主要知识点:掌握 Java 程序基本结构,变量定义,基本数据类型,各种运算符,
控制台输入输出 。
掌握:Java 类的创建,主方法,变量的定义,控制台输入各种类型的数据,基本运算符,控
制台输出 。
Eclipse 常用快捷键(所有快捷键可通过菜单 Help-Show Active Keybindings 进行查看)
Ctrl+:放大字体
Ctrl-:缩写字体
输入部分信息后,同时按下 Alt 和/,自动补齐代码,例如输入 syso 后同时按下 alt 和/后自
动补齐代码 System.out.println();
Ctrl+Shift+F :代码自动排版格式化
例题1:
Imagine that you and your band have just recorded a song, and you want to
permit fans to purchase and download the song over the Internet. Your
manager receives a commission equal to 12% of the total sales revenue for
your song, and the Internet site that lists your song receives a 3% commission.
Write a java program that asks the user for the title of the song, the purchase
price of the song (in dollars and cents), and the number of copies of the song
that have been sold. Calculate the total revenue generated by your song, the
commission received by your manager, and the commission received by the
Internet site. Use named constants for the commission rates.
(想象一下,你和你的乐队刚刚录制了一首歌,你想让粉丝们在网上购买和下载这首歌。你的经纪人会收到相当于你歌曲总销售收入的12%的佣金,而列出你歌曲的网站会收到3%的佣金。编写一个java程序,向用户提供歌曲的标题,歌曲的购买价格(以美元和美分计算),以及已售出的歌曲拷贝数量。计算你的歌曲所产生的总收入,你的经纪人收到的佣金,以及互联网网站收到的佣金。对佣金率使用常量命名。)
package test1.test;
import java.util.Scanner;
public class SongRevence {
// 有若干个method方法,有且只有一个main方法(程序执行的入口)
public static void main(String[] args) {
final float MANAGER_COMMISSION=0.12f;//常量定义 常量名一般全大写 多单词用下划线隔开
final float SITE_COMMISION=0.03f;
// 1.定义变量:名字 类型
String songTitle;// 顾名思义,驼峰命名
float price;
int copies;
float totalRevence;
float managerRevence;
float siteRevence;
// 2.通过键盘输入给某些变量赋值
Scanner input = new Scanner(System.in);
System.out.print("请输入歌曲名称:");
songTitle = input.nextLine();
System.out.print("请输入歌曲单价:");
price = input.nextFloat();
System.out.print("请输入下载份数:");
copies = input.nextInt();
// 3.通过运算赋值给某些变量
totalRevence = price * copies;
managerRevence = totalRevence * MANAGER_COMMISSION;
siteRevence=totalRevence*SITE_COMMISION;
// 4.输出某些变量到控制台中
System.out.println("总利润="+totalRevence);
System.out.println("经纪人利润="+managerRevence);
System.out.println("站点利润="+siteRevence);
}
}
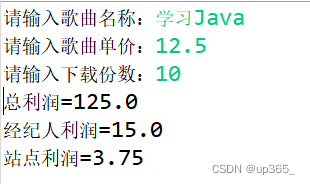
例题2:
A box of biscuits can hold 24 biscuits and a container can hold 75 boxes of
biscuits. Write a program that prompts the user to enter the total number of
biscuits. The program then outputs the number of boxes and the number of
containers to ship the biscuits. Note that each box must contain the specified
number of biscuits and each container must contain the specified number of
boxes. If the last box of biscuits contains less than the number of specified
biscuits, you can discard it, and output the number of leftover biscuits.
Similarly, if the last container contains less than the number of specified
boxes, you can discard it, and output the number of leftover boxes .
(一盒饼干可以装24块饼干,一个容器可以装75盒饼干。编写一个程序,提示用户输入饼干的总数。然后,该程序输出运送饼干的盒子数量和容器数量。请注意,每个盒子必须包含指定数量的饼干,而每个容器必须包含指定数量的盒子。如果最后一盒饼干所包含的数量少于指定的饼干的数量,你可以丢弃它,并输出剩余饼干的数量。类似地,如果最后一个容器包含的数量少于指定的盒子,您可以丢弃它,并输出剩余盒子的数量。)
package test1.excise;
import java.util.Scanner;
public class Biscuit {
public static void main(String[] args) {
int num;
int boxes;
int containers;
int dbiscuits;
int dboxes;
Scanner input = new Scanner(System.in);
System.out.println("请输入饼干总块数:");
num=input.nextInt();
boxes=num/24;
dbiscuits=num%24;
containers=boxes/75;
dboxes=boxes%75;
System.out.println("饼干盒数"+boxes);
System.out.println("容器数"+containers);
}
}
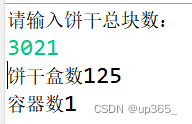
例题3:
Emulate a vending machine. Calculate the number of dollars and coins
required to express a given amount of change in dollars, 50, 20, 10 and 5 cent
coins. For example, $9.85 would be $9, 1 x 50c, 1 x 20x and 1 x 5c coins.
Then modify it so that it also prints out the number of $1 and $2 coins.
package exercise1;
import java.util.Scanner;
public class vending_machine1 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("请输入钱数:");
float x=input.nextFloat();
int y=(int)x;
int a,b,c,d,e,f;
a=y/2;
b=y%2;
y=(int)(x*100);
y%=100;
c=y/50;
y%=50;
d=y/20;
y%=20;
e=y/10;
y%=10;
f=y/5;
System.out.println("2美元数量:"+a);
System.out.println("1美元数量:"+b);
System.out.println("50美分数量:"+c);
System.out.println("20美分数量:"+d);
System.out.println("10美分数量:"+e);
System.out.println("5美分数量:"+f);
}
}
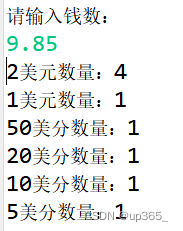
例题4:
You have several pictures of different sizes that you would like to frame. A
local picture framing store offers two types of frames – regular and fancy. The
frames are available in white and can be ordered in any colour the customer
desires. Suppose that each frame is 1 inch wide. The cost of colouring the
frame is $0.10 per inch. The cost of a regular frame is $0.15 per inch and the
cost of a fancy frame is $0.25 per inch. The cost of putting a cardboard paper
behind the picture is $0.02 per square inch and the cost of putting glass on top
of the picture is $0.07 per square inch. The customer can also choose to put
crowns on the corners, which cost $0.35 per crown.
Write a program that prompts the user to input the following information and
then output the cost of framing the picture.
a) The length and width, in inches, of the picture
b) The type of the frame
c) Customer’s choice of colour to colour the frame
d) If the user wants to put the crowns, then the number of crowns.
package exercise1;
import java.util.Scanner;
public class frame2 {
public static void main(String[] args) {
double imagel, imagew;
int x;
String color;
String str="white";
int crown;
double money = 0;
double s;
Scanner input = new Scanner(System.in);
System.out.print("请输入图片的长和宽(单位inch):");
imagel = input.nextDouble();
imagew = input.nextDouble();
System.out.print("请输入框架类型(普通:0;高级:1):");
x = input.nextInt();
System.out.print("请输入框架颜色:");
input.nextLine();
color = input.nextLine();
System.out.print("请输入皇冠👑数量:");
crown = input.nextInt();
s = (imagel + 2) * (imagew + 2) - imagel * imagew;
money += imagel * imagew * (0.02 + 0.07);
if (x == 0) {
money += s * 0.15;
} else {
money += s * 0.25;
}
if (color.compareTo(str)==0) {
money += 0;
} else {
money += s * 0.1;
}
money += crown * 0.35;
System.out.println("成本为:" + money);
}
}
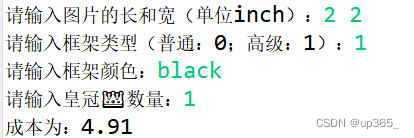
注意:
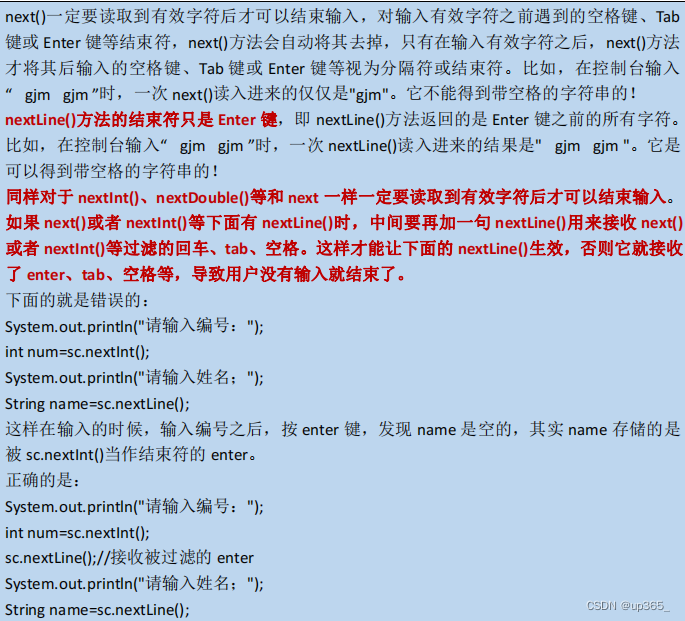