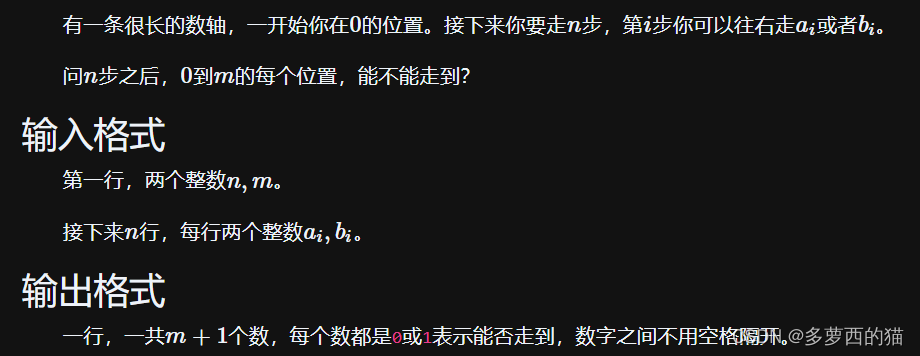
#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
const int maxn = 105, maxm = 1e5 + 5;
int n, m;
int f[maxn][maxm];
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n >> m;
f[0][0] = 1;
For (i, 1, n) {
int t1, t2;
cin >> t1 >> t2;
For (j, 0, m) {
if (f[i - 1][j]) {
f[i][j + t1] = 1;
f[i][j + t2] = 1;
}
}
}
For (i, 0, m) {
if (f[n][i]) cout << 1; else cout << 0;
}
return 0;
}
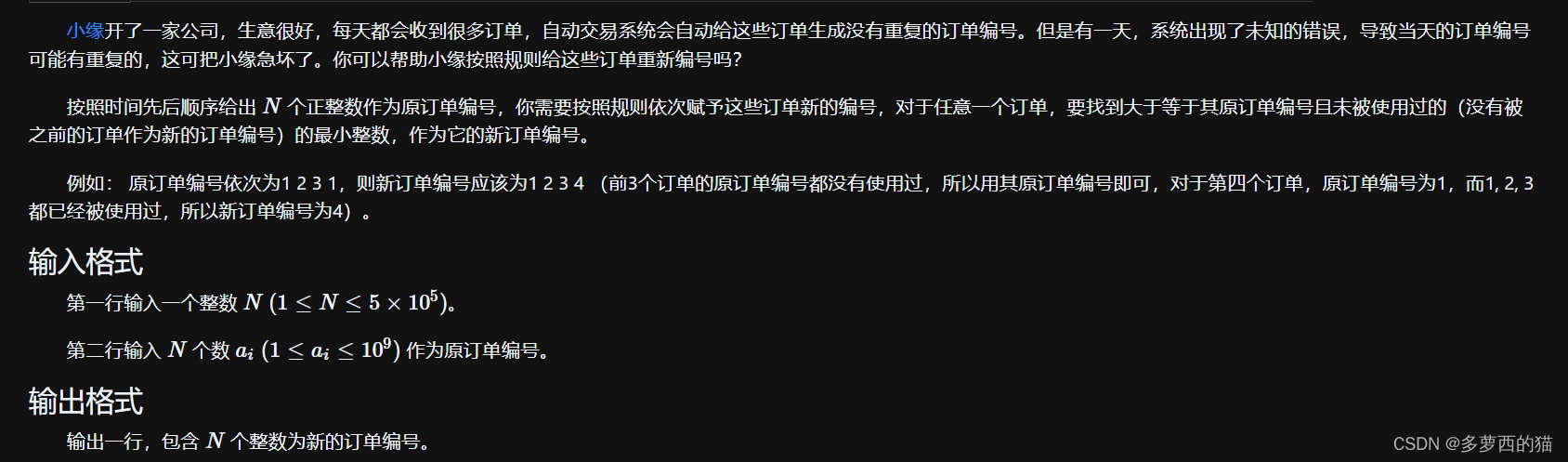
#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
const int maxn = 5e5 + 5;
int n, t;
int a[maxn];
set <pair<int, int> > st;
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n;
st.insert({2e9, 1});
For (i, 1, n) {
cin >> t;
auto iter = st.lower_bound({t, 0});
if (iter->second <= t) {
cout << t << " ";
if (iter->second <= t - 1)
st.insert({t - 1, iter->second});
if (t + 1 <= iter->first)
st.insert({iter->first, t + 1});
st.erase(iter);
} else {
cout << iter->second << " ";
if (iter->second + 1 <= iter->first)
st.insert({iter->first, iter->second + 1});
st.erase(iter);
}
}
return 0;
}
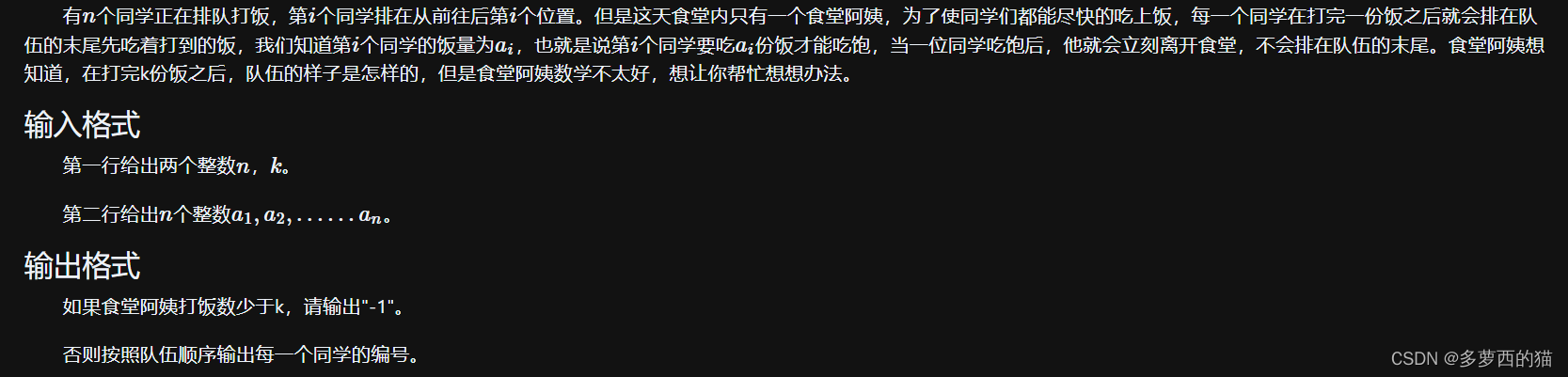
#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
ll n, k;
const int maxn = 1e5 + 5;
int a[maxn];
ll s[maxn];
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n >> k;
For (i, 1, n) cin >> a[i], s[i] = s[i - 1] + a[i];
if (s[n] < k ) { puts("-1"); exit(0); }
ll l = 0, r = 1e9;
while (l + 1 < r) {
ll mid = l + r >> 1;
ll sum = 0;
For (i, 1, n) {
if (a[i] <= mid) sum += a[i];
else sum += mid;
}
if (sum > k) r = mid;
else l = mid;
}
ll sum = 0;
For (i, 1, n) {
if (a[i] <= l) sum += a[i];
else sum += l;
}
ll res = k - sum;
queue <int> q;
int cnt = 0;
For (i, 1, n) {
if (a[i] >= l + 1) {
if (cnt >= res) {
cout << i << " ";
}
else {
cnt++;
if (a[i] > l + 1)
q.push(i);
}
}
}
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
return 0;
}
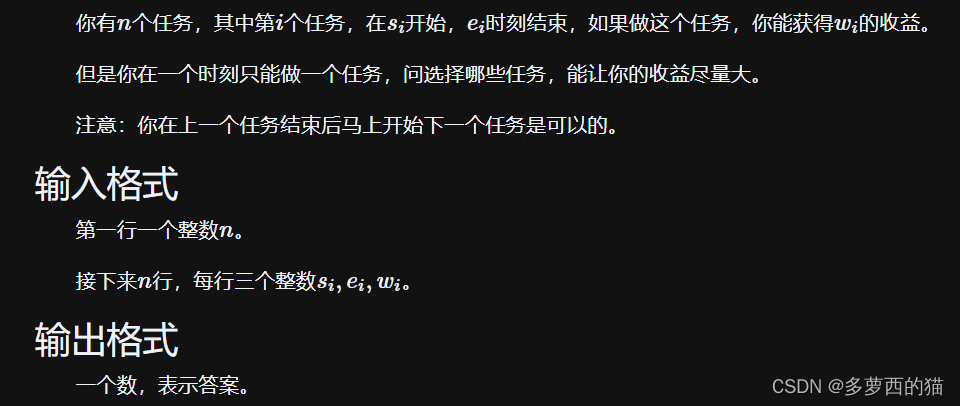
#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
const int maxn = 1e3 + 5;
struct node {
int s, e, w;
} a[maxn];
int f[maxn];
int n;
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n;
For (i, 1, n) {
cin >> a[i].s >> a[i].e >> a[i].w;
}
sort(a + 1, a + 1 + n, [](node a, node b){
return (a.s < b.s || (a.s == b.s && a.e < b.e) || (a.s == b.s && a.e == b.e && a.w < b.w));
});
For (i, 1, n) f[i] = a[i].w;
For (i, 1, n) {
For (j, 1, i - 1) {
if (a[j].e <= a[i].s && f[j] + a[i].w > f[i]) {
f[i] = f[j] + a[i].w;
}
}
}
int ans = 0;
For (i, 1, n) {
ans = max(ans, f[i]);
}
cout << ans;
return 0;
}

#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
int n;
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n;
For (i, 1, n) {
For (j, 1, n) {
For (l, 1, n) {
if (((i == l || i == n - l + 1) && (j >= l || j <= n - l + 1))
|| ((j == l || j == n - l + 1) && (i >= l || i <= n - l + 1)))
{
if (l % 2 == 1) cout << '+'; else cout << '.';
break;
}
}
}
cout << '\n';
}
return 0;
}
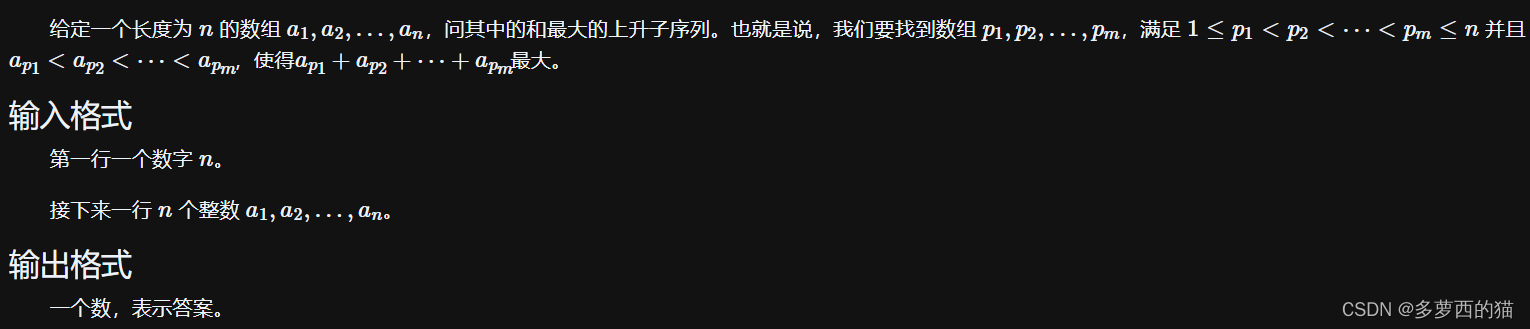
/*
板子题
*/
#include <bits/stdc++.h>
#define For(i, a, b) for (int i = a; i <= b; i++)
#define _For(i, a, b) for (int i = a; i >= b; i--)
typedef long long ll;
using namespace std;
const int maxn = 1e3 + 5;
int n;
int a[maxn], f[maxn];
int main(){
ios::sync_with_stdio(false); cin.tie(0); cout.tie(0);
cin >> n;
For (i, 1, n) cin >> a[i];
For (i, 1, n) f[i] = a[i];
For (i, 1, n) {
For (j, 1, i - 1) {
if (a[i] > a[j] && f[i] < f[j] + a[i]) f[i] = f[j] + a[i];
}
}
int ans = 0;
For (i, 1, n) {
ans = max(ans, f[i]);
}
cout << ans;
return 0;
}