二叉排序树构建
结构
typedef int KeyType;
typedef struct node{
KeyType key;
int data;
struct node*lchild,*rchild;
}BSTNode;
创建
BSTNode* CreateBST(int a[],int n){
BSTNode *bt=NULL;
int i=0;
while(i<n){
InsertBST(bt,a[i]);
i++;
}
return bt;
}
展示
void DispBST(BSTNode *b){
if(b!=NULL){
printf("%d",b->key);
if(b->lchild!=NULL||b->rchild!=NULL){
printf("(");
DispBST(b->lchild);
if(b->rchild!=NULL)printf(",");
DispBST(b->rchild);
printf(")");
}
}
}
查找(递归非递归)
int SearchBST(BSTNode *bt,KeyType k){
if(bt==NULL)
return 0;
else if(k==bt->key){
cout<<bt->key<<" ";
return 1;
}
else if(k<bt->key)
SearchBST(bt->lchild,k);
else
SearchBST(bt->rchild,k);
cout<<bt->key<<" ";
}
void SearchBST2(BSTNode *bt,int k,int path[],int i){
int j;
if(bt==NULL)
return ;
else if(k==bt->key){
path[i+1]=bt->key;
for(j=0;j<=i+1;j++)
cout<<path[j]<<" ";
cout<<endl;
}else{
path[i+1]=bt->key;
if(k<bt->key)
SearchBST2(bt->lchild,k,path,i+1);
else
SearchBST2(bt->rchild,k,path,i+1);
}
}
删除
bool DeleteBST(BSTNode *&bt,KeyType k)
{
if (bt==NULL) return false;
else
{
if (k<bt->key)
return DeleteBST(bt->lchild,k);
else if (k>bt->key)
return DeleteBST(bt->rchild,k);
else
{
Delete(bt);
return true;
}
}
}
结果
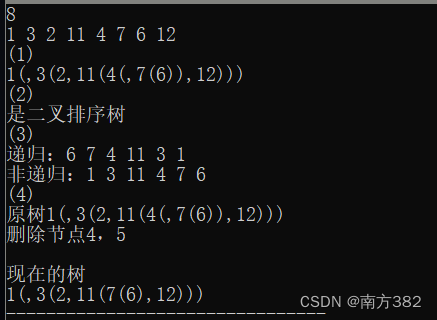