目录
一、基本数据类型包装类
Java语言是一个面向对象的语言,但是Java中的基本数据类型却是不面向对象的,这在实际使用时存在很多的不便,为了解决这个不足,在设计类时为每个基本数据类型设计了一个对应的类进表示,这样八个和基本数据类型对应的类统称为包装类.
包装类(如:Integer,Double等)这些类封装了一个相应的基本数据类型数值,并为其提供了一系列操作方法。
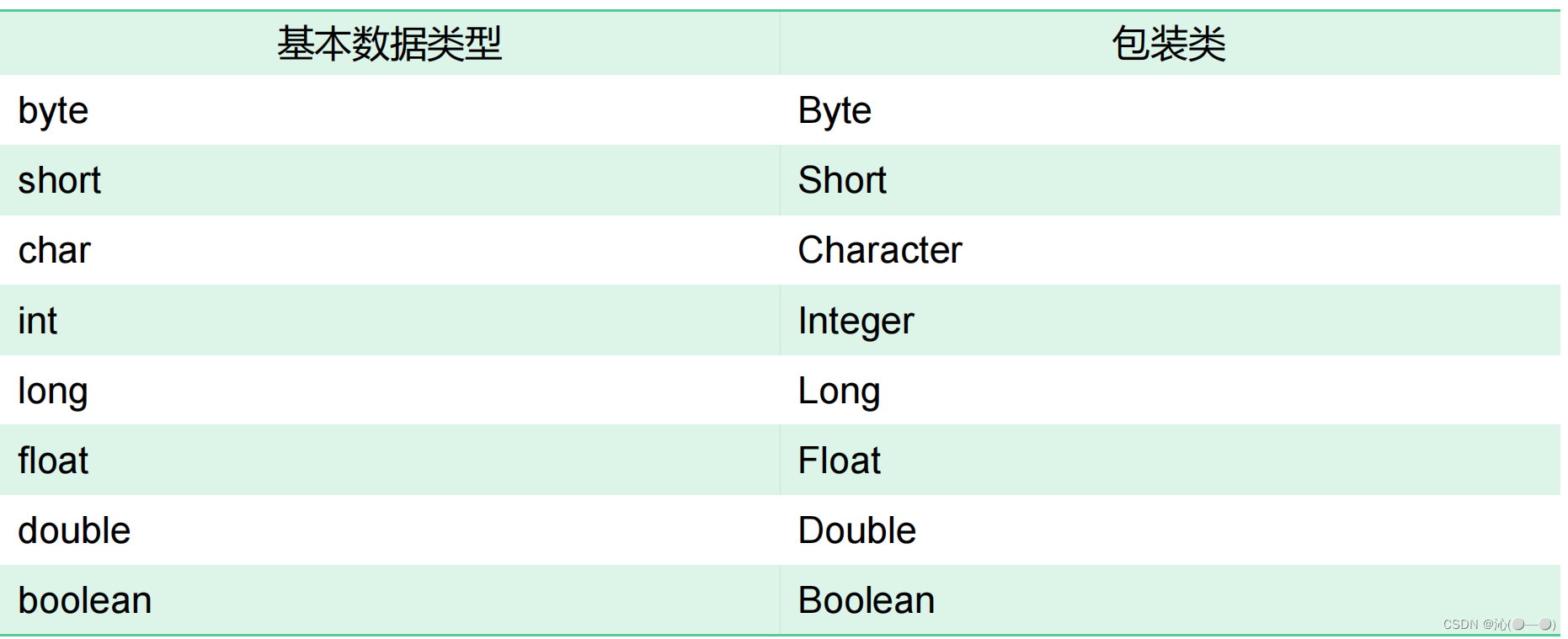
对于包装类来说,这些类的用途主要包含两种:
●
作为和基本数据类型对应的类类型存在。
●
包含每种基本数据类型的相关属性如最大值、最小值等,以及相关的操作方法。
1.1包装类常用的方法
以下方法以
java.lang.Integer
为例
public static final int MAX_VALUE
最大的
int
型数
public static final int MIN_VALUE
最小的
int
型数
构造方法
Integer(int a);
Integer(String a);
比较方法
static int compareTo(Integer a);
boolean equals(Object); 判断两个数的值是否相等
int max(int a,int b); 返回两中的最大值
int min(int a,int b); 返回两中的最小值
转换方法
static toBinaryString(int i); 将数字转换为二进制数
static String toHexString(int i); 将数字转换为十六进制数
static String toOctalString(int i); 将数字转换为八进制数
int intValue(); 输出int型数据
static int parseInt(String s);
String toString(); 输出Integer对象
static Integer valueOf(int i) 将基本数据类Integer包装类型
static Integer valueOf(String s)
public class IntegerDemo1 {
public static void main(String[] args) {
/*
构造方法
用Integer对象来表示int值, 符合面向对象
*/
Integer i1 = new Integer(10);
Integer i2 = new Integer("10");
System.out.println(i1.compareTo(i2));//比较两个包装类型值大小
int i = i1.intValue();//把对象中的int值返回 包装类型 转 基本类型
System.out.println(Integer.MAX_VALUE);
System.out.println(Integer.MIN_VALUE);
System.out.println(Integer.BYTES);
System.out.println(Integer.SIZE);
System.out.println(Integer.toBinaryString(3));//返回整数的二进制数
System.out.println(Integer.max(10,5));//返回两个整数较大的一个
String s = "10";
Integer i3 = new Integer(s);
int i4 = Integer.parseInt(s);
//new Long(10);
}
}
1.2装箱和拆箱
●
装箱
自动将基本数据类型转换为包装器类型 ,装箱的时候自动调用的是Integer的valueOf(int)方法。
●
拆箱
自动将包装器类型转换为基本数据类型 ,拆箱的时候自动调用的是Integer的intValue方法。
基本类型包装类型 和 基本类型之间转换
有时候,使用面向对象的方式操作,就需要把基本类型包装成包装类型(对象)
但有时候,需要使用的是基本数据类型
这时有一个需求,在基本类型包装类型 和 基本类型之间转换之间进行转换
经常需要在基本类型包装类型 和 基本类型之间转换之间进行转换
那么java对这一转换方式进行了简化
自动装箱: 把基本类型转为包装类型, 隐式的使用new 或 valueOf() 其中一个
自动拆箱:把包装类型自动转为基本类型 隐式调用了intvalue()
public class IntegerDemo3 {
public static void main(String[] args) {
int a = 10;
Integer i1 = a; //包装类型是一个对象
Integer i2 = new Integer(10);
int b = i2; //隐式调用了intvalue()
}
}
二、String类
2.1String类概述
字符串是由多个字符组成的一串数据(字符序列)的字符串常量,
java中所有字符串都是String类的实例.
字符串值不变,一旦创建后值就不能改变,因为底层存储字符串的数组是final修饰的
private final char value[]; 每次创建新字符串对象时,为其赋值
一旦要改变字符串的值,那么就必须创建一个新的字符串对象
private final char value[]; 每次创建新字符串对象时,为其赋值
一旦要改变字符串的值,那么就必须创建一个新的字符串对象
public class StringDemo1 {
public static void main(String[] args) {
char c = 'a';
String s = "abc";// "abc" 隐式的创建了一个String类的对象
s += "def";
// s = null;
System.out.println(s);
new String("abcd");
}
}
有两种创建形式:
●
第一种:
String s = "abc";
先在栈中创建一个对String类的对象引用变量s,然后去字符串常量池中查找
有没有"abc", 如果没有,则在常量池中添加”abc”, s引用变量指向常量池中
的”abc”,如果常量池中有,则直接指向改地址即可,不用重新创建.
●
第二种:
一概在堆中创建新对象,值存储在堆内存的对象中。
String s = new String("abc");
public class StringDemo2 {
public static void main(String[] args) {
String s1 = "abc";
String s2 = "abc";
System.out.println(s1==s2);//true
String s3 = new String("abc");
String s4 = new String("abc");
System.out.println(s3==s4);//false
System.out.println(s1.equals(s4));//true
}
}
2.2String类的常用方法
构造方法
public String() 创建一个空的字符串对象
public String(String str) 创建一个字符串对象
public String(byte[] bytes) 将byte[]数组转化成字符串
public String(char[] value) 将char[]数组转化成字符串
getBytes(); 将字符串用默认的编码转为byte数组
getBytes("GBK"); 将字符串用指定的编码转为byte数组
toCharArray(); 将字符串转为字符数组
getBytes("GBK"); 将字符串用指定的编码转为byte数组
toCharArray(); 将字符串转为字符数组
public class StringDemo3 {
public static void main(String[] args) throws UnsupportedEncodingException {
String s = new String();//无参构造 里面是一个 ""
String s1 = new String("abcd");
byte[] bytes = "你好".getBytes();//传输时,将字符串转为 byte数组(数字化) 编码
String s2 = new String(bytes); //解码
System.out.println(s2);
byte[] bytes = "你好".getBytes("GBK");//传输时,将字符串转为 byte数组(数字化) 编码
String s2 = new String(bytes,"GBK"); //解码
System.out.println(s2);
char [] chars = "bcda".toCharArray();
Arrays.sort(chars);
String s2 = new String(chars);
System.out.println(s2);
String s3 = String.valueOf(chars);
}
}
判断功能
boolean equals(Object obj) 判断两个字符是否相等
boolean equalsIgnoreCase(String str) 判断两个字符是否相等,忽略大小写字母
boolean contains(String str) 判断字符串是否包含指定字符串
boolean isEmpty() 判断字符串是否为空
boolean startsWith(String prefix) 判断字符串是否以指定子串开头
boolean endsWith(String suffix) 判断字符串是否以指定子串结尾
public class StringDemo4 {
public static void main(String[] args) {
String s = "abcdefg";
System.out.println(s.contains("bc"));//true
System.out.println(s.isEmpty());//false
System.out.println(s.startsWith("ac"));//false
System.out.println(s.endsWith("g"));//true
System.out.println("a".compareTo("b"));//比较字符串大小的
}
}
获取功能
int length() 获取字符串 的长度
char charAt(int index) 获取指定索引的字符
int indexOf(String str) 获取指定字符首次出现的索引
int indexOf(String str,int fromIndex) 获取 指定字符首次出现的位置,从指定位置开始
String substring(int start) 从指定位置开始截取字符串,直到结尾
String substring(int start,int end) 截取指定位置的字符串
public class StringDemo5 {
public static void main(String[] args) {
String s = "abcdefgc1";
//012345678
System.out.println(s.length());
System.out.println(s.charAt(2));
System.out.println(s.indexOf("c"));
System.out.println(s.indexOf("c",3));
System.out.println(s.lastIndexOf("c"));
System.out.println(s.lastIndexOf("c",6));
System.out.println(s.indexOf("bc"));//返回第一个字符首次出现的位置
String s1 = s.substring(2);
System.out.println(s);//abcdefgc1
System.out.println(s1);//cdefgc1
String s2 = s.substring(0,4);
System.out.println(s2);
}
}
转换功能
static String valueOf(char[] chs) 把char[]数组转换成字符串
String toLowerCase() 全部转换为小写字母
String toUpperCase() 全部转换为大写字母
String concat(String str) 将字符串与指定字符串连接起来
Stirng[] split(分割符); 将子符串用指定分割符分割开来
public class StringDemo6 {
public static void main(String[] args) {
String s = "abcdEFG";
System.out.println(s.toLowerCase());
System.out.println(s.toUpperCase());
s +="yyyy";
s +=1000; // + 拼接其他类型数据
String s1 = s.concat("xxxxx"); //只能连接字符串
System.out.println(s1);
/* String s2 = "ab:cd:E:G";
String[] strings = s2.split(":");
System.out.println(Arrays.toString(strings));
*/
String users = "account=1000,password=111;account=1000,password=111;account=1000,password=111";
String[] us = users.split(";");
System.out.println(us[0]);
System.out.println(us[1]);
System.out.println(us[2]);
}
}
替换功能
String replace(char old,char new) 使用新的字符将旧的字符代替
String replace(String old,String new) 使用新的字符串将旧的字符串
replaceAll(String regex, String replacement) 使用正则表达式去替换字符串
replaceFirst(String regex, String replacement) 使用正则表达式去匹配第一个目标字符
String trim() 去掉字符串前后的空格