目录
打开test1. c文件,将其内容打印到终端
代码:
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc,char const *a[]){
//打开文件
int fd=open("./test1.c", O_RDWR);
char str[20000]={0};
read(fd,str, sizeof(str));
printf("%s",str);
return 0;
}
test1.c的内容:
运行结果:
改进
代码:
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc,char const *argcv[]){
//打开test1. c文件,将其内容打印到终端
//打开文件
int a=open("./test1.c", O_RDWR);
char str[2000]={0};
if(a){
//读
int b=read(a,str, sizeof(str));
if (b){
printf("%s\n",str);
}else{
//printf("error");
perror("read error!");
return -1;
}
}else{
perror("open error!");
return -1;
}
return 0;
}
运行结果: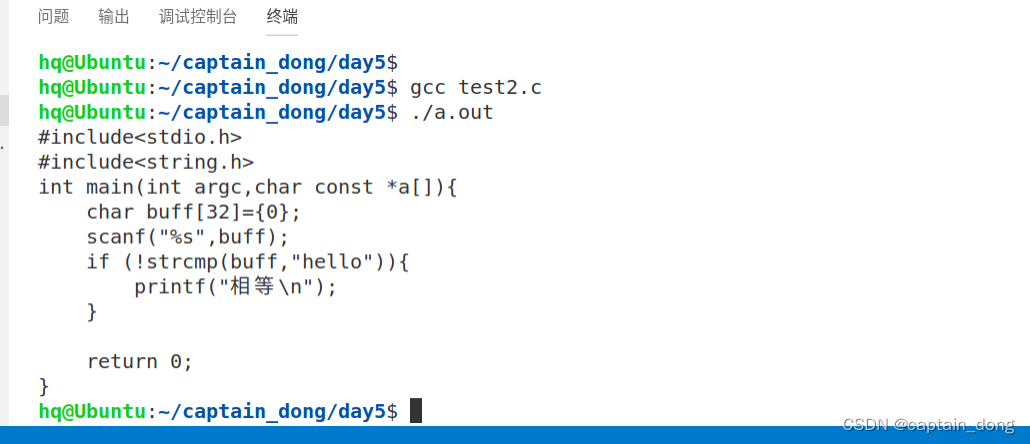
上述程序可以完成打开文件 "test1.c" 并将其内容打印到终端的功能。
具体来说,代码中使用
open()
函数打开文件 "test1.c" 并返回文件描述符a
。如果文件打开成功,代码中接着使用read()
函数读取文件内容并将其存储到str
数组中。最后,使用printf()
函数将str
数组中的内容打印到终端上。需要注意的是,代码中使用了硬编码方式指定了待打开的文件路径为 "./test1.c",这意味着需要保证当前工作目录下确实存在名为 "test1.c" 的文件,否则程序会出错。
另外,代码中还使用了一些系统调用函数,并对它们的返回值进行了简单的错误处理。这是一个好的编程习惯,可以让程序在发生错误时能够及时提示并退出。
改进~改进:
上述代码已经基本实现了打开文件并将其内容打印到终端的功能。以下是一些建议来改进代码:
错误处理:你在代码中使用了简单的错误处理,但是没有处理 open() 和 read() 函数可能返回的更多错误情况。建议在发生错误时给出更具体的错误提示,以便于排查问题。
关闭文件:在代码的最后没有调用 close() 函数关闭打开的文件。为了避免资源泄漏,建议在文件读取完毕后调用 close(a) 来关闭文件描述符。
鲁棒性:代码中没有对文件打开和读取过程中可能发生的异常情况进行处理,如文件不存在、文件权限不足等。建议在打开文件前检查文件是否存在,并在打开和读取过程中处理可能发生的异常。
参数检查:代码中没有对命令行参数进行检查,例如没有检查 argc 的值以确保有足够的参数传入。建议添加必要的参数检查,以提高程序的健壮性。
安全性:代码中使用了固定大小的字符数组 str[2000] 来存储文件内容。如果文件内容超过数组大小,可能会造成缓冲区溢出。建议使用动态内存分配或者循环读取的方式来避免这个问题。
代码:
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 1024
int main(int argc, char const *argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <filename>\n", argv[0]);
return 1;
}
const char* filename = argv[1];
// 打开文件
int fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("Failed to open file");
return 1;
}
// 读取文件内容并打印
char buffer[BUFFER_SIZE];
ssize_t bytesRead;
while ((bytesRead = read(fd, buffer, sizeof(buffer))) > 0) {
if (write(STDOUT_FILENO, buffer, bytesRead) != bytesRead) {
perror("Failed to write to stdout");
close(fd);
return 1;
}
}
if (bytesRead == -1) {
perror("Failed to read file");
close(fd);
return 1;
}
// 关闭文件
if (close(fd) == -1) {
perror("Failed to close file");
return 1;
}
return 0;
}