float_number = 7.0
# Another way of declaring float is using float() function.
float_number_via_function = float(7)
float_negative = -35.59
assert float_number == float_number_via_function
assert isinstance(float_number, float)
assert isinstance(float_number_via_function, float)
assert isinstance(float_negative, float)
# Float can also be scientific numbers with an “e” to indicate
# the power of 10.
float_with_small_e = 35e3
float_with_big_e = 12E4
assert float_with_small_e == 35000
assert float_with_big_e == 120000
assert isinstance(12E4, float)
assert isinstance(-87.7e100, float)
def test_complex_numbers():
“”“Complex Type”“”
complex_number_1 = 5 + 6j
complex_number_2 = 3 - 2j
assert isinstance(complex_number_1, complex)
assert isinstance(complex_number_2, complex)
assert complex_number_1 * complex_number_2 == 27 + 8j
def test_number_operators():
“”“Basic operations”“”
# Addition.
assert 2 + 4 == 6
# Multiplication.
assert 2 * 4 == 8
# Division always returns a floating point number.
assert 12 / 3 == 4.0
assert 12 / 5 == 2.4
assert 17 / 3 == 5.666666666666667
# Modulo operator returns the remainder of the division.
assert 12 % 3 == 0
assert 13 % 3 == 1
# Floor division discards the fractional part.
assert 17 // 3 == 5
# Raising the number to specific power.
assert 5 ** 2 == 25 # 5 squared
assert 2 ** 7 == 128 # 2 to the power of 7
# There is full support for floating point; operators with
# mixed type operands convert the integer operand to floating point.
assert 4 * 3.75 - 1 == 14.0
File
- Read File
- Write a File
- Copy File
- Move File
- Readline
Read a file
在Python 3中,如果文件未以二进制模式打开,则编码将由locale.getpreferredencoding(False)或用户输入确定。
with open(“/etc/hosts”, encoding=“utf-8”) as f:
… content = f.read()
…
print(type(content))
In python3 binary mode
with open(“/etc/hosts”, “rb”) as f:
… content = f.read()
…
print(type(content))
In python2
## The content of the file is a byte string, not a Unicode string.
with open(“/etc/passwd”) as f:
… content = f.read()
print(type(content))
print(type(content.decode(“utf-8”)))
Write a File
file_content = “Python is great!”
with open(“check.txt”, “w”) as file:
… file.write(file_content)
Copy a file
from distutils.file_util import copy_file
copy_file(“a”, “b”)
( b , 1)
Move a File
from distutils.file_util import move_file
move_file(“./a”, “./b”)
./b
Readline
## If you are not using linux machine try to change the file path to read
with open(“/etc/hosts”) as f:
… for line in f:
… print(line, end= )
…
127.0.0.1 localhost
255.255.255.255 broadcasthost
::1 localhost
Python Functions
- Lambda
- Declare Function
- Document Function
- Get Function Name
- Arguments
- Decorator
- Generator
Lambda
fn = lambda x: x**3
fn(2)
8
(lambda x: x**3)(2)
8
(lambda x: [x * _ for _ in range(10)])(3)
[0, 3, 6, 9, 12, 15, 18, 21, 24, 27]
Declare Function
def fn_name(): ## Where fn_name represents the function name
…
…
Get Function Name
## First Declare a function
def fn_name():
…
…
fn_name.__name__
fn_name
Document a Function
## Document a function with three single quotes
def check():
This function is documented
return
check.__doc__
This function is documented
Arguments
def multiply(a, b=0): ## b is 0 by default
… return a * b
…
multiply(1, 3) ## 3 * 1
3
multiply(5) ## 5 * 0
0
multiply(5, b=3) ## 5 * 3
15
Generator
def count(start, step):
while True:
yield start
start += step
counter = count(10, 5)
next(counter)
(15)
next(counter) ## Increments by 5 from the previous result
(20)
next(counter)
(25)
next(counter), next(counter), next(counter)
(30, 35, 40)
Decorator
from functools import wraps
def decorator_func(func):
… @wraps(func)
… def wrapper(*args, **kwargs):
… print(“Before calling {}.”.format(func.__name__))
… ret = func(*args, **kwargs)
… print(“After calling {}.”.format(func.__name__))
… return ret
… return wrapper
…
@decorator_func
… def check():
… print(“Inside check function.”)
…
check()
Before calling check.
Inside check function.
After calling check.
Classes
- Definition
- Class Objects
- Instance Objects
- Method Objects
- Inheritance
- Multiple Inheritance
Class Definition
Python是一种面向对象的编程语言。 Python中的几乎所有东西都是一个对象,具有其属性和方法。 Class类似于对象构造函数,或者是用于创建对象的“蓝图”。
def test_class():
"""Class definition."""
# Class definitions, like function definitions (def statements) must be executed before they
# have any effect. (You could conceivably place a class definition in a branch of an if
# statement, or inside a function.)
class GreetingClass:
"""Example of the class definition
This class contains two public methods and doesn t contain constructor.
"""
name = Bisrat
def say_hello(self):
"""Class method."""
# The self parameter is a reference to the class itself, and is used to access variables
# that belongs to the class. It does not have to be named self , you can call it
# whatever you like, but it has to be the first parameter of any function in the class.
return Hello + self.name
def say_goodbye(self):
"""Class method."""
return Goodbye + self.name
# When a class definition is entered, a new namespace is created, and used as the local scope —
# thus, all assignments to local variables go into this new namespace. In particular, function
# definitions bind the name of the new function here.
# Class instantiation uses function notation. Just pretend that the class object is a
# parameterless function that returns a new instance of the class. For example the following
# code will creates a new instance of the class and assigns this object to the local variable.
greeter = GreetingClass()
assert greeter.say_hello() == Hello Bisrat
assert greeter.say_goodbye() == Goodbye Bisrat
>>> print(type(content))
<class str >
Class Objects
def test_class_objects():
“”“Class Objects.
Class objects support two kinds of operations:
- attribute references
- instantiation.
“””
# ATTRIBUTE REFERENCES use the standard syntax used for all attribute references in
# Python: obj.name. Valid attribute names are all the names that were in the class’s namespace
# when the class object was created. For class MyCounter the following references are valid
# attribute references:
class ComplexNumber:
“”“Example of the complex numbers class”“”
real = 0
imaginary = 0
def get_real(self):
“”“Return real part of complex number.”“”
return self.real
def get_imaginary(self):
“”“Return imaginary part of complex number.”“”
return self.imaginary
assert ComplexNumber.real == 0
# __doc__ is also a valid attribute, returning the docstring belonging to the class
assert ComplexNumber.__doc__ == Example of the complex numbers class
# Class attributes can also be assigned to, so you can change the value of
# ComplexNumber.counter by assignment.
ComplexNumber.real = 10
assert ComplexNumber.real == 10
# CLASS INSTANTIATION uses function notation. Just pretend that the class object is a
# parameterless function that returns a new instance of the class. For example
# (assuming the above class):
complex_number = ComplexNumber()
assert complex_number.real == 10
assert complex_number.get_real() == 10
# Let s change counter default value back.
ComplexNumber.real = 10
assert ComplexNumber.real == 10
# The instantiation operation (“calling” a class object) creates an empty object. Many classes
# like to create objects with instances customized to a specific initial state. Therefore a
# class may define a special method named __init__(), like this:
class ComplexNumberWithConstructor:
“”“Example of the class with constructor”“”
def __init__(self, real_part, imaginary_part):
self.real = real_part
self.imaginary = imaginary_part
def get_real(self):
“”“Return real part of complex number.”“”
return self.real
def get_imaginary(self):
“”“Return imaginary part of complex number.”“”
return self.imaginary
complex_number = ComplexNumberWithConstructor(3.0, -4.5)
assert complex_number.real, complex_number.imaginary == (3.0, -4.5)
Instance Object
def test_instance_objects():
# DATA ATTRIBUTES need not be declared; like local variables, they spring into existence when
# they are first assigned to. For example, if x is the instance of MyCounter created above,
# the following piece of code will print the value 16, without leaving a trace.
# pylint: disable=too-few-public-methods
class DummyClass:
pass
dummy_instance = DummyClass()
# pylint: disable=attribute-defined-outside-init
dummy_instance.temporary_attribute = 1
assert dummy_instance.temporary_attribute == 1
del dummy_instance.temporary_attribute
Method Objects
class MyCounter:
“”“A simple example of the counter class”“”
counter = 10
def get_counter(self):
“”“Return the counter”“”
return self.counter
def increment_counter(self):
“”“Increment the counter”“”
self.counter += 1
return self.counter
def test_method_objects():
“”“Method Objects.”“”
# object types can have methods as well. For example, list objects have methods called append,
counter = MyCounter()
assert counter.get_counter() == 10
get_counter = counter.get_counter
assert get_counter() == 10
assert counter.get_counter() == 10
assert MyCounter.get_counter(counter) == 10
Inheritance
# pylint: disable=too-few-public-methods
class Person:
“”“Example of the base class”“”
def __init__(self, name):
self.name = name
def get_name(self):
“”“Get person name”“”
return self.name
# The syntax for a derived class definition looks like this.
class Employee(Person):
def __init__(self, name, staff_id):
Person.__init__(self, name)
# You may also use super() here in order to avoid explicit using of parent class name:
# >>> super().__init__(name)
self.staff_id = staff_id
def get_full_id(self):
“”“Get full employee id”“”
return self.get_name() + , + self.staff_id
def test_inheritance():
“”“Inheritance.”“”
# There’s nothing special about instantiation of derived classes: DerivedClassName() creates a
# new instance of the class. Method references are resolved as follows: the corresponding class
# attribute is searched, descending down the chain of base classes if necessary, and the method
# reference is valid if this yields a function object.
person = Person( Bisrat )
employee = Employee( John , A23 )
assert person.get_name() == Bisrat
assert employee.get_name() == John
assert employee.get_full_id() == John, A23
# Python has two built-in functions that work with inheritance:
#
# - Use isinstance() to check an instance’s type: isinstance(obj, int) will be True only if
# obj.__class__ is int or some class derived from int.
#
# - Use issubclass() to check class inheritance: issubclass(bool, int) is True since bool is
# a subclass of int. However, issubclass(float, int) is False since float is not a subclass
# of int.
assert isinstance(employee, Employee)
assert not isinstance(person, Employee)
assert isinstance(person, Person)
assert isinstance(employee, Person)
assert issubclass(Employee, Person)
assert not issubclass(Person, Employee)
Multiple Inheritance
def test_multiple_inheritance():
"""Multiple Inheritance"""
class Clock:
"""Clock class"""
time = 10:17 PM
def get_time(self):
"""Get current time
Method is hardcoded just for multiple inheritance illustration.
"""
return self.time
class Calendar:
"""Calendar class"""
date = 12/08/2018
def get_date(self):
"""Get current date
Method is hardcoded just for multiple inheritance illustration.
"""
return self.date
# Python supports a form of multiple inheritance as well. A class definition with multiple
# base classes looks like this.
class CalendarClock(Clock, Calendar):
calendar_clock = CalendarClock()
assert calendar_clock.get_date() == 12/08/2018
assert calendar_clock.get_time() == 11:23 PM
Date and Time
- DateTime
- Time Delta
- Calendar
datetime
from datetime import date
from datetime import time
from datetime import datetime
today = date.today()
print(today)
2019-03-04
print(today.day, today.month, today.year)
(4, 3, 2019)
print(today.weekday()) ## returns an integers in the range 0 to 6, where 0 represents Monday and 6 represents Sunday.
0
today = datetime.now()
print(today)
2019-03-04 21:55:56.228000
time = datetime.time(datetime.now())
print(time)
21:56:16.040000
Time Delta
This module is used to perform date and time calculations.
from datetime import date
from datetime import time
from datetime import datetime
from datetime import timedelta
print(timedelta(days=365, hours=5, minutes=1)) # 365 days, 5:01:00
today = datetime.now()
print(today + timedelta(days=60)) # 2019-03-29 18:41:46.720811
print(today - timedelta(days=57)) # 2018-12-02 18:42:36.774421
timedelta对象采用以下参数:天,秒,微秒,毫秒,分钟,小时,周。 要查找过去或将来的日期,只需使用带有当前日期所需差异的加号或减号。
Calendar
日历相关操作并以格式化方式显示
import calendar
c = calendar.TextCalendar(calendar.MONDAY)
st = c.formatmonth(2017, 1, 0,0)
print(st)
hc = calendar.HTMLCalendar(calendar.MONDAY)
st = hc.formatmonth(2017, 1)
print(st)
#Output
January 2017
Mo Tu We Th Fr Sa Su
1
2 3 4 5 6 7 8
9 10 11 12 13 14 15
16 17 18 19 20 21 22
23 24 25 26 27 28 29
30 31
<table border="0" cellpadding="0" cellspacing="0" class="month">
<tr><th colspan="7" class="month">January 2017</th></tr>
<tr><th class="mon">Mon</th><th class="tue">Tue</th><th class="wed">Wed</th><th class="thu">Thu</th><th class="fri">Fri</th><th class="sat">Sat</th><th class="sun">Sun</th></tr>
<tr><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="sun">1</td></tr>
<tr><td class="mon">2</td><td class="tue">3</td><td class="wed">4</td><td class="thu">5</td><td class="fri">6</td><td class="sat">7</td><td class="sun">8</td></tr>
<tr><td class="mon">9</td><td class="tue">10</td><td class="wed">11</td><td class="thu">12</td><td class="fri">13</td><td class="sat">14</td><td class="sun">15</td></tr>
<tr><td class="mon">16</td><td class="tue">17</td><td class="wed">18</td><td class="thu">19</td><td class="fri">20</td><td class="sat">21</td><td class="sun">22</td></tr>
<tr><td class="mon">23</td><td class="tue">24</td><td class="wed">25</td><td class="thu">26</td><td class="fri">27</td><td class="sat">28</td><td class="sun">29</td></tr>
<tr><td class="mon">30</td><td class="tue">31</td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td><td class="noday"> </td></tr>
</table>
.HTMLCalendar以表格的形式返回HTML代码。Calendar.SUNDAY表示格式化日历中的第一天是星期日,在上边的例子中,使用了Calendar.MONDAY。因此,我们可以在输出中看到表示中的第一天是星期一。
Iterating through dates of a month
import calendar
c = calendar.TextCalendar(calendar.MONDAY)
for i in c.itermonthdays(2017, 8):
print(i, end = )
print( )
for name in calendar.month_name:
print(name, end = )
print( )
for day in calendar.day_name:
print(day, end = )
Output:
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 0 0 0
January February March April May June July August September October November December
Monday Tuesday Wednesday Thursday Friday Saturday Sunday
Regular Expressions
非特殊字符匹配
Regular Expression Examples
Web Data Handling
- Make a Request
- Read
- Get Code
- HTML Parsing
- JSON Parsing
- XML Parsing
Make a Request
import urllib.request ## urllib module is used to send request and receive response from a server. It can used to get html / JSON / XML data from an api.
webData = request.urlopen(“http://www.google.com”) ## It opens a connection to google.com and returns an object of class http.client.HTTPResponse
Read
.read()返回网页的HTML数据。
Get Code
.getcode() 返回连接建立的状态代码。
HTML Parsing
from html.parser import HTMLParser
class MyHTMLParser(HTMLParser):
def error(self, message):
pass
parser = MyHTMLParser()
f = open(“check.html”)
if f.mode == r : # file successfully opened
contents = f.read()
parser.feed(contents)
JSON Parsing
import json
json_data = json.loads(response)
XML Parsing
import xml.dom.minidom
doc = minidom.parse(“check.xml”)
Asyncio
- Definition
- asyncio.run
- Socket with Asyncio
- Move File
- Readline
asyncio是Python 3.5中包含的一个库,采用非常非常明确的异步编程方法:只有标记为async的方法编写的代码才能以异步方式调用任何代码。
asyncio.run
Python 3.7中的新功能
现在能在网上找到很多很多的学习资源,有免费的也有收费的,当我拿到1套比较全的学习资源之前,我并没着急去看第1节,我而是去审视这套资源是否值得学习,有时候也会去问一些学长的意见,如果可以之后,我会对这套学习资源做1个学习计划,我的学习计划主要包括规划图和学习进度表。
分享给大家这份我薅到的免费视频资料,质量还不错,大家可以跟着学习
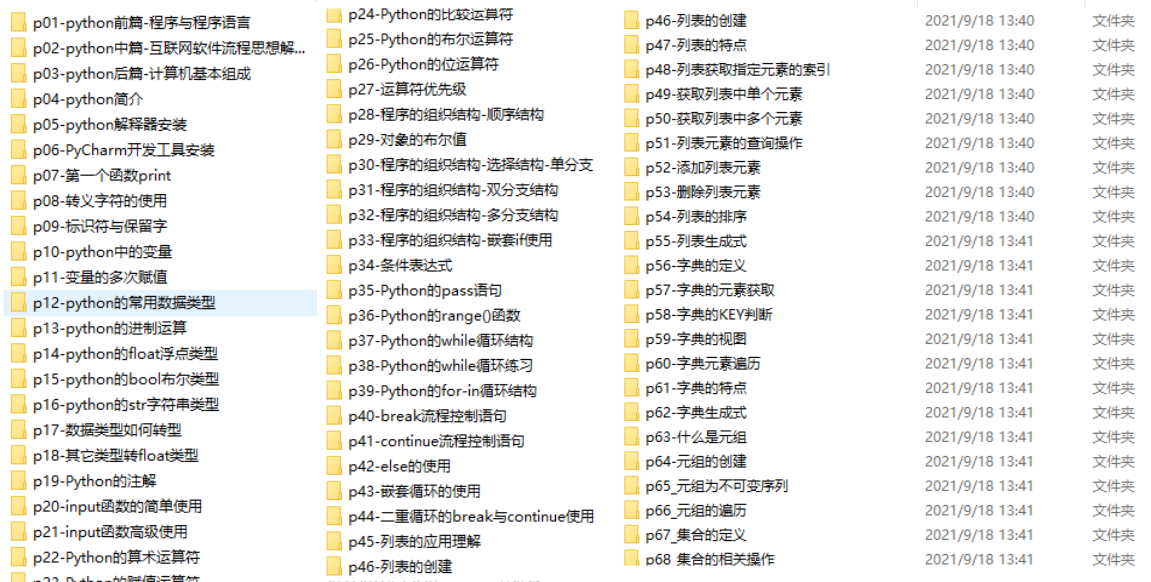
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**