文章目录
jdbc连接数据库
点击目录最后面查看完整代码。
什么是jdbc
jdbc是 Java连接数据库的简称,提供连接各种常用数据库的能力。
完成jdbc的步骤
新建web动态项目
导入jar包
直接把连接的jar包拖入lib文件夹,然后build path
写三个类
连数据库工具:util
数据访问类:dao
实体类:bean(entity)
DBConn.java
注意导包都是sql相关包
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
加载驱动类:找到对应的然后写上
//加载驱动类
private static String driver = "com.mysql.jdbc.Driver";
// navicat新建连接时的主机地址和端口号
// :127.0.0.1/
// jdbc:数据库://localhost:3306/数据库名 问号及以后是字符编码设置
private static String url = "jdbc:mysql://localhost:3306/students?useUnicode=true&characterEncoding=UTF-8";
获取连接
//获取连接
public static Connection getConnection(){
Connection conn = null;
//加载驱动
Class.forName(driver);
//获取连接
conn = DriverManager.getConnection(url, "root","123456");//navicat新建连接时的用户名和密码
System.out.println("连接成功");
return conn;
}
然后会有报错,把下面两行代码选中然后放入try/catch块即可
关闭连接
用完肯定要关啊
代码解释
写下面的代码就行,然后根据报错提示加入对应的try/catch即可
//关闭连接
public static void closeAll(ResultSet rs,PreparedStatement ps,Connection conn){
if(rs != null){
rs.close();
}
if(ps != null){
ps.close();
}
if(conn != null){
conn.close();
}
}
最后写一个main方法调用测试一下
public static void main(String[] args) {
Connection connection = getConnection();
closeAll(null,null,connection);
}
运行结果
Students.java
实体类的代码很好写,确保和数据库字段一致即可,避免出现不一致导致的麻烦(不一致当然可以解决,但是我忘了……)
生成对应get,set方法,有参构造,无参构造,toString()方法。
再加一个:少了id的有参构造,因为在设计数据库时,id设置的是自增,不需要手动输入。所以在添加用户时,不用手动录入id,所以就调一个没有id的构造函数。
完整代码在最下面。
StudentDao.java
dao类比较规范的是写一个接口,再写一个实现类,这里为了简便,直接写在一起了。
这个就是业务控制层,在这里运行卖main就行。
用这个运行,不要发布到服务器
package com.web.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.web.entity.Students;
import com.web.util.DBConn;
public class StudentDao {
private Connection conn = null;
//获取sql语句,执行
private PreparedStatement ps = null;
//结果集,存放数据库中获取的数据
private ResultSet rs = null;
/**
* 查询全部学生信息
* @return
*/
public List<Students> selectStuAll(){
List<Students> list = new ArrayList<Students>();
//获取连接,要不然你拿不到数据库数据
conn = DBConn.getConnection();
//执行sql语句
String sql = "select * from stus";
try {
ps = conn.prepareStatement(sql);//获取执行sql的对象
rs = ps.executeQuery();//真正执行sql语句,保存在rs
//保存数据
while(rs.next()){
Students stus = new Students();
stus.setId(rs.getInt("id"));
stus.setName(rs.getString("name"));
stus.setSex(rs.getString("sex"));
stus.setAge(rs.getInt("age"));
stus.setBirthday(rs.getString("birthday"));
stus.setAddress(rs.getString("address"));
list.add(stus);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs,ps,conn);
}
return list;
}
//查询
public Students selectStudentById(int id){
Students stu = null;
//获取连接
conn = DBConn.getConnection();
//执行sql语句
String sql = "select * from stus where id=?";
try {
ps = conn.prepareStatement(sql);//获取执行sql的对象
//给问号赋值
// 第几个问号,传的是什么
//只能是? 问号是占位符
ps.setInt(1,id);
rs = ps.executeQuery();//真正执行sql语句,保存在rs
while(rs.next()){
stu = new Students();
stu.setId(rs.getInt("id"));
stu.setName(rs.getString("name"));
stu.setSex(rs.getString("sex"));
stu.setAge(rs.getInt("age"));
stu.setBirthday(rs.getString("birthday"));
stu.setAddress(rs.getString("address"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs,ps,conn);
}
return stu;
}
//增加
public void addStu(Students stu){
conn = DBConn.getConnection();
String sql = "insert into stus(name,sex,age,birthday,address) values(?,?,?,?,?)";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, stu.getName());
ps.setString(2, stu.getSex());
ps.setInt(3, stu.getAge());
ps.setString(4, stu.getBirthday());
ps.setString(5, stu.getAddress());
//更新数据库
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DBConn.closeAll(rs, ps, conn);
}
}
//修改
public void updateStu(Students stu){
conn = DBConn.getConnection();
String sql = "update stus set name=?,address=? where id = ?";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, stu.getName());
ps.setString(2, stu.getAddress());
ps.setInt(3, stu.getId());
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DBConn.closeAll(rs, ps, conn);
}
}
//删除
public void delStu(int id){
conn = DBConn.getConnection();
String sql = "delete from stus where id = ?";
try {
ps = conn.prepareStatement(sql);
ps.setInt(1,id);
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs, ps, conn);
}
}
public static void main(String[] args) {
StudentDao dao = new StudentDao();
//查询
//Students s = dao.selectStudentById(2);
//System.out.println(s);
//添加
//Students stu = new Students("Amy","女",22,"2002-12-6","临汾市");
// dao.addStu(stu);
//修改
//获取原本对象,然后对相应值继续宁修改
//Students s = dao.selectStudentById(4);
// s.setName("李华华");
// s.setAddress("北京市");
// dao.updateStu(s);
//查询所有数据
//删除
dao.delStu(7);
List<Students> list = dao.selectStuAll();
System.out.println(list);
}
}
运行结果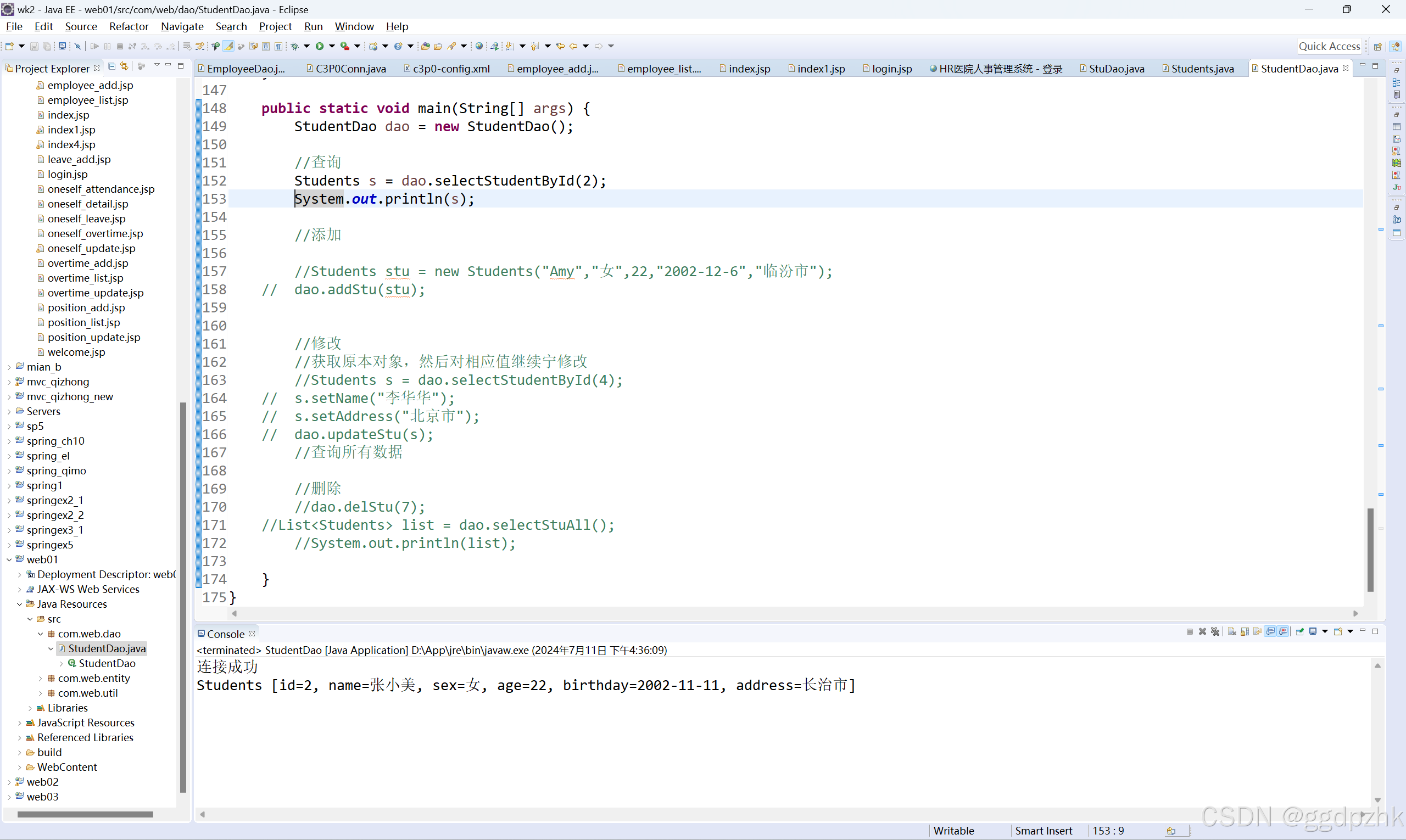
完整代码
package com.web.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.web.entity.Students;
import com.web.util.DBConn;
public class StudentDao {
private Connection conn = null;
//获取sql语句,执行
private PreparedStatement ps = null;
//结果集,存放数据库中获取的数据
private ResultSet rs = null;
/**
* 查询全部学生信息
* @return
*/
public List<Students> selectStuAll(){
List<Students> list = new ArrayList<Students>();
//获取连接,要不然你拿不到数据库数据
conn = DBConn.getConnection();
//执行sql语句
String sql = "select * from stus";
try {
ps = conn.prepareStatement(sql);//获取执行sql的对象
rs = ps.executeQuery();//真正执行sql语句,保存在rs
//保存数据
while(rs.next()){
Students stus = new Students();
stus.setId(rs.getInt("id"));
stus.setName(rs.getString("name"));
stus.setSex(rs.getString("sex"));
stus.setAge(rs.getInt("age"));
stus.setBirthday(rs.getString("birthday"));
stus.setAddress(rs.getString("address"));
list.add(stus);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs,ps,conn);
}
return list;
}
public Students selectStudentById(int id){
Students stu = null;
//获取连接
conn = DBConn.getConnection();
//执行sql语句
String sql = "select * from stus where id=?";
try {
ps = conn.prepareStatement(sql);//获取执行sql的对象
//给问号赋值
// 第几个问号,传的是什么
//只能是? 问号是占位符
ps.setInt(1,id);
rs = ps.executeQuery();//真正执行sql语句,保存在rs
while(rs.next()){
stu = new Students();
stu.setId(rs.getInt("id"));
stu.setName(rs.getString("name"));
stu.setSex(rs.getString("sex"));
stu.setAge(rs.getInt("age"));
stu.setBirthday(rs.getString("birthday"));
stu.setAddress(rs.getString("address"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs,ps,conn);
}
return stu;
}
//增加
public void addStu(Students stu){
conn = DBConn.getConnection();
String sql = "insert into stus(name,sex,age,birthday,address) values(?,?,?,?,?)";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, stu.getName());
ps.setString(2, stu.getSex());
ps.setInt(3, stu.getAge());
ps.setString(4, stu.getBirthday());
ps.setString(5, stu.getAddress());
//更新数据库
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DBConn.closeAll(rs, ps, conn);
}
}
public void updateStu(Students stu){
conn = DBConn.getConnection();
String sql = "update stus set name=?,address=? where id = ?";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, stu.getName());
ps.setString(2, stu.getAddress());
ps.setInt(3, stu.getId());
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
DBConn.closeAll(rs, ps, conn);
}
}
public void delStu(int id){
conn = DBConn.getConnection();
String sql = "delete from stus where id = ?";
try {
ps = conn.prepareStatement(sql);
ps.setInt(1,id);
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBConn.closeAll(rs, ps, conn);
}
}
public static void main(String[] args) {
StudentDao dao = new StudentDao();
//查询
//Students s = dao.selectStudentById(2);
//System.out.println(s);
//添加
//Students stu = new Students("Amy","女",22,"2002-12-6","临汾市");
// dao.addStu(stu);
//修改
//获取原本对象,然后对相应值继续宁修改
//Students s = dao.selectStudentById(4);
// s.setName("李华华");
// s.setAddress("北京市");
// dao.updateStu(s);
//查询所有数据
//删除
dao.delStu(7);
List<Students> list = dao.selectStuAll();
System.out.println(list);
}
}
package com.web.entity;
public class Students {
private int id;
private String name;
private String sex;
private int age;
private String birthday;
private String address;
public Students(int id, String name, String sex, int age, String birthday, String address) {
super();
this.id = id;
this.name = name;
this.sex = sex;
this.age = age;
this.birthday = birthday;
this.address = address;
}
public Students( String name, String sex, int age, String birthday, String address) {
super();
this.name = name;
this.sex = sex;
this.age = age;
this.birthday = birthday;
this.address = address;
}
public Students() {
super();
// TODO Auto-generated constructor stub
}
@Override
public String toString() {
return "Students [id=" + id + ", name=" + name + ", sex=" + sex + ", age=" + age + ", birthday=" + birthday
+ ", address=" + address + "]";
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
package com.web.util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class DBConn {
//加载驱动类
private static String driver = "com.mysql.jdbc.Driver";
//获取连接
//地址要记住
// 137.0.0.1 数据库库名
//private static String url = "jdbc:mysql://localhost:3306/students?serverTimezone=GMT%2B8";
private static String url = "jdbc:mysql://localhost:3306/students?useUnicode=true&characterEncoding=UTF-8";
//获取连接
public static Connection getConnection(){
Connection conn = null;
try {
//加载驱动
Class.forName(driver);
//获取连接
conn = DriverManager.getConnection(url, "root","123456");
System.out.println("连接成功");
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return conn;
}
//关闭连接
public static void closeAll(ResultSet rs,PreparedStatement ps,Connection conn){
if(rs != null){
try {
rs.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(ps != null){
try {
ps.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(conn != null){
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
public static void main(String[] args) {
Connection connection = getConnection();
closeAll(null,null,connection);
}
}