seleinum框架
框架的思想:
解决我们测试过程中的问题:大量的重复步骤,用自动化来实现
1)配置和程序的分离
2)测试数据和程序的分离
3)不懂编程的人员可以方便使用:使用的时候不需要写程序
4)有日志功能,实现无人值守
5)自动发报告
6)框架中不要有重复的代码,实现高度的封装和复用
推荐使用关键字驱动、混合驱动
为什么要编写程序呢?
通用性的东西满足不了个性化的需求
测试的工具:python+selenium
接口测试用例:excel
一、搭建自动化测试的目录结构
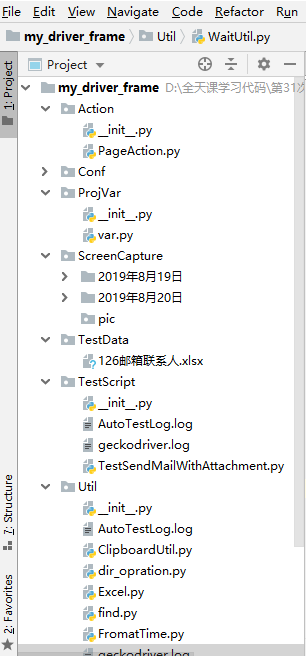
分层如下:
1、Action包: 放置关键字文件
2、Config目录: 所有配置文件,日志的配置文件;把所有的项目相关的配置均放到这里,用python支持较好的配置文件格式ini进行配置。实现配置与代码的分离
3、Projvar包:项目所有的公共变量,如:目录、自定义变量名称;
4、ScreenCapture目录:存放截屏目录,按照年月日小时来建目录;
5、TestData目录:放测试将数据文件,可以把所有的testcase的参数化相关文件放在这里,一般采用xlsx、xml等格式。实现数据与代码分离;
6、TestScript包:测试脚本,主程序
7、Util包:封装的公共的类,包括读取config的类,写log的类,读取excel、xml
的类、生成报告的类(如HTMLTestRunner)、数据库的连接、发送邮件等类和方法,都在这里
8、ReadMe.txt(加个说明性文件,告诉团队框架需要使用的环境及方法)
二、搭建步骤
在ProjVar包创建一个var.py用来存放公共变量
import os
#浏览器驱动存放的位置
firefoxDriverFilePath = "e:\\geckodriver"
chromeDriverFilePath = "e:\\chromedriver"
ieDriverFilePath = "e:\\IEDriverServer"
# 当前文件所在目录的父目录的绝对路径
ProjDirPath = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
test_step_id_col_no = 0
test_step_result_col_no = 8
test_step_error_info_col_no = 9
test_step_capture_pic_path_col_no = 10
test_case_id_col_no= 0
test_case_sheet_name=2
test_case_is_executed_flag_col_no= 3
test_case_is_hybrid_test_data_sheet_col_no=4
test_case_start_time_col_no= 6
test_case_end_time_col_no= 7
test_case_elapsed_time_col_no= 8
test_case_result_col_no = 9
if __name__=="__mian__":
print(ProjDirPath)
2、在Utli包里面创建公共的类、函数
2.1封装excel的类
from openpyxl import *
import os.path
from openpyxl.styles import NamedStyle,Font, colors
from ProjVar.var import *
from Util.FromatTime import *
class Excel:
def __init__(self,excel_file_path):
if os.path.exists(excel_file_path):
self.excel_file_path = excel_file_path
self.wb = load_workbook(self.excel_file_path)
else:
print("%s e文件的路劲不存在,请重新设定!" %excel_file_path)
#通过sheet名字来获取操作的sheet
def set_sheet_by_name(self,sheet_name):
if sheet_name in self.wb.sheetnames:
self.sheet = self.wb[sheet_name]
else:
print("%s sheet不存在,请重新指定!" %sheet_name)
#通过序号来获取操作的sheet
def set_sheet_by_index(self,index):
if isinstance(index,int) and 1<= index <= len(self.get_all_sheet_names()):
sheet_name = self.get_all_sheet_names()[index-1]
self.sheet = self.wb[sheet_name]
else:
print("%s sheet 序号不存在,请重新设定" %index)
#获取当前sheet和title的名称
def get_current_sheet_name(self):
return self.sheet.title
#获取所有sheet的名称
def get_all_sheet_names(self):
return self.wb.sheetnames
#获取sheet的总行数,从0开始,返回list
def get_rows_object(self):
return list(self.sheet.rows)
#获取sheet的总列数,从0开始,返回list
def get_cols_object(self):
return list(self.sheet.columns)
#获取某行的对象,第一行从0开始
def get_row(self,row_no):
return self.get_rows_object()[row_no]
#获取某一列对象,第一列从0开始
def get_col(self,col_no):
return self.get_cols_object()[col_no]
#获取某个单元格对象
def get_cell_value(self,row_no,col_no):
if isinstance(row_no,int) and isinstance(col_no,int) and \
1<=row_no<=len(self.get_rows_object()) and \
1 <= row_no <= len(self.get_cols_object()):
return self.sheet.cell(row=row_no, column=col_no).value
else:
print("%s,%s 行号或者列号不存在,请重新设定行号或者列表读取!" % (row_no,col_no))
#给某一个单元格写入指定内容,行号、列号从1开始
#调用此方法时,excel不要处于打开状态
def write_cell_value(self,row_no,col_no,value,color = None):
if isinstance(row_no,int) and isinstance(col_no,int):
if color is None:
font = Font(bold=False, size=10, color=colors.BLACK)
self.sheet.cell(row=row_no, column=col_no).font = font
self.sheet.cell(row=row_no, column=col_no).value = value
elif color == "green":
font = Font(bold=True, size=13, color=colors.GREEN)
self.sheet.cell(row=row_no, column=col_no).font = font
self.sheet.cell(row=row_no, column=col_no).value = value
elif color == "red":
font = Font(bold=True, size=13, color=colors.RED)
self.sheet.cell(row=row_no, column=col_no).font = font
self.sheet.cell(row=row_no, column=col_no).value = value
self.wb.save(self.excel_file_path)
else:
print("%s,%s 行号或者列号不是数字,请重新设定行号或者列表读取!" % (row_no, col_no))
def write_current_time(self,row_no,col_no):
if isinstance(row_no, int) and isinstance(col_no, int):
self.sheet.cell(row=row_no, column=col_no).value = get_current_date_and_time()
self.wb.save(self.excel_file_path)
if __name__ == "__main__":
excel_file_path = ProjDirPath+r"\TestData\126邮箱联系人.xlsx"
#print(excel_file_path )
excel_obj = Excel(excel_file_path)
#Excel("e:\\a.xlsx") #测试路劲不存在的情况
# excel_obj.set_sheet_by_name("测试邮件")
# excel_obj.set_sheet_by_name("测试邮件1111")
excel_obj.set_sheet_by_index(1)
# print(excel_obj.get_current_sheet_name())
#excel_obj.set_sheet_by_index(5)
#print(excel_obj.get_rows_object())
#print(excel_obj.get_cols_object())
#print(excel_obj.get_row(2))
print(excel_obj.get_cell_value(2,2))
print(excel_obj.write_cell_value(5,7,"hello~~"))
print(excel_obj.write_current_time(6,8))
2.2 封装获取页面元素的类
from selenium.webdriver.support.ui import WebDriverWait
import time
#获取单个页面元素对象
def getElement(driver,localtorType,localtorExpression):
try:
element = WebDriverWait(driver,5).until(lambda x:x.find_element(by = localtorType,value=localtorExpression))
return element
except Exception as e:
raise e
#获取多个页面元素对象
def getElement(driver,localtorType,localtorExpression):
try:
elements = WebDriverWait(driver,5).until(lambda x:x.find_elements(by=localtorType,value=localtorExpression))
return elements
except Exception as e:
raise e
if __name__ =="__main__":
from selenium import webdriver
#进行单元测试
driver = webdriver.Firefox(executable_path="e:\\geckodriver")
driver.maximize_window()
driver.get("https://mail.126.com/")
time.sleep(2)
lb = getElement(driver,"id","lbNormal")
print(lb)
driver.quit()
2.3 封装获取当前日期、时间的类
import time
import datetime
import locale
# 获取当前的日期
def get_current_date():
time_tup = time.localtime()
current_date = str(time_tup.tm_year) + "年" + \
str(time_tup.tm_mon) + "月" + str(time_tup.tm_mday)+"日"
return current_date
# 获取当前的时间
def get_current_time():
time_str = datetime.datetime.now()
locale.setlocale(locale.LC_CTYPE, 'chinese')
now_time = time_str.strftime('%H点%M分钟%S秒')
return now_time
# 获取当前的时间
def get_current_hour():
time_str = datetime.datetime.now()
now_hour = time_str.strftime('%H')
return now_hour
def get_current_date_and_time():
return get_current_date()+" "+get_current_time()
if __name__ == "__main__":
print( get_current_date())
print(get_current_time())
print(get_current_hour())
print(get_current_date_and_time())
2.4 封装创建目录的类
import os
from Util.FromatTime import *
from ProjVar.var import *
def make_dir(dir_path):
if not os.path.exists(dir_path):
try:
os.mkdir(dir_path)
except Exception as e:
print("创建%s目录失败" %dir_path)
raise e
def make_current_date_dir(default_dir_path = None):
if default_dir_path is None:
dir_path = get_current_date()
else:
dir_path = os.path.join(default_dir_path,get_current_date())
if not os.path.exists(dir_path):
try:
os.mkdir(dir_path)
except Exception as e:
print("创建%s目录失败" % dir_path)
raise e
return dir_path
def make_current_hour_dir(default_dir_path = None):
if default_dir_path is None:
dir_path = get_current_hour()
else:
dir_path = os.path.join(default_dir_path,get_current_hour())
if not os.path.exists(dir_path):
try:
os.mkdir(dir_path)
except Exception as e:
print("创建%s目录失败" % dir_path)
raise e
return dir_path
if __name__ == "__main__":
make_dir(ProjDirPath+"\\"+"ScreenCapture\\"+"pic")
dir_path = make_current_date_dir(ProjDirPath+"\\"+"ScreenCapture\\")
dir_path=make_current_hour_dir(dir_path+"\\")
print(dir_path)
2.5 封装日志的类
#encoding=utf-8
import logging.config
import logging
from ProjVar.var import ProjDirPath
print(ProjDirPath+"\\Conf\\"+"Logger.conf")
#读取日志配置文件
logging.config.fileConfig(ProjDirPath+"\\Conf\\"+"Logger.conf")
#选择一个日志格式
logger = logging.getLogger("example01")
def debug(message):
print ("debug")
logger.debug(message)
def info(message):
logger.info(message)
def error(message):
print("error")
logger.error(message)
def warning(message):
logger.warning(message)
if __name__=="__main__":
debug("hi")
info("gloryroad")
warning("hello")
error("something error!")
2.6 封装显示等待的类
#encoding=utf-8
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
class WaitUtil(object):
def __init__(self,driver):
self.locationTypeDict = {
"xpath":By.XPATH,
"id":By.ID,
"name":By.NAME,
"css_selector":By.CSS_SELECTOR,
"class_name":By.CLASS_NAME,
"tag_name":By.TAG_NAME,
"link_text":By.LINK_TEXT,
"partial_link_text":By.PARTIAL_LINK_TEXT,
}
self.driver = driver
self.wait = WebDriverWait(driver,30)
def presenceOfElementLocated(self,locatorMethod,locatorExpression,*args):
"""显式等待页面元素出现在DOM中,但并不一定可见,存在则返回该页面元素"""
try:
#if self.locationTypeDict.has_key(locatorMethod.lower()):
if locatorMethod.lower() in self.locationTypeDict:
self.wait.until(EC.presence_of_element_located((self.locationTypeDict[locatorMethod.lower()],locatorExpression)))
else:
raise Exception(u"未找到定位方式,请确认定位方式是否书写正确")
except Exception as e:
raise e
def frameToBeAvailableAndSwitchToIt(self,locationType,locatorExpression,*args):
"""检查frame是否存在,存在则切换进frame控件中"""
try:
self.wait.until(EC.frame_to_be_available_and_switch_to_it((self.locationTypeDict[locationType.lower()],locatorExpression)))
except Exception as e:
raise e
def visibilityOfElementLocated(self,locationMethod,locatorExperssion,*args):
"""显式等待页面元素出现在DOM中,并且可见,存在则返回该页面元素对象"""
try:
self.wait.until(EC.visibility_of_element_located((self.locationTypeDict[locationMethod.lower()],locatorExperssion)))
except Exception as e:
raise e
if __name__ == "__main__":
from selenium import webdriver
driver = webdriver.Firefox(executable_path = "e:\\geckodriver")
driver.get("https://mail.126.com/")
waitUtil = WaitUtil(driver)
driver.find_element_by_id("lbNormal").click()
# waitUtil.presenceOfElementLocated("id","lbNormal")
waitUtil.frameToBeAvailableAndSwitchToIt("xpath",'//iframe[contains(@id,"x-URS-iframe")]')
waitUtil.visibilityOfElementLocated("xpath","//input[@name='email']")
waitUtil.presenceOfElementLocated("xpath","//input[@name='email']")
driver.quit()
## 根据需求如果还用其他的类,封装好后放在此目下面 ##
3、在action包里面,创建关键字函数
#encoding=utf-8
from selenium import webdriver
from ProjVar.var import *
from Util.find import *
from Util.KeyBoardUtil import KeyBoardKeys
from Util.ClipboardUtil import Clipboard
from Util.WaitUtil import WaitUtil
from selenium.webdriver.firefox.options import Options
from selenium.webdriver.chrome.options import Options
import time
from Util.FromatTime import *
#定义全局变量driver
driver = None
#定义全局的等待类实例对象
waitUtil = None
def open(browserName):
#打开浏览器
global driver,waitUtil
try:
if browserName.lower() == "ie":
driver = webdriver.Ie(executable_path = ieDriverFilePath)
elif browserName.lower == "chrome":
#创建Chrome浏览器的一个Options实例对象
chrome_options = Options()
#添加屏蔽--ignore--certificate--errors提示信息的设置参数项
chrome_options.add_experimental_option("excludeSwitches",["ignore-certificate-errors"])
driver = webdriver.Chrome(executable_path = chromeDriverFilePath,chrome_options = chrome_options)
else:
driver = webdriver.Firefox(executable_path = firefoxDriverFilePath)
#driver对象创建成功后,创建等待类实例对象
waitUtil = WaitUtil(driver)
except Exception as e:
raise e
def visit(url):
#访问某个网站
global driver
try:
driver.get(url)
except Exception as e:
raise e
def close_browser():
#关闭浏览器
global driver
try:
driver.quit()
except Exception as e:
raise e
def sleep(sleepSeconds):
#强制等待
try:
time.sleep(int(sleepSeconds))
except Exception as e:
raise e
def clear(locationType,locatorExpression):
#清空输入框默认内容
global driver
try:
getElement(driver,locationType,locatorExpression).clear()
except Exception as e:
raise e
def input_string(locationType,locatorExpression,inputContent):
#在页面输入框中输入数据
global driver
try:
getElement(driver,locationType,locatorExpression).send_keys(inputContent)
except Exception as e:
raise e
def click(locationType,locatorExpression,*args):
#点击页面元素
global driver
try:
getElement(driver,locationType,locatorExpression).click()
except Exception as e:
raise e
def assert_string_in_pagesource(assertString,*args):
#断言页面源码是否存在某个关键字或关键字符串
global driver
try:
assert assertString in driver.page_source,u"%s not found in page source!" % assertString
except AssertionError as e:
raise AssertionError(e)
except Exception as e:
raise e
def assert_title(titleStr,*args):
#断言页面标题是否存在给定的关键字符串
global driver
try:
assert titleStr in driver.title,u"%s not found in page title!" % titleStr
except AssertionError as e:
raise AssertionError(e)
except Exception as e:
raise e
def getTitle(*args):
#获取页面标题
global driver
try:
return driver.title
except Exception as e:
raise e
def getPageSource(*args):
#获取页面源码
global driver
try:
return driver.page_source
except Exception as e:
raise e
def switch_to_frame(locationType,frameLocatorExpressoin,*args):
#切换进frame
global driver
try:
driver.switch_to.frame(getElement(driver,locationType,frameLocatorExpressoin))
except Exception as e:
print("frame error!")
raise e
def switch_to_default_content(*args):
#切换妯frame
global driver
try:
driver.switch_to.default_content()
except Exception as e:
raise e
def paste_string(pasteString,*args):
#模拟Ctrl+V操作
try:
Clipboard.setText(pasteString)
#等待2秒,防止代码执行过快,而未成功粘贴内容
time.sleep(2)
KeyBoardKeys.twoKeys("ctrl","v")
except Exception as e:
raise e
def press_tab_key(*args):
#模拟tab键
try:
KeyBoardKeys.oneKey("tab")
except Exception as e:
raise e
def press_enter_key(*args):
#模拟enter键
try:
KeyBoardKeys.oneKey("enter")
except Exception as e:
raise e
def maximize(*args):
#窗口最大化
global driver
try:
driver.maximize_window()
except Exception as e:
raise e
def capture(file_path):
try:
driver.save_screenshot(file_path)
except Exception as e:
raise e
def waitPresenceOfElementLocated(locationType,locatorExpression,*args):
"""显式等待页面元素出现在DOM中,但不一定可见,存在则返回该页面元素对象"""
global waitUtil
try:
waitUtil.presenceOfElementLocated(locationType,locatorExpression)
except Exception as e:
raise e
def waitFrameToBeAvailableAndSwitchToIt(locationType,locatorExprssion,*args):
"""检查frame是否存在,存在则切换进frame控件中"""
global waitUtil
try:
waitUtil.frameToBeAvailableAndSwitchToIt(locationType,locatorExprssion)
except Exception as e:
raise e
def waitVisibilityOfElementLocated(locationType,locatorExpression,*args):
"""显式等待页面元素出现在Dom中,并且可见,存在返回该页面元素对象"""
global waitUtil
try:
waitUtil.visibilityOfElementLocated(locationType,locatorExpression)
except Exception as e:
raise e
4、在Config目录下存放所有配置文件,比如:日志的配置文件;把所有的项目相关的配置均放到这里,实现配置与代码的分离
日志配置文件
[loggers]
keys=root,example01,example02
[logger_root]
level=DEBUG
handlers=hand01,hand02
[logger_example01]
handlers=hand01,hand02
qualname=example01
propagate=0
[logger_example02]
handlers=hand01,hand03
qualname=example02
propagate=0
###############################################
[handlers]
keys=hand01,hand02,hand03
[handler_hand01]
class=StreamHandler
level=DEBUG
formatter=form01
args=(sys.stderr,)
[handler_hand02]
class=FileHandler
level=DEBUG
formatter=form01
args=('AutoTestLog.log', 'a')
[handler_hand03]
class=handlers.RotatingFileHandler
level=INFO
formatter=form01
args=('AutoTestLog.log', 'a', 10*1024*1024, 5)
###############################################
[formatters]
keys=form01,form02
[formatter_form01]
format=%(asctime)s %(filename)s[line:%(lineno)d] %(levelname)s %(message)s
datefmt=%Y-%m-%d %H:%M:%S
[formatter_form02]
format=%(name)-12s: %(levelname)-8s %(message)s
datefmt=%Y-%m-%d %H:%M:%S
5、在ProjVar包里面存放项目所有的公共变量,如:目录、自定义变量名称;
import os
#浏览器驱动存放的位置
firefoxDriverFilePath = "e:\\geckodriver"
chromeDriverFilePath = "e:\\chromedriver"
ieDriverFilePath = "e:\\IEDriverServer"
# 当前文件所在目录的父目录的绝对路径
ProjDirPath = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
test_step_id_col_no = 0
test_step_result_col_no = 8
test_step_error_info_col_no = 9
test_step_capture_pic_path_col_no = 10
test_case_id_col_no= 0
test_case_sheet_name=2
test_case_is_executed_flag_col_no= 3
test_case_is_hybrid_test_data_sheet_col_no=4
test_case_start_time_col_no= 6
test_case_end_time_col_no= 7
test_case_elapsed_time_col_no= 8
test_case_result_col_no = 9
if __name__=="__mian__":
print(ProjDirPath)
6、ScreenCapture目录:所有生成的日志、截屏报告均放在这里
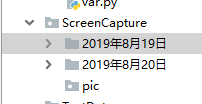
7、TestData目录:存放测试文件
126邮箱联系人.xlsx
8、TestScript包用来创建测试脚本,主程序
#encoding=utf-8
import re
from Util.dir_opration import make_current_date_dir, make_current_hour_dir
from Util.Excel import *
from Action.PageAction import *
import traceback
from Util.log import *
def get_test_case_sheet(test_cases_excel_path):
test_case_sheet_names = []
excel_obj = Excel(test_cases_excel_path)
excel_obj.set_sheet_by_index(1)
test_case_rows = excel_obj.get_rows_object()[1:]
for row in test_case_rows:
if row[3].value=='y':
print(row[2].value)
test_case_sheet_names.append((int(row[0].value)+1,row[2].value))
return test_case_sheet_names
#print(get_test_case_sheet(ProjDirPath+"\\TestData\\126邮箱联系人.xlsx"))
def execute(test_cases_excel_path,row_no,test_case_sheet_name):
excel_obj = Excel(test_cases_excel_path)
excel_obj.set_sheet_by_name(test_case_sheet_name)
#获取除第一行之外的所有行对象
test_step_rows = excel_obj.get_rows_object()[1:]
#拼接开始时间:当前年月日+当前时间
start_time = get_current_date_and_time()
#开始计时
start_time_stamp = time.time()
#设置默认用例时执行成功的,如果抛异常则说明用例执行失败
test_result_flag = True
for test_step_row in test_step_rows:
if test_step_row[6].value == "y":
test_action = test_step_row[2].value
locator_method = test_step_row[3].value
locator_exp = test_step_row[4].value
test_value = test_step_row[5].value
print(test_action,locator_method,locator_exp,test_value)
if locator_method is None:
if test_value is None:
command = test_action + "()"
else:
command = test_action + "('%s')" %test_value
else:
if test_value is None:
command = test_action + "('%s','%s')" %(locator_method,locator_exp)
else:
command = test_action + "('%s','%s','%s')" %(locator_method,locator_exp,test_value)
print(command)
eval(command)
#处理异常
try:
info(command)
eval(command)
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_result_col_no, "执行成功")
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_error_info_col_no, "")
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_capture_pic_path_col_no, "")
info("执行成功") # 加入日志信息
except Exception as e:
test_result_flag = False
traceback.print_exc()
error(command + ":" + traceback.format_exc()) # 加入日志信息
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_result_col_no, "失败", "red")
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_error_info_col_no, \
command + ":" + traceback.format_exc())
dir_path = make_current_date_dir(ProjDirPath + "\\" + "ScreenCapture\\")
dir_path = make_current_hour_dir(dir_path + "\\")
pic_path = os.path.join(dir_path, get_current_time() + ".png")
capture(pic_path)
excel_obj.write_cell_value(int(test_step_row[0].value) + 1, test_step_capture_pic_path_col_no, pic_path)
# 拼接结束时间:年月日+当前时间
end_time = get_current_date() + " " + get_current_time()
# 计时结束时间
end_time_stamp = time.time()
# 执行用例时间等于结束时间-开始时间;需要转换数据类型int()
elapsed_time = int(end_time_stamp - start_time_stamp)
# 将时间转换成分钟;整除60
elapsed_minutes = int(elapsed_time // 60)
# 将时间转换成秒;除60取余
elapsed_seconds = elapsed_time % 60
# 拼接用例执行时间;分+秒
elapsed_time = str(elapsed_minutes) + "分" + str(elapsed_seconds) + "秒"
# 判断用例是否执行成功
if test_result_flag:
test_case_result = "测试用例执行成功"
else:
test_case_result = "测试用例执行失败"
# 需要写入的时第一个sheet
excel_obj.set_sheet_by_index(1)
# 写入开始时间
excel_obj.write_cell_value(int(row_no), test_case_start_time_col_no, start_time)
# 写入结束时间
excel_obj.write_cell_value(int(row_no), test_case_end_time_col_no, end_time)
# 写入执行时间
excel_obj.write_cell_value(int(row_no), test_case_elapsed_time_col_no, elapsed_time)
# 写入执行结果;
if test_result_flag:
# 用例执行成功,写入执行结果
excel_obj.write_cell_value(int(row_no), test_case_result_col_no, test_case_result)
else:
# 用例执行失败,用red字体写入执行结果
excel_obj.write_cell_value(int(row_no), test_case_result_col_no, test_case_result, "red")
# 清理excel记录的结果数据
def clear_test_data_file_info(test_data_excel_file_path):
excel_obj = Excel(test_data_excel_file_path)
excel_obj.set_sheet_by_index(1)
test_case_rows = excel_obj.get_rows_object()[1:]
for test_step_row in test_case_rows:
excel_obj.set_sheet_by_index(1)
if test_step_row[test_case_is_executed_flag_row_no].value == "y":
excel_obj.write_cell_value(
int(test_step_row[test_case_id_col_no].value) + 1, test_case_start_time_col_no, "")
excel_obj.write_cell_value(
int(test_step_row[test_case_id_col_no].value) + 1, test_case_end_time_col_no, "")
excel_obj.write_cell_value(
int(test_step_row[test_case_id_col_no].value) + 1, test_case_elapsed_time_col_no, "")
excel_obj.write_cell_value(
int(test_step_row[test_case_id_col_no].value) + 1, test_case_result_col_no, "")
excel_obj.set_sheet_by_name(test_step_row[test_case_sheet_name].value)
test_step_rows = excel_obj.get_rows_object()[1:]
for test_step_row in test_step_rows:
if test_step_row[test_step_id_col_no].value is None:
continue
excel_obj.write_cell_value(
int(test_step_row[test_step_id_col_no].value) + 1, test_step_result_col_no, "")
excel_obj.write_cell_value(
int(test_step_row[test_step_id_col_no].value) + 1, test_step_error_info_col_no, "")
excel_obj.write_cell_value(
int(test_step_row[test_step_id_col_no].value) + 1, test_step_capture_pic_path_col_no, "")
if __name__ == "__main__":
test_data_excel_file_path = ProjDirPath + "\\TestData\\126邮箱联系人.xlsx"
print(get_test_case_sheet(test_data_excel_file_path))
execute(test_data_excel_file_path,2,"登录")