特征工程
概念
从原始数据中提取、构建和选择特征,以便为机器学习模型提供有用的信息。
步骤:
- 特征提取
- 无量刚化(预处理)
归一化
标准化 - 降维
底方差过滤特征选择
主成分分析-PCA降维
API
在Python的scikit-learn
库中用于特征工程的常用工具
-
DictVectorizer(字典特征提取):
- 将字典形式的数据转换为数值特征矩阵。这通常用于处理字典列表,其中每个字典代表一个样本的特征。
#DictVectorizer 字典列表特征提取 from sklearn.feature_extraction import DictVectorizer data = [ {'city': '成都', 'age': 30, 'temperature': 20}, {'city': '重庆', 'age': 33, 'temperature': 60}, {'city': '成都', 'age': 72, 'temperature': 40}, {'city': '广州', 'age': 28, 'temperature': 30}, {'city': '深圳', 'age': 35, 'temperature': 65}, {'city': '成都', 'age': 58, 'temperature': 42} ] transfer = DictVectorizer(sparse=True) data_new = transfer.fit_transform(data) x = data_new.toarray() print(x) print(transfer.get_feature_names_out()) ''' 结果: [[30. 0. 1. 0. 0. 20.] [33. 0. 0. 0. 1. 60.] [72. 0. 1. 0. 0. 40.] [28. 1. 0. 0. 0. 30.] [35. 0. 0. 1. 0. 65.] [58. 0. 1. 0. 0. 42.]] ['age' 'city=广州' 'city=成都' 'city=深圳' 'city=重庆' 'temperature'] ''' import pandas pandas.DataFrame(x, columns=transfer.get_feature_names_out()) ''' 结果: age city=广州 city=成都 city=深圳 city=重庆 temperature 0 30.0 0.0 1.0 0.0 0.0 20.0 1 33.0 0.0 0.0 0.0 1.0 60.0 2 72.0 0.0 1.0 0.0 0.0 40.0 3 28.0 1.0 0.0 0.0 0.0 30.0 4 35.0 0.0 0.0 1.0 0.0 65.0 5 58.0 0.0 1.0 0.0 0.0 42.0 '''
- 将字典形式的数据转换为数值特征矩阵。这通常用于处理字典列表,其中每个字典代表一个样本的特征。
-
CountVectorizer(文本特征提取):
- 将文本数据转换为词频特征矩阵。它统计文本中每个单词出现的次数,并将这些次数作为特征。
#CountVectorizer 文本特征提取 from sklearn.feature_extraction.text import CountVectorizer #英文文本提取 data = ["the Yellow River's water comes from the sky", "Once the river rushes to the sea, it never turns back."] transfer = CountVectorizer(stop_words=['the', 'to', 'from']) data_new = transfer.fit_transform(data) print(data_new) print(transfer.get_feature_names_out()) ''' 结果: (0, 11) 1 (0, 5) 1 (0, 10) 1 (0, 1) 1 (0, 8) 1 (1, 5) 1 (1, 4) 1 (1, 6) 1 (1, 7) 1 (1, 2) 1 (1, 3) 1 (1, 9) 1 (1, 0) 1 ['back' 'comes' 'it' 'never' 'once' 'river' 'rushes' 'sea' 'sky' 'turns' 'water' 'yellow'] ''' #中文文本提取 import jieba data1 = "黄河之水天上来,奔流到海不复回" def jieba_cut(word): return " ".join(list(jieba.cut(word))) data = [jieba_cut(data1)] print(data) tokenizer = CountVectorizer() data_new = tokenizer.fit_transform(data) print(data_new) pd.DataFrame(data_new.toarray(), columns=tokenizer.get_feature_names_out()) ''' 结果: ['黄河 之水 天上 来 , 奔流 到 海不复 回'] (0, 4) 1 (0, 0) 1 (0, 1) 1 (0, 2) 1 (0, 3) 1 之水 天上 奔流 海不复 黄河 0 1 1 1 1 1 '''
- 将文本数据转换为词频特征矩阵。它统计文本中每个单词出现的次数,并将这些次数作为特征。
-
TfidfVectorizer(TF-IDF文本特征词的重要程度特征提取):
- 将文本数据转换为TF-IDF特征矩阵。TF-IDF代表词频-逆文档频率,是一种统计方法,用于评估单词对于一个文档集或一个语料库中的其中一份文档的重要性。
from sklearn.feature_extraction.text import TfidfVectorizer # TfidfVectorizer TF-IDF文本特征词的重要程度特征提取 data = ["君不见,黄河之水天上来,奔流到海不复回。", "君不见,高堂明镜悲白发,朝如青丝暮成雪。", "人生得意须尽欢,莫使金樽空对月。", "天生我材必有用,千金散尽还复来。", "烹羊宰牛且为乐,会须一饮三百杯。", "岑夫子,丹丘生,将进酒,杯莫停。" ] data = [jieba_cut(i) for i in data] tokenizer = TfidfVectorizer() data_new = tokenizer.fit_transform(data) pd.DataFrame(data_new.toarray(), columns=tokenizer.get_feature_names_out()) ''' 结果: 一饮 三百杯 丹丘 为乐 之水 人生 会须 千金 \ 0 0.000000 0.000000 0.0 0.000000 0.419871 0.000000 0.000000 0.0 1 0.000000 0.000000 0.0 0.000000 0.000000 0.000000 0.000000 0.0 2 0.000000 0.000000 0.0 0.000000 0.000000 0.447214 0.000000 0.0 3 0.000000 0.000000 0.0 0.000000 0.000000 0.000000 0.000000 0.5 4 0.408248 0.408248 0.0 0.408248 0.000000 0.000000 0.408248 0.0 5 0.000000 0.000000 0.5 0.000000 0.000000 0.000000 0.000000 0.0 君不见 复来 ... 杯莫停 海不复 烹羊 白发 莫使 金樽空 \ 0 0.344300 0.0 ... 0.0 0.419871 0.000000 0.000000 0.000000 0.000000 1 0.317453 0.0 ... 0.0 0.000000 0.000000 0.387131 0.000000 0.000000 2 0.000000 0.0 ... 0.0 0.000000 0.000000 0.000000 0.447214 0.447214 3 0.000000 0.5 ... 0.0 0.000000 0.000000 0.000000 0.000000 0.000000 4 0.000000 0.0 ... 0.0 0.000000 0.408248 0.000000 0.000000 0.000000 5 0.000000 0.0 ... 0.5 0.000000 0.000000 0.000000 0.000000 0.000000 青丝 须尽欢 高堂 黄河 0 0.000000 0.000000 0.000000 0.419871 1 0.387131 0.000000 0.387131 0.000000 2 0.000000 0.447214 0.000000 0.000000 3 0.000000 0.000000 0.000000 0.000000 4 0.000000 0.000000 0.000000 0.000000 5 0.000000 0.000000 0.000000 0.000000 [6 rows x 31 columns] '''
- 将文本数据转换为TF-IDF特征矩阵。TF-IDF代表词频-逆文档频率,是一种统计方法,用于评估单词对于一个文档集或一个语料库中的其中一份文档的重要性。
-
MinMaxScaler(归一化):
- 将特征缩放到指定的范围内,通常是0到1或-1到1。这是一种归一化方法,使得不同特征具有相同的尺度。
from sklearn.preprocessing import MinMaxScaler #原始数据类型为list data = np.random.randint(1, 100, size=15) data = data.reshape(3, 5) print(data) transformer = MinMaxScaler(feature_range=(0, 1)) data_new = transformer.fit_transform(data) print(data_new) ''' [[48 3 26 10 46] [53 78 10 81 61] [62 85 12 22 53]] [[0. 0. 1. 0. 0. ] [0.35714286 0.91463415 0. 1. 1. ] [1. 1. 0.125 0.16901408 0.46666667]] ''' # 原始数据类型为DataFrame print(data) data = pd.DataFrame(data=data, index=range(0, 3), columns=["一列", "二列", "三列", "4裂", "wu烈"]) transfer = MinMaxScaler(feature_range=(0, 1)) data_new = transfer.fit_transform(data) print(data_new) ''' [[48 3 26 10 46] [53 78 10 81 61] [62 85 12 22 53]] [[0. 0. 1. 0. 0. ] [0.35714286 0.91463415 0. 1. 1. ] [1. 1. 0.125 0.16901408 0.46666667]] '''
- 将特征缩放到指定的范围内,通常是0到1或-1到1。这是一种归一化方法,使得不同特征具有相同的尺度。
-
StandardScaler(标准化):
- 通过Z分数(即标准差)对特征进行标准化,使得特征具有均值为0和标准差为1。这可以减少异常值的影响。
import pandas as pd from sklearn.preprocessing import StandardScaler data = pd.read_csv('../src/dating.txt') print(data.shape) print(type(data)) transfer = StandardScaler() data_new = transfer.fit_transform(data) print(data_new[0:5]) ''' (1000, 4) <class 'pandas.core.frame.DataFrame'> [[ 0.33193158 0.41660188 0.24523407 1.24115502] [-0.87247784 0.13992897 1.69385734 0.01834219] [-0.34554872 -1.20667094 -0.05422437 -1.20447063] [ 1.89102937 1.55309196 -0.81110001 -1.20447063] [ 0.2145527 -1.15293589 -1.40400471 -1.20447063]] '''
- 通过Z分数(即标准差)对特征进行标准化,使得特征具有均值为0和标准差为1。这可以减少异常值的影响。
-
VarianceThreshold(低方差过滤降维):
- 移除方差低于指定阈值的特征。这是一种降维技术,用于移除变化不大的特征,从而减少数据的复杂性。
from sklearn.feature_selection import VarianceThreshold print(data) transformer = VarianceThreshold(threshold=1000) data_new = transformer.fit_transform(data) print(data_new) ''' 一列 二列 三列 4裂 wu烈 0 21 5 74 28 22 1 98 12 58 66 81 2 10 97 90 35 81 [[21 5] [98 12] [10 97]] '''
- 移除方差低于指定阈值的特征。这是一种降维技术,用于移除变化不大的特征,从而减少数据的复杂性。
-
PCA(主成分分析降维):
- 一种统计方法,用于将数据转换到较低维度的空间,同时尽可能保留原始数据的方差。PCA是一种常用的线性降维技术。
from scipy.stats import pearsonr data = pd.read_csv('../src/factor_returns.csv') data = data.iloc[:, 1:-2] r1 = pearsonr(data["market_cap"], data["return_on_asset_net_profit"]) print(r1) ''' PearsonRResult(statistic=0.21477388362301314, pvalue=1.359507950014138e-25) '''
- 一种统计方法,用于将数据转换到较低维度的空间,同时尽可能保留原始数据的方差。PCA是一种常用的线性降维技术。
作业
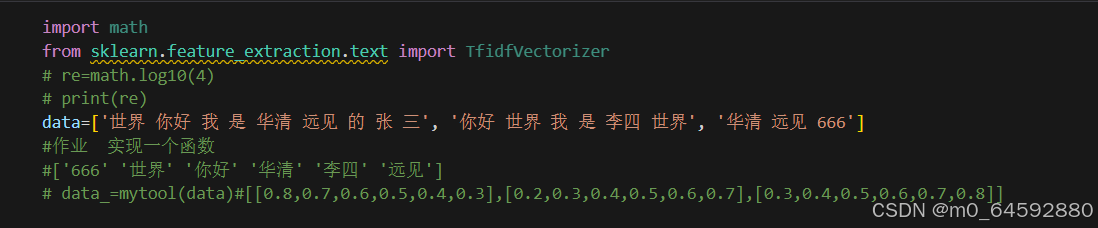
一个文本特征出现在几个字符串中
data = ['世界 你好 我 是 华清 远见 的 张 三', '你好 世界 我 是 李四 世界', '华清 远见 666']
def count_mun(list, word):
count = 0
for w in list:
num = 0
for i in w:
if i in word:
num += 1
if num == len(word):
count += 1
return count
num = count_mun(data, "你好")
print(num)
from sklearn.feature_extraction.text import TfidfVectorizer
# re=math.log10(4)
# print(re)
data = ['世界 你好 我 是 华清 远见 的 张 三', '你好 世界 我 是 李四 世界', '华清 远见 666']
# 作业 实现一个函数
# ['666' '世界' '你好' '华清' '李四' '远见']
# data_=mytool(data)#[[0.8,0.7,0.6,0.5,0.4,0.3],[0.2,0.3,0.4,0.5,0.6,0.7],[0.3,0.4,0.5,0.6,0.7,0.8]]
tokenizer = TfidfVectorizer()
data_new = tokenizer.fit_transform(data)
data_new = data_new.toarray()
# print(tokenizer.get_feature_names_out())
print(data_new)
num = 0
for i in tokenizer.get_feature_names_out():
count = 0
for j in data_new[:, num]:
if j != 0:
count += 1
num += 1
print(i, "出现在了", count, "个字符串中")