springboot生成图片验证码,使用开源kaptcha,三步搞定
引言:
1. 使用图片验证码或者一些其他人机校验方式,能够分担部分压力,及增加部分反爬高度。
1. 本文使用谷歌开源kaptcha进行生成图片验证码,可以自定义图片验证码长度,颜色样式,干扰度,生成中文或英文或数字图片验证码。
一、Pom导入依赖
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
二、自定义图片验证码相关配置
@Configuration
public class CaptchaConfig {
@Bean
public Producer captcha() {
Properties properties = new Properties();
properties.setProperty("kaptcha.image.width", "150");
properties.setProperty("kaptcha.image.height", "50");
properties.setProperty("kaptcha.textproducer.char.string", "0123456789");
properties.setProperty("kaptcha.textproducer.char.length", "2");
properties.setProperty("kaptcha.textproducer.font.color", "red");
Config config = new Config(properties);
DefaultKaptcha defaultKaptcha = new DefaultKaptcha();
defaultKaptcha.setConfig(config);
return defaultKaptcha;
}
}
三、编写接口生成图片验证码
@RestController
@CrossOrigin
@RequestMapping("/user")
public class UserController {
@Autowired
private Producer captchaProducer;
@GetMapping("/captcha")
public void getCaptcha(HttpServletRequest request, HttpServletResponse response) {
try {
response.setContentType("image/jpeg");
String capText = captchaProducer.createText();
request.getSession().setAttribute("captcha", capText);
BufferedImage image = captchaProducer.createImage(capText);
ServletOutputStream out = response.getOutputStream();
ImageIO.write(image, "jpg", out);
try {
out.flush();
} finally {
out.close();
}
} catch (IOException e) {
log.info("登录:获取图片验证码异常!");
}
}
}
四、测试接口
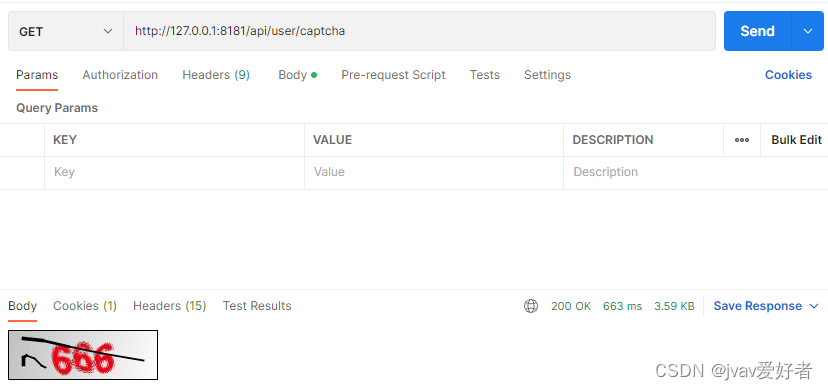
五、验证码比对
public boolean isSuccessCaptcha(HttpServletRequest request) {
String captcha = request.getParameter("captcha");
Object sessionCaptcha = request.getSession().getAttribute("userCaptcha");
if (sessionCaptcha == null) {
return false;
}
return sessionCaptcha.toString().equals(captcha);
}