instance:
hostname: eureka7001
client:
#false表示不向注册中心注册自己
register-with-eureka: false
#false表示自己就是注册中心,我的职责是维护服务实例,并不需要去检索服务
fetch-registry: false
service-url:
#设置与eureka server交互的地址查询服务和注册服务都依赖这个地址
defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
[](https://gitee.com/vip204888/java-p7)4\. 测试
-------------------------------------------------------------------------
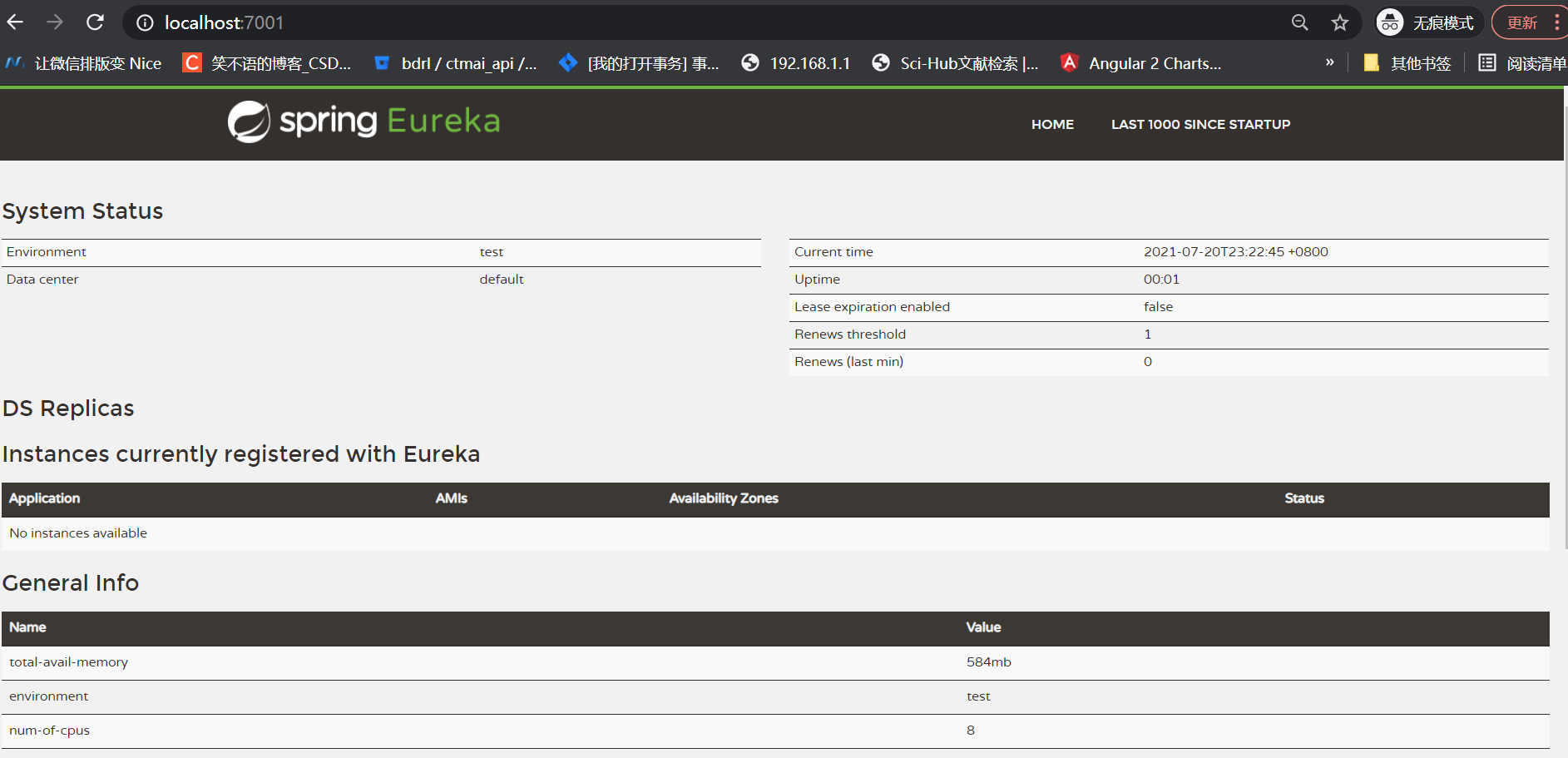
[](https://gitee.com/vip204888/java-p7)三、Eureka Server集群部署
======================================================================================
[](https://gitee.com/vip204888/java-p7)1\. pom依赖引用(和单机版一致)
--------------------------------------------------------------------------------------
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
<!--web相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-actuator</artifactId>
</dependency>
[](https://gitee.com/vip204888/java-p7)2\. 配置启动类
----------------------------------------------------------------------------
//eureka-7001
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
//表示服务注册中心
@EnableEurekaServer
@SpringBootApplication
public class EurekaApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaApplication.class,args);
}
}
//eureka-7002
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
//表示服务注册中心
@EnableEurekaServer
@SpringBootApplication
public class EurekaApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaApplication.class,args);
}
}
[](https://gitee.com/vip204888/java-p7)3\. yml配置
----------------------------------------------------------------------------
7001
server:
port: 7001
spring:
application:
name: cloud-eureka-service
eureka:
instance:
hostname: eureka7001
client:
#false表示不向注册中心注册自己
register-with-eureka: false
#false表示自己就是注册中心,我的职责是维护服务实例,并不需要去检索服务
fetch-registry: false
service-url:
#设置与eureka server交互的地址查询服务和注册服务都依赖这个地址
defaultZone: http://eureka7002:7002/eureka/
7002
server:
port: 7002
spring:
application:
name: cloud-eureka-service
eureka:
instance:
hostname: eureka7002
client:
#false表示不向注册中心注册自己
register-with-eureka: false
#false表示自己就是注册中心,我的职责是维护服务实例,并不需要去检索服务
fetch-registry: false
service-url:
#设置与eureka server交互的地址查询服务和注册服务都依赖这个地址
defaultZone: http://eureka7001:7001/eureka/
[](https://gitee.com/vip204888/java-p7)4\. 测试
-------------------------------------------------------------------------
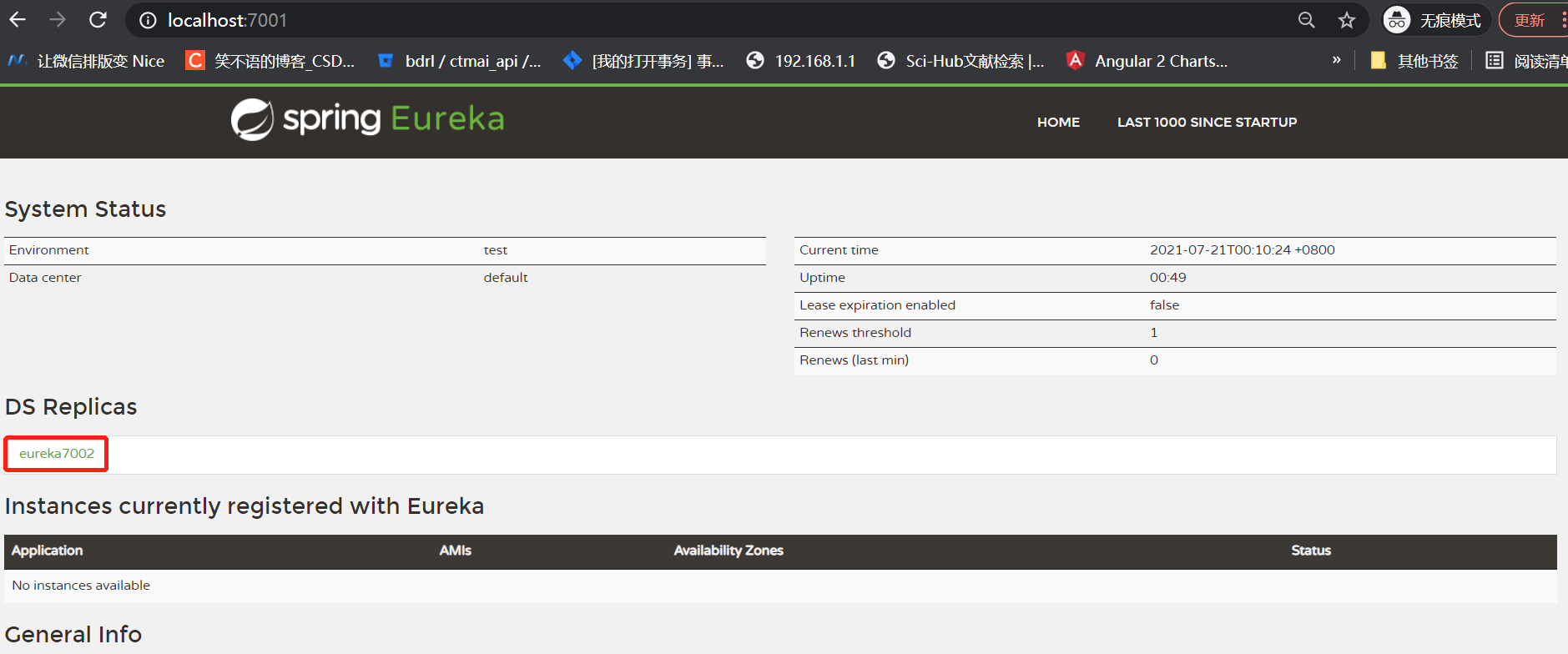
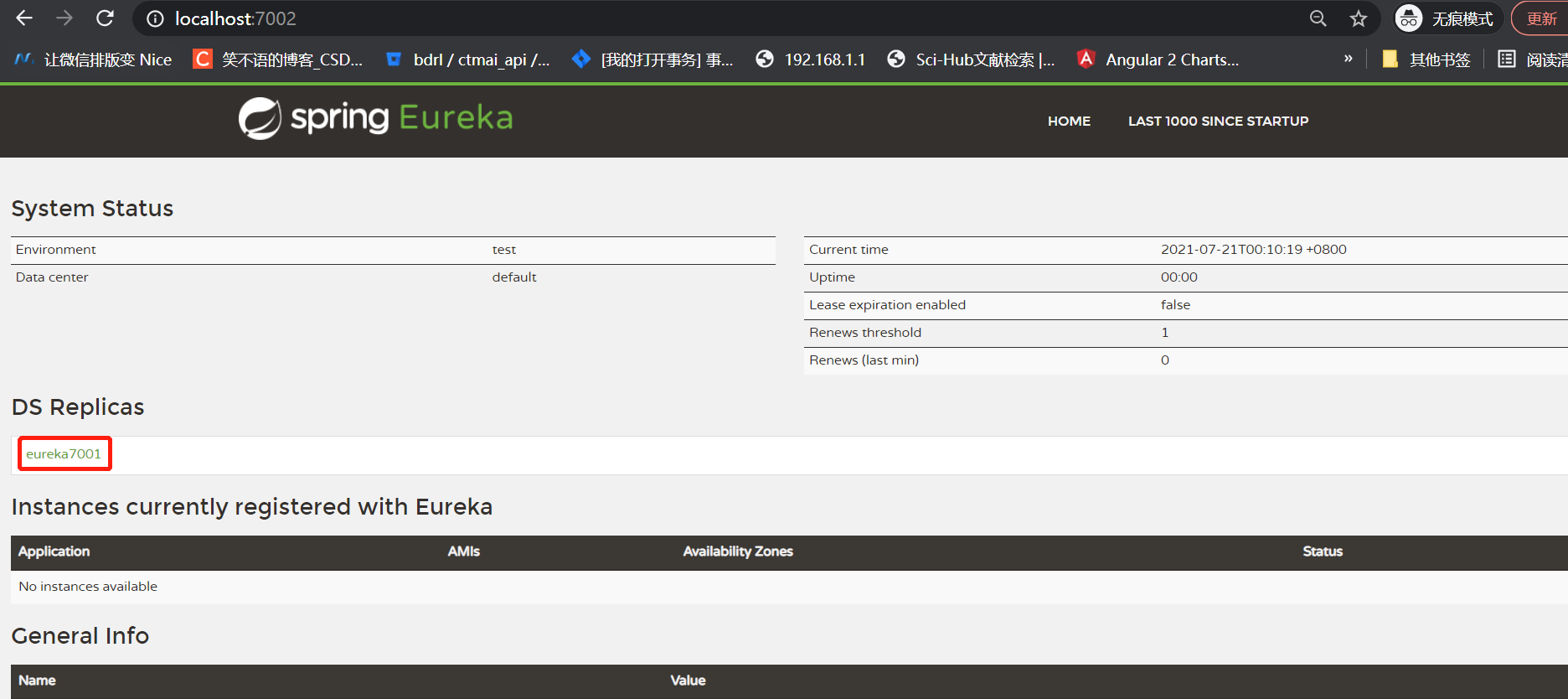
[](https://gitee.com/vip204888/java-p7)四、Eureka client部署(服务消费者和服务提供者)
=================================================================================================
[](https://gitee.com/vip204888/java-p7)1\. pom依赖引入
------------------------------------------------------------------------------
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!--web相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
[](https://gitee.com/vip204888/java-p7)2\. 配置主启动类
-----------------------------------------------------------------------------
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@EnableEurekaClient
@SpringBootApplication
public class PaymentApplication {
public static void main(String[] args) {
SpringApplication.run(PaymentApplication.class,args);
}
}
[](https://gitee.com/vip204888/java-p7)3\. yml配置
----------------------------------------------------------------------------
server:
port: 8888
eureka:
client:
register-with-eureka: true
fetch-registry: true
service-url:
defaultZone: http://eureka7001:7001/eureka,http://eureka7002:7002/eureka
instance:
prefer-ip-address: true
instance-id: payment-8888
[](https://gitee.com/vip204888/java-p7)4\. 测试
-------------------------------------------------------------------------
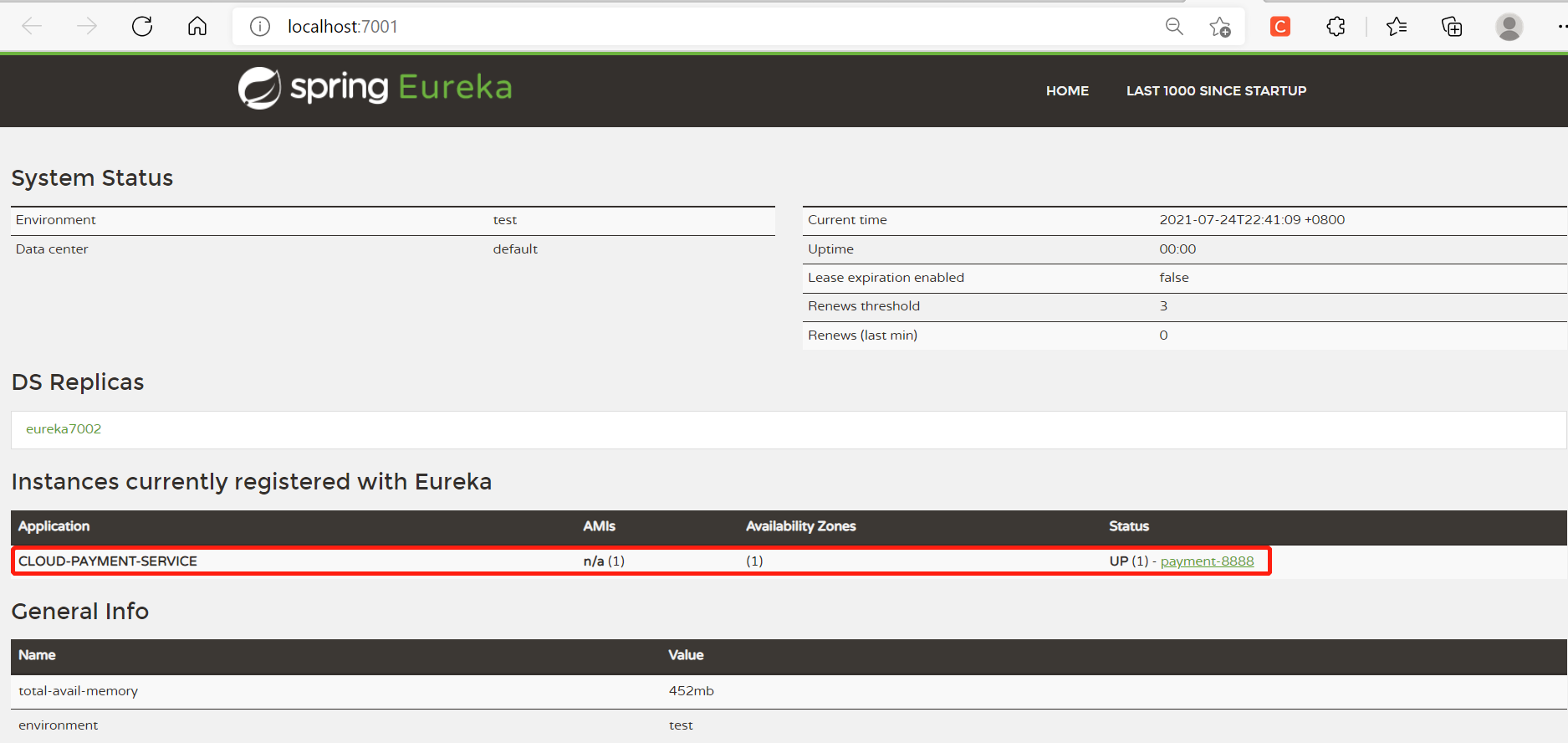
[](https://gitee.com/vip204888/java-p7)五、配置actuator信息
=================================================================================
eureka:
client:
register-with-eureka: true
fetch-registry: true
service-url:
defaultZone: http://eureka7001:7001/eureka,http://eureka7002:7002/eureka
instance:
prefer-ip-address: true #显示主机ip名称
instance-id: payment-8888 #实例id
management:
endpoint:
health:
show-details: always #显示详细信息
endpoints:
web:
exposure:
exclude: env #排除actuator暴露的接口
include: ["health","info","mappings"] #设置暴露的actuator接口
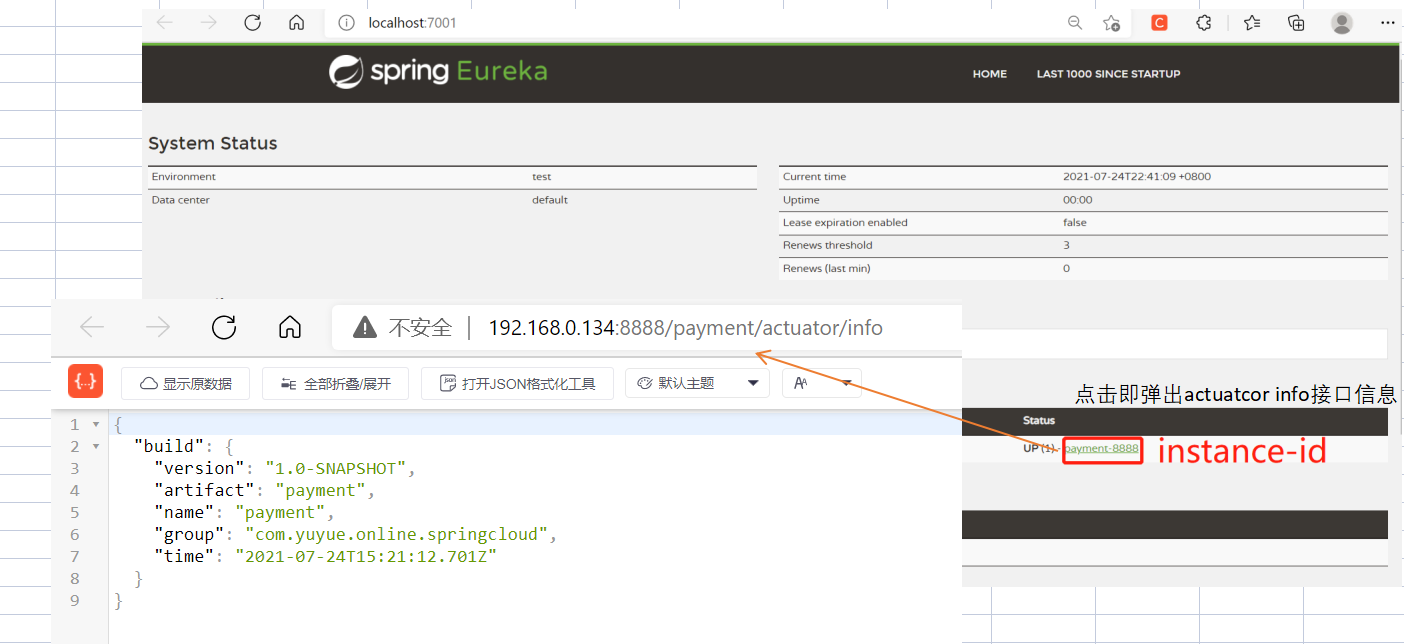
[](https://gitee.com/vip204888/java-p7)_**温馨提示**_
-----------------------------------------------------------------------------
> 点击如果/info获取空信息获取到的数据为空,需要做如下配置:
1. pom文件配置,添加springboot插件
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<finalName>${project.name}</finalName>
</configuration>
</plugin>
</plugins>
</build>
2. 执行springboot:build-info
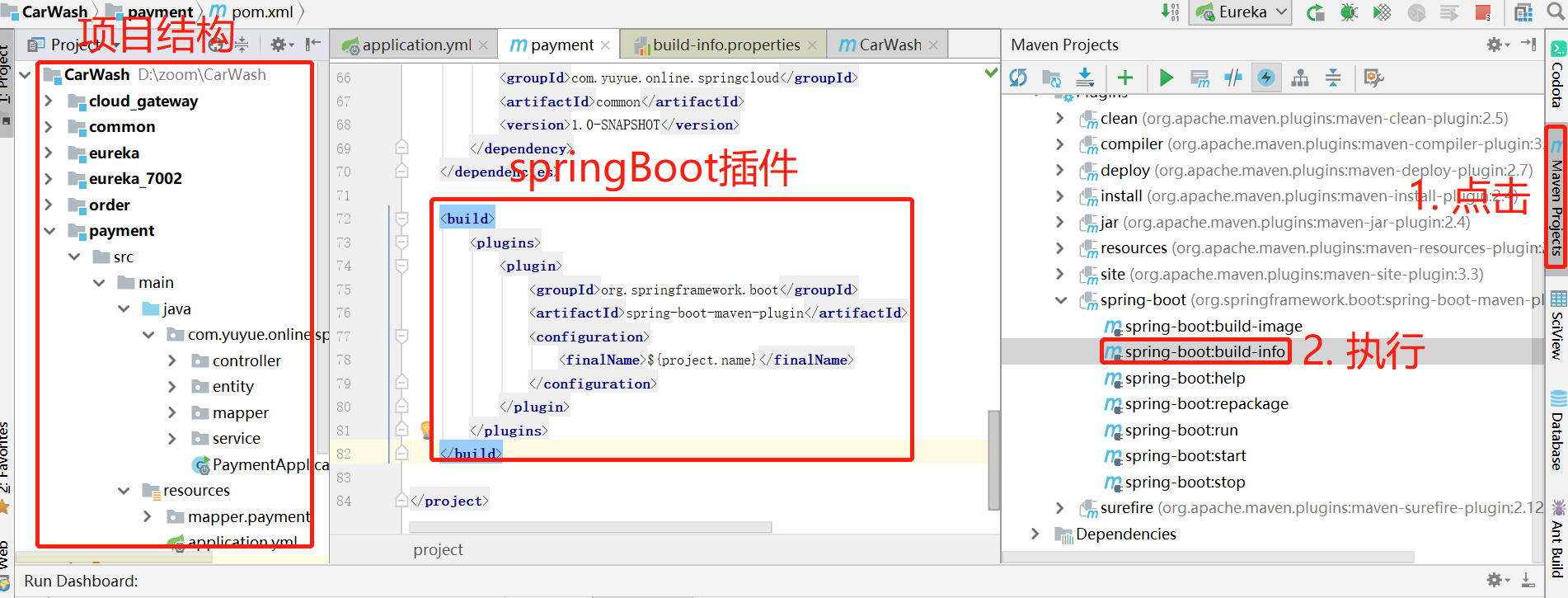
3. 执行成功后,重启项目即可获取info的build信息
最后
最后,强调几点:
- 1. 一定要谨慎对待写在简历上的东西,一定要对简历上的东西非常熟悉。因为一般情况下,面试官都是会根据你的简历来问的; 能有一个上得了台面的项目也非常重要,这很可能是面试官会大量发问的地方,所以在面试之前好好回顾一下自己所做的项目;
- 2. 和面试官聊基础知识比如设计模式的使用、多线程的使用等等,可以结合具体的项目场景或者是自己在平时是如何使用的;
- 3. 注意自己开源的Github项目,面试官可能会挖你的Github项目提问;
我个人觉得面试也像是一场全新的征程,失败和胜利都是平常之事。所以,劝各位不要因为面试失败而灰心、丧失斗志。也不要因为面试通过而沾沾自喜,等待你的将是更美好的未来,继续加油!
以上面试专题的答小编案整理成面试文档了,文档里有答案详解,以及其他一些大厂面试题目。
如何获取整理好的Java面试专题资料?
面试答案
; 能有一个上得了台面的项目也非常重要,这很可能是面试官会大量发问的地方,所以在面试之前好好回顾一下自己所做的项目;
- 2. 和面试官聊基础知识比如设计模式的使用、多线程的使用等等,可以结合具体的项目场景或者是自己在平时是如何使用的;
- 3. 注意自己开源的Github项目,面试官可能会挖你的Github项目提问;
我个人觉得面试也像是一场全新的征程,失败和胜利都是平常之事。所以,劝各位不要因为面试失败而灰心、丧失斗志。也不要因为面试通过而沾沾自喜,等待你的将是更美好的未来,继续加油!
以上面试专题的答小编案整理成面试文档了,文档里有答案详解,以及其他一些大厂面试题目。
如何获取整理好的Java面试专题资料?
面试答案
[外链图片转存中…(img-bunzYfm1-1628146221815)]
[外链图片转存中…(img-Dshek4tF-1628146221817)]