6种读取文件内容方式
以下介绍6中读取文件内容的方式:
Scanner 可以按行、按自定义分隔符读取数据(特殊字符除外)(jdk1.7)
BufferedReader 缓冲字符流(jdk1.1)
Files.lines 返回Stream 流式数据,按行处理(jdk1.8)
Files.readAllLines 返回 List(jdk1.8)
Files.readString 读取string,文件最大2G(jdk11)
Files.readAllBytes 读取byte[],文件最大2G(jdk1.7)
目标读取 InputFiledList.txt 中的内容,位置如下:
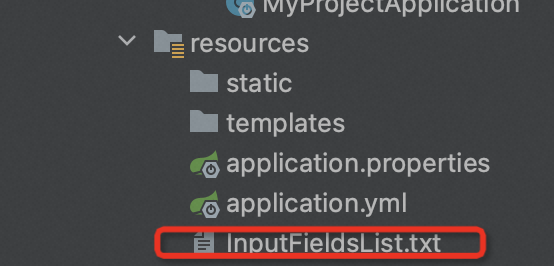
文件内容:
tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world
1、Scanner
Scanner 自jdk1.5提供,特点可以按行、按自定义分隔符读取数据,包括文字、数字。
代码如下:
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* Scanner
*/
@Test
public void testScanner() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
String path = resourceLoader.getResource(filePath).getURL().getPath();
Scanner scanner = new Scanner(new FileInputStream(path));
/**
* 按行读取数据
*/
while (scanner.hasNextLine()) {
System.out.println("按行读取数据:" + scanner.nextLine());
}
System.out.println();
/**
* 按自定义分隔符读取数据
*/
scanner = new Scanner(new FileInputStream(path));
scanner.useDelimiter("\\|");
while (scanner.hasNext()) {
System.out.println("按分隔符 | 读取数据:" + scanner.next());
}
}
输出结果
按行读取数据:tableFields:name,address,city
按行读取数据:excludeFields:age,weight
按行读取数据:exampleString:this is an example|hello world
按分隔符 | 读取数据:tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example
按分隔符 | 读取数据:hello world
2、BufferedReader
缓冲流方式,这种方式可以通过管道流嵌套的方式,组合使用,比较灵活。
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* BufferedReader
*/
@Test
public void testBufferedReader() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
String path = resourceLoader.getResource(filePath).getURL().getPath();
BufferedReader bufferedReader = new BufferedReader(new FileReader(path));
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
}
输出结果
tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world
3、Files.lines
如果你是需要按行去处理数据文件的内容,这种方式是我推荐大家去使用的一种方式,代码简洁,使用java 8的Stream流将文件读取与文件处理融合。
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* lines
*/
@Test
public void testFileLines() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
URI uri = resourceLoader.getResource(filePath).getURI();
Stream<String> lines = Files.lines(Paths.get(uri));
lines.forEach(line -> {
System.out.println(line);
});
}
结果
tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world
4、Files.readAllLines
readAllLines 会返回一个字符串集合。
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* readAllLines
*/
@Test
public void testFileReadAllLine() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
URI uri = resourceLoader.getResource(filePath).getURI();
List<String> lines = Files.readAllLines(Paths.get(uri));
lines.forEach(System.out::println);
}
结果
tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world
5、Files.readString
从 java11开始,为我们提供了一次性读取一个文件的方法。文件不能超过2G,同时要注意你的服务器及JVM内存。这种方法适合快速读取小文本文件。
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* readString
*/
@Test
public void testReadString() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
URI uri = resourceLoader.getResource(filePath).getURI();
// String lines = Files.readString(Paths.get(uri));
// System.out.println("lines = " + lines);
}
结果
content = tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world
6、Files.readAllBytes
如果你没有JDK11(readAllBytes()始于JDK7),仍然想一次性的快速读取一个文件的内容转为String,该怎么办?先将数据读取为二进制数组,然后转换成String内容。这种方法适合在没有JDK11的请开给你下,快速读取小文本文件。
private static final String filePath = "classpath:generateComparisonSql.txt";
/**
* readAllBytes
*/
@Test
public void testReadAllBytes() throws IOException {
DefaultResourceLoader resourceLoader = new DefaultResourceLoader();
URI uri = resourceLoader.getResource(filePath).getURI();
byte[] bytes = Files.readAllBytes(Paths.get(uri));
System.out.println("content = " + new String(bytes, StandardCharsets.UTF_8));
}
结果
content = tableFields:name,address,city
excludeFields:age,weight
exampleString:this is an example|hello world