不要让 except 裸奔!裸奔很爽,但有隐忧。
(本笔记适合学完 Python 五大基本数据类型,有了些 Python 基础的 coder 翻阅)
-
Python 官网:https://www.python.org/
-
Free:大咖免费“圣经”教程《 python 完全自学教程》,不仅仅是基础那么简单……
地址:https://lqpybook.readthedocs.io/
自学并不是什么神秘的东西,一个人一辈子自学的时间总是比在学校学习的时间长,没有老师的时候总是比有老师的时候多。
—— 华罗庚

本文质量分:
CSDN质量分查询入口:http://www.csdn.net/qc
◆ Don’t bare except
1、记下这篇笔记的契机——
今天读到齐老师的分享文章“ Python 中的 EAFP 原则——一个编程实践中的原则”,好有感触。因为——
我为图方便,一直在
try:
…
except Exception as error:
…
没有预见到那样做的隐忧。此后,一定谨记齐老教诲,Don’t “bare except”!(不要让 except 裸奔!)
只要代码有错误捕获,铁定要标明预见到的错误类型。
要了解 try … except …,不让 except 裸奔,我们得熟悉常见的 Python 错误/异常。下面,我们一起来了解 Python 的十大错误/异常——
2、Python 十大常见错误/异常
2.1 TypeError
类型错误:当操作对象或函数参数使用了 不适当类型的对象时,引发 TypeError 。
例如——
>>> int(sorted)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: int() argument must be a string, a bytes-like object or a real number, not 'builtin_function_or_method'
>>> tuple(45)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
>>> list(789)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
>>>
- int ,由帮助文档文档可知,参数只可以是数字( int, float )、字符串 str 。
Convert a number or string to an integer, or return 0 if no arguments. (摘录 help(int) 文本 )
- tuple ,参数只可以是缺省或者可迭代对象。
If no argument is given, the constructor returns an empty tuple.
If iterable is specified the tuple is initialized from iterable’s items.
If the argument is a tuple, the return value is the same object. (摘录 help(tuple) 文本 )
- list,只可以不给参数或给出可迭代对象。
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified. (摘录 help(list) 文本 )
试图用函数 sorted 作 int 的参数,必报 TypeError 错误。用 int 作 tuple 、list 的参数,也是必定报错 TypeError 的。
2.2 ValueError
值错误:即操作对象或参数对象值不“合法”。当函数参数对象或操作的对象具有正确的类型但不合法时触发 ValueError 。
如——
int('g')
ValueError: invalid literal for int() with base 10: ‘g’
如果 int 的参数是字符串,一定要是整型数字字符串,否则报 ValueError 。
2.3 NameError
名字错误:即变量名错误。当尝试访问一个未定义的变量时,会抛出 NameError 异常。
如——
>>> print(name) # 变量 name 未定义
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'name' is not defined
>>>
2.4 IndexError
索引错误:即引用索引超出索引范围。当尝试访问列表、元组或字符串等序列中不存在的索引时引发。
如——
>>> s = '索引错误:即引用索引超出索引范围。当尝试 访问列表、元组或字符串等序列中不存在的索引时引发。'
>>> len(s)
45
>>> s[45]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: string index out of range
>>> lis = [2, 5, 8]
>>> lis[9]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: list index out of range
>>> nums = 6, 8, 9, 56, 13
>>> type(nums)
<class 'tuple'>
>>> nums[99]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: tuple index out of range
>>>
2.5 KeyError
键错误:当尝试访问字典中不存在的键时引发。
>>>
>>> {}[5]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 5
>>> d = {'d': 67, 'e': 99}
>>> d['a']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'a'
>>> d.get('a')
>>> d.get('a', 'None')
'None'
>>> d.get('a', None)
>>> d.get('a', 0)
0
>>> type(d.get('a'))
<class 'NoneType'>
>>> str(d.get('a'))
'None'
>>>
如命令行模式试炼可见,引用不存在的字典 key 会报错 KeyError 。其实,字典不但可以 dict[key] 引用,也可以 dict.get(key) 引用 key 的 value 。后者引用不存在的 key ,不会报错,默认返回 None 。如果设置第二个参数,字典中有 key ,返回 key 的值;字典中无 key ,则返回第二个参数对象。
引用字典 key ,dict.get(key) 比 dict[key] 更适宜些。
2.6 ZeroDivisionError
零为除数错误:当尝试除以零时引发。 (数学中,除不可以做除数,除数不可以是零)
>>>
>>> 5/0
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: division by zero
>>> d = 5 - 5
>>> 6/d
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: division by zero
>>>
2.7 IOError
I/OError ,输入输出错误。当尝试读取不存在的文件或无法访问文件时引发。
Python 3.11.1 (main, Dec 7 2022, 05:56:18) [Clang 14.0.6 (https://android.googlesource.com/toolchain/llvm-project 4c603efb0 on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> open('file4.py')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
FileNotFoundError: [Errno 2] No such file or directory: 'file4.py'
>>>
我好纳闷了,IOError 到我的环境,怎么就变成了 FileNotFoundError ?
- 原来——
FileNotFoundError 是 IOError 的一种情形。
- IOError 官方文档摘录
OS exceptions¶
The following exceptions are subclasses of OSError, they get raised depending on the system error code.
exception BlockingIOError
Raised when an operation would block on an object (e.g. socket) set for non-blocking operation. Corresponds to errno EAGAIN, EALREADY, EWOULDBLOCK and EINPROGRESS.
In addition to those of OSError, BlockingIOError can have one more attribute:
characters_written
An integer containing the number of characters written to the stream before it blocked. This attribute is available when using the buffered I/O classes from the io module. (摘录于 IOError 官方文档)
IOError 最新版本官方文档地址:https://docs.python.org/3.12/library/exceptions.html?highlight=ioerror#IOError
2.8 ImportError
当尝试导入不存在的模块或包时引发。例如——
~ $ python
Python 3.11.1 (main, Dec 7 2022, 05:56:18) [Clang 14.0.6 (https://android.googlesource.com/toolchain/llvm-project 4c603efb0 on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> import mymodule
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ModuleNotFoundError: No module named 'mymodule'
>>>
>>> import pandas
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ModuleNotFoundError: No module named 'pandas'
>>>
注: mymodule ,是没有定义的模块名称,也就是无中生有。pandas ,我的 Python 3.11.10 一直没有 pip install pandas 成功。
ImportError 在我的 Python 3.6.6 和 3.11.1 上,都是“ModuleNotFoundError” 了。
2.9 AttributeError
属性错误:当尝试访问对象不存在的属性时引发。例如下面故意张冠李戴地整 str 、list 、set 、dict 其没有的方法,无一例外,都引发了 AributeError 。
>>> >>> list.add() Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: type object 'list' has no attribute 'add'
>>> str.append()
Traceback (most recent call last):
File "<stdin>", line 1, in <module> AttributeError: type object 'str' has no attribute 'append'
>>> set.replace('d', '')
Traceback (most recent call last):
File "<stdin>", line 1, in <module> AttributeError: type object 'set' has no attribute 'replace'
>>> str.get('x', 0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: type object 'str' has no attribute 'get'
>>> dict.join([])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: type object 'dict' has no attribute 'join'
>>>
2.10 Keyboardinterrupt
键盘中断异常:当用户 Ctrl + C 中断程序执行时引发。
>>>
>>> input('\n请输入:')
请输入:Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyboardInterrupt
>>>
3、自定义异常类型
Python 除了上面的基本异常类型,还可以定义自己特有的异常类型。
3.1 自定义异常类型
我定义的异常类型,就是打印出 raise 时实例自定义异常类型时给定的字符串的异常提示。您可以根据需要自行设定,自定义异常类型功用。
class MyException(Exception):
def __init__(self, error_tip):
self.tip = error_tip
def __str__(self):
return self.tip
3.2 触发自定义异常类型
自定义异常类型,不同于 Python “官方”异常类型,是需要手动 raise 。设定触发条件,用 raise 关键字主动抛出自动义异常类型。
if __name__ == '__main__':
gray = '\033[2m' # 灰色。
blue = '\033[34;1m' # 亮蓝。
red = '\033[31m' # 红色。
nocolor = '\033[0m' # 还原 python 默认配色。
print(f"{gray}\n{'='*50}{' 这是个 Python 用户自定义异常示例 ':~^38}{nocolor}")
try:
name = input(f"\n{'您的姓名:':>7}").strip()
if name == '梦幻精灵': # 条件达成 raise 殿堂。
raise MyException(f"{red}\n\n{name:^46}\n{' 这是我的名字!':~^44}\n{nocolor}")
else:
print(f"\n\n{blue}{'':~^50}\n{f' {name}, 很高兴认识您!':^38}\n{'':~^50}\n{nocolor}")
except MyException as error:
print(error)
finally: # 关键字 finally 之后的代码块,总是要招待的。
print(f"{gray}\n{' 这是个 Python 用户自定义异常示例 ':~^38}\n{'='*50}\n{nocolor}")
- 触发自定义异常
再列举一个触发自定义异常类型的实例——
print(f"{gray}\n{'='*50}{' 这是个 Python 用户自定义异常示例 ':~^38}{nocolor}")
try:
num = input(f"\n{'请输入1~100的数字:':8>}")
if not num.isdigit():
raise MyException(f"{red}\n{' 请数字!':~^46}\n{nocolor}")
elif int(num) < 1 or int(num) > 100:
raise MyException(f"{red}\n{' 您输入的数字超出范围!':~^39}\n{nocolor}")
else:
print(f"{green}\n{f' {num},输入正确。':~^44}\n{nocolor}")
except MyException as error:
print(error)
finally:
print(f"{gray}\n{' 这是个 Python 用户自定义异常示例 ':~^38}\n{'='*50}\n{nocolor}")
- 代码运行效果截屏图片
其实,也可以把超出范围的异常捕获分开——
elif int(num) < 1 or int(num) > 100:
raise MyException(f"{red}\n{' 您输入的数字超出范围!':~^39}\n{nocolor}")
elif int(num) < 1:
raise MyException(f"{red}\n{' 您输入的数字超出下限1!':~^39}\n{nocolor}")
elif int(num) > 100:
raise MyException(f"{red}\n{' 您输入的数字超出上限100!':~^39}\n{nocolor}")
- 代码运行效果截屏图片
- 关于颜色控制字符串,请点击下边链接翻阅——
神奇的 \033 ,让打印出彩
( 2595 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122953893
点赞:3 踩 :0 收藏:7 打赏:0 评论:0
本篇博文笔记于 2022-02-15 23:01:54 首发,最晚于 2022-02-20 13:24:21 修改。
4、完整源码
#!/sur/bin/nve python
# coding: utf-8
class MyException(Exception):
def __init__(self, error_tip):
self.tip = error_tip
def __str__(self):
return self.tip
if __name__ == '__main__':
gray = '\033[2m' # 灰色。
blue = '\033[34;1m' # 亮蓝。
green = '\033[32m' # 绿色。
red = '\033[31m' # 红色。
nocolor = '\033[0m' # 还原 python 默认配色。
'''# raise one
print(f"{gray}\n{'='*50}{' 这是个 Python 用户自定义异常示例 ':~^38}{nocolor}")
try:
name = input(f"\n{'您的姓名:':>7}").strip()
if name == '梦幻精灵':
raise MyException(f"{red}\n\n{name:^46}\n{' 这是我的名字!':~^43}\n{nocolor}")
else:
print(f"\n\n{blue}{'':~^50}\n{f' {name}, 很高兴认识您!':^38}\n{'':~^50}\n{nocolor}")
except MyException as error:
print(error)
finally:
print(f"{gray}\n{' 这是个 Python 用户自定义异常示例 ':~^38}\n{'='*50}\n{nocolor}")'''
# raise two
print(f"{gray}\n{'='*50}{' 这是个 Python 用户自定义异常示例 ':~^38}{nocolor}")
try:
num = input(f"\n{'请输入1~100的数字:':8>}")
if not num.isdigit():
raise MyException(f"{red}\n{' 请输入数字!':~^44}\n{nocolor}")
elif int(num) < 1:
raise MyException(f"{red}\n{' 您输入的数字超出下限1!':~^39}\n{nocolor}")
elif int(num) > 100:
raise MyException(f"{red}\n{' 您输入的数字超出上限100!':~^39}\n{nocolor}")
else:
print(f"{green}\n{f' {num},输入正确。':~^44}\n{nocolor}")
except MyException as error:
print(error)
finally:
print(f"{gray}\n{' 这是个 Python 用户自定义异常示例 ':~^38}\n{'='*50}\n{nocolor}")
上一篇: 冒泡排序小练习(接收键盘录入(含输入字符串排错)字符串,拆分、转整、冒泡排序(递减)输出)
下一篇:
我的HOT博:
本次共计收集 214 篇博文笔记信息,总阅读量 34.60w,平均阅读量 1616。已生成 22 篇阅读量不小于 3000 的博文笔记索引链接。数据采集于 2023-05-28 05:47:47 完成,用时 4 分 31.83 秒。
- 让QQ群昵称色变的神奇代码
( 55081 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122566500
点赞:24 踩 :0 收藏:81 打赏:0 评论:17
本篇博文笔记于 2022-01-18 19:15:08 首发,最晚于 2022-01-20 07:56:47 修改。 - ChatGPT国内镜像站初体验:聊天、Python代码生成等
( 52916 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/129035387
点赞:125 踩 :0 收藏:788 打赏:0 评论:75
本篇博文笔记于 2023-02-14 23:46:33 首发,最晚于 2023-03-22 00:03:44 修改。 - pandas 数据类型之 DataFrame
( 8415 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124525814
点赞:6 踩 :0 收藏:26 打赏:0 评论:0
本篇博文笔记于 2022-05-01 13:20:17 首发,最晚于 2022-05-08 08:46:13 修改。 - 罗马数字转换器|罗马数字生成器
( 6486 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122592047
点赞:0 踩 :0 收藏:1 打赏:0 评论:0
本篇博文笔记于 2022-01-19 23:26:42 首发,最晚于 2022-01-21 18:37:46 修改。 - Python字符串居中显示
( 6340 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122163023
点赞:1 踩 :0 收藏:6 打赏:0 评论:1
本篇博文笔记于 2021-12-26 23:35:29 发布。 - 个人信息提取(字符串)
( 6001 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124244618
点赞:1 踩 :0 收藏:9 打赏:0 评论:0
本篇博文笔记于 2022-04-18 11:07:12 首发,最晚于 2022-04-20 13:17:54 修改。 - 斐波那契数列的递归实现和for实现
( 5330 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122355295
点赞:4 踩 :0 收藏:2 打赏:0 评论:8
本篇博文笔记于 2022-01-06 23:27:40 发布。 - 练习:字符串统计(坑:f‘string‘报错)
( 4912 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121723096
点赞:0 踩 :0 收藏:1 打赏:0 评论:0
本篇博文笔记于 2021-12-04 22:54:29 发布。 - 练习:尼姆游戏(聪明版/傻瓜式•人机对战)
( 4653 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121645399
点赞:14 踩 :0 收藏:42 打赏:0 评论:0
本篇博文笔记于 2021-11-30 23:43:17 发布。 - Python列表(list)反序(降序)的7种实现方式
( 4492 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/128271700
点赞:4 踩 :0 收藏:16 打赏:0 评论:8
本篇博文笔记于 2022-12-11 23:54:15 首发,最晚于 2023-03-20 18:13:55 修改。 - 回车符、换行符和回车换行符
( 4472 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123109488
点赞:0 踩 :0 收藏:2 打赏:0 评论:0
本篇博文笔记于 2022-02-24 13:10:02 首发,最晚于 2022-02-25 20:07:40 修改。 - python清屏
( 4463 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/120762101
点赞:0 踩 :0 收藏:5 打赏:0 评论:0
本篇博文笔记于 2021-10-14 13:47:21 发布。 - 密码强度检测器
( 3945 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121739694
点赞:1 踩 :0 收藏:4 打赏:0 评论:0
本篇博文笔记于 2021-12-06 09:08:25 首发,最晚于 2022-11-27 09:39:39 修改。 - 罗马数字转换器(用罗马数字构造元素的值取模实现)
( 3857 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122608526
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2022-01-20 19:38:12 首发,最晚于 2022-01-21 18:32:02 修改。 - 练习:生成100个随机正整数
( 3801 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122558220
点赞:1 踩 :0 收藏:4 打赏:0 评论:0
本篇博文笔记于 2022-01-18 13:31:36 首发,最晚于 2022-01-20 07:58:12 修改。 - 练习:班里有人和我同生日难吗?(概率probability、蒙特卡洛随机模拟法)
( 3623 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124424935
点赞:1 踩 :0 收藏:2 打赏:0 评论:0
本篇博文笔记于 2022-04-26 12:46:25 首发,最晚于 2022-04-27 21:22:07 修改。 - 我的 Python.color() (Python 色彩打印控制)
( 3516 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123194259
点赞:2 踩 :0 收藏:7 打赏:0 评论:0
本篇博文笔记于 2022-02-28 22:46:21 首发,最晚于 2022-03-03 10:30:03 修改。 - 练习:仿真模拟福彩双色球——中500w巨奖到底有多难?跑跑代码就晓得了。
( 3283 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/125415626
点赞:3 踩 :0 收藏:4 打赏:0 评论:3
本篇博文笔记于 2022-06-22 19:54:20 首发,最晚于 2022-06-23 22:41:33 修改。 - 聊天消息敏感词屏蔽系统(字符串替换 str.replace(str1, *) )
( 3155 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124539589
点赞:3 踩 :0 收藏:2 打赏:0 评论:3
本篇博文笔记于 2022-05-02 13:02:39 首发,最晚于 2022-05-21 06:10:42 修改。 - Linux 脚本文件第一行的特殊注释符(井号和感叹号组合)的含义
( 3111 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123087606
点赞:0 踩 :0 收藏:4 打赏:0 评论:3
本篇博文笔记于 2022-02-23 13:08:07 首发,最晚于 2022-04-04 23:52:38 修改。 - 练习:求列表(整数列表)平衡点
( 3052 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121737612
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2021-12-05 23:28:10 发布。 - random.sample()将在python 3.9x后续版本中被弃用
( 3044 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/120657230
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2021-10-08 18:35:09 发布。
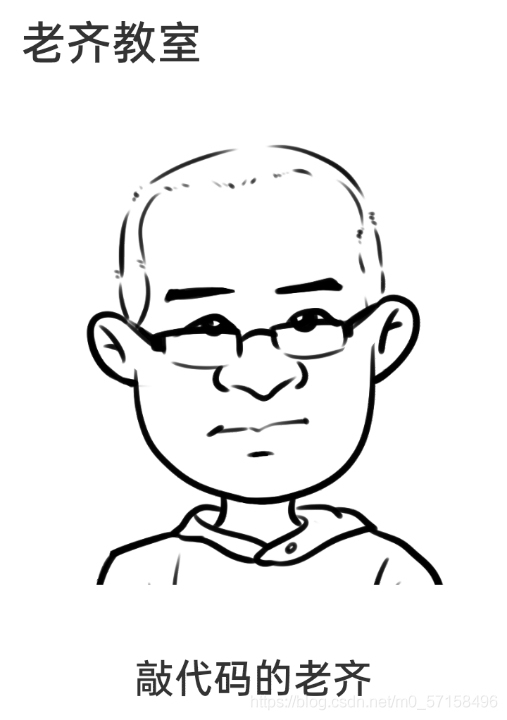
精品文章:
- 好文力荐:齐伟书稿 《python 完全自学教程》 Free连载(已完稿并集结成书,还有PDF版本百度网盘永久分享,点击跳转免费🆓下载。)
- OPP三大特性:封装中的property
- 通过内置对象理解python'
- 正则表达式
- python中“*”的作用
- Python 完全自学手册
- 海象运算符
- Python中的 `!=`与`is not`不同
- 学习编程的正确方法
来源:老齐教室
◆ Python 入门指南【Python 3.6.3】
好文力荐:
- 全栈领域优质创作者——[寒佬](还是国内某高校学生)博文“非技术文—关于英语和如何正确的提问”,“英语”和“会提问”是编程学习的两大利器。
- 【8大编程语言的适用领域】先别着急选语言学编程,先看它们能干嘛
- 靠谱程序员的好习惯
- 大佬帅地的优质好文“函数功能、结束条件、函数等价式”三大要素让您认清递归
CSDN实用技巧博文: