Vue路由
18. Vue-路由基础
安装 cnpm install --save vue-router
官方文档:https://router.vuejs.org/zh/
src/main.js中
(1)引入VueRouter:import VueRouter from "vue-router"
(2)使用VueRouter:Vue.use(VueRouter);
(3)创建路由规则
Copyconst router = newVueRouter({
routes: [
{
path: '/hello',
component: Hello
}
]
});
(4)注入router
CopynewVue({
el: '#app',
router, // 添加路由components: { App },
template: '<App/>'
})
(5)App.vue中显示路由组件:<router-view />,访问http://localhost:8080/#/hello显示相应视图
也可以使用<router-view></router-view>
示例:
新建src/components/hello.vue
Copy<template><divclass="hello">
Hello
</div></template><script>exportdefault{
name: 'chachao',
data(){
return {
}
}
}
</script><stylelang='css'></style>
修改src/main.js
CopyimportVuefrom'vue'importAppfrom'./App'importVueRouterfrom"vue-router"// 引入VueRouterimportHellofrom"./components/hello"// 引入相关组件Vue.config.productionTip = falseVue.use(VueRouter);
const router = newVueRouter({
routes: [
{
path: '/hello',
component: Hello
}
]
});
newVue({
el: '#app',
router, // 添加路由components: { App },
template: '<App/>'
})
修改src/App.vue
Copy<template><divid="app"><p>哈哈</p><router-view /></div></template><script>import $ from"jquery"exportdefault {
name: 'App',
data(){
return {
}
}
}
</script><stylelang="css"></style>
19. Vue-路由跳转
在组件template中使用 <router-link to="/hello">Hello</router-link>添加跳转连接
示例:
新建src/components/hello.vue
Copy<template><divclass="hello">
Hello组件
</div></template><script>exportdefault{
name: 'hello',
data(){
return {
}
}
}
</script><stylelang='css'></style>
新建新建src/components/hi.vue
Copy<template><divclass="hi">
Hi组件
</div></template><script>exportdefault{
name: 'hi',
data(){
return {
}
}
}
</script><stylelang='css'></style>
src/main.js中配置路径
CopyimportVuefrom'vue'importAppfrom'./App'importVueRouterfrom"vue-router"// 引入VueRouterimportHellofrom"./components/hello"// 引入相关组件importHifrom"./components/hi"// 引入相关组件Vue.config.productionTip = falseVue.use(VueRouter);
const router = newVueRouter({
routes: [
{
path: '/hello',
component: Hello
},
{
path: '/hi',
component: Hi
},
]
});
newVue({
el: '#app',
router, // 添加路由components: { App },
template: '<App/>'
})
src/App.vue中使用
Copy<template><divid="app"><divclass="nav"><ul><li><router-linkto="/hello">Hello</router-link></li><li><router-linkto="/hi">Hi</router-link></li></ul></div><router-view/></div></template><script>exportdefault {
name: 'App',
data(){
return {
}
}
}
</script><stylelang="css"></style>
路由模块化处理
将src/main.js中路由相关的配置移动到src/router/index.js中
(1)src下新建router文件夹,新建src/router/index.js:
CopyimportVuefrom'vue'importVueRouterfrom"vue-router"importHellofrom"../components/hello"//改为两个..importHifrom"../components/hi"//改为两个..Vue.use(VueRouter);
exportdefaultnewVueRouter({ // 改为export default以使其可外部访问routes: [
{
path: '/hello',
component: Hello
},
{
path: '/hi',
component: Hi
},
]
});
(2)src/main.js改为
CopyimportVuefrom'vue'importAppfrom'./App'import router from'./router'// 自动导入该目录中的index.jsVue.config.productionTip = falsenewVue({
el: '#app',
router, // 添加路由components: { App },
template: '<App/>'
})
(3)src/components/hello.vue,src/components/hi.vue,src/App.vue配置同上
也可以新建src/components/nav.vue来引入hello和hi组件
Copy<template><divclass="nav"><ul><li><router-linkto="/hello">Hello</router-link></li><li><router-linkto="/hi">Hi</router-link></li></ul></div></template><script>exportdefault{
name: 'nav2',
data(){
return {
}
},
}
</script><stylelang='css'>.nav{
width: 100%;
height: 50px;
line-height: 50px;
background: #fff;
}
.navul {
clear: both;
overflow: hidden;
}
.navulli {
float: left;
margin-left: 30px;
list-style: none;
}
</style>
修改App.vue导入Nav组件
Copy<template><divid="app"><Nav/><router-view/></div></template><script>importNavfrom"./components/nav"exportdefault {
name: 'App',
data(){
return {
}
},
components: {
Nav
}
}
</script><style>body{
background-color: #f1f1f1;
}
*{
margin: 0;
padding: 0;
}
</style>
比起写死的 会好一些,理由如下:
无论是 HTML5 history 模式还是 hash 模式,它的表现行为一致,所以,当你要切换路由模式,或者在 IE9 降级使用 hash 模式,无须作任何变动。
在 HTML5 history 模式下,router-link 会守卫点击事件,让浏览器不再重新加载页面。
当你在 HTML5 history 模式下使用 base 选项之后,所有的 to 属性都不需要写 (基路径) 了。
router-link可以指定渲染的标签,默认为a标签
Copy<router-linktag="li"to="hello">Hello</router-link>
配置默认重定向到的页面
routes配置中添加
Copy{ path: '/', redirect: '/hello' },
src/router/index.js,完整代码:
CopyimportVuefrom'vue'importVueRouterfrom"vue-router"importHellofrom"../components/hello"//改为两个..importHifrom"../components/hi"//改为两个..Vue.use(VueRouter);
exportdefaultnewVueRouter({ // 改为export default以使其可外部访问routes: [
{ path: '/', redirect: '/hello' }, // 配置默认重定向到hello组件视图
{ path: '/hello', component: Hello },
{ path: '/hi', component: Hi },
]
});
20. Vue-路由嵌套
在路由配置router中可以使用children添加嵌套路由。
Copy{ path: '/hello',
component: Hello,
redirect: '/hello/hello1', // Hello充电线到/hello/hello1children:[ // 添加嵌套路由
{path: 'hello1', component: Hello1},
{path: 'hello2', component: Hello2},
]
}
在router-link中连接子路由要使用全路径
Copy<ul><li><router-linkto="/hello/hello1">Hello1</router-link></li><li><router-linkto="/hello/hello2">Hello2</router-link></li></ul><router-view/>
示例:
新建src/components/hello1.vue
Copy<template><divclass="hello1">
Hello1组件
</div></template><script>exportdefault{
name: 'hello1',
data(){
return {
}
}
}
</script><stylelang='css'></style>
新建src/components/hello2.vue
Copy<template><divclass="hello2">
Hello2组件
</div></template><script>exportdefault{
name: 'hello2',
data(){
return {
}
}
}
</script><stylelang='css'></style>
编辑src/routeindex.js
CopyimportVuefrom'vue'importVueRouterfrom"vue-router"importHellofrom"../components/hello"//改为两个..importHifrom"../components/hi"//改为两个..importHello1from"../components/hello1"importHello2from"../components/hello2"Vue.use(VueRouter);
exportdefaultnewVueRouter({ // 改为export default以使其可外部访问routes: [
{ path: '/', redirect: '/hello' }, // 配置默认重定向到hello组件视图
{ path: '/hello', component: Hello, redirect: '/hello/hello1', // Hello充电线到/hello/hello1children:[ // 添加嵌套路由
{path: 'hello1', component: Hello1},
{path: 'hello2', component: Hello2},
]},
{ path: '/hi', component: Hi },
]
});
编辑src/components/hello.vue
Copy<template><divclass="hello">
Hello组件
<ul><li><router-linkto="/hello/hello1">Hello1</router-link></li><li><router-linkto="/hello/hello2">Hello2</router-link></li></ul><router-view/></div></template><script>exportdefault{
name: 'hello',
data(){
return {
}
}
}
</script><stylelang='css'></style>
21. Vue-路由参数传递
router-link
Copy<router-linkto="/hi">Hi</router-link>
也可以写为
Copy<router-linkv-bind:to='{ path: "/hi"}'>Hi</router-link>
(1)配置理由信息:route中为路由添加name和参数
Copy{ path: '/hi/:id/:count', component: Hi, name: 'hi'},
(2)配置路径调整信息
Copy<router-link v-bind:to='{ name: "hi", params: {id: "100", count: "10"}}'>Hi</router-link>
(3)读取信息
Copy{{ $route.params.id }}
示例:
修改src/route/index.js
CopyimportVuefrom'vue'importVueRouterfrom"vue-router"importHellofrom"../components/hello"//改为两个..importHifrom"../components/hi"//改为两个..Vue.use(VueRouter);
exportdefaultnewVueRouter({ // 改为export default以使其可外部访问routes: [
{ path: '/', redirect: '/hello' }, // 配置默认重定向到hello组件视图
{ path: '/hello', component: Hello},
{ path: '/hi/:id/:count', component: Hi, name: 'hi'}, //添加name和参数id和count
]
});
修改src/components/nav.vue
Copy<template><divclass="nav"><ul><li><router-linkto="/hello">Hello</router-link></li><li><!-- <router-link to="/hi">Hi</router-link> --><!-- <router-link v-bind:to='{ path: "/hi"}'>Hi</router-link> --><router-linkv-bind:to='{ name: "hi", params: {id: "100", count: "10"}}'>Hi</router-link><!--使用参数--></li></ul></div></template><script>exportdefault{
name: 'nav2',
data(){
return {
}
},
}
</script><stylelang='css'></style>
修改src/components/h1.vue
Copy<template><divclass="hi">
Hi组件:
{{ $route.params.id }} - {{ $route.params.count }}
</div></template><script>exportdefault{
name: 'hi',
data(){
return {
}
}
}
</script><stylelang='css'></style>
22. Vue-路由高亮
可以通过对router-link-active设置样式实现高亮
Copy.router-link-active{
color: red !important;
}
可以在VueRouter中使用linkActiveClass: "active"设置active类并添加属性
还可以通过mode: "history"设置历史模式
示例:
src/route/index.js
CopyimportVuefrom'vue'importVueRouterfrom"vue-router"importHellofrom"../components/hello"//改为两个..importHifrom"../components/hi"//改为两个..importHello1from"../components/hello1"importHello2from"../components/hello2"Vue.use(VueRouter);
exportdefaultnewVueRouter({ // 改为export default以使其可外部访问linkActiveClass: "active",
mode: "history", // 使用历史模式routes: [
{ path: '/', redirect: '/hello' }, // 配置默认重定向到hello组件视图
{ path: '/hello', component: Hello, redirect: '/hello/hello1', // Hello充电线到/hello/hello1children:[ // 添加嵌套路由
{path: 'hello1', component: Hello1},
{path: 'hello2', component: Hello2},
]},
{ path: '/hi/:id/:count', component: Hi, name: 'hi'}, //添加name和参数id和count
]
});
src/App.vue中
Copy<template><divid="app"><Nav/><router-view/></div></template><script>importNavfrom"./components/nav"exportdefault {
name: 'App',
data(){
return {
}
},
components: {
Nav
}
}
</script><style>body{
background-color: #f1f1f1;
}
*{
margin: 0;
padding: 0;
}
a:link,a:hover,a:active,a:visited{
color: blue;
}
/* .router-link-active{
color: red !important;
}*/.active{
color: red !important;
}
</style>
23. Vue-element-ui组件
官方文档:https://element.eleme.cn/#/zh-CN/component/installation
安装element-ui: npm i element-ui --save
安装按需加载的依赖: npm install babel-plugin-component --save-dev
修改.babelrc
Copy{"presets":[["env",{"modules":false,"targets":{"browsers":["> 1%","last 2 versions","not ie <= 8"]}}],"stage-2"],"plugins":["transform-vue-jsx","transform-runtime",["component",{"libraryName":"element-ui","styleLibraryName":"theme-chalk "}]]}
引入组件(main.js)
Copyimport { Button } from'element-ui'Vue.use(Button)
使用组件
Copy<el-button type="primary">主要按钮</el-button>
注
npm install -d 就是npm install --save-dev
npm insatll -s 就是npm install --save
使用 --save-dev 安装的 插件,被写入到 devDependencies 域里面去,而使用 --save 安装的插件,则是被写入到 dependencies 区块里面去。
问题: throttle-debounce/throttle 安装不上 --未出现
presets中添加["es2015", { "modules": false }] 会报错
示例:
src/main.js
CopyimportVuefrom'vue'importAppfrom'./App'import { Button, Select, Option, Steps, Step } from'element-ui'// 按需导入Vue.use(Button)
Vue.use(Select)
Vue.use(Option)
Vue.use(Steps)
Vue.use(Step)
Vue.config.productionTip = falsenewVue({
el: '#app',
components: { App },
template: '<App/>'
})
src/App.vue
Copy<template><divid="app"><el-buttontype="primary">主要按钮</el-button><el-selectv-model="value"placeholder="请选择"><el-optionv-for="item in options":key="item.value":label="item.label":value="item.value"></el-option></el-select><br/><el-steps:active="active"finish-status="success"><el-steptitle="步骤 1"></el-step><el-steptitle="步骤 2"></el-step><el-steptitle="步骤 3"></el-step></el-steps><el-buttonstyle="margin-top: 12px;" @click="next">下一步</el-button></div></template><script>exportdefault {
name: 'App',
data(){
return {
active: 0,
options: [{
value: '选项1',
label: '黄金糕'
}, {
value: '选项2',
label: '双皮奶'
}, {
value: '选项3',
label: '蚵仔煎'
}, {
value: '选项4',
label: '龙须面'
}, {
value: '选项5',
label: '北京烤鸭'
}],
}
},
methods: {
next() {
if (this.active++ > 2) this.active = 0;
}
}
}
</script><style></style>
24. Vue-常用组件swiper
swiper常用于移动端网站的内容触摸滑动
官网文档:https://github.surmon.me/vue-awesome-swiper/
中文文档:https://www.swiper.com.cn/api/
安装:npm install --save vue-avesome-swiper
(1)main.js中引入swipper
CopyimportVueAwesomeSwiperfrom'vue-awesome-swiper'import'swiper/dist/css/swiper.css'Vue.use(VueAwesomeSwiper)
(2)组件中使用
Copy<swiper:options="swiperOption"class="hello"><swiper-slidev-for="(slide, index) in swiperSlides":key="index"><p>{{ slide.title }}</p><p>{{ slide.type }}</p></swiper-slide><divclass="swiper-pagination"slot="pagination"></div><divclass="swiper-button-prev"slot="button-prev"></div><divclass="swiper-button-next"slot="button-next"></div></swiper>
(3)选项设置
Copydata() {
return {
swiperOption: {
pagination: {
el: '.swiper-pagination'
},
navigation: { // 配置左右按钮可点nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
},
swiperSlides: [],
}
},
示例:
src/main.js
CopyimportVuefrom'vue'importAppfrom'./App'importVueAwesomeSwiperfrom'vue-awesome-swiper'importAxiosfrom'axios'import'swiper/dist/css/swiper.css'Vue.prototype.$axios = Axios; // 不使用 Vue.use(Axios),而是绑定原型Vue.use(VueAwesomeSwiper)
// Axios.defaults.baseURL = 'https://httpbin.org'Vue.config.productionTip = falsenewVue({
el: '#app',
components: { App },
template: '<App/>'
})
src/App.vue
Copy<template><divid="app"><swiper:options="swiperOption"class="hello"><swiper-slidev-for="(slide, index) in swiperSlides":key="index"><p>{{ slide.title }}</p><p>{{ slide.type }}</p></swiper-slide><divclass="swiper-pagination"slot="pagination"></div><divclass="swiper-button-prev"slot="button-prev"></div><divclass="swiper-button-next"slot="button-next"></div></swiper></div></template><script>exportdefault {
name: 'App',
data() {
return {
swiperOption: {
pagination: {
el: '.swiper-pagination'
},
navigation: { // 配置左右按钮可点nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
},
swiperSlides: [],
}
},
mounted() {
this.$axios.get('https://httpbin.org/json')
.then(res => {
console.log(res.data.slideshow.slides)
this.swiperSlides = res.data.slideshow.slides;
})
.catch(error =>{console.log(error)})
// setInterval(() => {// console.log('simulate async data')// if (this.swiperSlides.length < 10) {// this.swiperSlides.push(this.swiperSlides.length + 1)// }// }, 3000)
}
}
</script><style>.hello {
width: 600px;
height: 400px;
background-color: #f1f1f1;
}
</style>
如有不懂还要咨询下方小卡片,博主也希望和志同道合的测试人员一起学习进步
在适当的年龄,选择适当的岗位,尽量去发挥好自己的优势。
我的自动化测试开发之路,一路走来都离不每个阶段的计划,因为自己喜欢规划和总结,
测试开发视频教程、学习笔记领取传送门!!!
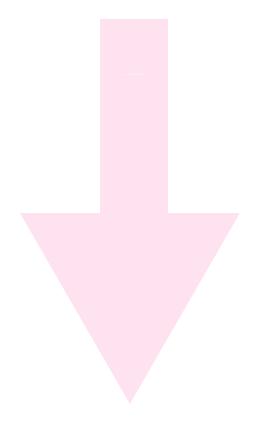