A. Lucky?
A ticket is a string consisting of six digits. A ticket is considered lucky if the sum of the first three digits is equal to the sum of the last three digits. Given a ticket, output if it is lucky or not. Note that a ticket can have leading zeroes.
Input
The first line of the input contains an integer t t t ( 1 ≤ t ≤ 1 0 3 1 \leq t \leq 10^3 1≤t≤103) — the number of testcases.
The description of each test consists of one line containing one string consisting of six digits.
Output
Output t t t lines, each of which contains the answer to the corresponding test case. Output “YES” if the given ticket is lucky, and “NO” otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
5
213132
973894
045207
000000
055776
output
YES
NO
YES
YES
NO
Note
In the first test case, the sum of the first three digits is 2 + 1 + 3 = 6 2 + 1 + 3 = 6 2+1+3=6 and the sum of the last three digits is 1 + 3 + 2 = 6 1 + 3 + 2 = 6 1+3+2=6, they are equal so the answer is “YES”.
In the second test case, the sum of the first three digits is 9 + 7 + 3 = 19 9 + 7 + 3 = 19 9+7+3=19 and the sum of the last three digits is 8 + 9 + 4 = 21 8 + 9 + 4 = 21 8+9+4=21, they are not equal so the answer is “NO”.
In the third test case, the sum of the first three digits is 0 + 4 + 5 = 9 0 + 4 + 5 = 9 0+4+5=9 and the sum of the last three digits is 2 + 0 + 7 = 9 2 + 0 + 7 = 9 2+0+7=9, they are equal so the answer is “YES”.
Tutorial
模拟
Solution
for _ in range(int(input())):
n, ans, cnt = int(input()), 0, 6
while cnt > 3:
ans += n % 10
n //= 10
cnt -= 1
while cnt:
ans -= n % 10
n //= 10
cnt -= 1
print("NO" if ans else "YES")
B. Equal Candies
There are n n n boxes with different quantities of candies in each of them. The i i i-th box has a i a_i ai candies inside.
You also have n n n friends that you want to give the candies to, so you decided to give each friend a box of candies. But, you don’t want any friends to get upset so you decided to eat some (possibly none) candies from each box so that all boxes have the same quantity of candies in them. Note that you may eat a different number of candies from different boxes and you cannot add candies to any of the boxes.
What’s the minimum total number of candies you have to eat to satisfy the requirements?
Input
The first line contains an integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases.
The first line of each test case contains an integer n n n ( 1 ≤ n ≤ 50 1 \leq n \leq 50 1≤n≤50) — the number of boxes you have.
The second line of each test case contains n n n integers a 1 , a 2 , … , a n a_1, a_2, \dots, a_n a1,a2,…,an ( 1 ≤ a i ≤ 1 0 7 1 \leq a_i \leq 10^7 1≤ai≤107) — the quantity of candies in each box.
Output
For each test case, print a single integer denoting the minimum number of candies you have to eat to satisfy the requirements.
Example
input
5
5
1 2 3 4 5
6
1000 1000 5 1000 1000 1000
10
1 2 3 5 1 2 7 9 13 5
3
8 8 8
1
10000000
output
10
4975
38
0
0
Note
For the first test case, you can eat 1 1 1 candy from the second box, 2 2 2 candies from the third box, 3 3 3 candies from the fourth box and 4 4 4 candies from the fifth box. Now the boxes have [ 1 , 1 , 1 , 1 , 1 ] [1, 1, 1, 1, 1] [1,1,1,1,1] candies in them and you ate 0 + 1 + 2 + 3 + 4 = 10 0 + 1 + 2 + 3 + 4 = 10 0+1+2+3+4=10 candies in total so the answer is 10 10 10.
For the second test case, the best answer is obtained by making all boxes contain 5 5 5 candies in them, thus eating 995 + 995 + 0 + 995 + 995 + 995 = 4975 995 + 995 + 0 + 995 + 995 + 995 = 4975 995+995+0+995+995+995=4975 candies in total.
Tutorial
能让所有糖果数量相同只能向最少的糖果数对齐
Solution
for _ in range(int(input())):
n = int(input())
a = list(map(int, input().split()))
print(sum(a) - min(a) * n)
C. Most Similar Words
You are given n n n words of equal length m m m, consisting of lowercase Latin alphabet letters. The i i i-th word is denoted s i s_i si.
In one move you can choose any position in any single word and change the letter at that position to the previous or next letter in alphabetical order. For example:
- you can change ‘e’ to ‘d’ or to ‘f’;
- ‘a’ can only be changed to ‘b’;
- ‘z’ can only be changed to ‘y’.
The difference between two words is the minimum number of moves required to make them equal. For example, the difference between “best” and “cost” is 1 + 10 + 0 + 0 = 11 1 + 10 + 0 + 0 = 11 1+10+0+0=11.
Find the minimum difference of s i s_i si and s j s_j sj such that ( i < j ) (i < j) (i<j). In other words, find the minimum difference over all possible pairs of the n n n words.
Input
The first line of the input contains a single integer t t t ( 1 ≤ t ≤ 100 1 \le t \le 100 1≤t≤100) — the number of test cases. The description of test cases follows.
The first line of each test case contains 2 2 2 integers n n n and m m m ( 2 ≤ n ≤ 50 2 \leq n \leq 50 2≤n≤50, 1 ≤ m ≤ 8 1 \leq m \leq 8 1≤m≤8) — the number of strings and their length respectively.
Then follows n n n lines, the i i i-th of which containing a single string s i s_i si of length m m m, consisting of lowercase Latin letters.
Output
For each test case, print a single integer — the minimum difference over all possible pairs of the given strings.
Example
input
6
2 4
best
cost
6 3
abb
zba
bef
cdu
ooo
zzz
2 7
aaabbbc
bbaezfe
3 2
ab
ab
ab
2 8
aaaaaaaa
zzzzzzzz
3 1
a
u
y
output
11
8
35
0
200
4
Note
For the second test case, one can show that the best pair is (“abb”,“bef”), which has difference equal to 8 8 8, which can be obtained in the following way: change the first character of the first string to ‘b’ in one move, change the second character of the second string to ‘b’ in 3 3 3 moves and change the third character of the second string to ‘b’ in 4 4 4 moves, thus making in total 1 + 3 + 4 = 8 1 + 3 + 4 = 8 1+3+4=8 moves.
For the third test case, there is only one possible pair and it can be shown that the minimum amount of moves necessary to make the strings equal is 35 35 35.
For the fourth test case, there is a pair of strings which is already equal, so the answer is 0 0 0.
Tutorial
两两对比做模拟
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, m, ans = INF;
cin >> n >> m;
vector<string> s(n);
for (string &si : s) {
cin >> si;
}
for (int i = 1; i < n; ++i) {
for (int j = 0; j < i; ++j) {
int mid = 0;
for (int k = 0; k < m; ++k) {
mid += abs(s[i][k] - s[j][k]);
}
ans = min(ans, mid);
}
}
cout << ans << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
D. X-Sum
Timur’s grandfather gifted him a chessboard to practice his chess skills. This chessboard is a grid a a a with n n n rows and m m m columns with each cell having a non-negative integer written on it.
Timur’s challenge is to place a bishop on the board such that the sum of all cells attacked by the bishop is maximal. The bishop attacks in all directions diagonally, and there is no limit to the distance which the bishop can attack. Note that the cell on which the bishop is placed is also considered attacked. Help him find the maximal sum he can get.
Input
The first line of the input contains a single integer t t t ( 1 ≤ t ≤ 1000 1 \le t \le 1000 1≤t≤1000) — the number of test cases. The description of test cases follows.
The first line of each test case contains the integers n n n and m m m ( 1 ≤ n ≤ 200 1 \le n \le 200 1≤n≤200, 1 ≤ m ≤ 200 1 \leq m \leq 200 1≤m≤200).
The following n n n lines contain m m m integers each, the j j j-th element of the i i i-th line a i j a_{ij} aij is the number written in the j j j-th cell of the i i i-th row ( 0 ≤ a i j ≤ 1 0 6 ) (0\leq a_{ij} \leq 10^6) (0≤aij≤106)
It is guaranteed that the sum of n ⋅ m n\cdot m n⋅m over all test cases does not exceed 4 ⋅ 1 0 4 4\cdot10^4 4⋅104.
Output
For each test case output a single integer, the maximum sum over all possible placements of the bishop.
Example
input
4
4 4
1 2 2 1
2 4 2 4
2 2 3 1
2 4 2 4
2 1
1
0
3 3
1 1 1
1 1 1
1 1 1
3 3
0 1 1
1 0 1
1 1 0
output
20
1
5
3
Note
For the first test case here the best sum is achieved by the bishop being in this position:
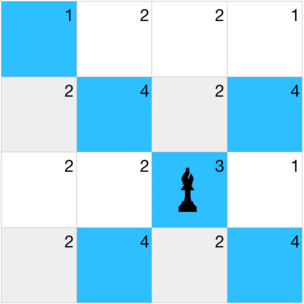
Tutorial
利用矩阵对角线性质,可以得出同一条对角线上的所有元素都满足
i
−
j
+
m
−
1
i - j + m - 1
i−j+m−1 , 同一条反对角线上的元素都满足
i
+
j
i + j
i+j,所以根据这个性质可以直接构造出
O
(
n
∗
m
)
O(n * m)
O(n∗m) 时间复杂度的方法
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, m, ans = 0;
cin >> n >> m;
int g[n][m];
vector<int> dig(n + m + 5), rdig(n + m + 5);
for (int i = 0; i < n; ++i) {
for (int j = 0; j < m; ++j) {
cin >> g[i][j];
dig[i - j + m - 1] += g[i][j];
rdig[i + j] += g[i][j];
}
}
for (int i = 0; i < n; ++i) {
for (int j = 0; j < m; ++j) {
ans = max(ans, dig[i - j + m - 1] + rdig[i + j] - g[i][j]);
}
}
cout << ans << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
E. Eating Queries
Timur has n n n candies. The i i i-th candy has a quantity of sugar equal to a i a_i ai. So, by eating the i i i-th candy, Timur consumes a quantity of sugar equal to a i a_i ai.
Timur will ask you q q q queries regarding his candies. For the j j j-th query you have to answer what is the minimum number of candies he needs to eat in order to reach a quantity of sugar greater than or equal to x j x_j xj or print -1 if it’s not possible to obtain such a quantity. In other words, you should print the minimum possible k k k such that after eating k k k candies, Timur consumes a quantity of sugar of at least x j x_j xj or say that no possible k k k exists.
Note that he can’t eat the same candy twice and queries are independent of each other (Timur can use the same candy in different queries).
Input
The first line of input contains a single integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases. The description of test cases follows.
The first line contains 2 2 2 integers n n n and q q q ( 1 ≤ n , q ≤ 1.5 ⋅ 1 0 5 1 \leq n, q \leq 1.5\cdot10^5 1≤n,q≤1.5⋅105) — the number of candies Timur has and the number of queries you have to print an answer for respectively.
The second line contains n n n integers a 1 , a 2 , … , a n a_1, a_2, \dots, a_n a1,a2,…,an ( 1 ≤ a i ≤ 1 0 4 1 \leq a_i \leq 10^4 1≤ai≤104) — the quantity of sugar in each of the candies respectively.
Then q q q lines follow.
Each of the next q q q lines contains a single integer x j x_j xj ( 1 ≤ x j ≤ 2 ⋅ 1 0 9 1 \leq x_j \leq 2 \cdot 10^9 1≤xj≤2⋅109) – the quantity Timur wants to reach for the given query.
It is guaranteed that the sum of n n n and the sum of q q q over all test cases do not exceed 1.5 ⋅ 1 0 5 1.5 \cdot 10^5 1.5⋅105.
Output
For each test case output q q q lines. For the j j j-th line output the number of candies Timur needs to eat in order to reach a quantity of sugar greater than or equal to x j x_j xj or print -1 if it’s not possible to obtain such a quantity.
Output
For each test case output a single integer, the maximum sum over all possible placements of the bishop.
Example
input
3
8 7
4 3 3 1 1 4 5 9
1
10
50
14
15
22
30
4 1
1 2 3 4
3
1 2
5
4
6
output
1
2
-1
2
3
4
8
1
1
-1
Note
For the first test case:
For the first query, Timur can eat any candy, and he will reach the desired quantity.
For the second query, Timur can reach a quantity of at least 10 10 10 by eating the 7 7 7-th and the 8 8 8-th candies, thus consuming a quantity of sugar equal to 14 14 14.
For the third query, there is no possible answer.
For the fourth query, Timur can reach a quantity of at least 14 14 14 by eating the 7 7 7-th and the 8 8 8-th candies, thus consuming a quantity of sugar equal to 14 14 14.
For the second test case:
For the only query of the second test case, we can choose the third candy from which Timur receives exactly 3 3 3 sugar. It’s also possible to obtain the same answer by choosing the fourth candy.
Tutorial
将数组从大到小排序后利用前缀和直接判断
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, q, x;
cin >> n >> q;
vector<int> a(n);
for (int &ai : a) {
cin >> ai;
}
ranges::sort(a, greater());
partial_sum(a.begin(), a.end(), a.begin());
while (q--) {
cin >> x;
if (x > a[n - 1]) {
cout << "-1\n";
} else {
cout << ranges::lower_bound(a, x) - a.begin() + 1 << endl;
}
}
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
F. Longest Strike
Given an array a a a of length n n n and an integer k k k, you are tasked to find any two numbers l l l and r r r ( l ≤ r l \leq r l≤r) such that:
- For each x x x ( l ≤ x ≤ r ) (l \leq x \leq r) (l≤x≤r), x x x appears in a a a at least k k k times (i.e. k k k or more array elements are equal to x x x).
- The value r − l r-l r−l is maximized.
If no numbers satisfy the conditions, output -1.
For example, if a = [ 11 , 11 , 12 , 13 , 13 , 14 , 14 ] a=[11, 11, 12, 13, 13, 14, 14] a=[11,11,12,13,13,14,14] and k = 2 k=2 k=2, then:
- for l = 12 l=12 l=12, r = 14 r=14 r=14 the first condition fails because 12 12 12 does not appear at least k = 2 k=2 k=2 times.
- for l = 13 l=13 l=13, r = 14 r=14 r=14 the first condition holds, because 13 13 13 occurs at least k = 2 k=2 k=2 times in a a a and 14 14 14 occurs at least k = 2 k=2 k=2 times in a a a.
- for l = 11 l=11 l=11, r = 11 r=11 r=11 the first condition holds, because 11 11 11 occurs at least k = 2 k=2 k=2 times in a a a.
A pair of l l l and r r r for which the first condition holds and r − l r-l r−l is maximal is l = 13 l = 13 l=13, r = 14 r = 14 r=14.
Input
The first line of the input contains a single integer t t t ( 1 ≤ t ≤ 1000 1 \le t \le 1000 1≤t≤1000) — the number of test cases. The description of test cases follows.
The first line of each test case contains the integers n n n and k k k ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \le n \le 2 \cdot 10^5 1≤n≤2⋅105, 1 ≤ k ≤ n 1 \leq k \leq n 1≤k≤n) — the length of the array a a a and the minimum amount of times each number in the range [ l , r ] [l, r] [l,r] should appear respectively.
Then a single line follows, containing n n n integers describing the array a a a ( 1 ≤ a i ≤ 1 0 9 1 \leq a_i \leq 10^9 1≤ai≤109).
It is guaranteed that the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each test case output 2 2 2 numbers, l l l and r r r that satisfy the conditions, or “-1” if no numbers satisfy the conditions.
If multiple answers exist, you can output any.
Example
input
4
7 2
11 11 12 13 13 14 14
5 1
6 3 5 2 1
6 4
4 3 4 3 3 4
14 2
1 1 2 2 2 3 3 3 3 4 4 4 4 4
output
13 14
1 3
-1
1 4
Tutorial
先将每个满足条件的元素统一放到一个数组中,再判断是否连续
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, k, x, ans = 0, cnt = 1, l = 0, r = 0;
cin >> n >> k;
vector<int> a;
map<int, int> mp;
for (int i = 0; i < n; ++i) {
cin >> x;
if (++mp[x] >= k) {
a.emplace_back(x);
}
}
if (a.empty()) {
cout << "-1\n";
return;
}
ranges::sort(a);
a.erase(unique(a.begin(), a.end()), a.end());
for (int i = 1; i < a.size(); ++i) {
if ((a[i] - a[i - 1]) ^ 1) {
if (cnt > ans) {
ans = cnt;
l = a[i - cnt], r = a[i - 1];
}
cnt = 1;
} else {
cnt++;
}
}
if (cnt > ans) {
ans = cnt;
l = a[a.size() - cnt], r = a[a.size() - 1];
}
cout << l << " " << r << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
G. White-Black Balanced Subtrees
You are given a rooted tree consisting of n n n vertices numbered from 1 1 1 to n n n. The root is vertex 1 1 1. There is also a string s s s denoting the color of each vertex: if s i = B s_i = \texttt{B} si=B, then vertex i i i is black, and if s i = W s_i = \texttt{W} si=W, then vertex i i i is white.
A subtree of the tree is called balanced if the number of white vertices equals the number of black vertices. Count the number of balanced subtrees.
A tree is a connected undirected graph without cycles. A rooted tree is a tree with a selected vertex, which is called the root. In this problem, all trees have root 1 1 1.
The tree is specified by an array of parents a 2 , … , a n a_2, \dots, a_n a2,…,an containing n − 1 n-1 n−1 numbers: a i a_i ai is the parent of the vertex with the number i i i for all i = 2 , … , n i = 2, \dots, n i=2,…,n. The parent of a vertex u u u is a vertex that is the next vertex on a simple path from u u u to the root.
The subtree of a vertex u u u is the set of all vertices that pass through u u u on a simple path to the root. For example, in the picture below, 7 7 7 is in the subtree of 3 3 3 because the simple path 7 → 5 → 3 → 1 7 \to 5 \to 3 \to 1 7→5→3→1 passes through 3 3 3. Note that a vertex is included in its subtree, and the subtree of the root is the entire tree.
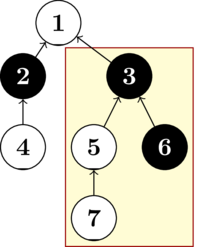
The picture shows the tree for n = 7 n=7 n=7, a = [ 1 , 1 , 2 , 3 , 3 , 5 ] a=[1,1,2,3,3,5] a=[1,1,2,3,3,5], and s = WBBWWBW s=\texttt{WBBWWBW} s=WBBWWBW. The subtree at the vertex 3 3 3 is balanced.
Input
The first line of input contains an integer t t t ( 1 ≤ t ≤ 1 0 4 1 \le t \le 10^4 1≤t≤104) — the number of test cases.
The first line of each test case contains an integer n n n ( 2 ≤ n ≤ 4000 2 \le n \le 4000 2≤n≤4000) — the number of vertices in the tree.
The second line of each test case contains n − 1 n-1 n−1 integers a 2 , … , a n a_2, \dots, a_n a2,…,an ( 1 ≤ a i < i 1 \le a_i < i 1≤ai<i) — the parents of the vertices 2 , … , n 2, \dots, n 2,…,n.
The third line of each test case contains a string s s s of length n n n consisting of the characters B \texttt{B} B and W \texttt{W} W — the coloring of the tree.
It is guaranteed that the sum of the values n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each test case, output a single integer — the number of balanced subtrees.
Example
input
3
7
1 1 2 3 3 5
WBBWWBW
2
1
BW
8
1 2 3 4 5 6 7
BWBWBWBW
output
2
1
4
Note
The first test case is pictured in the statement. Only the subtrees at vertices 2 2 2 and 3 3 3 are balanced.
In the second test case, only the subtree at vertex 1 1 1 is balanced.
In the third test case, only the subtrees at vertices 1 1 1, 3 3 3, 5 5 5, and 7 7 7 are balanced.
Tutorial
建图利用
D
F
S
DFS
DFS 直接判断有多少满足题意的子树
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, x, ans = 0;
char c;
cin >> n;
vector<int> g[n + 1], color(n + 1);
for (int i = 2; i <= n; ++i) {
cin >> x;
g[x].emplace_back(i);
}
for (int i = 1; i <= n; ++i) {
cin >> c;
color[i] = (c == 'W' ? -1 : 1);
}
function<int(int)> dfs = [&](int u) -> int {
int cnt = color[u];
for (int v : g[u]) {
cnt += dfs(v);
}
if (cnt == 0) {
ans++;
}
return cnt;
};
dfs(1);
cout << ans << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
H2. Maximum Crossings (Hard Version)
The only difference between the two versions is that in this version n ≤ 2 ⋅ 1 0 5 n \leq 2 \cdot 10^5 n≤2⋅105 and the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
A terminal is a row of n n n equal segments numbered 1 1 1 to n n n in order. There are two terminals, one above the other.
You are given an array a a a of length n n n. For all i = 1 , 2 , … , n i = 1, 2, \dots, n i=1,2,…,n, there should be a straight wire from some point on segment i i i of the top terminal to some point on segment a i a_i ai of the bottom terminal. You can’t select the endpoints of a segment. For example, the following pictures show two possible wirings if n = 7 n=7 n=7 and a = [ 4 , 1 , 4 , 6 , 7 , 7 , 5 ] a=[4,1,4,6,7,7,5] a=[4,1,4,6,7,7,5].

A crossing occurs when two wires share a point in common. In the picture above, crossings are circled in red.
What is the maximum number of crossings there can be if you place the wires optimally?
Input
The first line contains an integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases.
The first line of each test case contains an integer n n n ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \leq n \leq 2 \cdot 10^5 1≤n≤2⋅105) — the length of the array.
The second line of each test case contains n n n integers a 1 , a 2 , … , a n a_1, a_2, \dots, a_n a1,a2,…,an ( 1 ≤ a i ≤ n 1 \leq a_i \leq n 1≤ai≤n) — the elements of the array.
The sum of n n n across all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each test case, output a single integer — the maximum number of crossings there can be if you place the wires optimally.
Example
input
4
7
4 1 4 6 7 7 5
2
2 1
1
1
3
2 2 2
output
6
1
0
3
Note
The first test case is shown in the second picture in the statement.
In the second test case, the only wiring possible has the two wires cross, so the answer is 1 1 1.
In the third test case, the only wiring possible has one wire, so the answer is 0 0 0.
Tutorial
树状数组记录连过的位置,每到一个位置判断已经连过多少根线在目前为止的左边,即判断有多少个交叉点
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
#define lowbit(x) x & -x
const int N = 2e5 + 5;
int n, a;
int tree[N];
void add(int i, int x) {
while (i <= n) {
tree[i] += x;
i += lowbit(i);
}
}
int sum(int i) {
int cnt = 0;
while (i) {
cnt += tree[i];
i -= lowbit(i);
}
return cnt;
}
void solve() {
cin >> n;
memset(tree, 0, sizeof(tree));
int ans = 0;
for(int i = 0; i < n; ++i) {
cin >> a;
ans += sum(n) - sum(a - 1);
add(a, 1);
}
cout << ans << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}