1、ILog
package com.atguigu.design.demo.spring;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
import org.springframework.util.CollectionUtils;
import java.util.Collection;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public interface ILog {
void log(String msg);
}
@BeanLogType(LogType.FILE_LOG)
class FileLog implements ILog {
@Override
public void log(String msg) {
System.out.println("输出日志到文件:" + msg);
}
}
@BeanLogType(LogType.CONSOLE_LOG)
class ConsoleLog implements ILog {
@Override
public void log(String msg) {
System.out.println("输出日志到控制台:" + msg);
}
}
@Component
class LogClient implements ApplicationContextAware {
private static final Map<String, ILog> MAP = new ConcurrentHashMap<>();
public void log(String type, String msg) {
MAP.get(type).log(msg);
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
Map<String, Object> beanMap = applicationContext.getBeansWithAnnotation(BeanLogType.class);
if (CollectionUtils.isEmpty(beanMap)) {
return;
}
Collection<Object> beans = beanMap.values();
beans.forEach(bean -> {
LogType logType = bean.getClass().getAnnotation(BeanLogType.class).value();
MAP.put(logType.type, (ILog) bean);
});
}
}
2、LogType 枚举类
package com.atguigu.design.demo.spring;
public enum LogType {
FILE_LOG("1","文件日志"),
CONSOLE_LOG("2","控制台日志")
;
public String type;
public String desc;
LogType(String type, String desc) {
this.type = type;
this.desc = desc;
}
}
3、@BeanLogType 注解
package com.atguigu.design.demo.spring;
import org.springframework.stereotype.Component;
import java.lang.annotation.*;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Component
public @interface BeanLogType {
LogType value();
}
4、DemoController
package com.atguigu.design.demo.spring;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DemoController {
@Autowired
private LogClient logClient;
@GetMapping("log/{type}")
public String log(@PathVariable String type, @RequestParam("msg") String msg) {
logClient.log(type, msg);
return "日志输出成功!";
}
}
5、application.properties
spring.application.name=design-demo
server.port=10010
6、DesignDemoApplication
package com.atguigu.design.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DesignDemoApplication {
public static void main(String[] args) {
SpringApplication.run(DesignDemoApplication.class, args);
}
}
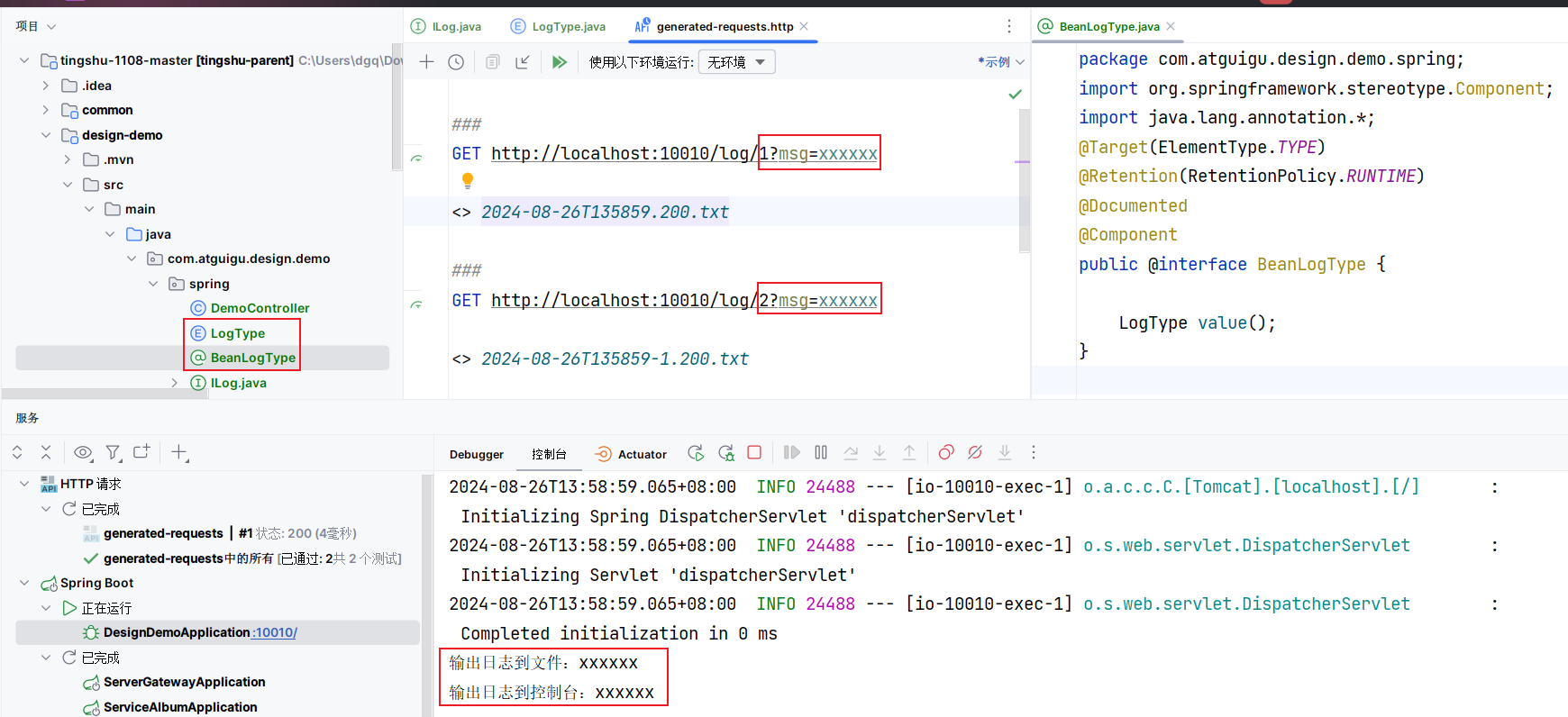