start, limit);
ResultVO resultVO = new ResultVO(ResStatus.OK, "success", new PageHelper<ProductCommentsVO>(count,pageCount,list));
return resultVO;
}
}
@Override
public ResultVO getCommentsCountByProductId(String productId) {
Example example = new Example(ProductComments.class);
Example.Criteria criteria = example.createCriteria();
criteria.andEqualTo("productId",productId);
int total = productCommentsMapper.selectCountByExample(example);
//好评
Example example2 = new Example(ProductComments.class);
Example.Criteria criteria2 = example2.createCriteria();
criteria2.andEqualTo("productId",productId);
criteria2.andEqualTo("commType",1);
int goodTotal = productCommentsMapper.selectCountByExample(example2);
//中评
Example example3 = new Example(ProductComments.class);
Example.Criteria criteria3 = example3.createCriteria();
criteria3.andEqualTo("productId",productId);
criteria3.andEqualTo("commType",0);
int midTotal = productCommentsMapper.selectCountByExample(example3);
//差评
Example example4 = new Example(ProductComments.class);
Example.Criteria criteria4 = example4.createCriteria();
criteria4.andEqualTo("productId",productId);
criteria4.andEqualTo("commType",-1);
int bedTotal = productCommentsMapper.selectCountByExample(example4);
//好评率
double percent = (Double.parseDouble(goodTotal + "") / Double.parseDouble(total + ""))*100;
String percentValue = String.format("%.2f", percent);
Map<String, Object> map = new HashMap<>();
map.put("total",total);
map.put("goodTotal",goodTotal);
map.put("midTotal",midTotal);
map.put("bedTotal",bedTotal);
map.put("percent",percentValue);
return new ResultVO(ResStatus.OK,"success",map);
}
控制层改造
@ApiOperation("商品评价信息")
// @ApiImplicitParams({
// @ApiImplicitParam(dataType = "int",name = "pageNum",value = "当前页码",required = true),
// @ApiImplicitParam(dataType = "int",name = "limit",value = "每页显示条数",required = true)
// })
@GetMapping("/detail-commonts/{pid}")
public ResultVO getProductCommonts(@PathVariable("pid") String pid, int pageNum, int limit){
return productCommontsService.listCommontsByProductId(pid,pageNum,limit);
}
@ApiOperation("商品评价统计信息")
@GetMapping("/detail-commontscount/{pid}")
public ResultVO getCommentsCount(@PathVariable("pid") String pid){
return productCommontsService.getCommentsCountByProductId(pid);
}