题目:
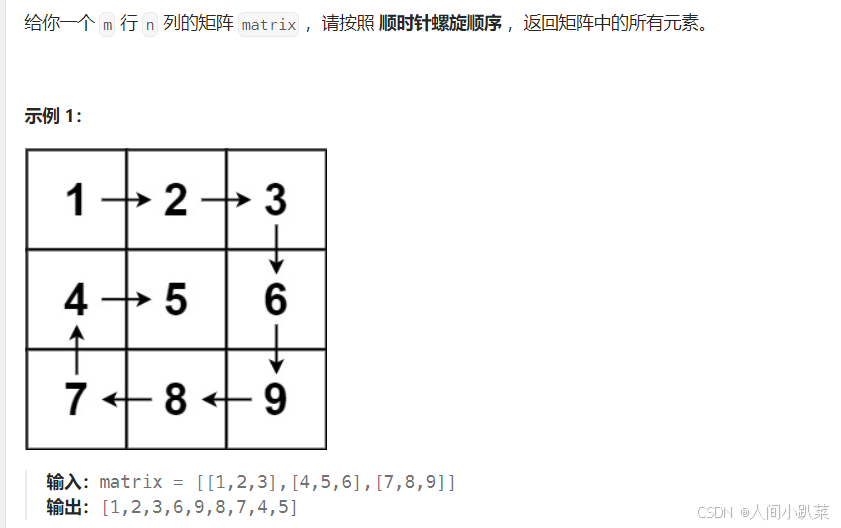
题解:
按层 计算
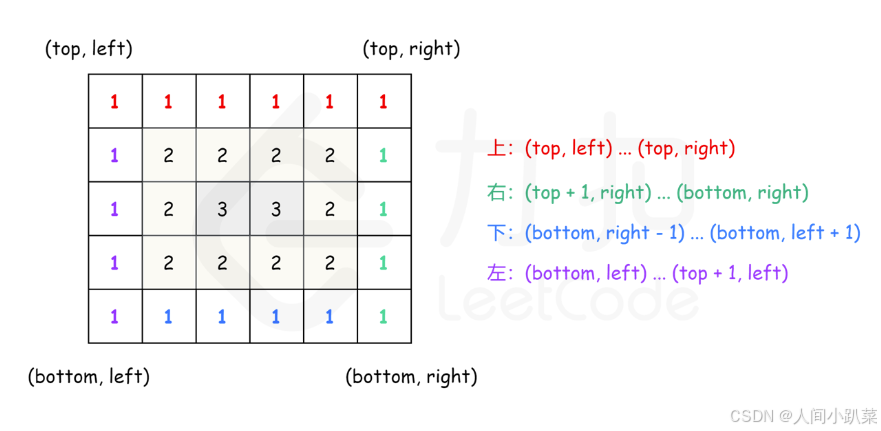
const spiralOrder = function(matrix) {
let rows = matrix.length;
let cols = matrix[0].length;
let res = [];
//特殊情况
if(rows === 0 || cols === 0) return res;
if(rows === 1) return matrix[0];
//设置每层的边界
let left = 0, right = cols - 1, top = 0, bottom = rows - 1;
while(left<= right && top <= bottom){
//从左到右
for(let i = left; i <= right; i++){
res.push(matrix[top][i]);
}
//从上到下
for(let i = top + 1; i <= bottom; i++){
res.push(matrix[i][right]);
}
//若该层完整,能四周走一圈
if(top < bottom && left < right){
//从右到左
for(let i = right - 1; i >= left; i--){
res.push(matrix[bottom][i]);
}
//从下到上
for(let i = bottom - 1; i > top; i--){
res.push(matrix[i][left]);
}
}
//改变边界
left++;right--;top++;bottom--;
}
return res;
}