data: {},
methods: {},
router: router // 3、在vue实例中注册路由对象
}); </script>
[](
)router-link 使用
=================================================================================
作用:在切换路由时可以自动给路由路径加入`#`不需要手动加入。
使用 **a标签** 切换路由: 需要在路径前面加 `#`;
使用 **router-link** 切换路由:
* `to`属性书写路由路径;`tag`属性将 router-link 渲染成指定的标签;
我要登录
点我注册
[](
)默认路由
=======================================================================
作用:用来在第一次进入界面是显示一个默认的组件;
const router = new VueRouter({
routes: [
// {path: "/", component: login},
{path: "/", redirect:"/login"}, // redirect:当访问的是默认路由"/"时, 跳转到指定的路由展示[推荐]
{path: "/login", component: login},
{path: "/register", component: register}
]
});
[](
)路由中参数的传递
===========================================================================
[](
)传统方式传递参数
---------------------------------------------------------------------------
1. URL 中通过 `?` 拼接参数:
我要登陆
2. 在组件中获取参数:通过 `this.$route.query.xxx` 来获取参数;
const login = {
template: "<h1>用户登录</h1>",
data() {return{}},
methods: {},
created() {
console.log("name=" + this.$route.query.name + ", pwd=" + this.$route.query.pwd)
}
};
[](
)restful 方式传递参数
---------------------------------------------------------------------------------
1. 通过使用路径方式传递参数:
const router = new VueRouter({
routes: [
{path: "/register/:name/:pwd", component: register}
]
});
<router-link to="/register/zhenyu/12345" tag="a">我要注册</router-link>
```
2. 在组件中获取参数:通过 `this.$route.params.xxx` 来获取参数;
```
const register = {
template: "<h1>用户注册</h1>",
data() {return{}},
methods: {},
created() {
console.log("name=" + this.$route.params.name + ", pwd=" + this.$route.params.pwd);
}
};
```
[](
)完整示例
-----------------------------------------------------------------------
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>路由中传递参数</title>
</head>
<body>
<div id="app">
<router-view></router-view>
<router-link to="/login?name=zhenyu&pwd=12345" tag="a">我要登陆</router-link>
<router-link to="/register/zhenyu/12345" tag="a">我要注册</router-link>
</div>
</body>
</html>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://unpkg.com/vue-router@3.3.4/dist/vue-router.js"></script>
<script> const login = {
template: "<h1>用户登录 {{this.$route.query.name}}</h1>",
data() {return{}},
methods: {},
created() {
console.log("name=" + this.$route.query.name + ", pwd=" + this.$route.query.pwd);
}
};
const register = {
template: "<h1>用户注册 {{this.$route.params.name}} </h1>",
data() {return{}},
methods: {},
created() {
console.log("name=" + this.$route.params.name + ", pwd=" + this.$route.params.pwd);
}
};
const router = new VueRouter({
routes: [
{path: "/", redirect: "/login"},
{path: "/login", component: login},
{path: "/register/:name/:pwd", component: register}
]
});
const app = new Vue({
el: "#app",
data: {},
methods: {},
router // 注册路由
}); </script>
```
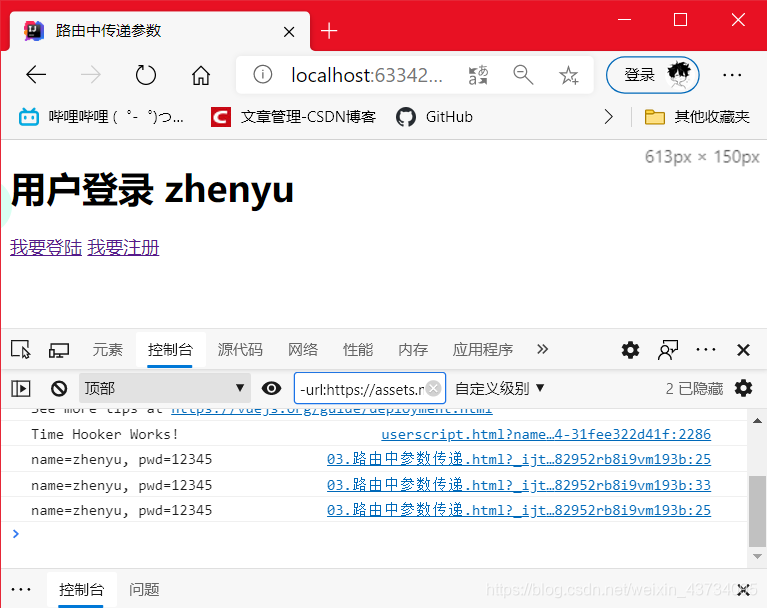
[](
)嵌套路由
=======================================================================
1. 声明最外层和内层组件对象;
2. 创建含有路由对象的路由对象(嵌套路由),通过 `chidren` 嵌套;
3. 注册与使用路由;
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>路由中传递参数</title>
</head>
<body>
<div id="app">
<router-link to="/product">商品管理</router-link>
<router-view></router-view>
</div>
<template id="product">
<div>
<h1>商品管理</h1>
<router-link to="/product/add">商品添加</router-link>
<router-link to="/product/edit">商品编辑</router-link>
<router-view></router-view>
</div>
</template>
</body>
</html>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://unpkg.com/vue-router@3.3.4/dist/vue-router.js"></script>
<script> // 声明最外层和内层组件对象
const product = {
template: '#product'
};
const add = {
template: "<h4>商品添加</h4>"
};
const edit = {
template: "<h4>商品编辑</h4>"
};
// 创建含有路由对象的路由对象(嵌套路由), 通过children嵌套
const router = new VueRouter({
routes: [
{
path: "/product",
component: product,
children: [
{path: "add", component: add},
{path: "edit", component: edit},
]
},
]
});
const app = new Vue({
el: "#app",
data: {},
methods: {},
router // 注册路由
}); </script>
```
[](
)路由结合 SpringBoot 案例
=====================================================================================
[](
)后台控制器
------------------------------------------------------------------------
这是一个简单的演示性的小项目,后台控制器返回一串 Json 字符串。
```
@RestController
@RequestMapping("user")
@CrossOrigin
public class UserController {
@GetMapping("findAll")
public List<User> findAll() {
List<User> list = Arrays.asList(
new User("21", "zhenyu", 21, new Date()),
new User("22", "小三", 24, new Date()),
new User("23", "小明", 25, new Date())