一、request对象
该对象代表了客户端的请求信息,主要用于接收通过HTTP传送到服务器端的数据(包括头信息、系统信息、请求方式以及请求参数等)。
JSP request是Java中实例化了的对象,主要用来获取客户端提交的数据。
request对象的作用域为一次请求。
常用方法:String getParameter(String name)——获取请求参数name的值
获取请求参数值
在一个请求中,可以通过使用“?”的方式来传递参数,然后通过request对象的getParameter()方法来获取参数的值。
eg. String id = request.getParameter("id");
上面的代码使用getParameter()方法从request对象中获取参数id的值,如果request对象中不存在此参数,那么该方法将返回null。
获取Form表单的信息
在一个表单中会有不同的标签元素,对于文本元素、单选按钮、单选下拉列表框而言,都可以使用getParameter()方法来获取其具体的值;但对于复选框以及多选列表框被选定的内容而言,就要使用getParameterValues()方法来获取了,该方法会返回一个字符串数组,通过遍历这个数组就可以得到用户选定的所有内容。
index.jsp页面:用来呈现表单的信息
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="show.jsp" method="post">
<ul style="list-style:none;line-height:30px">
<li>输入用户姓名:<input type="text" name="name"/><br></li>
<li>选择性别:
<input name="sex" type="radio" value="男"/>男
<input name="sex" type="radio" value="女"/>女
</li>
<li>
选择密码提示问题:
<select name="question">
<option value="母亲生日">母亲生日</option>
<option value="宠物名称">宠物名称</option>
<option value="电脑配置">电脑配置</option>
</select>
</li>
<li>请输入问题答案:<input type = "text" name = "key"/></li>
<li>
请选择个人爱好:
<div style="width:400px">
<input name="like" type="checkbox" value="唱歌跳舞"/>唱歌跳舞
<input name="like" type="checkbox" value="上网冲浪"/>上网冲浪
<input name="like" type="checkbox" value="户外登山"/>户外登山
<input name="like" type="checkbox" value="体育运动"/>体育运动<br/>
<input name="like" type="checkbox" value="读书看报"/>读书看报
<input name="like" type="checkbox" value="欣赏电影"/>欣赏电影
</div>
</li>
<li><input type="submit" value="提交"/></li>
</ul>
</form>
</body>
</html>
show.jsp页面:该页面是用来处理请求的,将用户提交的表单信息显示在页面中。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul style="list-style:none;line-height:30px">
<li>输入用户姓名:
<%=new String(request.getParameter("name").getBytes("ISO8859_1"),"UTF-8")%></li>
<li>选择性别:
<%=new String(request.getParameter("sex").getBytes("ISO8859_1"),"UTF-8")%></li>
<li>选择密码提示问题:
<%=new String(request.getParameter("question").getBytes("ISO8859_1"),"UTF-8")%>
</li>
<li>请输入问题答案:
<%=new String(request.getParameter("key").getBytes("ISO8859_1"),"UTF-8")%></li>
<li>
请选择个人爱好:
<%
String[] like=request.getParameterValues("like");
for(int i=0;i<like.length;i++){
%>
<%=new String(like[i].getBytes("ISO8859_1"),"UTF-8")+" " %>
<% }
%>
</li>
</ul>
</body>
</html>
运行结果如下:
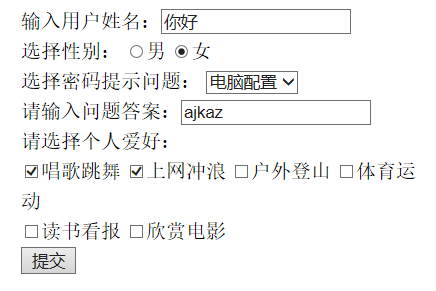
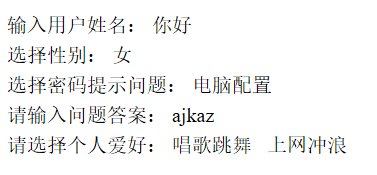
注:想要获得所有的参数名称可以使用getParameterNames()方法,则该方法返回一个Enumeration类型值。
获取请求客户端信息
在request对象中通过相应的方法还可以获取到客户端的相关信息,如HTTP报头文件、客户信息提交方式、客户端主机IP地址、端口号等。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul style = "line-height:24px">
<li>客户使用的协议:<%= request.getProtocol() %>
<li>客服端发送请求的方法:<%= request.getMethod() %>
<li>客户端请求路径:<%= request.getContextPath() %>
<li>客户机IP地址:<%= request.getRemoteAddr() %>
<li>客户机名称:<%= request.getRemoteHost() %>
<li>客户机请求端口号:<%= request.getRemotePort() %>
<li>接收客户信息的页面:<%= request.getServletPath() %>
<li>获取报头中的User-Agent值:<%= request.getHeader("user-agent") %>
<li>获取报头中的accept值:<%= request.getHeader("accept") %>
<li>获取报头中的Host值:<%= request.getHeader("host") %>
<li>获取报头中的accept-encoding值:<%= request.getHeader("accept-encoding") %>
<li>获取URI:<%= request.getRequestURI() %>
<li>获取URL:<%= request.getRequestURL() %>
</ul>
</body>
</html>
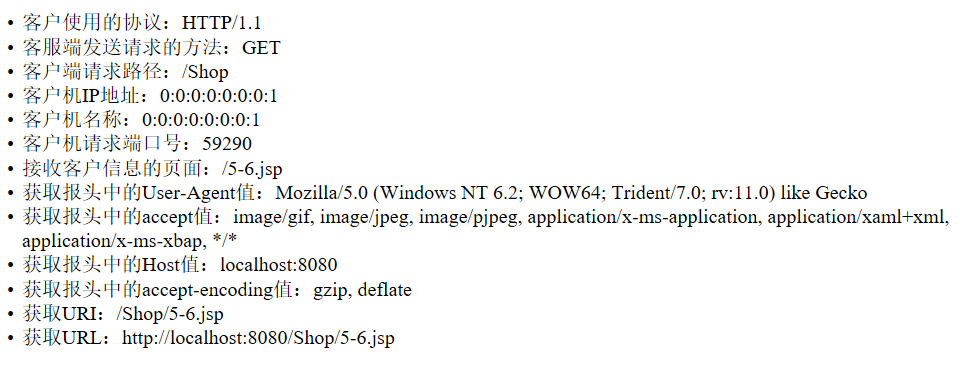
在作用域中管理属性
通过使用setAttribute()方法可以在request对象的属性列表中添加一个属性,然会在request对象的作用域范围内通过removeAttribute()方法将一个属性删除掉。
cookie管理
cookie是小段的文本信息,通过使用cookie可以表示用户身份、记录用户名及密码、跟踪重复用户。cookie在服务器端生成并发送给浏览器,浏览器将cookie的key/value保存到某个指定的目录中,服务器的名称与值可以由服务器端定义。
通过cookie的getCookies()方法可以获取到所有的cookie对象集合,然后通过cookie对象的getName()方法获取到指定名称的cookie,再通过getValue()方法即可获取到cookie对象的值。另外,将一个cookie对象发送到客户端使用了response对象的addCookie()方法。
实例:管理cookie
首先创建2-2.jsp页面文件,在其中创建form表单,用于让用户输入信息;并且从request对象中获取cookie,判断是否含有此服务器发送过的cookie。如果没有,则说明该用户第一次访问本站;如果有,则直接将值读取出来,并赋给对应的表单。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
String welcome = "第一次访问";
String[] info = new String[]{"", "", ""};
Cookie[] cook = request.getCookies(); //得到所有的cookie对象集合
if(cook != null)
{
for(int i = 0; i < cook.length; i ++ )
{
if(cook[i].getName().equals("mrCookInfo")) //获取指定名称的cookie
{
info = cook[i].getValue().split("#");
welcome = ", 欢迎回来!";
}
}
}
%>
<%= info[0] + welcome%>
<form action="2-1.jsp" method="post">
<ul style = "line-height:23px">
<li>姓 名:<input name = "name" type = "text" value = "<%=info[0] %>">
<li>出生日期:<input name = "birthday" type = "text" value = "<%=info[1]%>">
<li>邮箱地址:<input name = "mail" type = "text" value = "<%=info[2]%>">
<li><input type = "submit" value = "提交">
</ul>
</form>
</body>
</html>
接下来创建2-1.jsp页面文件,在该页面中通过request对象将用户输入的表单信息提取出来;创建一个cookie对象,并通过response对象的addCookie()方法将其发送到客户端。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
String name = request.getParameter("name");
String birthday = request.getParameter("birthday");
String mail = request.getParameter("mail");
Cookie myCook = new Cookie("mrCookInfo", name + "#" + birthday + "#" + mail);
myCook.setMaxAge(60 * 60 * 24 * 365);
response.addCookie(myCook);
%>
表单提交成功
<ul style = "line-height:24px">
<li>姓名:<%= name %>
<li>出生日期:<%= birthday %>
<li>电子邮箱:<%= mail %>
<li><a href = "2-2.jsp">返回</a>
</ul>
</body>
</html>
运行结果:
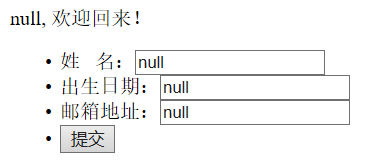
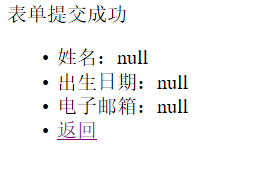
二、response对象
response代表的是对客户端的响应,主要是将JSP容器处理过的对象传回到客户端。response对象也具有作用域,它旨在JSP页面内有效。
常用方法:addCookie(Cookie cookie)——向客户端添加一个cookie对象
1)重定向网页:进行重定向操作后,request中的属性全部失效,并且进入一个新的request对象的作用域。
eg.response.sendRedirect("www.mingribook.com");
2)处理HTTP文件头
setHeader()方法通过两个参数——头名称与参数值的方式来设置HTTP文件头。
response.setHeader("refresh", "5")——设置网页每5秒自动刷新一次
response.setHeader("refresh", "2;URL=welcome.jsp")——设置2秒后自动跳转至指定的页面
3)设置输出缓冲
response.setBufferSize(0);——设置缓冲区大小为0kb,即不缓冲。
还可以使用isCommitted()方法来检测服务器端是否已经把数据写入客户端。
三、session对象
session对象是由服务器自动创建的与用户请求相关的对象。服务器为每个用户都生成一个session对象,用于保存该用户的信息,跟踪用户的操作状态。session对象内部使用Map类来保存数据,因此保存数据的格式为key/value。session对象的value可以是复杂的对象类型,而不仅仅局限于字符串类型。
方法 | 返回值 | 说明 |
getAttribute(String name) | Object | 获得指定名字的属性 |
getAttributeNames() | Enumeration | 获得session中所有属性对象 |
getCreationTime() | long | 获得session对象创建的时间 |
getId() | String | 获得session对象唯一编号 |
1)创建及获取session信息
session对象的setAttribute()方法可实现将信息保存在session范围内,而通过getAttribute()方法可以获取保存在session范围内的信息。
setAttribute(String key, Object obj)
key:保存在session范围内的关键字。
obj:保存在session范围内的对象。
getAttribute(String key)
key:指定保存在session范围内的关键字。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UFF-8">
<title>Insert title here</title>
</head>
<body>
<%
String sessionMessage = "session练习";
session.setAttribute("message", sessionMessage);
out.print("保存在session范围内的对象为:" + sessionMessage);
%>
</body>
</html>

注:session默认在服务器上的存储时间为30分钟,当客户端停止操作30分钟后,session中存储的信息会自动失效。此时调用getAttribute()等方法将出现异常。
2)从会话中移出指定的绑定对象
对于存储在session会话中的对象,如果想将其从session会话中移除,可以使用session对象的removeAttribute()方法。
语法格式:removeAttribute(String key)
3)销毁session
当调用session对象的invalidate()方法后,表示session对象被删除,即不可以再使用session对象。
语法格式:session.invalidate()
如果调用了session对象的invalidate()方法,之后再调用session对象的任何其他方法时,都将报出Session already invalidated异常。
4)session对象的应用
session对象是较常用的内置对象之一,与request对象相比其作用范围更大。
实例:在index.jsp页面中,提供用户输入用户名文本框;在session.jsp页面中,将用户输入的用户名保存在session对象中,用户在该页面中可以添加最喜欢去的地方;在result.jsp页面中,将用户输入的用户名与最想去的地方在页面中显示。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<form id = "form1" name = "form1" method = "post" action = "session.jsp">
<div align = "center">
<table width = "23%" border = "0">
<tr>
<td width = "50%"><div align="center">您的名字是:</div></td>
<td width = "50%">
<label>
<div align="center">
<input type = "text" name = "name"/>
</div>
</label>
</td>
</tr>
<tr>
<td colspan="2">
<label>
<div align = "center">
<input type = "submit" name = "Submit" value = "提交"/>
</div>
</label>
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
String name = request.getParameter("name");//获取用户填写的用户名
session.setAttribute("name", name);//将用户名保存在session对象中
%>
<div align = "center">
<form id = "form1" name = "form1" method = "post" action = "result.jsp">
<table width = "28%" border = "0">
<tr>
<td>您的名字是:</td>
<td><%=name %></td>
</tr>
<tr>
<td>您最喜欢去的地方是:</td>
<td>
<label><input type = "text" name = "address"/></label>
</td>
</tr>
<tr>
<td colspan = "2"><label>
<div align = "center">
<input type = "submit" name = "Submit" value = "提交"/>
</div></label>
</td>
</tr>
</table>
</form>
</div>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
//获取保存在session范围内的对象
String name = (String)session.getAttribute("name");
String solution = request.getParameter("address"); //获取用户输入的最喜欢去的地方
%>
<form id = "form1" name = "form1" method = "post" action = "">
<table width = "28%" boreder = "0">
<tr>
<td colspan = "2"><div align = "center"><strong>显示答案</strong></div></td>
</tr>
<tr>
<td width = "49%"><div align = "left">您的名字是:</div></td>
<td>
<label>
<div align = "left"><%= name %></div>
</label>
</td>
</tr>
<tr>
<td>
<label>
<div align = "left">您最喜欢去的地方是:</div>
</label>
</td>
<td>
<div align = "left"><%=solution %></div>
</td>
</tr>
</table>
</form>
</body>
</html>
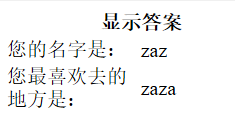
四、application对象
application对象可将信息保存在服务器中,直到服务器关闭,否则application对象中保存的信息会在整个应用中都有效。与session对象相比,application对象的生命周期更长,类似于系统的”全局变量“。
方法 | 返回值 | 说明 |
getAttribute(String name) | Object | 通过关键字返回保存在application对象中的信息 |
getAttributeNames() | Enumeratiion | 获取所有application对象使用的属性名 |
setAttribute(String key, Object obj) | void | 通过指定的名称将一个对象保存在application对象中 |
removeAttribute(String name) | void | 删除application对象中指定名称的属性 |
getRealPath() | String | 返回虚拟路径的真实路径 |
getInitParameter(String name) | String | 获取指定name的application对象属性的初始值 |