一、登录页
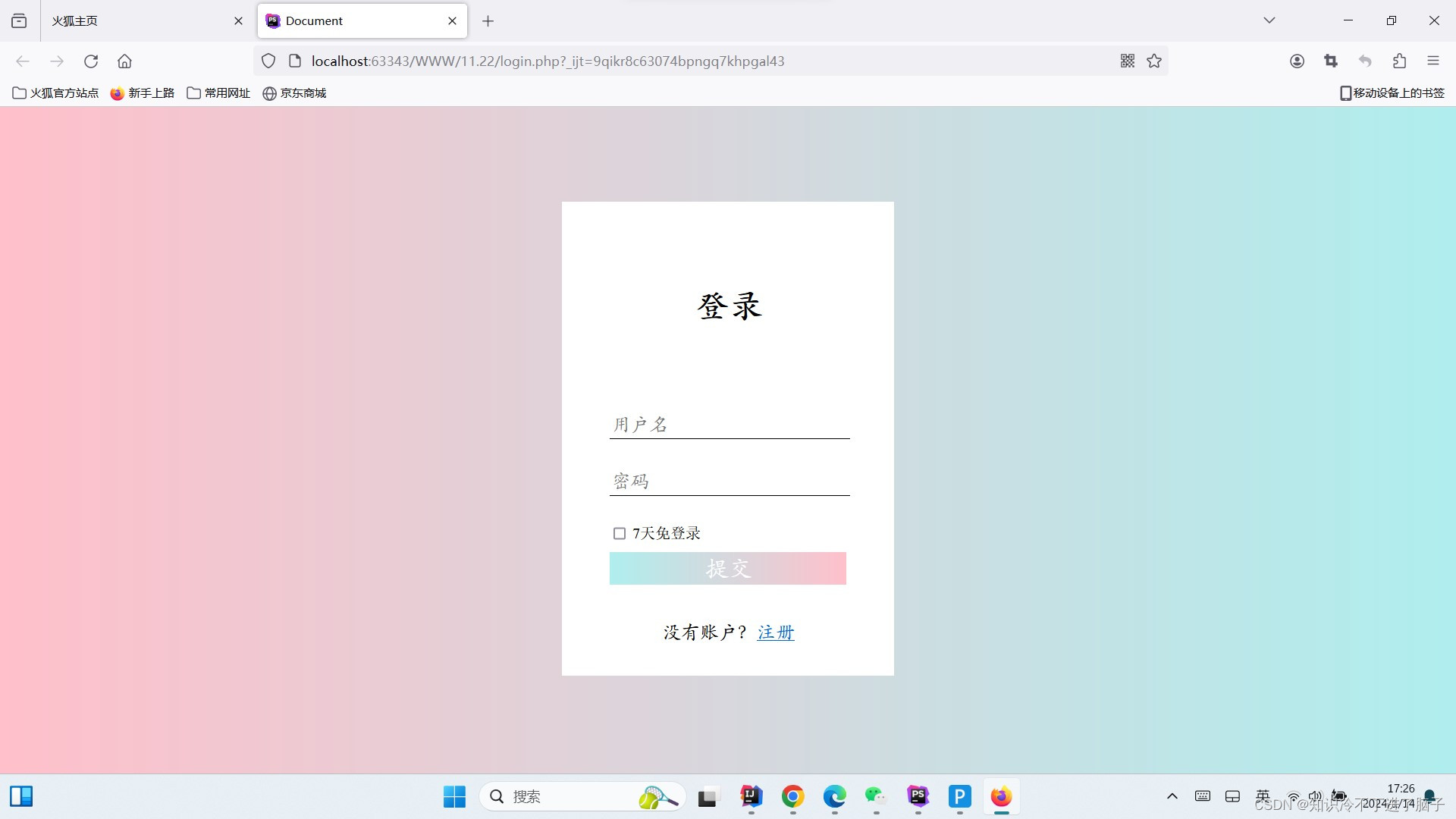
<?php
if (!empty($COOKIE['username'])){
header("location:index.php");
}else{
echo <<<str
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style type="text/css">
body{
padding: 0px;
margin: 0px;
background-image: linear-gradient(to right, pink, paleturquoise);
}
.box{
width:250px;
height: 500px;
background-color: white;
margin: 100px auto;
//设置弧度
border-radius: 15px;
padding: 0 50px;
}
.top{
font-size: 35px;
line-height: 220px;
text-align: center;
font-family: 华文楷体;
font-weight: bolder;
}
.text{
width: 100%;
border: 0px;
border-bottom: 1px solid black;
font-size: 20px;
margin-bottom: 30px;
outline: none;
font-family: 华文楷体;
}
.btn{
width: 100%;
font-size: 25px;
background-image: linear-gradient(to right, paleturquoise,pink);
border: 0;
font-family: 华文楷体;
color: white;
margin-top: 10px;
cursor: pointer;
}
.bottom{
font-size: 20px;
line-height: 100px;
text-align: center;
font-family: 华文楷体;
}
.expire{
font-size: 18px;
cursor: pointer;
font-family: 华文楷体;
}
</style>
<script type="text/javascript">
function sub() {
var user=document.getElementsByName('user')[0].value;
var password=document.getElementsByName('pwd')[0].value;
if (!user){
alert("用户名为空,请重新输入。")
return false;
}
if (!password){
alert("密码不能为空,请重新输入。")
return false;
}
document.getElementById("form").submit();
}
</script>
</head>
<body>
<div class="box">
<div class="top">
登录
</div>
<form action="checklogin.php" id="form" method="post">
<input type="text" name="user" placeholder="用户名" class="text">
<input type="password" name="pwd" placeholder="密码" class="text">
<input type="checkbox" name="expire" id="cookie" value="7" class="expire">
<label for="cookie">7天免登录</label>
<input type="button" value="提交" class="btn" onclick="sub()">
</form>
<div class="bottom">
没有账户?<a href="register.php">注册</a>
</div>
</div>
</body>
</html>
str;
}
?>
二、注册页
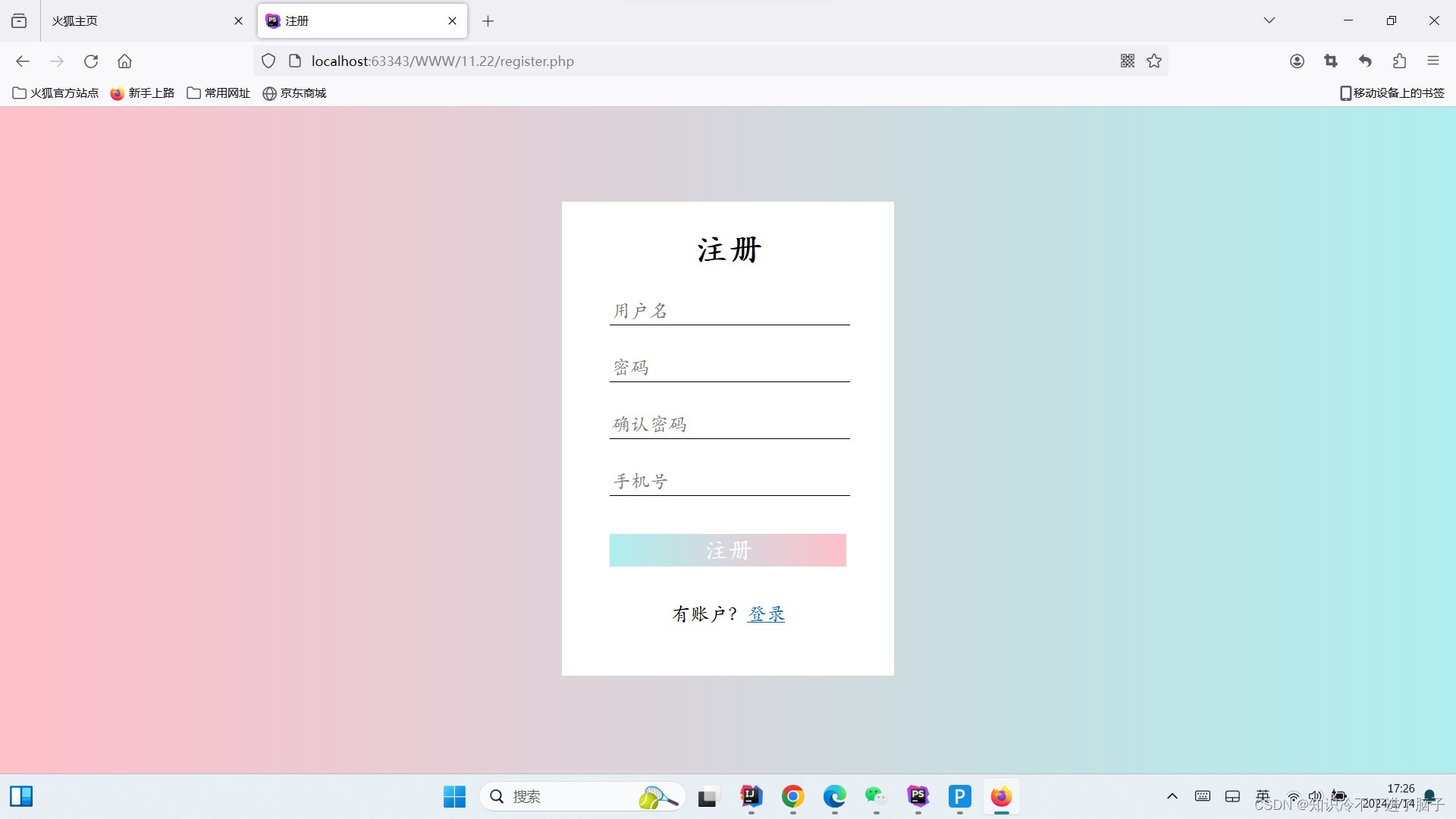
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>注册</title>
<style type="text/css">
body{
padding: 0px;
margin: 0px;
background-image: linear-gradient(to right, pink, paleturquoise);
}
.box{
width:250px;
height: 500px;
background-color: white;
margin: 100px auto;
//设置弧度
border-radius: 15px;
padding: 0 50px;
}
.top{
font-size: 35px;
line-height: 100px;
text-align: center;
font-family: 华文楷体;
font-weight: bolder;
}
.text{
width: 100%;
border: 0px;
border-bottom: 1px solid black;
font-size: 20px;
margin-bottom: 30px;
outline: none;
font-family: 华文楷体;
}
.btn{
width: 100%;
font-size: 25px;
background-image: linear-gradient(to right, paleturquoise,pink);
border: 0;
font-family: 华文楷体;
color: white;
margin-top: 10px;
cursor: pointer;
}
.bottom{
font-size: 20px;
line-height: 100px;
text-align: center;
font-family: 华文楷体;
}
</style>
<script type="text/javascript">
function sub() {
var user=document.getElementsByName('user')[0].value;
var password=document.getElementsByName('pwd')[0].value;
var password1=document.getElementsByName('pwd1')[0].value;
var tel=document.getElementsByName('tel')[0].value;
if (!user){
alert("用户名为空,请重新输入。")
return false;
}
if (!password){
alert("注册密码不能为空,请重新输入。")
return false;
}
if (!password1){
alert("确认密码不能为空,请重新输入。")
return false;
}
if (password1!=password){
alert("确认密码与注册密码不一致,请重新输入")
return false;
}
if (!tel){
alert("请您输入手机号")
return false;
}
if (tel.length!=11){
alert("亲,请输入正确格式的手机号哦")
return false;
}
document.getElementById("form").submit();
}
</script>
</head>
<body>
<div class="box">
<div class="top">
注册
</div>
<form action="doregister.php" id="form" method="post">
<input type="text" name="user" placeholder="用户名" class="text" >
<input type="password" name="pwd" placeholder="密码" class="text">
<input type="password" name="pwd1" placeholder="确认密码" class="text">
<input type="text" name="tel" placeholder="手机号" class="text">
<input type="button" value="注册" class="btn" onclick="sub()">
</form>
<div class="bottom">
有账户?<a href="login.php">登录</a>
</div>
</div>
</body>
</html>
三、注册功能实现
<?php
$conn=mysqli_connect("127.0.0.1","root","root","student2008");
if (mysqli_connect_errno()){
exit(mysqli_connect_error());
}
mysqli_set_charset($conn,'utf-8');
$username=$_POST['user'];
$password=$_POST['pwd'];
$result=mysqli_query($conn,"select * from user where username='".$username."' and password='".$password."'");
$num=mysqli_num_rows($result);
if($num>0){
echo "<script>alert('用户信息已存在');history.back();</script>";
}
$result1=mysqli_query($conn,"insert into user(username,password,tel) values (
'".$_POST['user']."','".$_POST['pwd']."','".$_POST['tel']."')");
if ($result1==true){
echo "<script>alert('注册成功');location.href='login_pwd.php';</script>";
}else{
echo "<script>alert('登录失败');location.href='register.php';</script>";
}
四、判断是否选择了七天免登录功能
<?php
//判断是否选择了七天免登录
$expire=empty($_POST['expire'])?-1:intval($_POST['expire']);
$conn=mysqli_connect("127.0.0.1","root","root","student2008");
if (mysqli_connect_errno()){
exit(mysqli_connect_error());
}
mysqli_set_charset($conn,'utf-8');
$username=$_POST['user'];
$password=$_POST['pwd'];
$result=mysqli_query($conn,"select * from user where username='".$username."' and password='".$password."'");
$num=mysqli_num_rows($result);
if ($num>0){
echo "登录成功";
//登陆成功,设置cookie
setcookie("username",$_POST['user'],time()+(3600*24*$expire));
setcookie("password",$_POST['pwd'],time()+(3600*24*$expire));
//登陆成功,注册session
session_start();
$_SESSION['name']=$_POST['user'];
//网页重定向
header("location:index.php");
}else{
echo "<script>alert('登录失败,请重新登录。');location.href='login.php'</script>)";
}