效果如下:
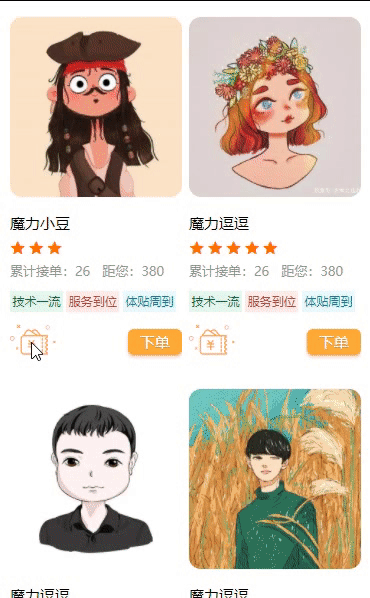
HTML:
<view class="whiteBg">
<people-list :peopleListData="peopleListData" @getCoupon="fatherCoupon"></people-list>
<coupon-list :couponListData = "couponListData"></coupon-list>
</view>
JS:
<script>
import PeopleList from '@/components/PeopleList.vue';
import CouponList from "@/components/CouponList.vue";
export default {
components: {
PeopleList,
CouponList,
},
data() {
return {
//列表数据
peopleListData:[
{
id:0,
imgUrl:"https://img1.baidu.com/it/u=2265759430,2688457354&fm=26&fmt=auto&gp=0.jpg",
name:"魔力小豆",
orders:"26",
space:"380",
tags:["技术一流","服务到位","体贴周到"],
coupon:[
{id:0,couponMoney:15,couponTxt:"下单直接抵扣",couponTitle:"折扣券"},
{id:1,couponMoney:20,couponTxt:"满20元抵扣",couponTitle:"代金券"},
{id:2,couponMoney:25,couponTxt:"联合抵扣",couponTitle:"邀请券"},
],
star:3
},{
id:1,
imgUrl:"https://img1.baidu.com/it/u=3429531540,1015649330&fm=26&fmt=auto&gp=0.jpg",
name:"魔力逗逗",
orders:"26",
space:"380",
tags:["技术一流","服务到位","体贴周到"],
coupon:[
{id:0,couponMoney:15,couponTxt:"下单直接抵扣",couponTitle:"折扣券"},
{id:1,couponMoney:20,couponTxt:"满20元抵扣",couponTitle:"代金券"},
{id:2,couponMoney:25,couponTxt:"联合抵扣",couponTitle:"邀请券"},
],
star:5
},{
id:2,
imgUrl:"https://img0.baidu.com/it/u=2779568578,1953729642&fm=26&fmt=auto&gp=0.jpg",
name:"魔力逗逗",
orders:"26",
space:"380",
tags:["技术一流","服务到位","体贴周到"],
coupon:[
{id:0,couponMoney:15,couponTxt:"下单直接抵扣",couponTitle:"折扣券"},
{id:1,couponMoney:20,couponTxt:"满20元抵扣",couponTitle:"代金券"},
{id:2,couponMoney:25,couponTxt:"联合抵扣",couponTitle:"邀请券"},
],
star:3
},{
id:3,
imgUrl:"https://img0.baidu.com/it/u=2668412444,819000202&fm=26&fmt=auto&gp=0.jpg",
name:"魔力逗逗",
orders:"26",
space:"380",
tags:["技术一流","服务到位","体贴周到"],
coupon:[],
star:1
}
],
couponListData:[],//优惠券数据
}
},
methods: {
//点击优惠券
fatherCoupon(id,index){
console.log("我是父级获取id" + id + "我是父级获取index" + index);
this.couponListData = this.peopleListData[index].coupon
//获取优惠券显示
this.$set(this.couponListData,'isCoupon',true);
}
}
}
</script>
子组件 PeopleList:
<!--技师列表组件-->
<template>
<view>
<view class="peopleList" v-for="(list,index) in peopleListData" :key="index">
<view @click="toPeopleContent(list.id)">
<image :src="list.imgUrl" mode="aspectFill" class="peopleImg"></image>
<view class="peopleName">{{list.name}}</view>
<view class="peopleStar">
<text class="iconfont" v-for="item in list.star"></text>
</view>
<view class="peopleInfo">累计接单:{{list.orders}}<text>距您:{{list.space}}</text></view>
<view class="peopleTags">
<text v-for="(tags,index) in list.tags" :key="index">{{tags}}</text>
</view>
</view>
<view class="peopleBt">
<text class="iconfont" @click="getCoupon(list.id,index)"
v-if="list.coupon.length != 0 && list.coupon != undefined"></text>
<text class="toOrder" @click="toOrders">下单</text>
</view>
</view>
</view>
</template>
<script>
export default{
name:"PeopleList",
props:{
peopleListData:Array
// id:0,//id
// imgUrl:"",//图片地址
// name:"",//名字名称标题
// orders:"",//订单量
// space:"",//距离
// tags:[],//标签数组
//coupon:[//优惠劵
//id
//couponMoney
//],
//star:3,//星评
},
data(){
return{}
},
methods:{
//跳转详情页
toPeopleContent(event){
//uni.redirectTo({
//url: '/pages/artificer/peopleContent?id=event'
//});
console.log("携带id跳转详情页" + event);
},
//获取优惠券
getCoupon(id,index){
console.log(id);
this.$emit("getCoupon",id,index)
},
//下订单
toOrders(){
console.log("下订单");
//uni.redirectTo({
//url: '/pages/artificer/peopleContent?id=event'
//});
},
}
}
</script>
<style>
.peopleList{display:inline-block;width:49%;margin:0 0 2rem 2%;overflow:hidden;vertical-align:top;}
.peopleList:nth-child(odd){margin-left:0;}
.peopleImg{width:100%;height:15rem;overflow:hidden;margin-bottom:1rem;border-radius:1rem;}
.peopleName{font-size:1.5rem;padding-bottom:0.5rem;}
.peopleStar .iconfont{color:#ff7101;font-size:1.6rem;margin-right:0.2rem;}
.peopleInfo{color:#969696;font-size:1.3rem;line-height:2.2rem;padding-bottom:0.6rem;}
.peopleInfo text{display:inline-block;margin-left:1rem;}
.peopleTags text{display:inline-block;margin-left:0.3rem;padding:0 0.2rem;font-size:1.2rem;line-height:1.8rem;}
.peopleTags text:nth-child(1){margin:0;background:#e5f8ed;color:#057332;}
.peopleTags text:nth-child(2){background:#fcebeb;color:#bc4242;}
.peopleTags text:nth-child(3){background:#e9f8ff;color:#007bb5;}
.peopleBt{display:flex;justify-content:flex-end;padding:1rem 0 .4rem 0;align-items:center;height:3rem;}
.peopleBt .iconfont{font-size:3rem;color:#ffaa37;margin-bottom:0.3rem;flex:1;}
.peopleBt .toOrder{background:#ffaa37;color:#fff;text-shadow:0 0.1rem 0 #e19631;box-shadow:0 0.2rem 0.3rem #f1c2aa;padding:0 1rem;border-radius:0.5rem;font-size:1.5rem;line-height:2.2rem;}
</style>
子组件CouponList:
<!--优惠券领取组件-->
<template>
<view class="couponBg" v-if="couponListData.isCoupon">
<swiper class="couponList" display-multiple-items="1" indicator-dots="true" indicator-active-color="#fff">
<swiper-item v-for="(coupons,index) in couponListData" :key="index">
<view class="coupon">
<view class="couponTop">
<text>{{coupons.couponTitle}}</text>
<view class="couponMoney"><text>{{coupons.couponMoney}}</text>元</view>
<text class="cff9c98">{{coupons.couponTxt}}</text>
</view>
<view class="couponBt" @click="getCoupon(coupons.id)">立即领取</view>
</view>
</swiper-item>
</swiper>
<view @click="couponClose" class="iconfont"></view>
</view>
</template>
<script>
export default {
name:"CouponList",
props:{
couponListData:Array,
//id技师或者商家id
//couponId优惠券id
//couponMoney优惠券金额
//couponTxt 使用说明
//couponTitle 劵类型
//isCoupon: true&false 组件是否显示
},
data() {
return {
}
},
methods:{
//关闭
couponClose(){
this.couponListData.isCoupon = false
},
//领取优惠券
getCoupon(id){
console.log("领取优惠券id" + id)
}
}
}
</script>
<style>
.couponBg{position:fixed;left:0;top:0;right:0;bottom:0;z-index:99;background:rgba(0,0,0,0.5);display:flex;justify-content:center;align-items:center;flex-direction:column;}
.couponBg .iconfont{color:#dbd6cf;font-size:4rem;}
.couponList{width:100%;height:20rem !important;white-space:nowrap;}
.coupon{width:46%;margin:0 auto 2rem auto;text-align:center;color:#fff;}
.couponTop{background:linear-gradient(to bottom,#fd6d47,#e82618);border-radius:1rem;padding:2rem 0;}
.couponMoney{padding:0.8rem 0;}
.couponMoney text{font-size:3rem;font-weight:bold;display:inline-block;margin-right:0.6rem;}
.cff9c98{color:#ff9c98;}
.couponBt{background:linear-gradient(to bottom,#e72316,#e1100a);color:#fff;padding:1rem 0;border-radius:1rem;font-size:1.7rem;border-top:0.1rem dotted #f6bdbb;}
</style>