-
@Date 2021/6/22 13:55
-
@Description :
*/
public interface MailService {
/**
* 发送邮件
* @param mailDto d
*/
void send(MailDto mailDto);
}
import com.zh.wit.sendMail.dto.MailDto;
import com.zh.wit.sendMail.service.MailService;
import lombok.RequiredArgsConstructor;
import org.springframework.mail.MailSender;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Service;
/**
-
@Author YMG
-
@Date 2021/6/22 13:57
-
@Description :
*/
@Service
@RequiredArgsConstructor
@Component
public class MailServiceImpl implements MailService {
private final MailSender mailSender;
@Override
public void send(MailDto mailDto) {
// new 一个简单邮件消息对象
SimpleMailMessage message = new SimpleMailMessage();
// 和配置文件中的的username相同,相当于发送方(配置文件在下properties)
message.setFrom("ymg@163.com");
// 收件人邮箱
message.setBcc(mailDto.getMail());
//抄送人
message.setCc("ymg@163.com");
message.setSubject(mailDto.getTitle());
// 正文
message.setText(mailDto.getContent());
// 发送
mailSender.send(message);
}
}
④配置application.properties
server.port=9029
#邮件配置
#邮箱服务器地址,如果是QQ邮箱—>(smtp.qq.com)
spring.mail.host=smtp.163.com
#发送邮件的邮箱(自己邮箱)
spring.mail.username=ymg@163.com
#第一步页面配置得到的授权密码
spring.mail.password=EXIPENWAJYHERYDX
spring.mail.default-encoding=UTF-8
#(注意!!!以上配置,本地默认25端口访问邮箱服务器,如果需要放到服务器上,必须添加如下配置,用465端口访问才能访问到邮箱服务器)
#登录服务器是否需要认证
spring.mail.properties.mail.smtp.auth=true
#SSL证书Socket工厂
spring.mail.properties.mail.smtp.socketFactory.class=javax.net.ssl.SSLSocketFactory
#使用SMTPS协议465端口
spring.mail.properties.mail.smtp.socketFactory.port=465
⑤编写定时器执行的方法,不要问为什么写controller,因为前期好测试,使用定时器的话,主程序启动千万别忘了配置
@EnableScheduling注解(启动项目加载定时器)
import com.zh.wit.bo.CommonResult;
import com.zh.wit.bo.RestResult;
import com.zh.wit.sendMail.dto.MailDto;
import com.zh.wit.sendMail.service.MailService;
import com.zh.wit.utils.Per;
import lombok.RequiredArgsConstructor;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.web.bind.annotation.*;
import java.io.IOException;
/**
* @Author YMG
* @Date 2021/6/22 13:59
* @Description :
*/
@RequiredArgsConstructor
@RestController
@RequestMapping(value = "/api/mail")
public class MailController {
private final MailService mailService;
//spring定时器注解(每天凌晨三点三分发送邮件),因为凌晨三点三分眼前都还是你
@Scheduled(cron = "0 03 03 ? * *")
public void sendMail() throws IOException {
//收件人数组(可以添加多个邮箱)
String[] str = {"xxx@qq.com","xxx163.com"};
MailDto mailDto = new MailDto();
mailDto.setMail(str);
mailDto.setTitle("今天你也要开心!");
//以下是发送的内容
mailDto.setContent(Per.getWe());
mailService.send(mailDto);
}
@GetMapping(value = "/send")
public RestResult<Object> sendShe(){
return RestResult.result(CommonResult.FIND_SUCCESS,"hello");
}
}
```
⑥处理邮件发送的内容,心知天气[https://www.seniverse.com/](https://gitee.com/vip204888/java-p7)数据解析(这个数据解析写的比较乱,可自行处理一下)
```
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.URLConnection;
import java.nio.charset.StandardCharsets;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
* @Author YMG
* @Date 2021/6/24 14:55
* @Description : 以下每个URL地址都是(心知天气的API) 只需要更换 城市 还有 购买的key就能访问到数据
*/
public class Per {
public static String getWe() throws IOException {
URL url = new URL("https://api.seniverse.com/v3/weather/daily.json?key=SIJ_kXNjjhOcycKI6&location=成都&language=zh-Hans&unit=c&start=0&days=1");
URLConnection connectionData = url.openConnection();
connectionData.setConnectTimeout(1000);
BufferedReader br = new BufferedReader(new InputStreamReader(
connectionData.getInputStream(), StandardCharsets.UTF_8));
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
String data = sb.toString();
JSONObject jsonData = JSON.parseObject(data);
JSONArray results = jsonData.getJSONArray("results");
JSONArray daily = results.getJSONObject(0).getJSONArray("daily");
JSONObject jsonObject = daily.getJSONObject(0);
Map<String, Object> dailyMap = new HashMap<>();
for (Map.Entry<String, Object> entry : jsonObject.entrySet()) {
dailyMap.put(entry.getKey(), entry.getValue());
}
return getOneS() + "\n" + "\n" + getYe()
+ "\n" + "===================="
+ "\n" + "白天天气:" + "\t" + dailyMap.get("text_day")
+ "\n" + "晚间天气:" + "\t" + dailyMap.get("text_night")
+ "\n" + "最高温度(℃):" + "\t" + dailyMap.get("high")
+ "\n" + "最低温度(℃):" + "\t" + dailyMap.get("low")
+ "\n" + "相对湿度(%):" + "\t" + dailyMap.get("humidity")
+ "\n" + "当前能见度(km):" + "\t" + getSk().get("visibility")
+ "\n" + "气压(Pa):" + "\t" + getSk().get("pressure")
+ "\n" + "风向:" + "\t" + dailyMap.get("wind_direction")
+ "\n" + "风速(km/h):" + "\t" + dailyMap.get("wind_speed")
+ "\n" + "风力等级:" + "\t" + dailyMap.get("wind_scale")
+ "\n" + "===================="
+ "\n" + "\n" + getSe();
}
public static String getSe() throws IOException {
URL url = new URL("https://api.seniverse.com/v3/life/suggestion.json?key=SIJ_kXNjjhOcycKI6&location=成都&language=zh-Hans");
URLConnection connectionData = url.openConnection();
connectionData.setConnectTimeout(1000);
BufferedReader br = new BufferedReader(new InputStreamReader(
connectionData.getInputStream(), StandardCharsets.UTF_8));
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
String data = sb.toString();
JSONObject jsonData = JSON.parseObject(data);
JSONArray results = jsonData.getJSONArray("results");
JSONObject now = results.getJSONObject(0).getJSONObject("suggestion");
Map<String, Object> suMap = new HashMap<>();
for (Map.Entry<String, Object> entry : now.entrySet()) {
suMap.put(entry.getKey(), entry.getValue());
}
Map<String, Object> resultMap = new HashMap<>();
for (String s : suMap.keySet()) {
JSONObject jsonObject = JSON.parseObject(suMap.get(s).toString());
String resultStr = jsonObject.getString("brief") + "," + jsonObject.getString("details");
resultMap.put(s, resultStr);
}
return "生活指数:"
+ "\n" + "================================="
+ "\n" + "(空气污染):>>>" + "\t" + resultMap.get("air_pollution")
+ "\n" + "(紫外线):>>>" + "\t" + resultMap.get("uv")
+ "\n" + "(舒适度):>>>" + "\t" + resultMap.get("comfort")
+ "\n" + "(雨伞):>>>" + "\t" + resultMap.get("umbrella")
+ "\n" + "(化妆):>>>" + "\t" + resultMap.get("makeup")
+ "\n" + "(防晒):>>>" + "\t" + resultMap.get("sunscreen")
+ "\n" + "(过敏):>>>" + "\t" + resultMap.get("allergy")
+ "\n" + "(风寒):>>>" + "\t" + resultMap.get("chill")
+ "\n" + "(感冒):>>>" + "\t" + resultMap.get("flu")
+ "\n" + "(约会):>>>" + "\t" + resultMap.get("dating")
+ "\n" + "(运动):>>>" + "\t" + resultMap.get("sport")
+ "\n" + "(旅游):>>>" + "\t" + resultMap.get("travel")
+ "\n" + "(晾晒):>>>" + "\t" + resultMap.get("airing")
+ "\n" + "=================================";
}
public static Map<String, Object> getSk() throws IOException {
URL url = new URL("https://api.seniverse.com/v3/weather/now.json?key=SIJ_kXNjjhOcycKI6&location=成都&language=zh-Hans&unit=c");
URLConnection connectionData = url.openConnection();
connectionData.setConnectTimeout(1000);
BufferedReader br = new BufferedReader(new InputStreamReader(
connectionData.getInputStream(), StandardCharsets.UTF_8));
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
String data = sb.toString();
JSONObject jsonData = JSON.parseObject(data);
## 总结
在清楚了各个大厂的面试重点之后,就能很好的提高你刷题以及面试准备的效率,接下来小编也为大家准备了最新的互联网大厂资料。
> **[资料领取:点我即可免费领取](https://gitee.com/vip204888/java-p7)**
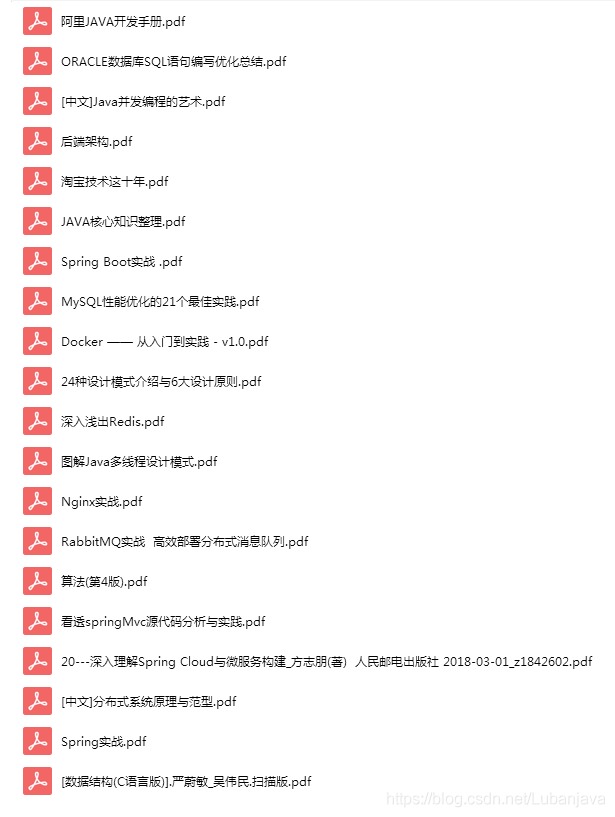
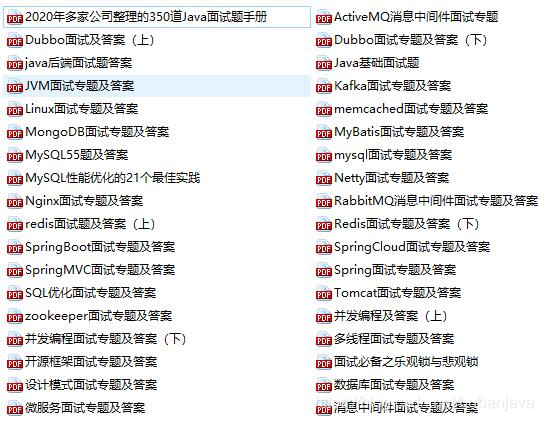
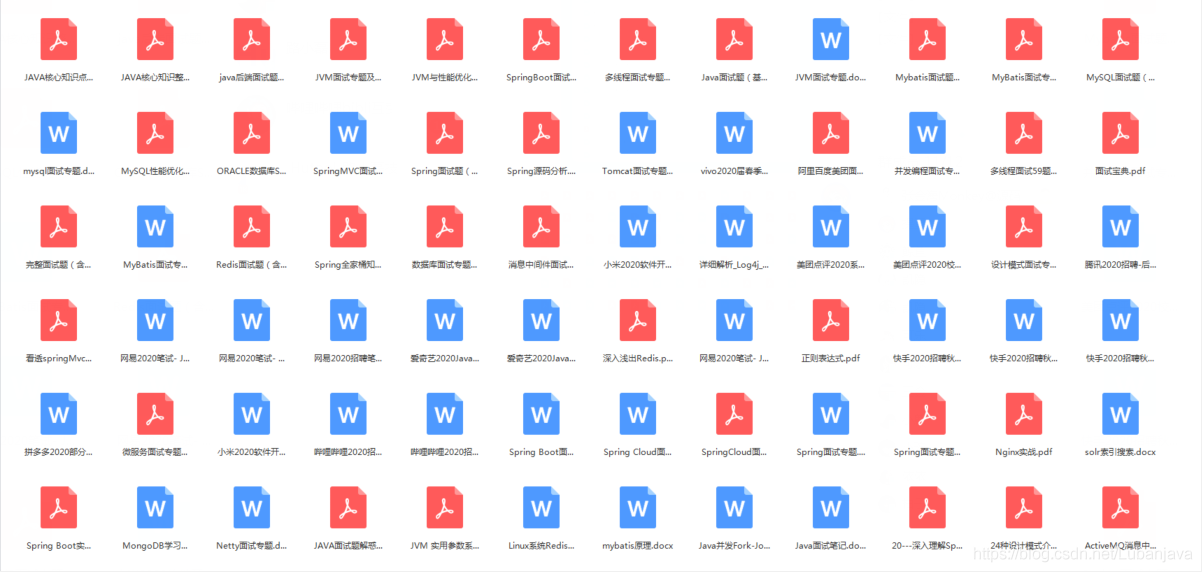
r sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
String data = sb.toString();
JSONObject jsonData = JSON.parseObject(data);
## 总结
在清楚了各个大厂的面试重点之后,就能很好的提高你刷题以及面试准备的效率,接下来小编也为大家准备了最新的互联网大厂资料。
> **[资料领取:点我即可免费领取](https://gitee.com/vip204888/java-p7)**
[外链图片转存中...(img-NsGAPSza-1628419154878)]
[外链图片转存中...(img-GfL96wJw-1628419154880)]
[外链图片转存中...(img-gPN7OQbD-1628419154882)]
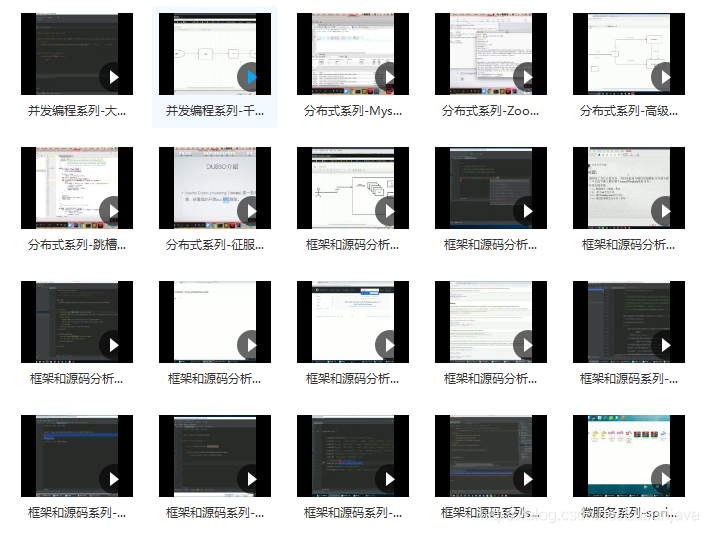