1、新增:
package com.miaosha.project.response;
public class CommonReturnType {
private String status;
private Object data;
//定义一个通用的创建方法
public static CommonReturnType create(Object result){
return CommonReturnType.create(result,"success");
}
public static CommonReturnType create(Object result, String status) {
CommonReturnType type = new CommonReturnType();
type.setStatus(status);
type.setData(result);
return type;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
}
package com.miaosha.project.controller;
import com.miaosha.project.controller.viewObject.UserVo;
import com.miaosha.project.response.CommonReturnType;
import com.miaosha.project.service.UserService;
import com.miaosha.project.service.model.UserModel;
import com.sun.org.apache.xpath.internal.operations.Mod;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller("user")
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/get")
@ResponseBody
public CommonReturnType getUser(@RequestParam("id") Integer id){
UserModel userModel = userService.getUserById(id);
UserVo userVo = convertFromUserModel(userModel);
return CommonReturnType.create(userVo);
}
private UserVo convertFromUserModel(UserModel userModel){
if(userModel == null){
return null;
}
UserVo userVo = new UserVo();
//字段名以及类型均一致
BeanUtils.copyProperties(userModel,userVo);
return userVo;
}
}
修改:
返回结果:
二、异常处理
package com.miaosha.project.error;
public interface CommonError {
public int getErrCode();
public String getErrMsg();
public CommonError setErrMsg(String errMsg);
}
package com.miaosha.project.error;
public enum EmBusinessError implements CommonError {
//通用错误类型00001
PARAMETER_VALIDATION_ERROR(00001,"参数不合法 "),
//以10000开头为用户信息相关定义
USER_NOT_EXIT(10001,"用户不存在")
;
private int errCode;
private String errMsg;
private EmBusinessError(int errCode,String errMsg){
this.errCode = errCode;
this.errMsg = errMsg;
}
@Override
public int getErrCode() {
return this.errCode;
}
@Override
public String getErrMsg() {
return this.errMsg;
}
@Override
public CommonError setErrMsg(String errMsg) {
this.errMsg = errMsg;
return this;
}
}
package com.miaosha.project.error;
/**
* 包装器业务异常类实现
*/
public class BusinessException extends Exception implements CommonError {
private CommonError commonError;
//直接接收EmBusinessError的传参用于构造业务异常
public BusinessException(CommonError commonError){
super();
this.commonError = commonError;
}
//接收自定义ErrMsg的方式用于构造业务异常
public BusinessException(CommonError commonError,String errMsg){
super();
this.commonError = commonError;
this.commonError.setErrMsg(errMsg);
}
@Override
public int getErrCode() {
return this.commonError.getErrCode();
}
@Override
public String getErrMsg() {
return this.commonError.getErrMsg();
}
@Override
public CommonError setErrMsg(String errMsg) {
this.setErrMsg(errMsg);
return this;
}
}
package com.miaosha.project.controller;
import com.miaosha.project.error.BusinessException;
import com.miaosha.project.error.EmBusinessError;
import com.miaosha.project.response.CommonReturnType;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.Map;
public class BaseController {
//定义exceptionhandler解决未被controller层吸收的exception
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.OK)
@ResponseBody
public Object handlerException(HttpServletRequest request, Exception ex){
Map<String,Object> responseData = new HashMap<>();
if(ex instanceof BusinessException){
BusinessException businessException = (BusinessException) ex;
responseData.put("errCode",businessException.getErrCode());
responseData.put("errMsg",businessException.getErrMsg());
}else{
responseData.put("errCode",EmBusinessError.UNKNOWN_ERROR.getErrCode());
responseData.put("errMsg",EmBusinessError.UNKNOWN_ERROR.getErrMsg());
}
return CommonReturnType.create(responseData,"fail");
}
}
三、短信发送:
package com.miaosha.project.controller;
import com.miaosha.project.controller.viewObject.UserVo;
import com.miaosha.project.error.BusinessException;
import com.miaosha.project.error.EmBusinessError;
import com.miaosha.project.response.CommonReturnType;
import com.miaosha.project.service.UserService;
import com.miaosha.project.service.model.UserModel;
import com.sun.org.apache.xpath.internal.operations.Mod;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import javax.xml.ws.ResponseWrapper;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
@Controller("user")
@RequestMapping("/user")
public class UserController extends BaseController{
@Autowired
private UserService userService;
@Autowired
private HttpServletRequest httpServletRequest;
//用户获取otp短信接口
@RequestMapping("/getOpt")
@ResponseBody
public CommonReturnType getOtp(@RequestParam("telphone") String telphone){
//按照规则生成otp验证码
Random random = new Random();
int randomInt = random.nextInt(99999);
randomInt += 10000;
String optCode = String.valueOf(randomInt);
//将otp验证码同对应用户的手机号关联,使用httpsession的方式绑定opt和手机号
httpServletRequest.getSession().setAttribute(telphone,optCode);
//将otp验证码发送给用户
System.out.println("telephone="+telphone+"&optCode="+optCode);
return CommonReturnType.create(null);
}
@RequestMapping("/get")
@ResponseBody
public CommonReturnType getUser(@RequestParam("id") Integer id) throws BusinessException {
UserModel userModel = userService.getUserById(id);
//若获取的用户对应信息不存在
if(userModel == null){
throw new BusinessException(EmBusinessError.USER_NOT_EXIT);
// userModel.setEncrptPassword("1234");//位置错误
}
//将核心领域模型用户对象转换为可供UI使用的ViewObject
UserVo userVo = convertFromUserModel(userModel);
return CommonReturnType.create(userVo);
}
private UserVo convertFromUserModel(UserModel userModel){
if(userModel == null){
return null;
}
UserVo userVo = new UserVo();
//字段名以及类型均一致
BeanUtils.copyProperties(userModel,userVo);
return userVo;
}
}
控制台打印:
四、前端页面:
<html>
<head>
<meta charset="utf-8">
<script src="static/assets/global/plugins/jquery-1.11.0.min.js" type="text/javascript"></script
</head>
<body>
<div>
<h3>获取otp信息</h3>
<div>
<label>手机号</label>
<div>
<input type="text" placeholder="手机号" name="telphone" id="telphone" />
</div>
</div>
<div>
<button id="getotp" type="submit">获取otp短信</button>
</div>
</div>
</body>
<script>
jQuery(document).ready(function(){
$("#getotp").on("click",function(){
var telphone = $("#telphone").val();
if(telphone == null || telphone == ""){
alert("手机号不能为空");
return false;
}
$.ajax({
type:"POST",
contentType:"application/x-www-form-urlencoded",
url:"http://localhost:8080/user/getOpt",
data:{
"telphone":$("#telphone").val(),
},
success:function(data){
if(data.status == "success"){
alert("otp已经发送到您的手机上,请注意查收")
}else{
alert("otp发送失败,原因为"+data.data.errMsg);
}
},
error:function(data){
alert("otp发送失败,原因为"+data.responseText);
}
});
return false;
});
});
</script>
</html>
启动:
访问:
五、页面美化
<html>
<head>
<meta charset="utf-8">
<link href="static/assets/global/plugins/bootstrap/css/bootstrap.min.css" style="stylesheet" type="text/css"/>
<link href="static/assets/global/css/components.css" rel="stylesheet" type="text/css"/>
<link href="static/assets/admin/pages/css/login.css" rel="stylesheet" type="text/css"/>
<script src="static/assets/global/plugins/jquery-1.11.0.min.js" type="text/javascript"></script
</head>
<body class="login">
<div class="content">
<h3 class="form-title">获取otp信息</h3>
<div class="form-group">
<label class="control-label">手机号</label>
<div>
<input class="form-control" type="text" placeholder="手机号" name="telphone" id="telphone" />
</div>
</div>
<div class="form-actions">
<button class="btn blue" id="getotp" type="submit">获取otp短信</button>
</div>
</div>
</body>
<script>
jQuery(document).ready(function(){
$("#getotp").on("click",function(){
var telphone = $("#telphone").val();
if(telphone == null || telphone == ""){
alert("手机号不能为空");
return false;
}
$.ajax({
type:"POST",
contentType:"application/x-www-form-urlencoded",
url:"http://localhost:8080/user/getOpt",
data:{
"telphone":$("#telphone").val(),
},
success:function(data){
if(data.status == "success"){
alert("otp已经发送到您的手机上,请注意查收")
}else{
alert("otp发送失败,原因为"+data.data.errMsg);
}
},
error:function(data){
alert("otp发送失败,原因为"+data.responseText);
}
});
return false;
});
});
</script>
</html>
六、注册用户
getotp.html
<html>
<head>
<meta charset="utf-8">
<link href="static/assets/global/plugins/bootstrap/css/bootstrap.min.css" style="stylesheet" type="text/css"/>
<link href="static/assets/global/css/components.css" rel="stylesheet" type="text/css"/>
<link href="static/assets/admin/pages/css/login.css" rel="stylesheet" type="text/css"/>
<script src="static/assets/global/plugins/jquery-1.11.0.min.js" type="text/javascript"></script
</head>
<body class="login">
<div class="content">
<h3 class="form-title">获取otp信息</h3>
<div class="form-group">
<label class="control-label">手机号</label>
<div>
<input class="form-control" type="text" placeholder="手机号" name="telphone" id="telphone" />
</div>
</div>
<div class="form-actions">
<button class="btn blue" id="getotp" type="submit">获取otp短信</button>
</div>
</div>
</body>
<script>
jQuery(document).ready(function(){
$("#getotp").on("click",function(){
var telphone = $("#telphone").val();
if(telphone == null || telphone == ""){
alert("手机号不能为空");
return false;
}
$.ajax({
type:"POST",
contentType:"application/x-www-form-urlencoded",
url:"http://localhost:8080/user/getOtp",
data:{
"telphone":$("#telphone").val(),
},
xhrFields:{withCredentials:true},
success:function(data){
if(data.status == "success"){
alert("otp已经发送到您的手机上,请注意查收")
window.location.href="file:///D:/Workspace/Idea/register.html";
}else{
alert("otp发送失败,原因为"+data.data.errMsg);
}
},
error:function(data){
alert("otp发送失败,原因为"+data.responseText);
}
});
return false;
});
});
</script>
</html>
register.html
<html>
<head>
<meta charset="utf-8">
<link href="static/assets/global/plugins/bootstrap/css/bootstrap.min.css" style="stylesheet" type="text/css"/>
<link href="static/assets/global/css/components.css" rel="stylesheet" type="text/css"/>
<link href="static/assets/admin/pages/css/login.css" rel="stylesheet" type="text/css"/>
<script src="static/assets/global/plugins/jquery-1.11.0.min.js" type="text/javascript"></script
</head>
<body class="login">
<div class="content">
<h3 class="form-title">用户注册</h3>
<div class="form-group">
<label class="control-label">手机号</label>
<div>
<input class="form-control" type="text" placeholder="手机号" name="telphone" id="telphone" />
</div>
</div>
<div class="form-group">
<label class="control-label">验证码</label>
<div>
<input class="form-control" type="text" placeholder="验证码" name="otpCode" id="otpCode" />
</div>
</div>
<div class="form-group">
<label class="control-label">用户昵称</label>
<div>
<input class="form-control" type="text" placeholder="用户昵称" name="name" id="name" />
</div>
</div>
<div class="form-group">
<label class="control-label">性别</label>
<div>
<input class="form-control" type="text" placeholder="性别" name="gender" id="gender" />
</div>
</div>
<div class="form-group">
<label class="control-label">年龄</label>
<div>
<input class="form-control" type="text" placeholder="年龄" name="age" id="age" />
</div>
</div>
<div class="form-group">
<label class="control-label">密码</label>
<div>
<input class="form-control" type="password" placeholder="密码" name="password" id="password" />
</div>
</div>
<div class="form-actions">
<button class="btn blue" id="register" type="submit">提交注册</button>
</div>
</div>
</body>
<script>
jQuery(document).ready(function(){
$("#register").on("click",function(){
var telphone = $("#telphone").val();
var password = $("#password").val();
var age = $("#age").val();
var gender = $("#gender").val();
var name = $("#name").val();
var otpCode = $("#otpCode").val();
if(telphone == null || telphone == ""){
alert("手机号不能为空");
return false;
}
if(password == null || password == ""){
alert("密码不能为空");
return false;
}
if(age == null || age == ""){
alert("年龄不能为空");
return false;
}
if(gender == null || gender == ""){
alert("性别不能为空");
return false;
}
if(name == null || name == ""){
alert("姓名不能为空");
return false;
}
if(otpCode == null || otpCode == ""){
alert("短信验证码不能为空");
return false;
}
$.ajax({
type:"POST",
contentType:"application/x-www-form-urlencoded",
url:"http://localhost:8080/user/register",
data:{
"telphone":$("#telphone").val(),
"password":password,
"age":age,
"gender":gender,
"name":name,
"otpCode":otpCode
},
xhrFields:{withCredentials:true},
success:function(data){
if(data.status == "success"){
alert("注册成功")
window.location.href="file:///D:/Workspace/Idea/register.html";
}else{
alert("注册失败,原因为"+data.data.errMsg);
}
},
error:function(data){
alert("otp发送失败,原因为"+data.responseText);
}
});
return false;
});
});
</script>
</html>
package com.miaosha.project.service;
import com.miaosha.project.error.BusinessException;
import com.miaosha.project.service.model.UserModel;
public interface UserService {
UserModel getUserById(Integer id);
void register(UserModel userModel) throws BusinessException;
}
package com.miaosha.project.service.impl;
import com.alibaba.druid.util.StringUtils;
import com.miaosha.project.dao.UserDOMapper;
import com.miaosha.project.dao.UserPasswordDOMapper;
import com.miaosha.project.dataObject.UserDO;
import com.miaosha.project.dataObject.UserPasswordDO;
import com.miaosha.project.error.BusinessException;
import com.miaosha.project.error.EmBusinessError;
import com.miaosha.project.service.UserService;
import com.miaosha.project.service.model.UserModel;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDOMapper userDOMapper;
@Autowired
private UserPasswordDOMapper userPasswordDOMapper;
@Override
public UserModel getUserById(Integer id) {
UserDO userDO = userDOMapper.selectByPrimaryKey(id);
if(userDO == null){
return null;
}
//通过用户id获取用户加密信息
UserPasswordDO userPasswordDO = userPasswordDOMapper.selectByUserId(userDO.getId());
return convertFromDataObject(userDO,userPasswordDO);
}
/**
* 注册用户
* @param userModel
*/
@Override
@Transactional
public void register(UserModel userModel) throws BusinessException {
if(userModel == null){
throw new BusinessException(EmBusinessError.PARAMETER_VALIDATION_ERROR);
}
if(StringUtils.isEmpty(userModel.getName())
|| userModel.getGender() == null
|| userModel.getAge() == null
|| StringUtils.isEmpty(userModel.getTelphone())){
throw new BusinessException(EmBusinessError.PARAMETER_VALIDATION_ERROR);
}
UserDO userDO = convertFromModel(userModel);
userDOMapper.insertSelective(userDO);
UserPasswordDO userPasswordDO = convertPasswordFromModel(userModel);
userPasswordDOMapper.insertSelective(userPasswordDO);
return;
}
private UserPasswordDO convertPasswordFromModel(UserModel userModel){
if(userModel == null){
return null;
}
UserPasswordDO userPasswordDO = new UserPasswordDO();
userPasswordDO.setEncrptPassword(userModel.getEncrptPassword());
userPasswordDO.setUserId(userModel.getId());
return userPasswordDO;
}
private UserDO convertFromModel(UserModel userModel){
if(userModel == null){
return null;
}
UserDO userDO = new UserDO();
BeanUtils.copyProperties(userModel,userDO);
return userDO;
}
private UserModel convertFromDataObject(UserDO userDO, UserPasswordDO userPasswordDO){
if(userDO == null){
return null;
}
UserModel userModel = new UserModel();
BeanUtils.copyProperties(userDO,userModel);
if(userPasswordDO != null){
userModel.setEncrptPassword(userPasswordDO.getEncrptPassword());
}
return userModel;
}
}
package com.miaosha.project.controller;
import com.alibaba.druid.util.StringUtils;
import com.miaosha.project.controller.viewObject.UserVo;
import com.miaosha.project.error.BusinessException;
import com.miaosha.project.error.EmBusinessError;
import com.miaosha.project.response.CommonReturnType;
import com.miaosha.project.service.UserService;
import com.miaosha.project.service.model.UserModel;
import org.apache.tomcat.util.security.MD5Encoder;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
import javax.servlet.http.HttpServletRequest;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Random;
@Controller("user")
@RequestMapping("/user")
@CrossOrigin(allowedHeaders = "*",allowCredentials = "true")
public class UserController extends BaseController{
@Autowired
private UserService userService;
@Autowired
private HttpServletRequest httpServletRequest;
//用户注册
@RequestMapping(value = "/register",method = {RequestMethod.POST},consumes = {CONTENT_TYPE_FORMED})
@ResponseBody
public CommonReturnType register(@RequestParam("telphone") String telphone,
@RequestParam(name="otpCode") String otpCode,
@RequestParam(name="name") String name,
@RequestParam(name="gender") Integer gender,
@RequestParam(name="age") Integer age,
@RequestParam(name="password") String password) throws BusinessException, UnsupportedEncodingException, NoSuchAlgorithmException {
//验证手机号和对应的otpcode相符合
String inSessionOtpCode = (String) this.httpServletRequest.getSession().getAttribute(telphone);
if(!StringUtils.equals(otpCode,inSessionOtpCode)){
throw new BusinessException(EmBusinessError.PARAMETER_VALIDATION_ERROR,"短信验证码不符合");
}
//用户的注册流程
UserModel userModel = new UserModel();
userModel.setName(name);
userModel.setGender(new Byte(String.valueOf(gender.intValue())));
userModel.setAge(age);
userModel.setTelphone(telphone);
userModel.setRisterMode("byPhone");
userModel.setThirdPartyId("1235");
userModel.setEncrptPassword(this.EncodeByMd5(password));
userService.register(userModel);
return CommonReturnType.create(null);
}
public String EncodeByMd5(String str) throws NoSuchAlgorithmException, UnsupportedEncodingException {
MessageDigest md5 = MessageDigest.getInstance("MD5");
BASE64Encoder base64Encoder = new BASE64Encoder();
//加密字符串
String newStr = base64Encoder.encode(md5.digest(str.getBytes("utf-8")));
return newStr;
}
//用户获取otp短信接口
@RequestMapping(value = "/getOtp",method = {RequestMethod.POST},consumes = {CONTENT_TYPE_FORMED})
@ResponseBody
public CommonReturnType getOtp(@RequestParam("telphone") String telphone){
//按照规则生成otp验证码
Random random = new Random();
int randomInt = random.nextInt(99999);
randomInt += 10000;
String optCode = String.valueOf(randomInt);
//将otp验证码同对应用户的手机号关联,使用httpsession的方式绑定opt和手机号
httpServletRequest.getSession().setAttribute(telphone,optCode);
//将otp验证码发送给用户
System.out.println("telephone="+telphone+"&optCode="+optCode);
return CommonReturnType.create(null);
}
@RequestMapping("/get")
@ResponseBody
public CommonReturnType getUser(@RequestParam("id") Integer id) throws BusinessException {
UserModel userModel = userService.getUserById(id);
//若获取的用户对应信息不存在
if(userModel == null){
throw new BusinessException(EmBusinessError.USER_NOT_EXIT);
// userModel.setEncrptPassword("1234");//位置错误
}
//将核心领域模型用户对象转换为可供UI使用的ViewObject
UserVo userVo = convertFromUserModel(userModel);
return CommonReturnType.create(userVo);
}
private UserVo convertFromUserModel(UserModel userModel){
if(userModel == null){
return null;
}
UserVo userVo = new UserVo();
//字段名以及类型均一致
BeanUtils.copyProperties(userModel,userVo);
return userVo;
}
}
访问:file:///D:/Workspace/Idea/getotp.html 发送短信成功后 跳转到 file:///D:/Workspace/Idea/register.html用户注册页面
报错:进行不下去,控制台报错,Id无初始值,数据库的问题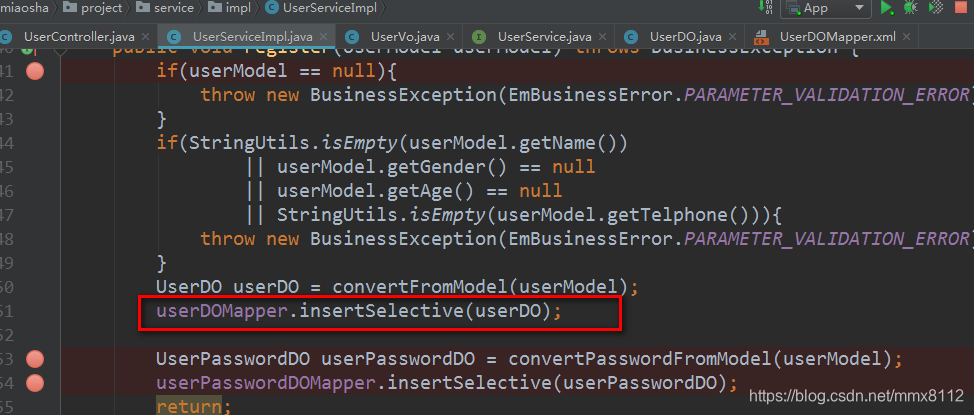
手机号唯一:
数据库建立索引:
再次输入相同的手机号会报错,控制台输出:
二遍问题:
httpServletRequest.getSession().setAttribute(telphone,otpCode);
String inSessionOtpCode = (String) httpServletRequest.getSession().getAttribute(telphone);没有引号,是key的名称