一、思路
- 通过onclick将点击事件和对应的方法绑定
- 通过id获取元素,设置一个基础值i
- 描述对应的方法:点击一次按钮,i做自加或自减,然后通过style.fontSize=i + "px"改变文本的样式,用if判定步入字体大于18px和小于14px,要超出时提醒并让其对应按钮消失
- 增加一个计时器,每隔300毫秒检测字体的大小,并对按钮的出现做调整
二、成品展示
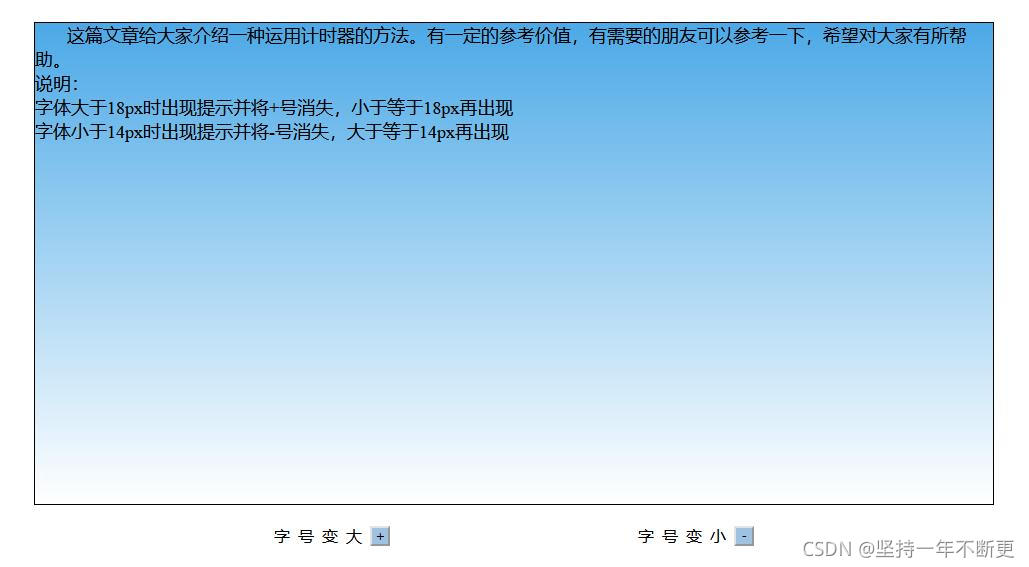
三、代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>字体的变大变小</title>
<style>
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* 居中对齐 */
body,html{
height: 100%;
}
body{
display: flex;
flex-direction: column;
justify-content:center;
align-items: center;
}
/* 样式 */
#maxDiv{
width: 50%;
min-width: 400px;
height: 50%;
min-height: 400px;
background:linear-gradient(rgb(76, 169, 231),#fff);/*线性渐变,默认从上到下*/
border: 1px solid black;
margin: 20px 0;
text-indent: 2em;
}
#minDiv{
width: 25%;
display: flex;
justify-content:space-between;
align-items: center;
}
Button{
width: 20px;
height: 20px;
background-color: rgb(160, 194, 226);
}
#leftButton,#rightButton{
display: flex;
justify-content: center;
align-items: center;
letter-spacing: 8px;
}
#text{
font-size: 14px;
color: black;
}
</style>
</head>
<body>
<div id="maxDiv">
<p id="text">这篇文章给大家介绍一种运用计时器的方法。
有一定的参考价值,有需要的朋友可以参考一下,
希望对大家有所帮助。<br/>
说明:<br/>字体大于18px时出现提示并将+号消失,小于等于18px再出现<br/>
字体小于14px时出现提示并将-号消失,大于等于14px再出现
</p>
</div>
<div id="minDiv">
<div id="leftButton">
<p>字号变大</p>
<button type="button" onclick="add()">+</button>
<!--另一种<input type="button" value+>-->
</div>
<div id="rightButton">
<p>字号变小</p>
<button type="button" onclick="cut()">-</button>
</div>
</div>
</body>
<script>
//获取元素
let text = document.getElementById("text");
let leftButton = document.getElementById("leftButton");
let rightButton = document.getElementById("rightButton");
let i=14;//如果设置在函数里面只会加一次++
//方法
function add(){
i++;//意思是每点一次变大按钮,i做一次自加
text.style.fontSize=i + "px";//利用+将i和px连接起来
if(text.style.fontSize>"18px"){//判断
alert("抱歉,不能更大了!");
leftButton.style.display="none";//大于18px时,变大按钮消失
}
if(text.style.fontSize>="14px"){//大于等于14px,减小按钮出现
rightButton.style.display=" flex";
}
}
function cut(){
i--;
text.style.fontSize=i + "px";
if(text.style.fontSize<"14px"){
alert("抱歉,不能更小了!");
rightButton.style.display="none";
}
if(text.style.fontSize<="18px"){
leftButton.style.display=" flex";
}
}
//计时器,每隔300毫秒执行一次函数,防止两个按钮都消失
setInterval(function(){
if(text.style.fontSize<="18px"){
leftButton.style.display=" flex";
}
if(text.style.fontSize>="14px"){
rightButton.style.display=" flex";
}
},300)
</script>
</html>