默认插槽: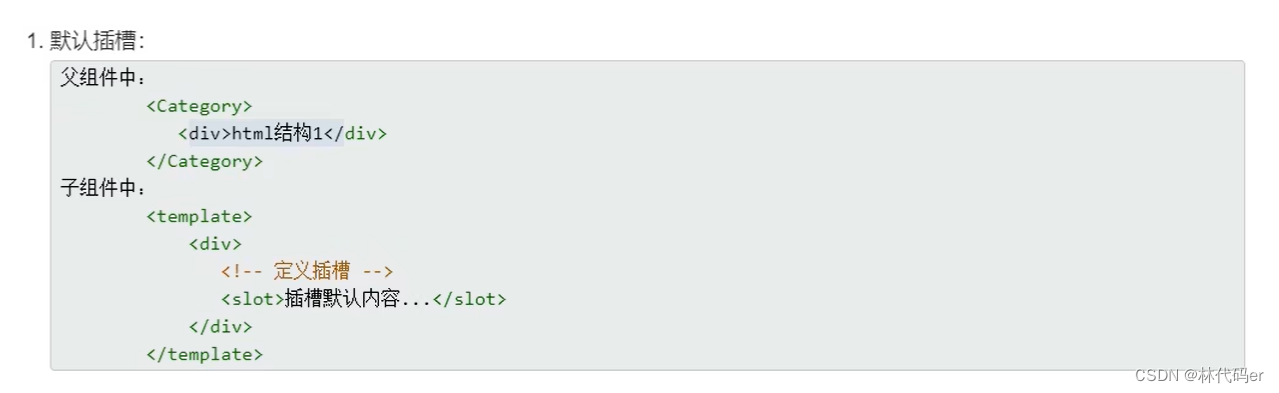
App.vue : 在app.vue中使用MyCategory,里面包裹的结构是不显示的,要想在页面中显示,就需要用到插槽。在子组件MyCategory中定义
<template>
<div class="container">
<MyCategory title="美食" :listData="foods">
<img src="https://img2.baidu.com/it/u=251961508,2775599777&fm=253&fmt=auto&app=120&f=JPEG?w=280&h=80" style="width: 200px;height: 200px" alt="">
</MyCategory>
<MyCategory title="游戏" :listData="games">
<ul>
<li v-for="(g,index) in games" :key="index">{{g}}</li>
</ul>
</MyCategory>
<MyCategory title="电影" :listData="films">
<video controls src="1186200849-1-16.mp4" style="width: 100%" alt=""></video>
</MyCategory>
</div>
</template>
<script>
import MyCategory from "@/components/Category";
export default {
name: 'App',
components: {MyCategory},
data(){
return {
foods:['火锅','烧烤','小龙虾','牛排'],
games:['红色警戒','穿越火线','qq飞车','和平精英'],
films:['《怦然心动》','《泰坦尼克号》','《魁拔》','《保你平安》']
}
}
}
</script>
<style>
.container{
display: flex;
justify-content: space-around;
}
</style>
Category.vue :在想要放入结构的位置,插入<solt></solt>默认插槽,app.vue中在子标签中写的结构才会被插入到插槽的位置。插槽标签里写的文本只有在插槽没数据时候才会显示。
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- <li v-for="(item,index) in listData" :key="index">{{ item }}</li>-->
<slot>我是一些默认值,当使用者没有传递具体结构时候,我会出现</slot>
</div>
</template>
<script>
export default {
name: "MyCategory",
props:['title','listData']
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
img{
width: 100%;
}
</style>
具名插槽:
App.vue : 可以用多个插槽,插入在不同位置,需要在子组件中定义<solt name="xxx"></slot>加上name属性名
<template>
<div class="container">
<MyCategory title="美食" :listData="foods">
<img slot="center" src="https://img2.baidu.com/it/u=251961508,2775599777&fm=253&fmt=auto&app=120&f=JPEG?w=280&h=80" style="width: 200px;height: 200px" alt="">
<a slot="footer" href="#">更多美食</a>
</MyCategory>
<MyCategory title="游戏" :listData="games">
<ul slot="center">
<li v-for="(g,index) in games" :key="index">{{g}}</li>
</ul>
<div slot="footer" class="footer">
<a href="#">单机游戏</a>
<a href="#">网络游戏</a>
</div>
</MyCategory>
<MyCategory title="电影" :listData="films">
<video slot="center" controls src="1186200849-1-16.mp4" style="width: 100%" alt=""></video>
<template slot="footer">
<div class="footer">
<a href="#">经典</a>
<a href="#">搞笑</a>
<a href="#">动作</a>
</div>
<h4>欢迎前来观影</h4>
</template>
</MyCategory>
</div>
</template>
<script>
import MyCategory from "@/components/Category";
export default {
name: 'App',
components: {MyCategory},
data(){
return {
foods:['火锅','烧烤','小龙虾','牛排'],
games:['红色警戒','穿越火线','qq飞车','和平精英'],
films:['《怦然心动》','《泰坦尼克号》','《魁拔》','《保你平安》']
}
}
}
</script>
<style>
.container,.footer{
display: flex;
justify-content: space-around;
}
h4{
text-align: center;
}
</style>
Category.vue :<slot name="xxx"></slot>就可以定义多个插槽,放在不同位置
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- <li v-for="(item,index) in listData" :key="index">{{ item }}</li>-->
<slot name="center">我是一些默认值,当使用者没有传递具体结构时候,我会出现</slot>
<slot name="footer">我是一些默认值,当使用者没有传递具体结构时候,我会出现</slot>
</div>
</template>
<script>
export default {
name: "MyCategory",
props:['title','listData']
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
img{
width: 100%;
}
</style>
作用域插槽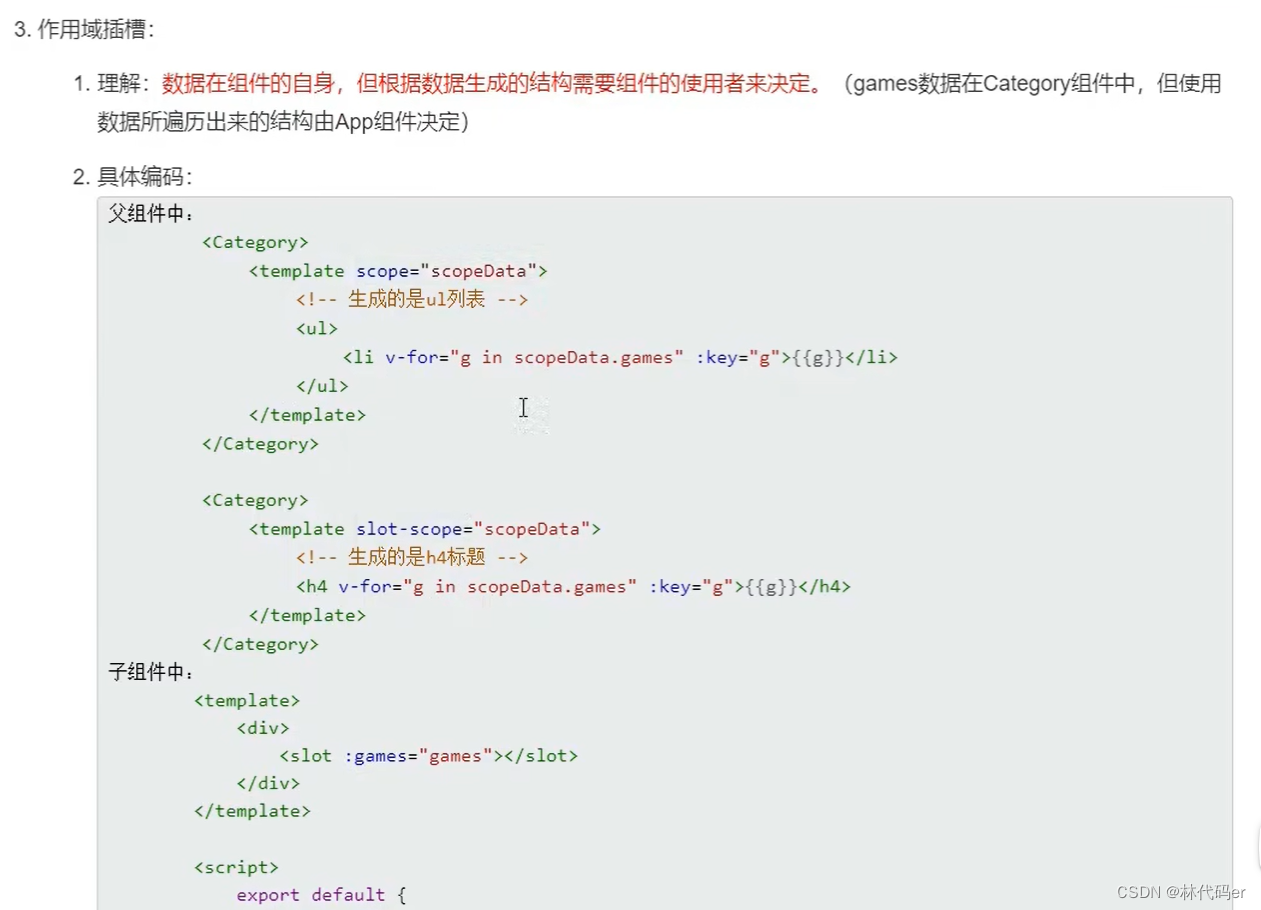
App.vue:最实用的一种,可以实现子组件向父组件的逆向传数据。 父组件中:<template scope="liner"></template> ,外面必须包着template标签,scope用来接收<slot>中传过来的数据,scope这里面的名子自己定义,取games数据时候用自己定义的scope中的名字来取xxx.games,如<slot>中定义了其他数据,也可以xxx.msg,这样取定义的其他数据。
<template>
<div class="container">
<MyCategory title="游戏">
<template scope="liner">
<ul>
<li v-for="(g,index) in liner.games" :key="index">{{g}}</li>
</ul>
</template>
</MyCategory>
<MyCategory title="游戏">
<template scope="liners">
<ol style="color: #bd362f">
<li v-for="(g,index) in liners.games" :key="index">{{g}}</li>
</ol>
</template>
</MyCategory>
<MyCategory title="游戏">
<template scope="linerz">
<h4 v-for="(g,index) in linerz.games" :key="index">{{g}}</h4>
</template>
</MyCategory>
</div>
</template>
<script>
import MyCategory from "@/components/Category";
export default {
name: 'App',
components: {MyCategory},
}
</script>
<style>
.container,.footer{
display: flex;
justify-content: space-around;
}
h4{
text-align: center;
}
</style>
Category.vue : 子组件中:<slot :games="games"></slot> 将data中的数据games绑定给games,给插槽传递了games数据
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- 1.子组件中:<slot :games="games">我是默认的一些内容</slot> 将data中的数据games绑定给games,给插槽传递了games数据
2.父组件中:<template scope="liner"></template> ,外面必须包着template标签,scope用来取slot中传过来的数据,scope这里面的名子自己定义,取games数据时候用自己定义的scope中的名字xxx.games
-->
<slot :games="games">我是默认的一些内容</slot>
</div>
</template>
<script>
export default {
name: "MyCategory",
props:['title'],
data(){
return {
games:['红色警戒','穿越火线','qq飞车','和平精英'],
}
}
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
img{
width: 100%;
}
</style>