前言
关于模糊查询搜索并高亮显示结果,大多都用es分布式搜索引擎进行实现,但整合es的过程比较复杂,像创建索引库、导入文档数据、同步更新DB数据,各种请求Request应接不暇。因此,在此直接用了Mybatis-Plus进行实现,并对搜索结果进行了高亮处理,虽然MP模糊搜索性能很差,但平常编码测试自己玩玩还是可以的。
具体实现
1.数据表
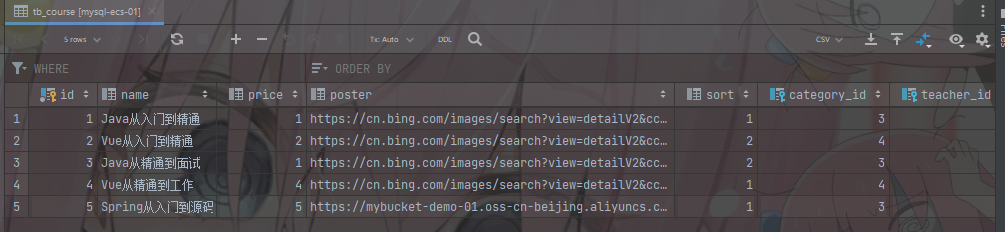
2.Controller层
@RestController
@RequestMapping("/course")
@Api(description = "课程接口")
public class CourseController {
@Autowired
private CourseService courseService;
@ApiOperation("分页模糊查询所有匹配课程,并高亮显示结果")
@PostMapping("getAll/course/highlighter/{currentPage}/{pageSize}/{name}")
public Result getAllCourseHighLighter(@PathVariable Integer currentPage,
@PathVariable Integer pageSize,
@PathVariable String name) {
return courseService.getAllCourseHighLighter(currentPage, pageSize, name);
}
}
3.Service层
@Slf4j
@Service
public class CourseServiceImpl extends ServiceImpl<CourseMapper, Course> implements CourseService {
@Override
public Result getAllCourseHighLighter(Integer currentPage, Integer pageSize, String name) {
IPage<Course> page = new Page<>(currentPage, pageSize);
//可用MP方便地添加一系列过滤、排序条件
lambdaQuery().like(Course::getName, name).page(page);
long total = page.getTotal();
List<Course> courseList = page.getRecords().stream().map(o -> {
String originName = o.getName();
//替换模糊查询字段值 -> 如果前端是Vue的话直接用v-html="name"展示即可高亮显示
String newName = originName.replaceAll(name, "<span style='color: rgb(246, 93, 142)'>" + name + "</span>");
o.setName(newName);
return o;
}).collect(Collectors.toList());
return Result.ok().data(MapUtil.builder()
.put("total", total)
.put("courseList", courseList).build()).msg("成功分页、高亮、模糊查询所有课程");
}
}
4.测试结果
{
"flag": true,
"code": 200,
"msg": "成功分页、高亮、模糊查询所有课程",
"data": {
"total": 2,
"courseList": [
{
"id": 1,
//成功为搜索字段加上高亮样式
"name": "<span style='color: rgb(246, 93, 142)'>Java</span>从入门到精通",
"price": 1,
"poster": "https://cn.bing.com/images/search?view=detailV2&ccid=S3ilIbGn&id=C4ABDD41B61EB8FB3575D6ADAF34DC3B283A1F0C&thid=OIP.S3ilIbGnQ2MhpsxIijA3dAHaEK&mediaurl=https%3a%2f%2fpic1.zhimg.com%2fv2-96727fec3160ad7114bd424377a90ee8_r.jpg&exph=1080&expw=1920&q=%e9%ab%98%e6%b8%85%e7%94%b5%e8%84%91%e5%8a%a8%e6%bc%ab%e5%a3%81%e7%ba%b8&simid=607994978101366522&FORM=IRPRST&ck=6F8344C168E5DD4ADE4E923F7DA58E5E&selectedIndex=7",
"categoryId": 3,
"teacherId": 1,
"createTime": "2023-01-09 11:10:02",
"updateTime": "2023-01-09 11:10:02",
"isDeleted": 0,
"sort": 1
},
{
"id": 3,
"name": "<span style='color: rgb(246, 93, 142)'>Java</span>从精通到面试",
"price": 1,
"poster": "https://cn.bing.com/images/search?view=detailV2&ccid=SCg60HrS&id=637D0A1947C6C3698F642AE12F11FC672E24C60C&thid=OIP.SCg60HrSm5lcRm0gcGlDAAHaEK&mediaurl=https%3a%2f%2fpic2.zhimg.com%2fv2-63dad01ff5c14e79d1622dc54865f5ed_r.jpg&exph=1080&expw=1920&q=%e9%ab%98%e6%b8%85%e7%94%b5%e8%84%91%e5%8a%a8%e6%bc%ab%e5%a3%81%e7%ba%b8&simid=608002438460677649&FORM=IRPRST&ck=F0EFE8FF0A26FFB2ECC6AAF24F4BE4EC&selectedIndex=10",
"categoryId": 3,
"teacherId": 1,
"createTime": "2023-01-09 11:11:26",
"updateTime": "2023-01-09 11:11:26",
"isDeleted": 0,
"sort": 2
}
]
}
}
5.前端展示
前端如果是Vue的话直接用v-html=name即可实现高亮展示,这里就不具体做测试了。
总结
Mybatis-Plus真香。