目录
1 新建spring_mvc_demo com.atguigu
5 .在resources下面创建配置文件springmvc.xml
五 . @RequestMapping注解的method属性
六 .@RequestMapping注解的params属性(了解)
七 @RequestMapping注解的headers属性(了解)
@RequestMapping注解
一.准备工作
1 新建spring_mvc_demo com.atguigu
2. 导入依赖
<packaging>war</packaging> <dependencies> <!-- SpringMVC --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.3.1</version> </dependency> <!-- 日志 --> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.2.3</version> </dependency> <!-- ServletAPI --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> <!-- Spring5和Thymeleaf整合包 --> <dependency> <groupId>org.thymeleaf</groupId> <artifactId>thymeleaf-spring5</artifactId> <version>3.0.12.RELEASE</version> </dependency> </dependencies>
3 .添加web模板
第一种方式
在spring_mvc_demo后面添加\src\main\webapp\
整体D:\review_ssm\workspace\SSM\spring_mvc_demo\src\main\webapp\WEB-INF\web.xml
第二种方式:
4 . 在web.xml里面进行进行配置
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--
配置SpringMVC的前端控制器DispatcherServlet 配置SpringMVC的前端控制器,对浏览器发送的请求统一进行处理
SpringMVC的配置文件默认的位置和名称:
位置:WEB-INF下
名称:<servlet-name>-servlet.xml,当前配置下的配置文件名为SpringMVC-servlet.xml
url-pattern中/和/*的区别:
/:匹配浏览器向服务器发送的所有请求(不包括.jsp)
/*:匹配浏览器向服务器发送的所有请求(包括.jsp)
-->
<servlet>
<servlet-name>SpringMVC</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<!-- 通过初始化参数指定SpringMVC配置文件的位置和名称 -->
<init-param>
<!-- contextConfigLocation为固定值 -->
<param-name>contextConfigLocation</param-name>
<!-- 使用classpath:表示从类路径查找配置文件,例如maven工程中的src/main/resources -->
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<!--
作为框架的核心组件,在启动过程中有大量的初始化操作要做
而这些操作放在第一次请求时才执行会严重影响访问速度
因此需要通过此标签将启动控制DispatcherServlet的初始化时间提前到服务器启动时
将DispatcherServlet的初始化时间提前到服务器启动时
-->
<load-on-startup>1</load-on-startup>
</servlet>
<!--
设置springMVC的核心控制器所能处理的请求的请求路径
/所匹配的请求可以是/login或.html或.js或.css方式的请求路径
但是/不能匹配.jsp请求路径的请求
-->
<servlet-mapping>
<servlet-name>SpringMVC</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
5 .在resources下面创建配置文件springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!--扫描控制层组件-->
<context:component-scan base-package="com.atguigu.controller"></context:component-scan>
<!-- 配置Thymeleaf视图解析器 -->
<bean id="viewResolver" class="org.thymeleaf.spring5.view.ThymeleafViewResolver">
<property name="order" value="1"/>
<property name="characterEncoding" value="UTF-8"/>
<property name="templateEngine">
<bean class="org.thymeleaf.spring5.SpringTemplateEngine">
<property name="templateResolver">
<bean class="org.thymeleaf.spring5.templateresolver.SpringResourceTemplateResolver">
<!-- 视图前缀 -->
<property name="prefix" value="/WEB-INF/templates/"/>
<!-- 视图后缀 -->
<property name="suffix" value=".html"/>
<property name="templateMode" value="HTML5"/>
<property name="characterEncoding" value="UTF-8" />
</bean>
</property>
</bean>
</property>
</bean>
</beans>
6 创建控制层
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class TestRequestMappingController {
}
7 . 创建入口控制层 用来访问首页
@Controller
public class ProtalController {
@RequestMapping("/") //访问首页
public String protal(){
return "index";
}
}
8 . 创建首页
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
</body>
</html>
9 . 部暑工程
10 .启动tomcat
11 .测试结果
二 .@RequestMapping注解的功能
三 .@RequestMapping注解的位置
解释:@Target({ElementType.TYPE, ElementType.METHOD})
@Target:当前注解所能标识的位置 我们可以把他标记在一个类上TYPE 也可以把它标记在一个方法上METHOD
现在我们演示把它标记在一个类
第一步: 在TestRequestMappingController类写hello()方法
/*
* 1、@RequestMapping注解标识的位置
@RequestMapping标识一个类:设置映射请求的请求路径的初始信息
@RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* */
@Controller
@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping("/hello") //设置映射的具体信息
public String hello() {
return "success";
}
}
第二步:写success.html首页
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>success.html</h1>
</body>
</html>
第三步:写index.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
</body>
</html>
第四步:测试
报404的原因是
因为在类上加上了@RequestMapping("/test")之后 我们当前访问的请求路径应该是test下面的hello 才能匹配到控制器方法hello()
解决办法如下
怎么用
2个控制层都可以对hello路径进行处理 这个时候就会报错 所以我们要在类上加上@RequestMapping("/test") 这时我们的控制器方法所匹配的请求的请求路径为/test/hello
TestRequestMappingController
ProtalController
启动tomcat
报错:
Caused by: java.lang.IllegalStateException: Ambiguous mapping. Cannot map 'testRequestMappingController' method
com.atguigu.controller.TestRequestMappingController#hello()
to { [/hello]}: There is already 'protalController' bean method模糊不清的一个映射 不能去映射'testRequestMappingController'里面的hello()方法
因为 'protalController里面已经有了一个方法进行映射了
总结
* 1、@RequestMapping注解标识的位置 * @RequestMapping标识一个类:设置映射请求的请求路径的初始信息 * @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
四 . @RequestMapping注解的value属性
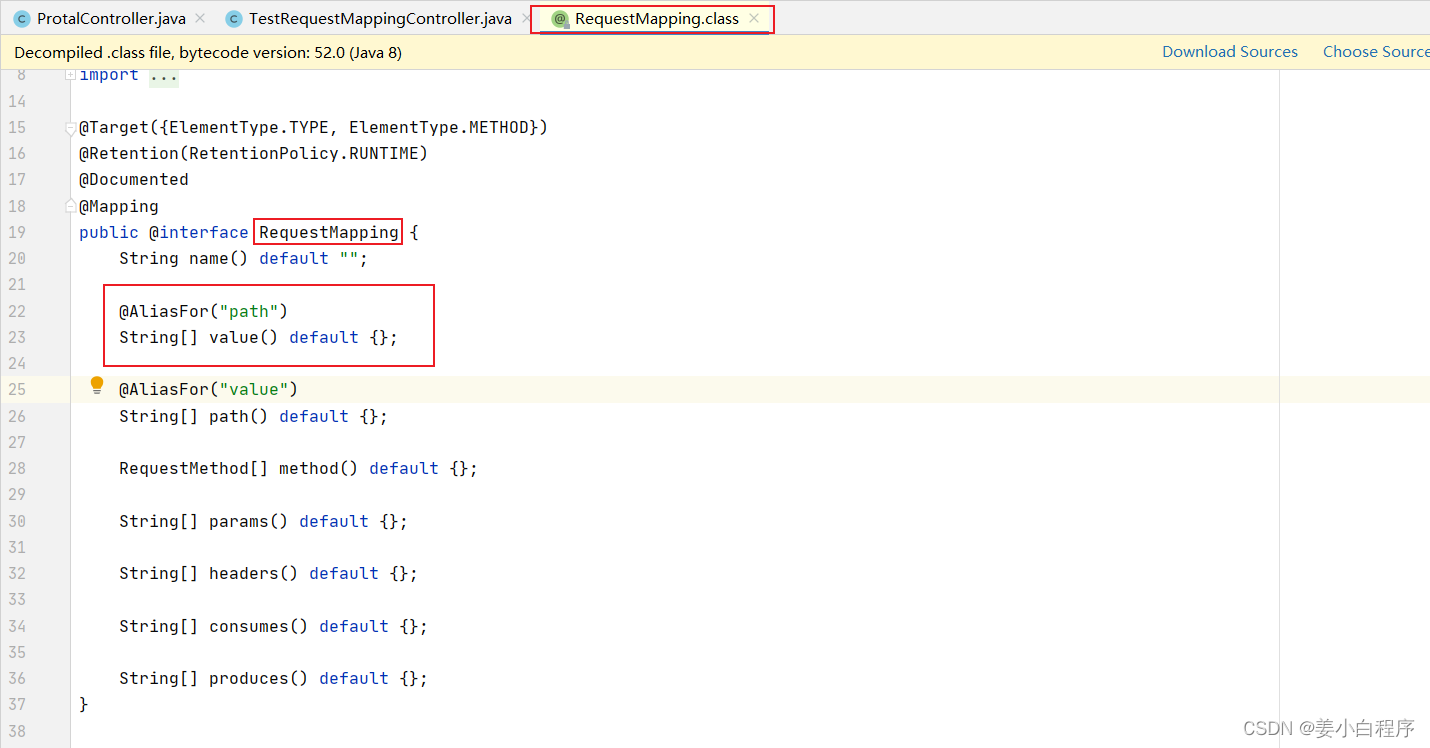
TestRequestMappingController
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping({"/hello","/abc"}) //设置映射的具体信息
public String hello() {
return "success";
}
}
index.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
</body>
</html>
测试
总结:
@RequestMapping注解value属性 * 作用:通过请求的请求路径匹配请求 * value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值 * 则当前请求就会被注解所标识的方法进行处理
五 . @RequestMapping注解的method属性
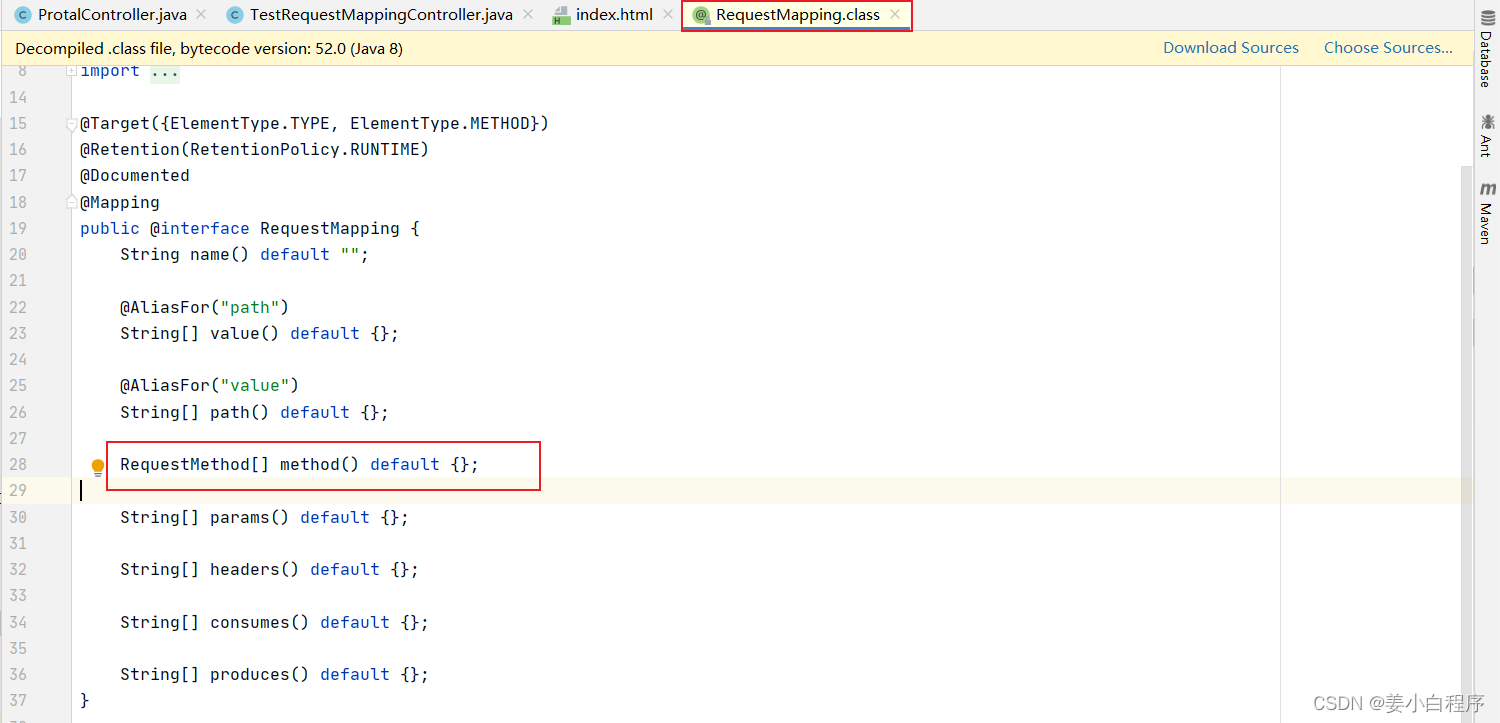
/** * The HTTP request methods to map to, narrowing the primary mapping: * GET, POST, HEAD, OPTIONS, PUT, PATCH, DELETE, TRACE. * <p><b>Supported at the type level as well as at the method level!</b> * When used at the type level, all method-level mappings inherit this * HTTP method restriction. */ RequestMethod[] method() default {};
Crtl+左健 打开RequestMethod[] 枚举类型
表单提交发出的是post或是axios里面把请求方式设置成post 其他的都是get,比如超链接来发送请求 地址栏直接输入地址来发送请求 都是get
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
method = RequestMethod.GET
)
public String hello() {
return "success";
}
}
测试
现在我们把请求方式设置成post
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
method = RequestMethod.POST
)
public String hello() {
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
</body>
</html>
[点击并拖拽以移动]
注意:表单在没有设置post请求时 默认的还是get请求 也就是说上面三种都是get请求
而现在我们在控制层里面的@RequestMapping注解里面把请求方式设置层了post请求,
若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配,此时页面报错:405 - Request method 'xxx' not supported
测试
现在我们把表单的请求方式改成post 重新部暑
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
</body>
</html>
现在我们让他匹配get和post
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET}
)
public String hello() {
return "success";
}
}
重新部暑
派生注解:
在@RequestMapping的基础上,结合请求方式的一些派生注解: * @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
总结:
@RequestMapping注解的method属性 * 作用:通过请求的请求方式匹配请求 * method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式 * 则当前请求就会被注解所标识的方法进行处理 * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配 * 此时页面报错:405 - Request method 'xxx' not supported * 在@RequestMapping的基础上,结合请求方式的一些派生注解: * @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
六 .@RequestMapping注解的params属性(了解)
/** * The parameters of the mapped request, narrowing the primary mapping. * <p>Same format for any environment: a sequence of "myParam=myValue" style * expressions, with a request only mapped if each such parameter is found * to have the given value. Expressions can be negated by using the "!=" operator, * as in "myParam!=myValue". "myParam" style expressions are also supported, * with such parameters having to be present in the request (allowed to have * any value). Finally, "!myParam" style expressions indicate that the * specified parameter is <i>not</i> supposed to be present in the request. * <p><b>Supported at the type level as well as at the method level!</b> * When used at the type level, all method-level mappings inherit this * parameter restriction. */ String[] params() default {};
第一种写法
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
params = {"username"}
)
public String hello() {
return "success";
}
}
报400 当前参数的条件与真实的请求参数不匹配
第一种方式
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
修改 index.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
</body>
</html>
第二种方式
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
</body>
</html>
第二种写法
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
params = {"username","!password"}
)
public String hello() {
return "success";
}
}
现在我们把它加个&password
第三种方式
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
params = {"username","!password","age=20"}
)
public String hello() {
return "success";
}
}
现在我们加上&age=20
第四种方式
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
params = {"username","!password","age=20","gender!=女"}
)
public String hello() {
return "success";
}
}
现在加上&gender=女
总结:
@RequestMapping注解的params属性 * 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置 * params可以使用四种表达式: * "param":表示当前所匹配请求的请求参数中必须携带param参数 * "!param":表示当前所匹配请求的请求参数中一定不能携带param参数 * "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value * "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配 * 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
七 @RequestMapping注解的headers属性(了解)
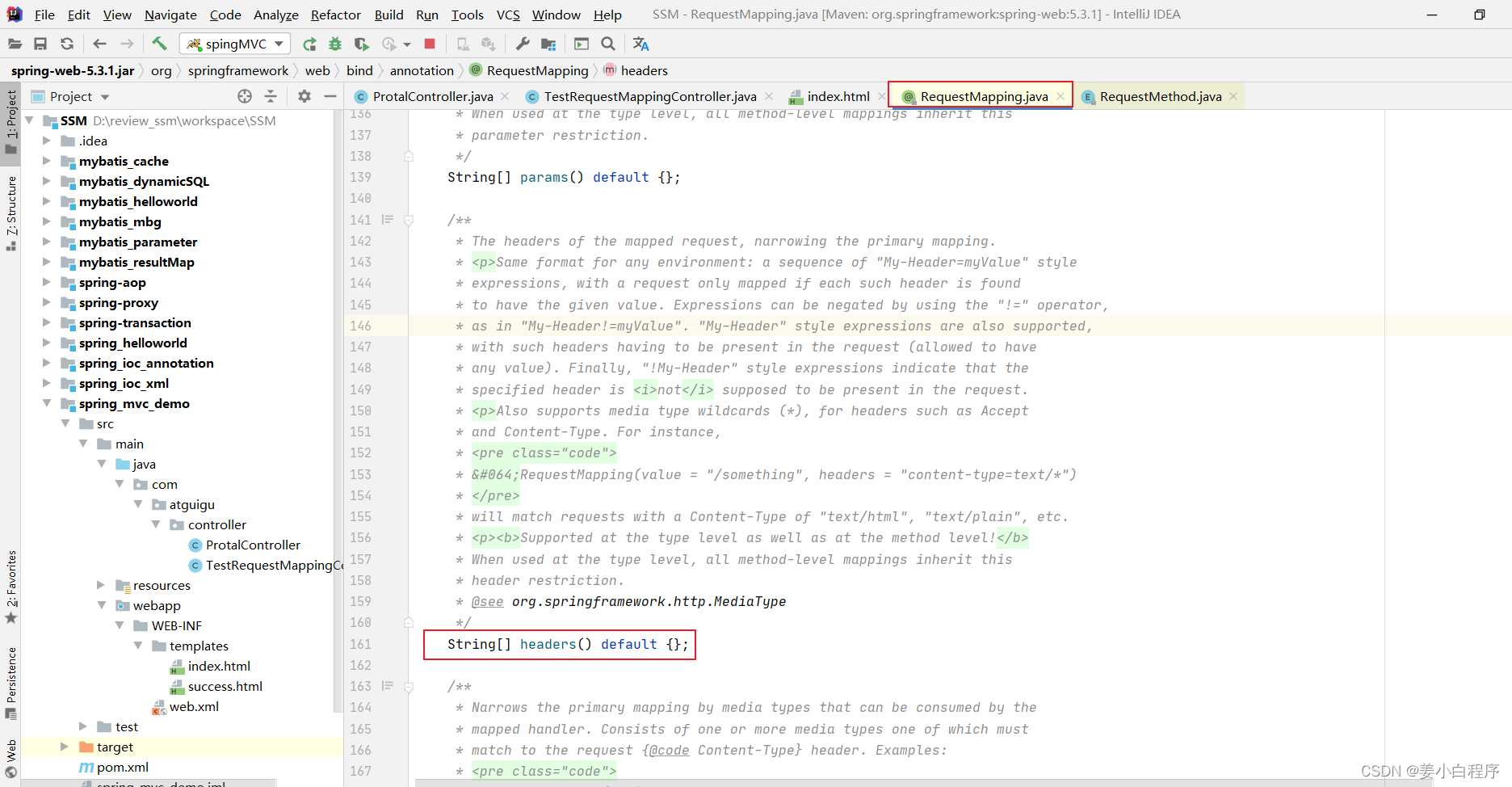
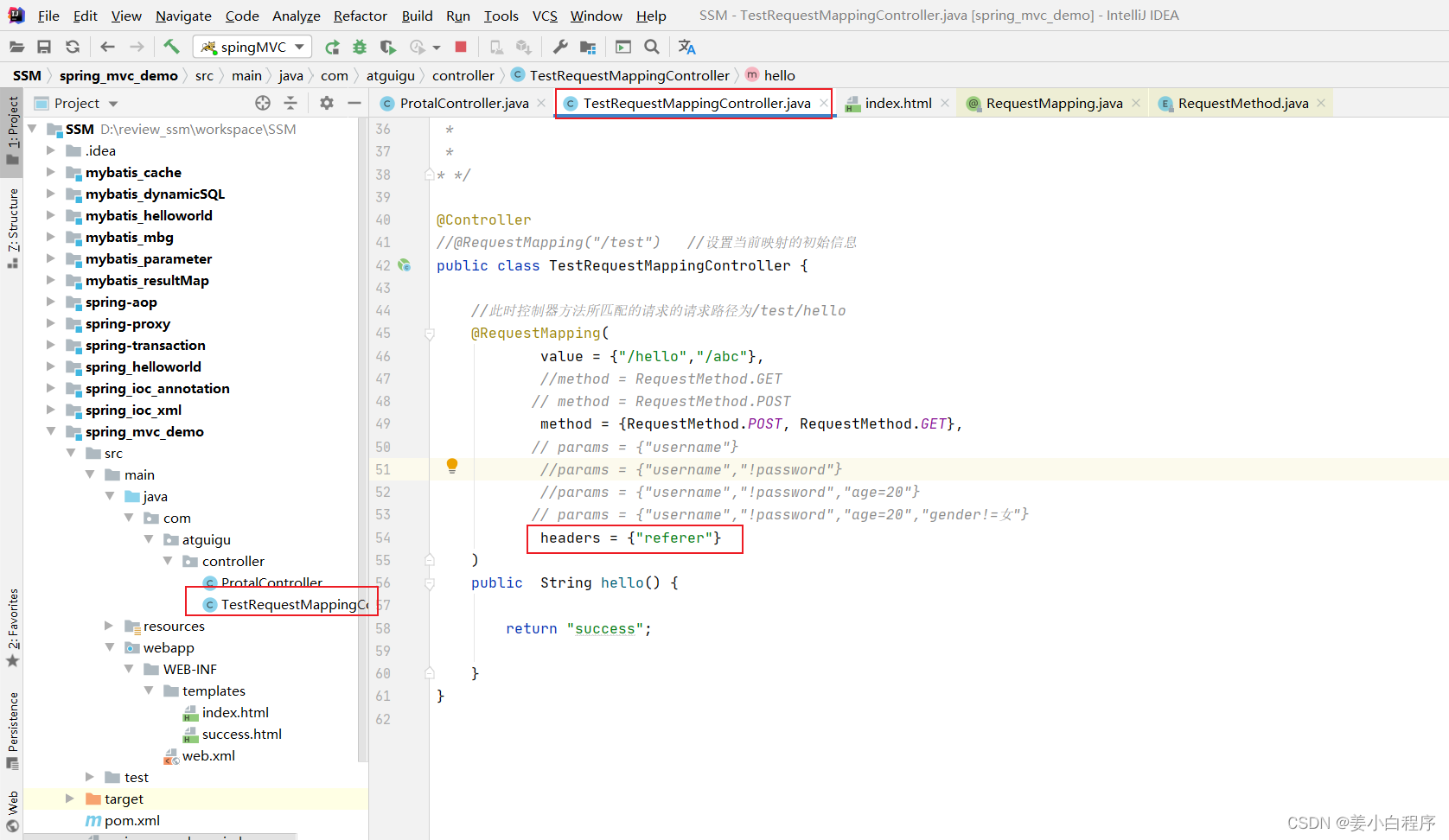
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
}
打开F12
如果这个时候 我们复制地址值 新建一个标签复制进去后 会报错
有首页的情况
总结:
@RequestMapping注解的headers属性 * 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置 * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配 * 此时页面报错:404
八 SpringMVC支持ant风格的路径
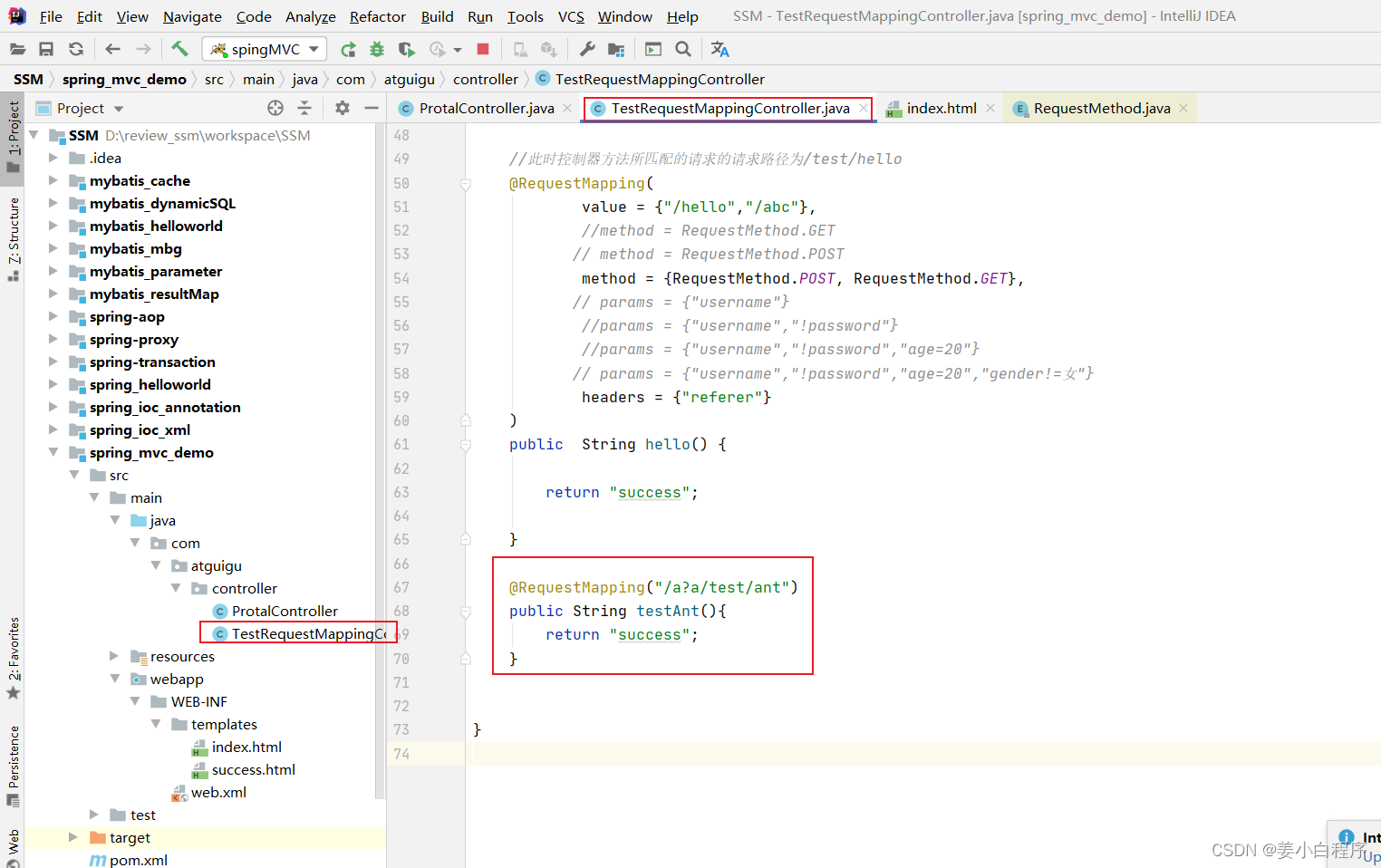
1 . ?:表示任意的单个字符
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
6、SpringMVC支持ant风格的路径
* 在@RequestMapping注解的value属性值中设置一些特殊字符
* ?:任意的单个字符(不包括?)
* *:任意个数的任意字符(不包括?和/)
* **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
@RequestMapping("/a?a/test/ant")
public String testAnt(){
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/aaa/test/ant}">测试@RequestMapping注解支持ant风格的路径</a><br>
</body>
</html>
但是不能写问号? 或是多个字符 如下所示 则会报错
2 . *:表示任意的0个或多个字符
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
6、SpringMVC支持ant风格的路径
* 在@RequestMapping注解的value属性值中设置一些特殊字符
* ?:任意的单个字符(不包括?)
* *:任意个数的任意字符(不包括?和/)
* **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
@RequestMapping("/a*a/test/ant")
public String testAnt(){
return "success";
}
}
但是不能写?和/
3 . **:表示任意层数的任意目录
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
6、SpringMVC支持ant风格的路径
* 在@RequestMapping注解的value属性值中设置一些特殊字符
* ?:任意的单个字符(不包括?)
* *:任意个数的任意字符(不包括?和/)
* **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
*
*
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
/* @RequestMapping("/a?a/test/ant")
public String testAnt(){
return "success";
}*/
/* @RequestMapping("/a*a/test/ant")
public String testAnt(){
return "success";
}*/
@RequestMapping("/**/test/ant")
public String testAnt(){
return "success";
}
}
总结:
SpringMVC支持ant风格的路径 * 在@RequestMapping注解的value属性值中设置一些特殊字符 * ?:任意的单个字符(不包括?) * *:任意个数的任意字符(不包括?和/) * **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
九 SpringMVC支持路径中的占位符(重点)
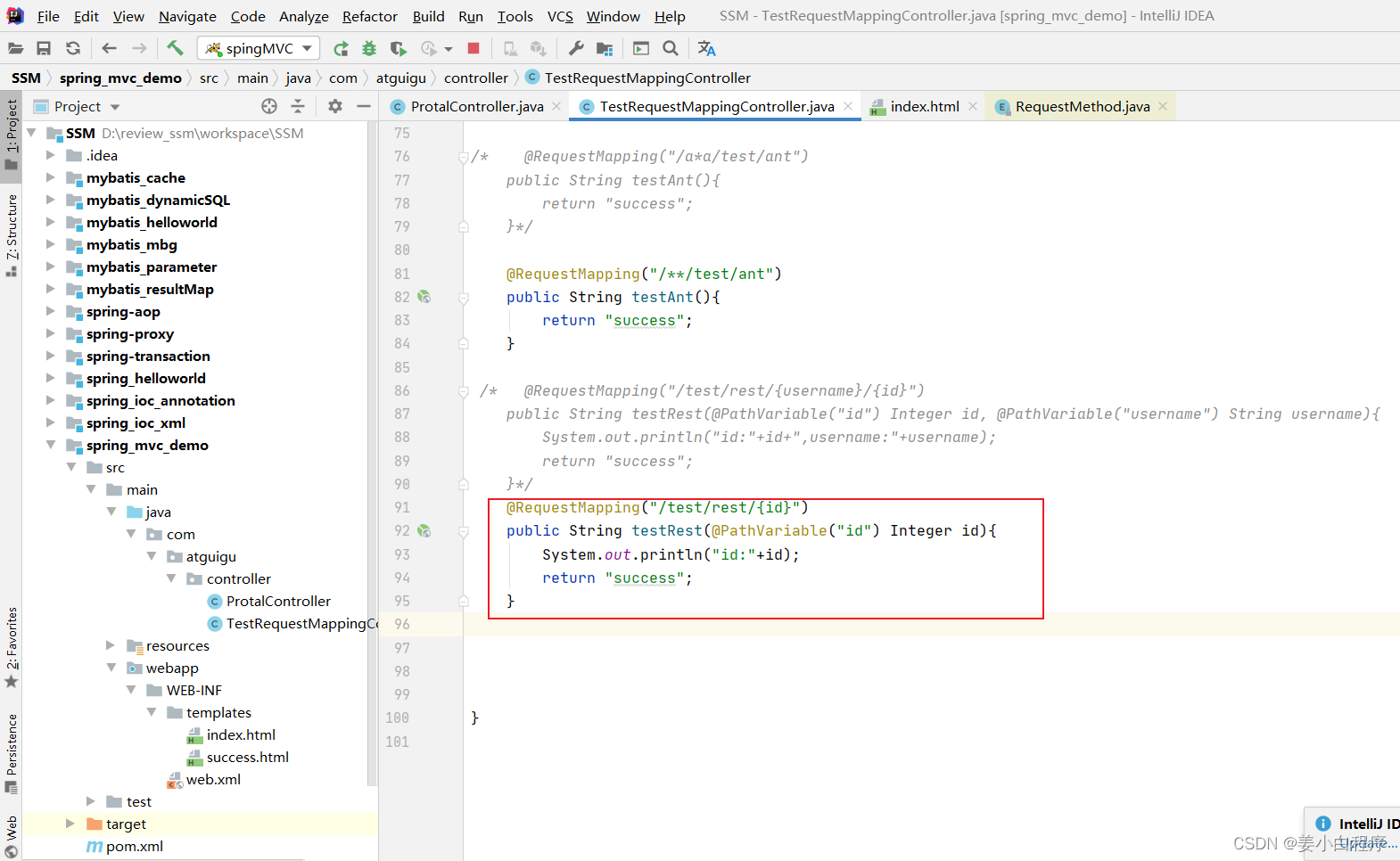
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
6、SpringMVC支持ant风格的路径
* 在@RequestMapping注解的value属性值中设置一些特殊字符
* ?:任意的单个字符(不包括?)
* *:任意个数的任意字符(不包括?和/)
* **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
*7、@RequestMapping注解使用路径中的占位符
* 传统:/deleteUser?id=1
* rest:/user/delete/1
* 需要在@RequestMapping注解的value属性中所设置的路径中,使用{xxx}的方式表示路径中的数据
* 在通过@PathVariable注解,将占位符所标识的值和控制器方法的形参进行绑定
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
/* @RequestMapping("/a?a/test/ant")
public String testAnt(){
return "success";
}*/
/* @RequestMapping("/a*a/test/ant")
public String testAnt(){
return "success";
}*/
@RequestMapping("/**/test/ant")
public String testAnt(){
return "success";
}
/* @RequestMapping("/test/rest/{username}/{id}")
public String testRest(@PathVariable("id") Integer id, @PathVariable("username") String username){
System.out.println("id:"+id+",username:"+username);
return "success";
}*/
@RequestMapping("/test/rest/{id}")
public String testRest(@PathVariable("id") Integer id){
System.out.println("id:"+id);
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/aaa/test/ant}">测试@RequestMapping注解支持ant风格的路径</a><br>
<a th:href="@{/test/rest/1}">测试@RequestMapping注解的value属性中的占位符</a><br>
</body>
</html>
现在我们加上username
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
* 1、@RequestMapping注解标识的位置
* @RequestMapping标识一个类:设置映射请求的请求路径的初始信息
* @RequestMapping标识一个方法:设置映射请求请求路径的具体信息
* 2、@RequestMapping注解value属性
* 作用:通过请求的请求路径匹配请求
* value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值
* 则当前请求就会被注解所标识的方法进行处理
* 3、@RequestMapping注解的method属性
* 作用:通过请求的请求方式匹配请求
* method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式
* 则当前请求就会被注解所标识的方法进行处理
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配
* 此时页面报错:405 - Request method 'xxx' not supported
* 在@RequestMapping的基础上,结合请求方式的一些派生注解:
* @GetMapping,@PostMapping,@DeleteMapping,@PutMapping
* 4、@RequestMapping注解的params属性
* 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置
* params可以使用四种表达式:
* "param":表示当前所匹配请求的请求参数中必须携带param参数
* "!param":表示当前所匹配请求的请求参数中一定不能携带param参数
* "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value
* "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配
* 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters:
*5、@RequestMapping注解的headers属性
* 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置
* 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配
* 此时页面报错:404
6、SpringMVC支持ant风格的路径
* 在@RequestMapping注解的value属性值中设置一些特殊字符
* ?:任意的单个字符(不包括?)
* *:任意个数的任意字符(不包括?和/)
* **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符
*7、@RequestMapping注解使用路径中的占位符
* 传统:/deleteUser?id=1
* rest:/user/delete/1
* 需要在@RequestMapping注解的value属性中所设置的路径中,使用{xxx}的方式表示路径中的数据
* 在通过@PathVariable注解,将占位符所标识的值和控制器方法的形参进行绑定
* */
@Controller
//@RequestMapping("/test") //设置当前映射的初始信息
public class TestRequestMappingController {
//此时控制器方法所匹配的请求的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
//method = RequestMethod.GET
// method = RequestMethod.POST
method = {RequestMethod.POST, RequestMethod.GET},
// params = {"username"}
//params = {"username","!password"}
//params = {"username","!password","age=20"}
// params = {"username","!password","age=20","gender!=女"}
headers = {"referer"}
)
public String hello() {
return "success";
}
/* @RequestMapping("/a?a/test/ant")
public String testAnt(){
return "success";
}*/
/* @RequestMapping("/a*a/test/ant")
public String testAnt(){
return "success";
}*/
@RequestMapping("/**/test/ant")
public String testAnt(){
return "success";
}
/* @RequestMapping("/test/rest/{id}")
public String testRest(@PathVariable("id") Integer id){
System.out.println("id:"+id);
return "success";
}*/
@RequestMapping("/test/rest/{username}/{id}")
public String testRest(@PathVariable("id") Integer id, @PathVariable("username") String username){
System.out.println("id:"+id+",username:"+username);
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/abc}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/aaa/test/ant}">测试@RequestMapping注解支持ant风格的路径</a><br>
<!--<a th:href="@{/test/rest/1}">测试@RequestMapping注解的value属性中的占位符</a><br>-->
<a th:href="@{/test/rest/admin/1}">测试@RequestMapping注解的value属性中的占位符</a><br>
</body>
</html>
总结:
@RequestMapping注解使用路径中的占位符 * 传统:/deleteUser?id=1 * rest:/user/delete/1 * 需要在@RequestMapping注解的value属性中所设置的路径中,使用{xxx}的方式表示路径中的数据 * 在通过@PathVariable注解,将占位符所标识的值和控制器方法的形参进行绑定
全部总结:
/* * 1、@RequestMapping注解标识的位置 * @RequestMapping标识一个类:设置映射请求的请求路径的初始信息 * @RequestMapping标识一个方法:设置映射请求请求路径的具体信息 * 2、@RequestMapping注解value属性 * 作用:通过请求的请求路径匹配请求 * value属性是数组类型,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值 * 则当前请求就会被注解所标识的方法进行处理 * 3、@RequestMapping注解的method属性 * 作用:通过请求的请求方式匹配请求 * method属性是RequestMethod类型的数组,即当前浏览器所发送请求的请求方式匹配method属性中的任何一中请求方式 * 则当前请求就会被注解所标识的方法进行处理 * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求方式不匹配 * 此时页面报错:405 - Request method 'xxx' not supported * 在@RequestMapping的基础上,结合请求方式的一些派生注解: * @GetMapping,@PostMapping,@DeleteMapping,@PutMapping * 4、@RequestMapping注解的params属性 * 作用:通过请求的请求参数匹配请求,即浏览器发送的请求的请求参数必须满足params属性的设置 * params可以使用四种表达式: * "param":表示当前所匹配请求的请求参数中必须携带param参数 * "!param":表示当前所匹配请求的请求参数中一定不能携带param参数 * "param=value":表示当前所匹配请求的请求参数中必须携带param参数且值必须为value * "param!=value":表示当前所匹配请求的请求参数中可以不携带param,若携带值一定不能是value * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求参数不匹配 * 此时页面报错:400 - Parameter conditions "username" not met for actual request parameters: *5、@RequestMapping注解的headers属性 * 作用:通过请求的请求头信息匹配请求,即浏览器发送的请求的请求头信息必须满足headers属性的设置 * 若浏览器所发送的请求的请求路径和@RequestMapping注解value属性匹配,但是请求头信息不匹配 * 此时页面报错:404 6、SpringMVC支持ant风格的路径 * 在@RequestMapping注解的value属性值中设置一些特殊字符 * ?:任意的单个字符(不包括?) * *:任意个数的任意字符(不包括?和/) * **:任意层数的任意目录,注意使用方式只能**写在双斜线中,前后不能有任何的其他字符 *7、@RequestMapping注解使用路径中的占位符 * 传统:/deleteUser?id=1 * rest:/user/delete/1 * 需要在@RequestMapping注解的value属性中所设置的路径中,使用{xxx}的方式表示路径中的数据 * 在通过@PathVariable注解,将占位符所标识的值和控制器方法的形参进行绑定 * */