目录
题目:使用记事本编写一个HelloWorld程序,并在命令行窗口编译运行,并打印输出结果
第2题:使用冒泡排序,实现对数组{25,24,12,76,101,96,28}的排序
(第三版)题目:使用do…while循环语句计算正数5的阶乘。
第1题 设计一个学生类Student和它的一个子类Undergraduate。
第2题 设计一个Shape接口和它的两个实现类Square和Circle。
第二题 利用Random类产生5个20~30之间的随机整数。
第一题 在HashSet集合中添加三个Person对象,把姓名相同的人当作同一个人,禁止重复添加。
第二题 选择合适的Map集合保存5位学员的学号和姓名,然后按学号的自然顺序的倒序将这些键值对一一打印出来。
第一题 编写一个程序,分别使用字节流和字符流拷贝一个文本文件。
第一章 Java开发入门
题目:使用记事本编写一个HelloWorld程序,并在命令行窗口编译运行,并打印输出结果
public class HelloWorld {
public static void main(String[] arg){
System.out.println("Hello World!");
}
}
第二章 Java编程基础
第1题 实现计算“1+3+5+7+…+99”的值。
(1)、使用循环语句实现自然数1~99的遍历。
(2)、在遍历过程中,通过条件判断当前遍历的数是否为奇数,如果是就累加,否则就不加。
public class Example01 {
public static void main(String[] args) {
int sum = 0;
System.out.println("自然数1-99的遍历结果:");
for (int i = 1; i < 100; i++) {
System.out.print(i + " ");
if (i % 3 == 0) {
sum += i;
}else{
continue;
}
}System.out.println();
System.out.println("奇数累加和:" + sum);
}
}
第2题:使用冒泡排序,实现对数组{25,24,12,76,101,96,28}的排序
import java.util.Arrays;
import java.util.Collections;
public class Example02 {
public static void main(String[] arg) {
Integer[] a = new Integer[] {25,24,12,76,101,96,28};
Arrays.sort(a);
System.out.println("升序排序:");
for (int i =0 ;i<a.length;i++){
System.out.print(a[i] + " ");
}
System.out.println();
System.out.println("降序排序:");
Arrays.sort(a,Collections.reverseOrder()); // 降序排序
for (int arr : a){
System.out.print(arr + " ");
}
}
}
(第三版)题目:使用do…while循环语句计算正数5的阶乘。
public class Example02 {
public static void main(String[] args){
// 计算正数5的阶乘
int num=1,i=1;
do{
i++;
num *= i;
}while(i<5);
System.out.println(num);
}
}
第三章 面向对象(上)
第1题 按照要求设计一个学生类Student。
(1)、Student类包含姓名、成绩两个属性。
(2)、分别给这两个属性定义两个方法,一个方法用于设置值,另一个方法用于获取值。
(3)、Student类中定义一个无参的构造方法何一个接收两个参数的构造方法,然后调用方法给姓名和成绩属性赋值。
(4)、在测试类中创建两个Studen对象,一个使用无参的构造方法,然后调用方法给姓名和成绩赋值,另一个使用有参的构造方法,子啊构造方法中给姓名和成绩赋值。
Student类
public class Student {
private String name; // 姓名
private double score; // 得分
public String getName() { // 获取值
return name;
}
public void setName(String name) { // 设置值
this.name = name;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
public void Person() {
System.out.println("调用的是无参构造方法");
}
public void Person(String name, double score) {
System.out.println("姓名:" + name + " 成绩:" + score);
}
}
测试类
public class Test {
public static void main(String[] args){
Student stu = new Student();
stu.setName("张三");
stu.setScore(98);
stu.Person();
stu.Person("李四",99);
}
}
第2题 定义一个0,1,1,2,3,5,…(斐波那契数列)
使用递归方法获取第n个数的数值。
第一种方法:
List类
public class List {
public void Feibo(int n) {
try {
int[] num = new int[]{0, 1};
if (n < 2) {
System.out.println("斐波那契数列第" + n + "位数为:" + num[n]);
}
}
catch (Exception e){
System.out.println("捕获的异常信息:" + e.getMessage());
}
int first = 0, second = 1, feibo = 0;
for (int i = 2; i <= n; i++) {
feibo = first + second;
first = second;
second = feibo;
}System.out.println("斐波那契数列第" + n + "位数为:" + feibo);
}
}
测试类
public class Test02 {
public static void main(String[] args){
List list = new List();
list.Feibo(2);
}
}
第二种方法:
List类
public class List_01 {
public int Feibo(int n) {
if (n==1){
return 0;
}else if(n==2){
return 1;
}
else{
return Feibo(n-1) + Feibo(n-2);
}
}
}
测试类
public class Test_02 {
public static void main(String[] args){
List_01 feibo = new List_01();
System.out.println(feibo.Feibo(5));
}
}
(第三版)题目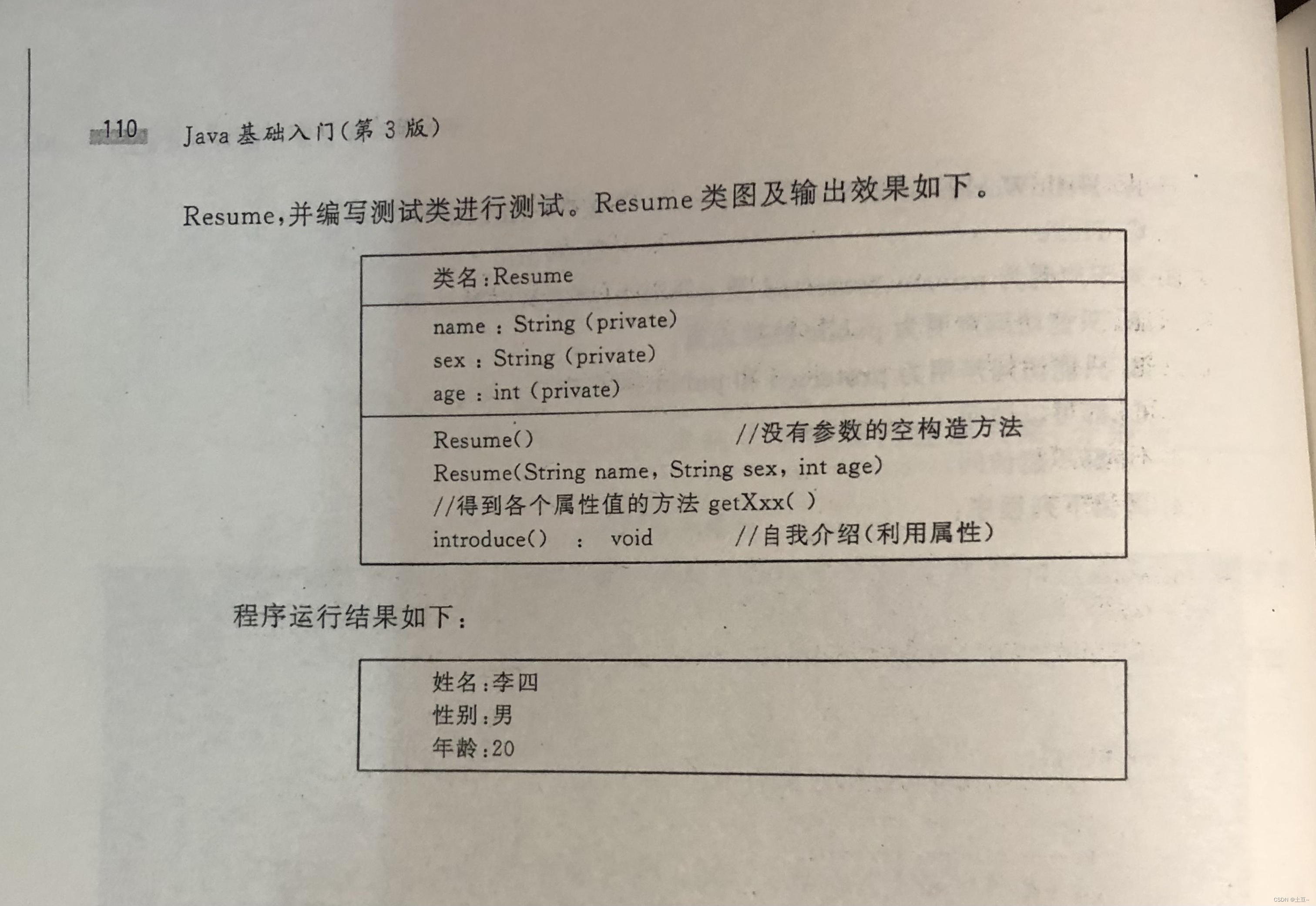
public class Resume {
private String name,sex;
private int age;
protected String getName(){
return name;
}protected void setName(String name){
this.name = name;
}protected String getSex(){
return sex;
}protected void setSex(String sex){
this.sex=sex;
}protected int getAge(){
return age;
}protected void setAge(int age){
this.age = age;
}
public void Resume(){
System.out.println("这是没有参数的构造方法");
}
public void Resume(String name,String sex, int age){
this.name = name;
this.sex = sex;
this.age = age;
}public void intoduce(){
System.out.println("姓名:" + getName() + "\n性别:" + getSex() + "\n年龄:" + getAge());
}
}
测试类
public class Test {
public static void main(String[] args){
Resume resume = new Resume();
// resume.Resume();
resume.Resume("李四","男",20);
resume.intoduce();
}
}
第四章 面向对象(下)
第1题 设计一个学生类Student和它的一个子类Undergraduate。
(1)、Student类有name和age属性,一个包含两个参数的构造方法,用于给name和age属性赋值,一个show()方法打印Student的属性信息。
(2)、本科生类UnderGraduate增加一个degree(学位)的属性。有一个包含三个参数的构造方法,前两个参数哦那与继承的name和age属性赋值,第三个参数给degree专业赋值,一个show()方法用于打印Undergraduate的属性信息。(3)、在测试类中分别创建Student对象和Undergraduate对象,调用它们的show()。
Student类
public class Student {
protected String name;
protected int age;
public String getName(){
return name;
}public void setName(String ame){
this.name = name;
}
public int getAge(){
return age;
}public void setAge(int age){
this.age = age;
}
public void show(){
System.out.println("学生:" + name + " 年龄:" + age);
}
}
Undergraduate类
public class Undergraduate extends Student {
private String degree;
public String getDegree() {
return degree;
}
public void setDegree(String degree) {
this.degree = degree;
}
// 重写父类方法。
public void show(String name, int age, String degree) {
super.name=name;
super.age = age;
super.show();
System.out.println("专业:" + degree);
}
}
测试类
public class Test {
public static void main(String[] args) {
Student student = new Student();
student.setName("张三");
student.setAge(18);
student.show();
Undergraduate stu2 = new Undergraduate();
stu2.show("李四",18,"软件技术");
}
}
第2题 设计一个Shape接口和它的两个实现类Square和Circle。
(1)、Shape接口中有一个抽象方法area(),方法接收有一个double类型的参数,发牛了一个double类型的结果。
(2)、Square和Circle中实现了Shape接口的area()抽象方法,分别求正方形和圆形的面积并返回。
Shape类
public interface Shape {
}
abstract class area{
public abstract double area(double a);
}
Square类
public class Square implements Shape {
area a = new area() {
@Override
public double area(double a) {
return Math.pow(a,2) / 2;
}
};
}
Circle类
public class Circle implements Shape{
area a = new area() {
@Override
public double area(double a) {
return (2*Math.PI*a);
}
};
}
测试类
public class Test {
public static void main(String[] args){
Circle circle = new Circle();
area a1 = circle.a;
System.out.println("圆的面积为;" + a1.area(2));
Square square = new Square();
area a = square.a;
System.out.println("正方形的面积为:" + a.area(+ 5));
}
}
(第三版)题目
父类 Employee
public class Employee {
private String name;
private int month;
public Employee(String name, int month) {
this.name = name;
this.month = month;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public double getSalary(int month){
double salary=0;
if(this.month==month)
salary+=100;
return salary;
}
}
SalariedEmployee子类
public class SalariedEmployee extends Employee {
private double monthSalary;
public SalariedEmployee(String name, int month,double monthSalary) {
super(name,month);
this.monthSalary=monthSalary;
}
public double getMonthSalary() {
return monthSalary;
}
public void setMonthSalary(double monthSalary) {
this.monthSalary = monthSalary;
}
@Override
public double getSalary(int month) {
// TODO Auto-generated method stub
double salary=0;
if(super.getMonth()==month)
salary+=100;
return monthSalary+salary;
}
}
HourlyEmployee子类
public class HourlyEmployee extends Employee {
private double hourlySalary;
private int hours;
public HourlyEmployee(String name,int month,double hourlySalary,int hours){
super(name,month);
this.hourlySalary=hourlySalary;
this.hours=hours;
}
public double getHourSalary() {
return hourlySalary;
}
public void setHourSalary(double hourSalary) {
this.hourlySalary = hourSalary;
}
public int getHours() {
return hours;
}
public void setHours(int hours) {
this.hours = hours;
}
@Override
public double getSalary(int month) {
// TODO Auto-generated method stub
if(hours<=160)
return hours*hourlySalary+super.getSalary(month);
else
return 160*hourlySalary+(hours-160)*hourlySalary*1.5+super.getSalary(month);
}
}
SalesEmployee子类
public class SalesEmployee extends Employee {
private double sales;
private double rate;
public SalesEmployee(String name,int month,double sales,double rate){
super(name, month);
this.sales=sales;
this.rate=rate;
}
public double getSales() {
return sales;
}
public void setSales(double sales) {
this.sales = sales;
}
public double getRate() {
return rate;
}
public void setRate(double rate) {
this.rate = rate;
}
@Override
public double getSalary(int month) {
// TODO Auto-generated method stub
return sales*rate+super.getSalary(month);
}
}
BasePlusSalesEmployee子类
public class BasePlusSalesEmployee extends SalesEmployee {
double baseSalary;
public BasePlusSalesEmployee(String name,int month,double sales,double rate,double baseSalary){
super(name,month,sales,rate);
this.baseSalary=baseSalary;
}
public double getBaseSalary(){
return baseSalary;
}
public void setBaseSalary(double baseSalary) {
this.baseSalary = baseSalary;
}
@Override
public double getSalary(int month) {
// TODO Auto-generated method stub
return getBaseSalary()+super.getSalary(month);
}
}
测试类
public class Test {
public static void main(String[] args) {
// TODO Auto-generated method stub
SalariedEmployee e1=new SalariedEmployee("张三",4,4500);
//System.out.println(e1.getSalary(5));
HourlyEmployee e2=new HourlyEmployee("李四",4,5,220);
//System.out.println(e2.getSalary(5));
SalesEmployee e3=new SalesEmployee("王五", 3,6500, 0.15);
//System.out.println(e3.getSalary(5));
BasePlusSalesEmployee e4 = new BasePlusSalesEmployee("赵六", 5,5000, 0.15, 2000);
//System.out.println(e4.getSalary(5));
Employee[] e=new Employee[4];
e[0] = e1;
e[1] = e2;
e[2] = e3;
e[3] = e4;
double totalSalary=0;
for(int i=0;i<e.length;i++){
totalSalary+=e[i].getSalary(5);
System.out.println(e[i].getName()+","+e[i].getSalary(5));
}
System.out.println("工资总额:"+totalSalary);
}
}
第五章 Java中的常用类
第一题 字符串大小写的转换并倒序输出
(1)、使用for循环将字符串“Hello World”从最后一个字符开始遍历。
(2)、遍历当前字符,如果是大写字符,就使用toLowerCase()方法将其转换为小写字符,反之则使用toUpperCase()方法将其转换为大写字符。
(3)、定义一个StringBuffer对象,调用append()方法依次添加遍历的字符,最后调用StringBuffer对象的toString()方法将其转换为大写字符。
public class Example01 {
public static void main(String[] args) {
StringBuffer say = new StringBuffer();
say.append("Hello World!");
System.out.println("使用SringBuffer逆序输出:" + say.reverse());
/* 课本题目要求如下 */
String word = "Hello World!";
char[] newword = word.toCharArray();
int len = newword.length;
System.out.print("for循环逆序输出:");
for (int i = len - 1; i >= 0; i--) {
System.out.print(newword[i]);
}
/* 大小写转换 */
System.out.println();
System.out.print("大小写转换:");
for (int j = 0; j < newword.length; j++) {
char isword = word.charAt(j);
if (Character.isUpperCase(isword)) {
System.out.print(Character.toLowerCase(isword));
} else if (Character.isLowerCase(isword)) {
System.out.print(Character.toUpperCase(isword));
} else {
System.out.print(isword);
}
}
/* StringBuffer对象遍历添加字符 */
System.out.println();
StringBuffer forString = new StringBuffer();
for (int x = 0; x < newword.length; x++) {
forString.append(newword[x]);
}
System.out.println("toSting()方法调用:" + forString.toString());
}
}
第二题 利用Random类产生5个20~30之间的随机整数。
public class Example02 {
public static void main(String[] args){
Random random = new Random();
System.out.println("生成5个20-30的随机整数:");
for (int i=0;i<5;i++){
System.out.print(random.nextInt(11)+20 + " ");
}
}
}
第六章 集合
第一题 在HashSet集合中添加三个Person对象,把姓名相同的人当作同一个人,禁止重复添加。
Person类中定义name和age属性,重写hashCode()方法和equals()方法,针对Person类的name属性进行比较,如果name相同,hashCode()方法的返回值相同,equals()方法返回true。
public class Person {
private String name;
private int age;
public Person(String name, int age){
this.name = name;
this.age = age;
}
public String toString(){
return name + " " + age;
}
public int hashCode(){
return name.hashCode();
}
public boolean equals(Object obj){
if (this == obj){
return true;
}if (!(obj instanceof Person)){
return false;
}
Person person = (Person) obj;
boolean b = this.name.equals(person.name);
return b;
}
}
测试类
public class Test {
public static void main(String[] args){
HashSet hash = new HashSet();
Person person1 = new Person("张三",18);
Person person2 = new Person("李四",17);
Person person3 = new Person("张三",16);
hash.add(person1);
hash.add(person2);
hash.add(person3);
System.out.println(hash);
}
}
第二题 选择合适的Map集合保存5位学员的学号和姓名,然后按学号的自然顺序的倒序将这些键值对一一打印出来。
(1)、创建TreeMap集合。
(2)、使用put方法将学号("1","2","3","4","5")和姓名("Luncy","Smith","Aimee","Amanda")存储到Map中,存的时候可以打乱顺序观察排序后的效果。
(3)、使用map.keySet()获取键的Set集合。
(4)、使用Set集合的iteration()方法获得Iterator对象用于迭代键。
(5)、使用Map集合的get()方法获取键所对应的值。
public class Example02 {
public static void main(String[] args){
// 1、创建TreeMap集合
TreeMap tree = new TreeMap();
// 2、使用put方法存放数据
tree.put(1,"Lucy");
tree.put(4,"Aimee");
tree.put(2,"John");
tree.put(5,"Amanda");
tree.put(3,"Smith");
// 3、获取键值集合
Set keySet = tree.keySet();
// 4、使用迭代器
Iterator it = keySet.iterator();
while (it.hasNext()){
Object key = it.next();
// 5、使用get获取健对应的值
Object value = tree.get(key);
System.out.println(key + ":" + value);
}
}
}
第七章 I/O流
第一题 编写一个程序,分别使用字节流和字符流拷贝一个文本文件。
(1)、使用FileInputStream、FileOutputStream和FileReader、FileWriter分别进行拷贝。
(2)、使用字节流拷贝时,定义一个1024长度的字节数组作为缓冲区,使用字符流拷贝,使用BufferedReader和BufferedWriter包装流进行包装。
import java.io.*;
import java.nio.charset.StandardCharsets;
public class Example01 {
public static void main(String[] args) throws IOException {
// 1、使用FileInputStream、FileOutputStream、FileReader、FileWriter拷贝文件
FileInputStream in = new FileInputStream("./write.txt");
int i = 0;
System.out.println("字节流获取:");
while ((i=in.read()) != -1){
System.out.print(i + " ");
}in.close();
FileOutputStream out = new FileOutputStream("./write.txt", true);
String str = "生活的意义就在于给亲近的人带来欢乐。\r";
out.write(str.getBytes(StandardCharsets.UTF_8));
out.close();
FileReader fileReader = new FileReader("./write.txt");
System.out.println("\n\nFilerReader获取:");
int len = 0;
while ((len = fileReader.read()) != -1){
System.out.print((char)len + " ");
}fileReader.close();
FileWriter filerWriter = new FileWriter("write.txt",true);
filerWriter.write("关于他们,\r\n");
filerWriter.write("我所能讲述的越少,\r\n");
filerWriter.write("他们于我便越亲近。\r\n");
filerWriter.close();
// 2、定义缓冲区
FileInputStream in_1 = new FileInputStream("./write.txt");
FileOutputStream out_1 = new FileOutputStream("./dest.txt");
int length = 0;
byte[] buff = new byte[1024];
long beginTime = System.currentTimeMillis();
while ((len = in_1.read()) != -1){
out_1.write(buff,0,length);
}long endTime = System.currentTimeMillis();
System.out.println("花费时间为:" + (endTime - beginTime + "毫秒"));
in_1.close();
out_1.close();
}
}