什么是AJAX
AJAX = Asynchronous JavaScript and XML(异步的 JavaScript 和 XML)
Ajax 不是一种新的编程语言,而是一种用于创建更好更快以及交互性更强的Web应用程序的技术。
AJAX 是一种在无需重新加载整个网页的情况下,能够更新部分网页的技术。
利用AJAX可以做什么
注册时,输入用户名自动检测用户是否已经存在。
登陆时,提示用户名密码错误
删除数据行时,将行ID发送到后台,后台在数据库中删除,数据库删除成功后,在页面DOM中将数据行也删除。
…等等
简单使用
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<filter>
<filter-name>encoding</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encoding</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
" target="_blank">https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 自动扫描指定的包,下面所有注解类交给IOC容器管理 -->
<context:component-scan base-package="com.kuang.controller"/>
<mvc:default-servlet-handler />
<mvc:annotation-driven />
<!-- 视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"
id="internalResourceViewResolver">
<!-- 前缀 -->
<property name="prefix" value="/WEB-INF/jsp/" />
<!-- 后缀 -->
<property name="suffix" value=".jsp" />
</bean>
</beans>
注意: <mvc:default-servlet-handler />这个很重要,没有它,使用ajax会报404的错误。
AjaxController
@Controller
public class AjaxController {
@RequestMapping("/a1")
public void ajax1(String name , HttpServletResponse response) throws IOException {
if ("admin".equals(name)){
response.getWriter().print("true");
}else{
response.getWriter().print("false");
}
}
}
index.jsp
需要下载jQuery
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>$Title$</title>
<%--<script src="--%>" target="_blank">https://code.jquery.com/jquery-3.1.1.min.js"></script>--%>
<script src="${pageContext.request.contextPath}/statics/js/jquery-3.1.1.min.js"></script>
<script>
function a1(){
$.post({
url:"${pageContext.request.contextPath}/a1",
data:{'name':$("#txtName").val()},
success:function (data,status) {
alert(data);
alert(status);
}
});
}
</script>
</head>
<body>
<%--onblur:失去焦点触发事件--%>
用户名:<input type="text" id="txtName" οnblur="a1()"/>
</body>
</html>
启动tomcat测试
打开浏览器的控制台,当我们鼠标离开输入框的时候,可以看到发出了一个ajax的请求!是后台返回给我们的结果!测试成功!
测试数据异步加载
AjaxController
后端导入数据
@RequestMapping("a2")
public List<User> a2(){
List<User> userList= new ArrayList<>();
//添加数据
userList.add(new User("菜菜",1,"男"));
userList.add(new User("前端",1,"女"));
userList.add(new User("运维",2,"男"));
return userList;
}
建立实体类
User
package com.cjh.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
private String name;
private int age;
private String sex;
}
注意:记得导入lombok包
建立动态页面
test2.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
<script src="" target="_blank">https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(function (){
$("#btn").click(function (){
/*
* $.post(url,param(可以省略),success)
* */
$.post("${pageContext.request.contextPath}/a2",function (data){
console.log(data);
})
})
});
</script>
</head>
<body>
<input type="button" value="加载数据" id="btn">
<table>
<tr>
<td>姓名</td>
<td>年龄</td>
<td>性别</td>
</tr>
<tbody>
<%--数据在后台--%>
</tbody>
</table>
</body>
</html>
注意:我们在使用Script的时候要逐步测试使用,不然后面会影响开发效率。
这里我们先测试一下,能不能拿到后端数据,拿到后端数据的方法就是写请求。
结果:
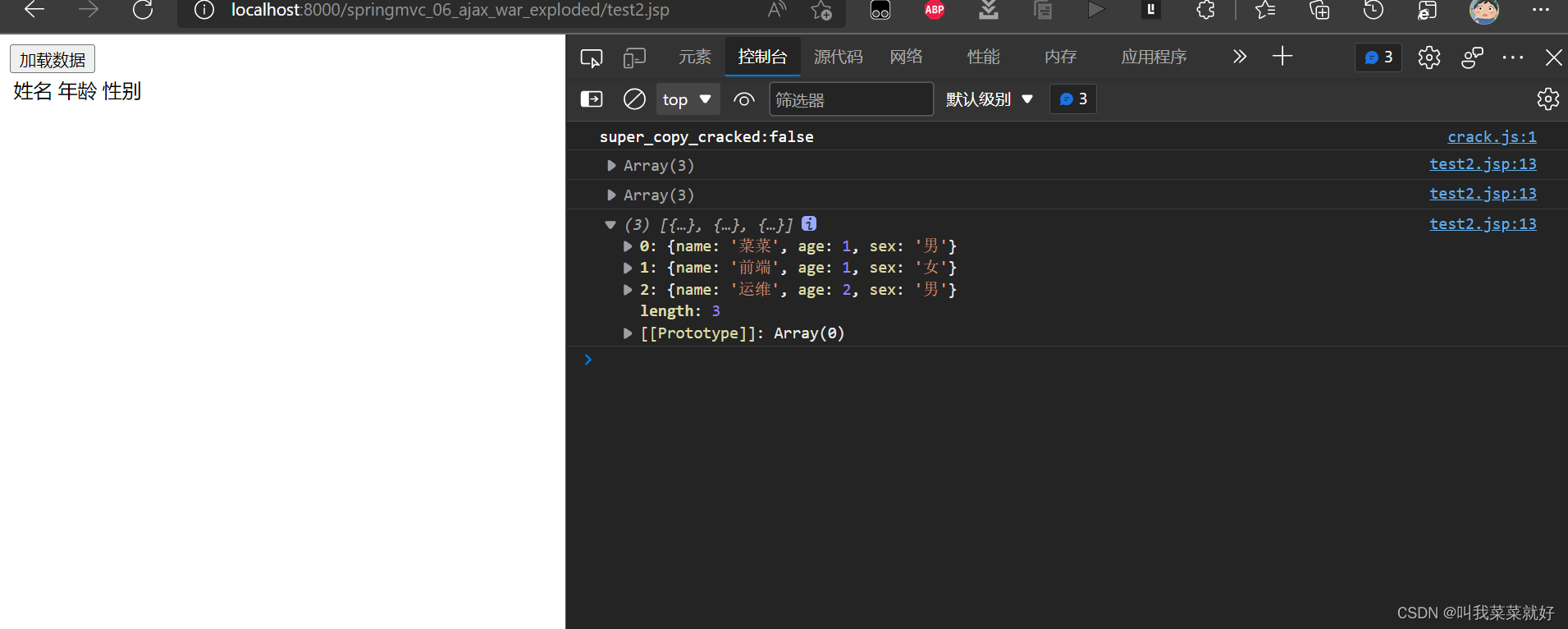
这里后台拿到了数据,接下来就可以把数据放入前端中。
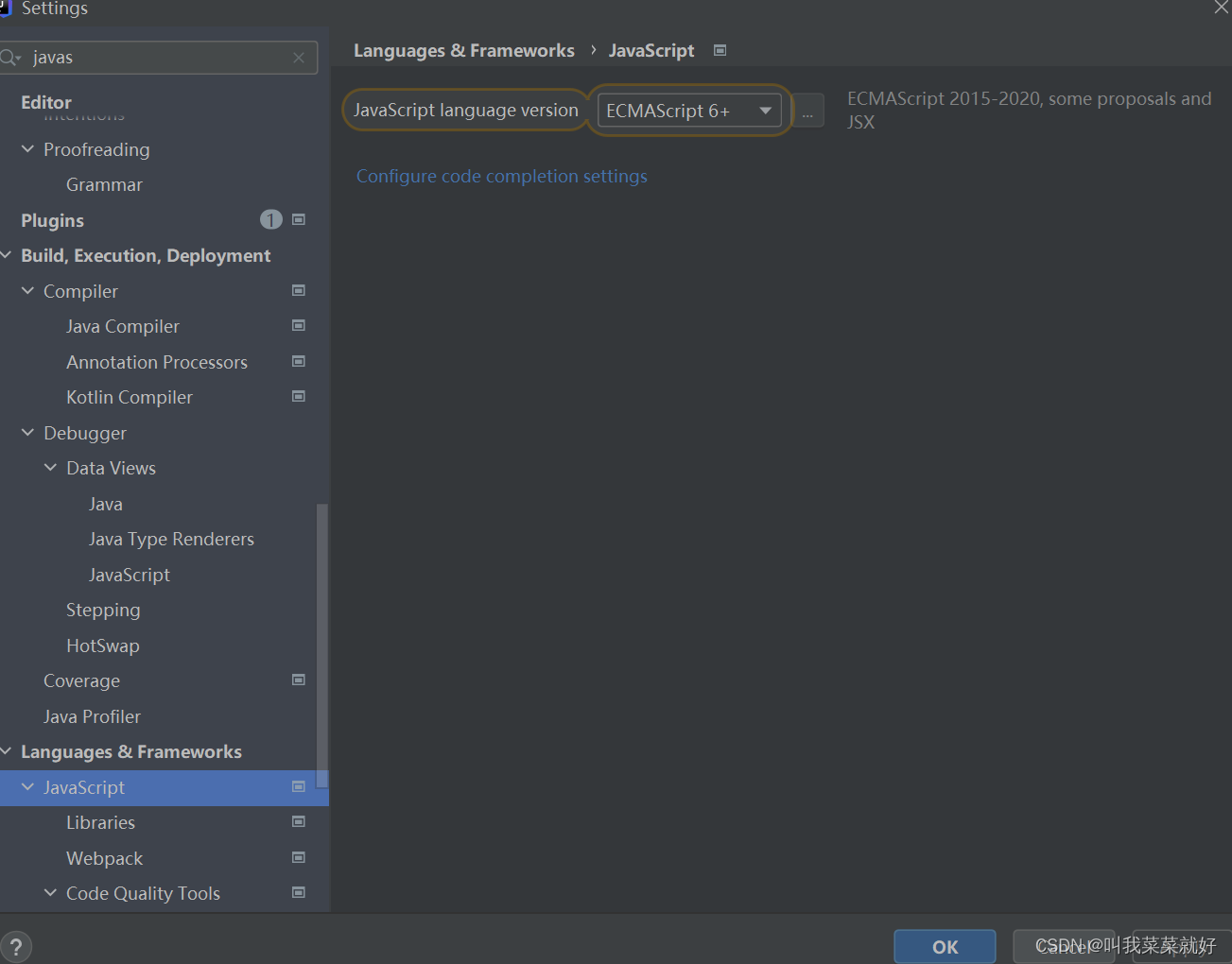
数据放入前端中:用拼接的方式,把数据循环放入
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
<script src="" target="_blank">https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(function (){
$("#btn").click(function (){
/*
* $.post(url,param(可以省略),success)
* */
$.post("${pageContext.request.contextPath}/a2",function (data){
//console.log(data);
var html = "";
for (let i = 0; i < data.length; i++) {
html += "<tr>"+
"<td>" + data[i].name + "</td>" +
"<td>" + data[i].age + "</td>" +
"<td>" + data[i].sex + "</td>" +
"</tr>"
}
$("#content").html(html);
});
})
});
</script>
</head>
<body>
<input type="button" value="加载数据" id="btn">
<table>
<tr>
<td>姓名</td>
<td>年龄</td>
<td>性别</td>
</tr>
<tbody id="content">
<%--数据在后台--%>
</tbody>
</table>
</body>
</html>
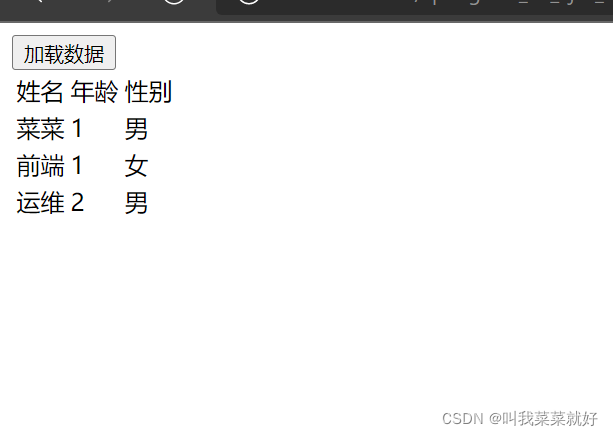
Ajax验证用户名体验
AjaxController
@RequestMapping("a3")
public String a3(String name,String pwd){
String msg="";
if(name!=null){
//admin 这些数据应该在数据库中查
if("admin".equals(name)){
msg = "ok";
}else {
msg="用户名有误";
}
}
if(pwd!=null){
//123456 这些数据应该在数据库中查
if("123456".equals(pwd)){
msg = "ok";
}else {
msg="密码有误";
}
}
return msg;
}
登陆页面
login.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
<script src="" target="_blank">https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
function a1(){
//发起请求
$.post({
url:"${pageContext.request.contextPath}/a3",
data:{"name":$("#name").val()},
success:function (data){
if(data.toString()==='ok'){
$("#userInfo").css("color","green");
}else{
$("#userInfo").css("color","red");
}
$("#userInfo").html(data);
}
})
}
function a2(){
//发起请求
$.post({
url:"${pageContext.request.contextPath}/a3",
data:{"pwd":$("#pwd").val()},
success:function (data){
if(data.toString()==='ok'){
$("#pwdInfo").css("color","green");
}else{
$("pwdInfo").css("color","red");
}
$("#pwdInfo").html(data);
}
})
}
</script>
</head>
<body>
<p>
用户名:<input type="text" id="name" οnblur="a1()">
<span id="userInfo"></span>
</p>
<p>
密码:<input type="text" id="pwd" οnblur="a2()">
<span id="pwdInfo"></span>
</p>
</body>
</html>
问题
1
服务器报了500的错误,这种属于服务器代码错误。
这里我们把url写错了
正确的:
"${pageContext.request.contextPath}/a3"
不能把a3写进里面,不然会找不到方法。
2
后台没有数据
可能是因为没有导入jQuery
<script src="" target="_blank">https://code.jquery.com/jquery-3.1.1.min.js"></script>
也可能是因为后端没有接收到数据,可以看响应
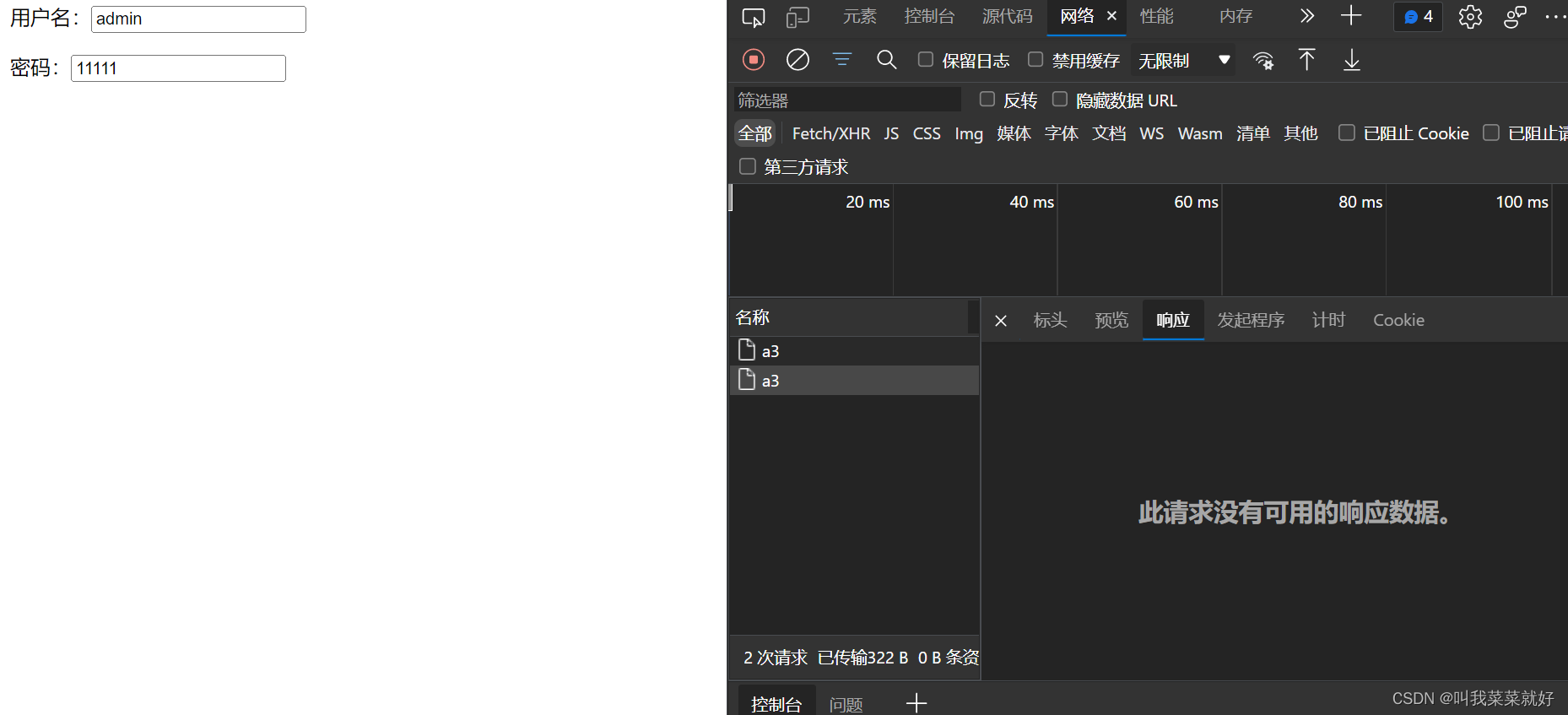
这里显示响应没有请求的数据。
解决:
很有可能是JSON乱码的问题
JSON乱码解决
也有可能是标签写错了
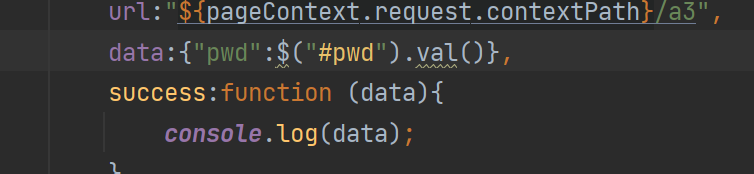
这里是通过标签获取相应的值的,如果标签错了,那么就拿不到前端的值,那么就是空。
3
如果是前端错误的话,修改完之后点击这个之后也可以成功修改,并且速度比较快
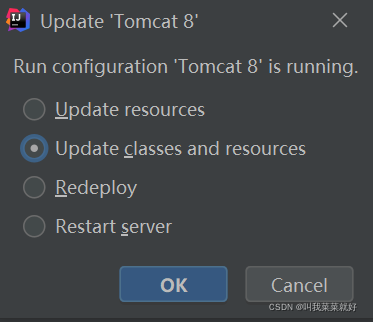
但是,如果是后端代码错误了,必须Restart server,重启服务器才可能修改成功。