I have been doing some very neat stuff using Hudson the past couple of weeks. This is something which is really awesome, and am amazed at Hudson every time I use it. Hudson has a built-in command line interface which I have been using recently. I didn't find good documentation for doing some simple things like creating a job, copying a job and so on.. Hence, decided to write a blog entry so would help me and many others as well.
First and foremost is to download the cli library, and you can do so easily from the Hudson-> Manage Hudson ->Hudson CLI link as shown below.
Now, click on the link and you will be presented with another page, were you click the download hudson-cli.jar link.
Next, open a command line and browse to where the hudson-cli.jar was downloaded, or even better copy to your favorite location.
At this point you should be able to run commands to create a job, enable a job, disable, copy and also delete a job. So, lets look at some of the commands.
1. Display help
java -jar hudson-cli.jar -s http://localhost:7223/ help
2. Create a Job, use the following command. config.xml is a sample configuration file which hudson needs for creating a job. You can find one in the hudson home folder. You also need to provide the configuration file next to the job name. Double click on the image below to see the parameters for passing in the configuration file. For some reason, each time I tried entering the same, blogpost automatically reformatted the same.
java -jar hudson-cli.jar -s http://localhost:7223/ create-job meera
If you go to the hudson main page, you should be able to see a new job called meera created.
Lets see a few more commands which can be executed:
3. Copy Job
java -jar hudson-cli.jar -s http://localhost:7223/ copy-job meera copy-meera
4. Disable a Job
java -jar hudson-cli.jar -s http://localhost:7223/ disable-job meera
5. Enable a Job
java -jar hudson-cli.jar -s http://localhost:7223/ enable-job meera
6. Delete a Job
java -jar hudson-cli.jar -s http://localhost:7223/ delete-job meera
Once you get an idea of how to use the command line interface, it is very easy to automate many things by using this interface.
2、update hudson job
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
File configFile = new File("config.xml");
put(client, hudson, "test", configFile);
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName, File configFile)
throws IOException,
HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/config.xml");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setRequestHeader("Content-type", "application/xml; charset=ISO-8859-1");
postMethod.setRequestBody(new FileInputStream(configFile));
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}
public static void main(String[] args) throws Exception {
3、copy hudson job
4、delete job
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
put(client, hudson, "copyNew");
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName) throws IOException, HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/doDelete");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}
public static void main(String[] args) throws Exception {
5、enable disable job
public static void main(String[] args) throws Exception {
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
put(client, hudson, "test");
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName) throws IOException, HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/disable");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}
First and foremost is to download the cli library, and you can do so easily from the Hudson-> Manage Hudson ->Hudson CLI link as shown below.
Now, click on the link and you will be presented with another page, were you click the download hudson-cli.jar link.
Next, open a command line and browse to where the hudson-cli.jar was downloaded, or even better copy to your favorite location.
At this point you should be able to run commands to create a job, enable a job, disable, copy and also delete a job. So, lets look at some of the commands.
1. Display help
java -jar hudson-cli.jar -s http://localhost:7223/ help
2. Create a Job, use the following command. config.xml is a sample configuration file which hudson needs for creating a job. You can find one in the hudson home folder. You also need to provide the configuration file next to the job name. Double click on the image below to see the parameters for passing in the configuration file. For some reason, each time I tried entering the same, blogpost automatically reformatted the same.
java -jar hudson-cli.jar -s http://localhost:7223/ create-job meera
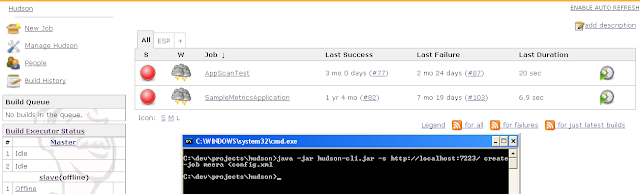
If you go to the hudson main page, you should be able to see a new job called meera created.
Lets see a few more commands which can be executed:
3. Copy Job
java -jar hudson-cli.jar -s http://localhost:7223/ copy-job meera copy-meera
4. Disable a Job
java -jar hudson-cli.jar -s http://localhost:7223/ disable-job meera
5. Enable a Job
java -jar hudson-cli.jar -s http://localhost:7223/ enable-job meera
6. Delete a Job
java -jar hudson-cli.jar -s http://localhost:7223/ delete-job meera
Once you get an idea of how to use the command line interface, it is very easy to automate many things by using this interface.
I am also working on creating a JAVA API for using the Hudson Remote API, and also a few build scripts as well. Will keep this blog updated on each of those as soon as I have them working.
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
1、hudson API create job
2、update hudson job
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
File configFile = new File("config.xml");
put(client, hudson, "test", configFile);
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName, File configFile)
throws IOException,
HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/config.xml");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setRequestHeader("Content-type", "application/xml; charset=ISO-8859-1");
postMethod.setRequestBody(new FileInputStream(configFile));
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}
3、copy hudson job
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
put(client, hudson, "copyNew");
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName) throws IOException, HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/doDelete");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}
5、enable disable job
HttpClient client = new HttpClient();
String hudson = "http://10.20.157.179:8080/hudson/";
put(client, hudson, "test");
}
private static void put(HttpClient client, String hudsonBaseURL, String jobName) throws IOException, HttpException {
PostMethod postMethod = new PostMethod(hudsonBaseURL + "/job/" + jobName + "/disable");
client.getParams().setAuthenticationPreemptive(true);
Credentials defaultcreds = new UsernamePasswordCredentials("admin", "sfhudson");
client.getState().setCredentials(new AuthScope("10.20.157.179", 8080), defaultcreds);
postMethod.setDoAuthentication(true);
try {
int status = client.executeMethod(postMethod);
System.out.println(status + "\n" + postMethod.getResponseBodyAsString());
} finally {
postMethod.releaseConnection();
}
}