遇到的问题:在使用postman调/oauth2/token这个接口的时候,报invalid_client。
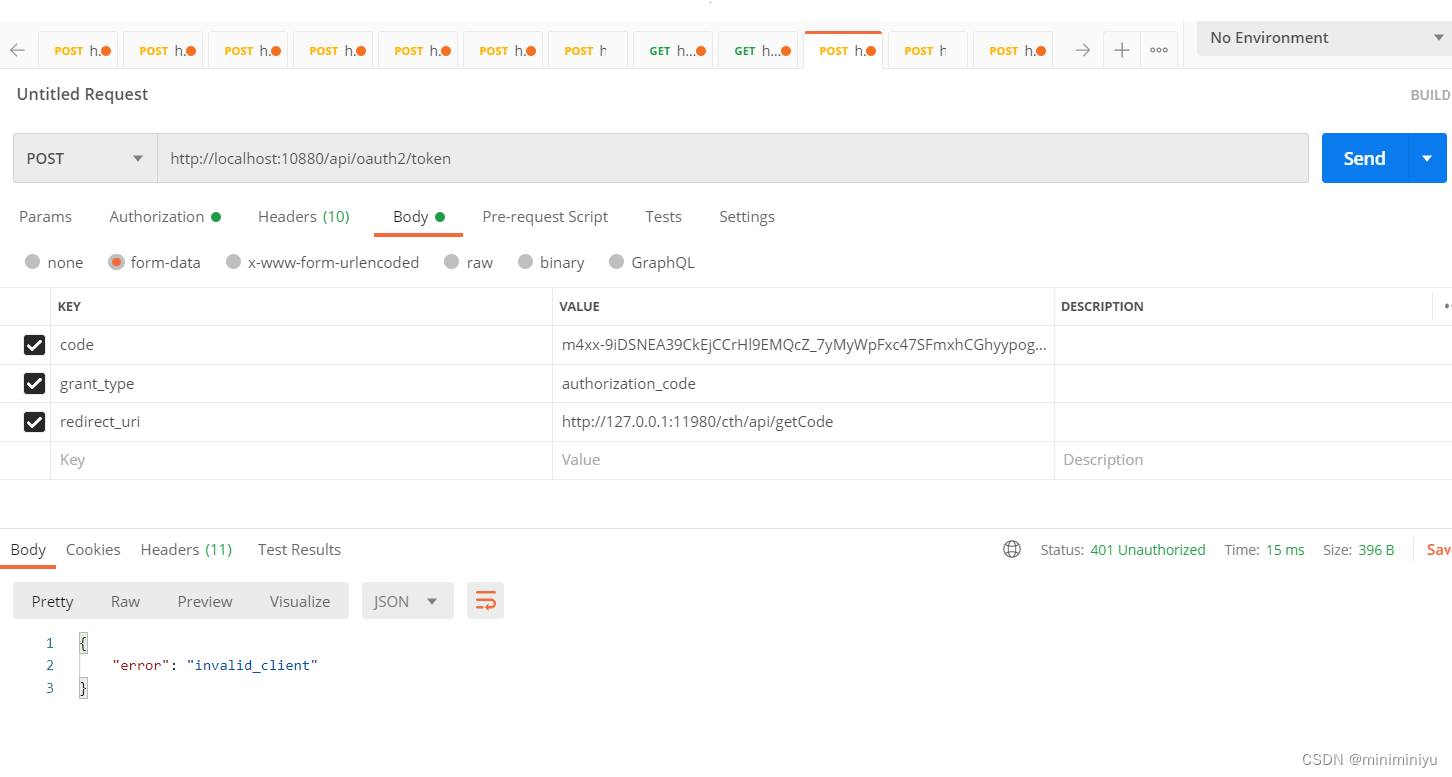
解决的方案:
我在网上看了好多解决办法都没能解决我遇到的问题,于是我打开源码,源码在
org.springframework.security.oauth2.core.OAuth2ErrorCodes.
找到报invalid_client的地方,自己debug进去,看看哪些地方调用了INVALID_CLIENT。
然后右击INVALID_CLIENT,选择 find usages,发现是
org.springframework.security.oauth2.server.authorization.authentication.ClientSecretAuthenticationProvider类中的throwInvalidClient()方法封装了这个错误,然后一个断点打在这里,追查是哪里调用了这个方法。
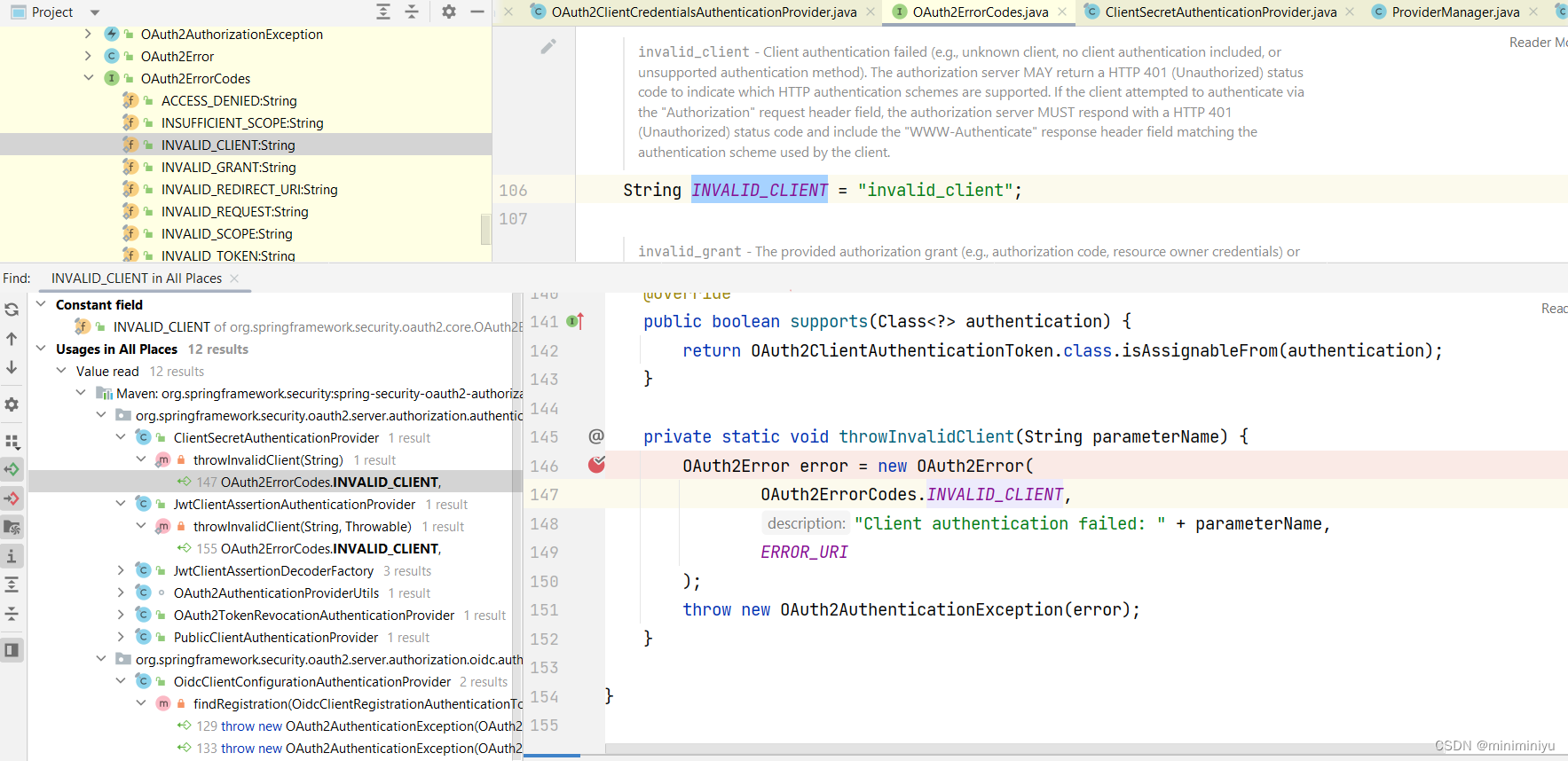
我遇到的问题是最后追查到ClientSecretAuthenticationProvider这个类的authenticate()方法中,我们可以看到是passwordEncoder.matches(clientSecret,registeredClient.getClientSecret())这行出了问题。
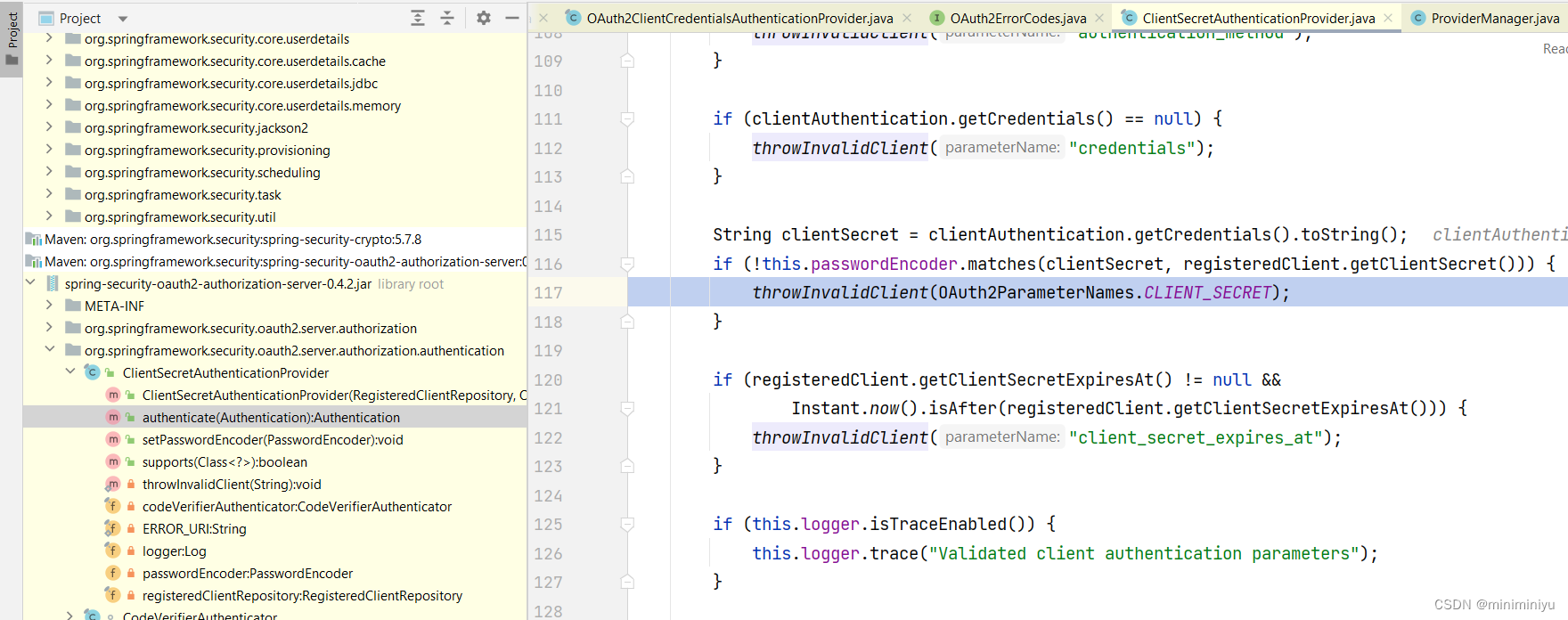
我自己定义了一个passwordEncoder,然后重写了matches方法。我原本的maches方法是这样的。
修改成了这样:
@Component
public class SSOPasswordEncoder implements PasswordEncoder {
@Override
public String encode(CharSequence rawPassword) {
return rawPassword.toString();
}
@Override
public boolean matches(CharSequence rawPassword, String encodedPassword) {
String digestPassword = null;
if(rawPassword instanceof String){
digestPassword = rawPassword.toString();
}else{
digestPassword = StringUtil.getDigest(rawPassword.toString());
}
return encode(digestPassword).equals(encodedPassword);
}
}
得到了正确的结果。
总结:即使有的时候报错都是invalid_client,但是导致invalid_client的原因可是不尽相同的,所以最好的办法就是找到是哪里抛出来的invalid_client,然后找到所有调用invalid_client的地方,都打上断点,一点点debug进去,看看是哪个条件不满足,最后抛了这个错误,然后修正那个不正确的条件,得以解决问题。