一、页面跳转方式
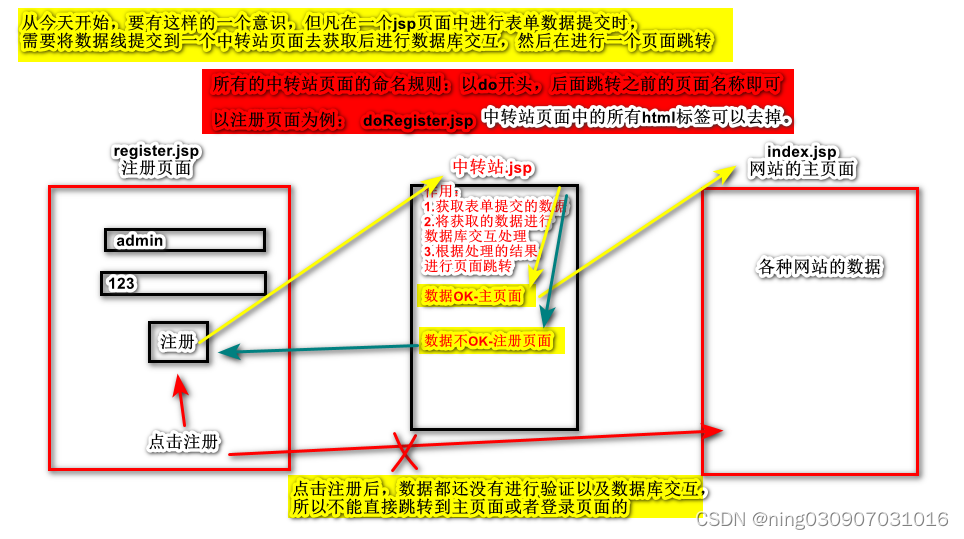
(1)javascript方式跳转
window.location.href = "跳转的地址"
(2)java方式跳转
① 转发
1.1 概述
由服务器端进行的页面跳转
1.2 方法
(1)获取转发器
RequestDispatcher rd = request.getRequestDispatcher("/跳转的地址")
(2)实现转发
转发器对象.forward(request,response);
通常简写: request.getRequestDispatcher("/跳转的地址").forward(request,response);
1.3 特点
(1)地址栏不发生变化,显示的是上一个页面的地址
(2)请求次数:只有1次请求,因为转发是服务端行为。
(3)根目录:http://localhost:8080/项目地址/,包含了项目的访问地址
(4)请求域中数据不会丢失
② 重定向
2.1 概述
由浏览器端进行的页面跳转
2.2 方法
response.sendRedirect("要跳转的地址");
2.3 特点
(1)地址栏:显示新的地址
(2)请求次数:2次
(3)根目录:http://localhost:8080/ 没有项目的名字
(4)请求域中的数据会丢失,因为是2次请求
③ 注意事项
3.1 什么时候使用转发,什么时候使用重定向?
如果要保留请求域中的数据,使用转发,否则使用重定向。
以后访问数据库,增删改使用重定向,查询使用转发。
3.2 转发或重定向后续的代码是否还会运行?
无论转发或重定向后续的代码都会执行
④ 重定向和转发的区别
<%
//设置编码格式
request.setCharacterEncoding("utf-8");
//获取用户名和密码
String username = request.getParameter("username");
String password = request.getParameter("password");
if("admin".equals(username)){
out.println("<script>");
out.println("alert('该用户已被注册,请重新进行注册');");
out.println("location.href='register.jsp'");
out.println("</script>");
}else{//可以注册
//直接可以跳转到index.jsp
/* 通过location页面跳转 */
out.println("<script>");
out.println("location.href='index.jsp'");
out.println("</script>");
/* 通过Java的方式 转发进行跳转 */
//response 内置对象 响应
request.getRequestDispatcher("index.jsp").forward(request, response);
/* 通过Java的方式 重定向进行跳转 */
response.sendRedirect("index.jsp");
/* lcoation和重定向(response.sendRedirect("index.jsp");)是一样
地址栏发生改变,数据不会保留
*/
/* 转发
地址栏不会发生改变,数据会保留 可以通过request对象进行获取。
*/
request.getRequestDispatcher("index.jsp").forward(request, response);
}
%>
(3)超链接方式
<a href = "路径">资源地址</a>
携带参数的注意事项: 第一个参数之前 (?) 参数与参数之间使用(&)
<!-- 携带参数的注意事项: 第一个参数之前 (?) 参数与参数之间使用(&) -->
<a href = "index.jsp?username=admin&password=123">跳转到主页</a>
<!-- js跳转 -->
<hr/>
<!-- 通过location跳转可以携带参数 后面可以通过request对象进行获取 -->
<button onclick = "add();">跳转到index.jsp</button>
<script type="text/javascript">
function add(){
/* 通过js的location对象进行页面跳转 */
location.href = "index.jsp?result=123";
}
</script>
二、JDBC API
(1)主要功能
与数据库建立连接、执行SQL 语句、处理结果
(2)常用对象
① DriverManager
依据数据库的不同,管理JDBC驱动
② Connection
负责连接数据库并担任传送数据的任务
③ PreparedStatement
由 Connection 产生、负责执行SQL语句
④ ResultSet
负责保存Statement执行后所产生的查询结果
(3) java通过jdbc连接Oracle数据库进行交互的步骤
1、加载JDBC驱动
Class.forName(JDBC驱动类);
2、与数据库建立连接
Connection conn=DriverManager.getConnection
("jdbc:oracle:thin:@127.0.0.1:1521:orcl","scott","admin");
3、发送SQL语句,并得到返回结果
4、处理返回结果
5、释放资源
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//1.设置编码
request.setCharacterEncoding("utf-8");
//2.获取数据
String username = request.getParameter("username");
String password = request.getParameter("password");
//进行Oracle数据库交互
//定义两个变量存储用户名以及密码
String uname = "";
String pwd = "";
//连接对象定义
Connection conn = null;
PreparedStatement ps = null;//执行对象
//结果集对象
ResultSet rs = null;
try{
//加载驱动
Class.forName("oracle.jdbc.driver.OracleDriver");
//b.建立连接
String url = "jdbc:oracle:thin:@localhost:1521:orcl";
conn = DriverManager.getConnection(url, "scott", "123");
//编写sql语句传入执行方法返回执行对象
String sql = "select * from tb_users where username = ? and upassword = ?";
ps = conn.prepareStatement(sql);
//占位符赋值
ps.setString(1, username);
ps.setString(2, password);
//返回结果集对象
rs = ps.executeQuery();
//遍历或者判断
if(rs.next()){
uname = rs.getString(2);
pwd = rs.getString(3);
}
}catch(Exception e){
e.printStackTrace();
}finally{
if(conn!=null&&!conn.isClosed()){
conn.close();
}
if(ps!=null){
ps.close();
}
if(rs!=null){
rs.close();
}
}
if(uname!="" && pwd!=""){
out.println("<script>alert('你已注册,请登录');location.href='login.jsp';</script>");
}else{
request.getRequestDispatcher("registerhome.jsp").forward(request, response);
}
%>