1 String练习
ascii
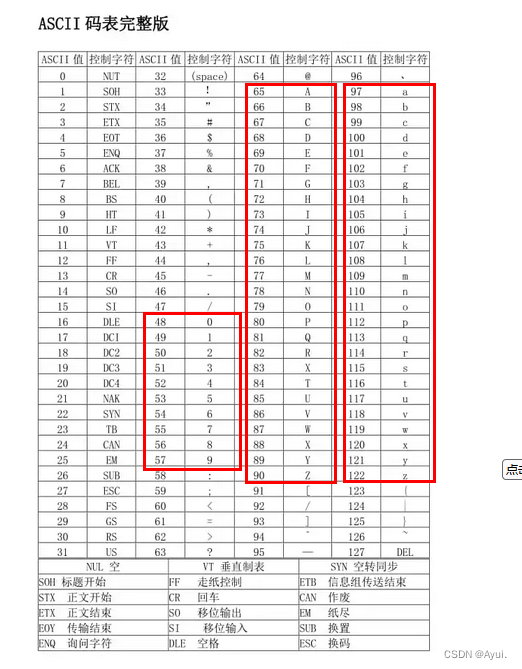
*1: 写一个方法判断注册的账号和密码是否合法:账号:由数字 字母组成 不能以数字开头 长度>=6
* 密码:由数字 字母 _ $ 组成 长度>=8 必须包含数字 字母 和特殊字符(_或者$)
*2: 写一个方法对字符串进行敏感词过滤: 我叫特朗普 我要进行暴力色情恐怖活动:::特朗普 暴力 色情 恐怖 为敏感词 被*替换
*3: 写一个方法对参数字符串反转
*4:写一个方法获取参数字符串中所有但不重复的字符:123abc123abcd---123abcd
*5: 写一个方法打印参数字符串中每个字符出现的次数
*6: 写一个方法获取参数字符串中所有数字字符对应的最大整数:abc109yh7---9710
*7: 写一个方法 去除叠词 :aaabccccb11122276---abcb1276
*8: 写一个方法 对字符串进行转换:我...我....叫....叫....叫....张....张....张....张....张....张....三....三....三....丰---->我叫张三丰
package day09_basic_class;
public class ZuoYe03 {
private static boolean pdName(String name) {
if(name.length()<6) {
return false;
}
for (int i = 0; i <name.length(); i++) {
char c=name.charAt(i);
if(i==0) {
if(c>=0&&c<=9) {
return false;
}
}
if(!(c>='0'&&c<='9')&&!(c>='A'&&c<='Z')&&!(c>='a'&&c<='z')) {
return false;
}
}
return true;
}
private static boolean pdPwd(String pwd) {
if(pwd.length()<8) {
return false;
}
boolean b_=false;
boolean ba=false;
boolean b1=false;
char[] arr=pwd.toCharArray();
for (int i = 0; i <arr.length; i++) {
char c=arr[i];
if(c=='_'||c=='$') {
b_=true;
}
if(c>='0'&&c<='9') {
b1=true;
}
if((c>='a'&&c<='z')||(c>='A'&&c<='Z')) {
ba=true;
}
if(!(c>='0'&&c<='9')&&!(c>='A'&&c<='Z')&&!(c>='a'&&c<='z')&&c!='_'&&c!='$') {
return false;
}
}
return b1&&ba&&b_;
}
public static boolean regist(String name,String pwd) {
return pdName(name)&&pdPwd(pwd);
}
public static String test02(String str) {
String newStr=str;
String[] arr= {"特朗普","暴力","恐怖","枪","色情"};
for (int i = 0; i < arr.length; i++) {
newStr=newStr.replace(arr[i],getXing(arr[i]));
}
System.out.println(str+"::::"+newStr);
return newStr;
}
private static String getXing(String str) {
String xing="";
for (int i = 0; i < str.length(); i++) {
xing+="*";
}
return xing;
}
public static String reverse(String str) {
String strNew="";
for (int i = 0; i < str.length(); i++) {
strNew=str.charAt(i)+strNew;
}
System.out.println(str+"::::"+strNew);
return strNew;
}
public static String test04(String str) {
String newStr="";
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
if(!newStr.contains(c+"")) {
newStr+=c;
}
}
System.out.println(str+"::::"+newStr);
return newStr;
}
public static void test051(String str) {
String ss=test04(str);
for (int i = 0; i < ss.length(); i++) {
char c=ss.charAt(i);
int ciShu=0;
for (int j = 0; j < str.length(); j++) {
char cc=str.charAt(j);
ciShu+=cc==c?1:0;
}
System.out.println(str+"中字符 "+c+" 出现的次数是"+ciShu);
}
}
public static void test052(String str) {
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
boolean b=false;
for (int j = 0; j < i; j++) {
char cc=str.charAt(j);
if(cc==c) {
b=true;
break;
}
}
if(b) {
continue;
}
int ciShu=1;
for (int j = i+1; j < str.length(); j++) {
char cc=str.charAt(j);
if(cc==c) {
ciShu++;
}
}
System.out.println(str+"中字符:::: "+c+" 出现的次数是"+ciShu);
}
}
public static int test06(String str) {
String ss="";
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
if(c>='0'&&c<='9') {
ss+=c;
}
}
char[] arr=ss.toCharArray();
for (int i = 0; i < arr.length-1; i++) {
for (int j = i+1; j < arr.length; j++) {
if(arr[i]<arr[j]) {
char k=arr[i];arr[i]=arr[j];arr[j]=k;
}
}
}
int n=0;
for (int i = arr.length-1,k=1; i >=0; i--,k*=10) {
n+=(arr[i]-'0')*k;
}
System.out.println(str+"::::"+ss+"::::"+n);
return n;
}
public static String test07(String str) {
String ss="";
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
if(i==0) {
ss+=c;
}else {
char cc=str.charAt(i-1);
if(cc!=c) {
ss+=c;
}
}
}
System.out.println(str+"::::"+ss);
return ss;
}
public static String test08(String str) {
String ss="";
ss=str.replace(".", "");
System.out.println(str+"::::"+ss);
ss=test07(ss);
System.out.println(str+"::::"+ss);
return ss;
}
public static void main(String[] args) {
test02("我叫特朗普 我要进行暴力色情恐怖活动");
reverse("abc12344");
test04("123abc123kkk");
test051("11133454566767sbsdbsdb");
test052("11133454566767sbsdbsdb");
test06("abc198aksj0861");
test07("abaaabbb111shshshdddd");
test08("我...我....叫....叫....叫....张....张....张....张....张....张....三....三....三....丰");
}
}
2 String的再次练习
public static void main(String[] args) {
test01("aaabbbbcccdabiiiioooo");
test02("a3b4c3dabi4o4");
test03("a3b4c3dabi4o4YYYTWTWTW");
}
public static String test01(String str) {
String ss="";
ss+=str.charAt(0);
int ciShu=1;
for (int i = 1; i < str.length(); i++) {
char c=str.charAt(i);
char cc=str.charAt(i-1);
if(c==cc) {
ciShu++;
}else {
if(ciShu!=1) {
ss+=ciShu;
}
ss+=c;
ciShu=1;
}
}
ss+=ciShu>1?ciShu:"";
System.out.println(str+":::"+ss);
return ss;
}
public static String test02(String str) {
String ss="";
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
if(c>'9'||c<'0') {
ss+=c;
}else {
for (int j = 1; j <=c-'0'-1; j++) {
ss+=str.charAt(i-1);
}
}
}
System.out.println(str+":::"+ss);
return ss;
}
public static String test03(String str) {
String ss="";
for (int i = 0; i < str.length(); i++) {
char c=str.charAt(i);
if(c>='a'&&c<='z'){
ss+=(char)(c-('a'-'A'));
}else if(c>='A'&&c<='Z'){
ss+=(char)(c+('a'-'A'));
}else if(c>'9'||c<'0') {
ss+=c;
}
}
System.out.println(str+":::"+ss);
return ss;
}
package day09_basic_class;
public class LianXi02 {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
System.out.println(new Student02());
}
}
}
class Student02{
private String name;
private int age;
private char sex;
private float score;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public float getScore() {
return score;
}
public void setScore(float score) {
this.score = score;
}
@Override
public String toString() {
return "Student02 [name=" + name + ", age=" + age + ", sex=" + sex + ", score=" + score + "]";
}
public int hashCode() {
return this.name.hashCode();
}
@Override
public boolean equals(Object obj) {
if(obj== null) {return false;}
if(!(obj instanceof Student02)) {return false;}
Student02 s=(Student02)obj;
return s.name.equals(this.name)&&s.age==this.age&&s.sex==this.sex&&s.score==this.score;
}
public Student02(String name, int age, char sex, float score) {
super();
this.name = name;
this.age = age;
this.sex = sex;
this.score = score;
}
public Student02() {
this.name=suiJIName();
this.sex=Math.random()>0.5?'男':'女';
this.score=(int)(Math.random()*1001+0)/10.0f;
this.age=(int)(Math.random()*8+18);
}
public static String suiJIName() {
String name="";
for (int i = 0; i < 4; i++) {
double n=Math.random();
char c;
if(n<10.0/62) {
c=(char)(Math.random()*10+'0');
}else if(n<36.0/62) {
c=(char)(Math.random()*26+'A');
}else {
c=(char)(Math.random()*26+'a');
}
name+=c;
}
return name;
}
}
3 Date
*Date:日期
*注意1: Date类中的大部分方法 都是过时的:::(有更前面更安全的替代者 但不影响当前类的使用)
* Date类是完全按美国人对日期的认知进行设计的 信息太少 转化为其他民族描述日期的算法 比较麻烦
* 不易于实现国际化
* 2: 注意导包:我们java开发使用的是java.util.Date
* 3: 日期也可以用毫秒值来记录:毫秒值是相当于历元(1970-1-1 0:0:0)
*构造方法:
* 1:无参数
* Date() :获取的是当前时间
* 2:参数是毫秒值
* Date(long date) :获取的是毫秒值对应的时间
* 3:参数是年月日 时分秒
* 年-1900 月-1
* Date(int year, int month, int date, int hrs, int min, int sec)
* Date(int year, int month, int date)
*普通方法:
* 1 比较
* boolean after(Date when)
* boolean before(Date when)
* 2 设置和获取时间参数
* int getXxx()
* void setXxx(int xxx)
* 3 Date与毫秒值之间的转换
* long getTime();获取当前时间对象对应的毫秒值
* void setTime(long time); 设置当前时间对象表示的时间为参数毫秒值对应的时间
* 4 String toLocaleString():获取当前操作系统的日期表示格式
package day09_basic_class;
import java.util.Date;
public class Demo06Date {
public static void main(String[] args) {
Date d1=new Date();
System.out.println(d1);
d1=new Date(1);
System.out.println(d1);
d1=new Date(2022-1900, 9-1, 8, 11, 49, 0);
System.out.println(d1);
Date d2=new Date(0);
System.out.println(d2);
System.out.println(d1.after(d2));
d2.setYear(2022-1900);
d2.setMonth(9-1);
d2.setDate(8);
System.out.println(d2);
System.out.println(date2Str(new Date()));
d2=new Date(2000-1900,1-1,1);
System.out.println(date2Str(d2));
System.out.println(d2.getTime()/1000/3600/24/365);
d2.setTime(0);
System.out.println(date2Str(d2));
System.out.println(d2.toLocaleString());
}
public static String date2Str(Date d) {
String weeks="日一二三四五六";
int year=d.getYear()+1900;
int month=d.getMonth()+1;
int date=d.getDate();
int hour=d.getHours();
int minute=d.getMinutes();
int second=d.getSeconds();
int week=d.getDay();
return year+"年"+month+"月"+date+"日 星期"+weeks.charAt(week)+" "+hour+":"+minute+":"+second;
}
}
4 Date练习
package day09_basic_class;
import java.util.Date;
public class LianXi03 {
public static void main(String[] args) {
System.out.println(changeStr2Int("12345"));
get2("2000-1-1");
}
public static int get1(String str) {
String yearStr=str.substring(0, str.indexOf("-"));
String monthStr=str.substring(str.indexOf("-")+1, str.lastIndexOf('-'));
String dayStr=str.substring(str.lastIndexOf('-')+1);
int year=changeStr2Int(yearStr);
int month=changeStr2Int(monthStr);
int day=changeStr2Int(dayStr);
Date date1=new Date(year-1900, month-1, day);
Date date2=new Date();
int days=(int)(Math.abs(date1.getTime()-date2.getTime())/1000/3600/24);
return days;
}
private static int changeStr2Int(String str) {
int n=0;
for (int i = str.length()-1,k=1; i >=0; i--,k*=10) {
char c=str.charAt(i);
n+=(c-'0')*k;
}
return n;
}
public static Date get2(String str) {
String yearStr=str.substring(0, str.indexOf("-"));
String monthStr=str.substring(str.indexOf("-")+1, str.lastIndexOf('-'));
String dayStr=str.substring(str.lastIndexOf('-')+1);
int year=changeStr2Int(yearStr);
int month=changeStr2Int(monthStr);
int day=changeStr2Int(dayStr);
Date date1=new Date(year-1900, month-1, day+100);
System.out.println(date1.toLocaleString());
return date1;
}
}
5 Calendar
* Calendar:日历:
* 注意事项1:Calendar类是抽象类:通过其静态方法static Calendar getInstance() 获取其子类对象
* 2: Calendar中包含了所有和时间相关的参数 来实现国际化
*
* 普通方法:
* 1 比较
* boolean after(Object when)
boolean before(Object when)
2 设置和获取指定的时间参数
int get(int field)
void set(int field, int value)
3 在指定参数字段 添加指定值
void add(int field, int amount)
4 calendar与date之间的转换
Date getTime() ;获取当前日历对象对应的相同时间的日期对象
void setTime(Date date);吧当前日历对象的时间设置为参数日期对象表示的时间
5 calendar与毫秒值之间的转换
long getTimeInMillis() ;获取当前日历对象表示的时间对应的毫秒值
void setTimeInMillis(long time) ;设置当前日历对象表示的时间为参数毫秒值对应的时间
public static void main(String[] args) {
Calendar c1=Calendar.getInstance();
System.out.println(c1);
System.out.println(c1.get(Calendar.YEAR));
System.out.println(c1.get(Calendar.MONTH)+1);
System.out.println(c1.get(Calendar.DAY_OF_MONTH));
System.out.println(calendar2Str(c1));
c1.add(Calendar.DAY_OF_MONTH, 1);
System.out.println(calendar2Str(c1));
Date d1=new Date(0);
System.out.println(calendar2Str(c1));
System.out.println(d1.toLocaleString());
System.out.println(c1.getTimeInMillis());
System.out.println(System.currentTimeMillis());
c1.setTimeInMillis(0);
System.out.println(calendar2Str(c1));
}
public static String calendar2Str(Calendar c) {
String weeks=" 日一二三四五六";
int year=c.get(Calendar.YEAR);
int month=c.get(Calendar.MONTH)+1;
int day=c.get(Calendar.DAY_OF_MONTH);
int week=c.get(Calendar.DAY_OF_WEEK);
int hour=c.get(Calendar.HOUR_OF_DAY);
int minute=c.get(Calendar.MINUTE);
int second=c.get(Calendar.SECOND);
return year+"年"+month+"月"+day+"日 星期"+(weeks.charAt(week))+" "+hour+":"+minute+":"+second;
}