需求:
1.绑定商品可用的优惠券,按面值大小倒序。
2.当优惠券支持多个商品使用时,勾选了当前优惠券,其它商品下同张优惠券置灰,不可选。
#效果图如下:
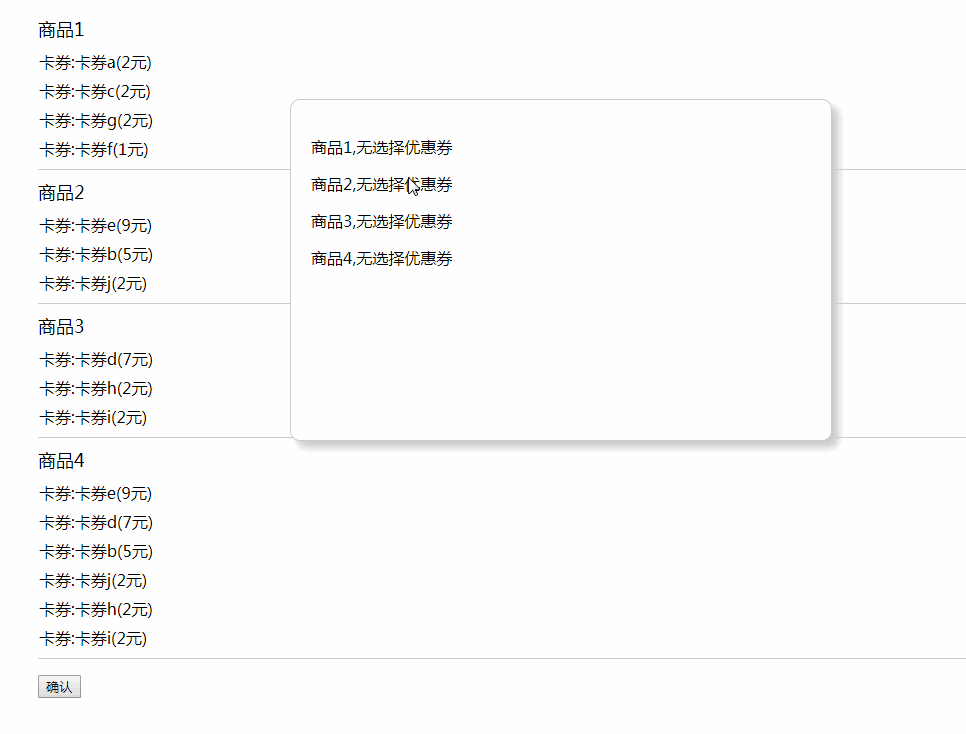
#完整代码如下:
<html>
<head>
<style type="text/css">
* {
-moz-user-select: none; /*火狐*/
-webkit-user-select: none; /*webkit浏览器*/
-ms-user-select: none; /*IE10*/
-khtml-user-select: none; /*早期浏览器*/
user-select: none;
}
#product .pli {
list-style-type: none;
padding: 5px 0;
border-bottom: 1px solid #ccc;
}
.pli p {
padding: 5px 0;
margin: 0;
font-size: 18px;
}
.ticket li {
line-height: 25px;
width: 200px;
}
.t_li {
cursor: pointer;
margin: 2px 0;
border: 1px solid #fff;
}
.t_li:hover {
background-color: #AFEEEE;
}
.ticket .check {
background-color: #48D1CC;
border-color: #1c6a9e;
}
.ticket .disabled {
background: #b1b1b1;
}
.msg {
padding: 20px;
display: none;
width: 500px;
height: 300px;
position: fixed;
top: 100px;
left: 300px;
border: 1px solid #ccc;
border-radius: 10px;
box-shadow: 7px 7px 11px 0px #ccc;
background-color: #fff;
}
</style>
<script type="application/javascript" src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script type="application/javascript">
var ticketList = new Array();
//获取优惠券
function getTicket() {
var t1 = {id: 1, type: 1, amount: 2, name: '卡券a'};
var t2 = {id: 2, type: 2, amount: 5, name: '卡券b'};
var t3 = {id: 3, type: 1, amount: 2, name: '卡券c'};
var t4 = {id: 4, type: 3, amount: 7, name: '卡券d'};
var t5 = {id: 5, type: 2, amount: 9, name: '卡券e'};
var t6 = {id: 6, type: 1, amount: 1, name: '卡券f'};
var t7 = {id: 7, type: 1, amount: 2, name: '卡券g'};
var t8 = {id: 8, type: 3, amount: 2, name: '卡券h'};
var t9 = {id: 9, type: 3, amount: 2, name: '卡券i'};
var t10 = {id: 10, type: 2, amount: 2, name: '卡券j'};
ticketList.push(t1);
ticketList.push(t2);
ticketList.push(t3);
ticketList.push(t4);
ticketList.push(t5);
ticketList.push(t6);
ticketList.push(t7);
ticketList.push(t8);
ticketList.push(t9);
ticketList.push(t10);
ticketList.sort(compare("amount"));
return ticketList;
}
function compare(prop) {
return function (obj1, obj2) {
var val1 = obj1[prop];
var val2 = obj2[prop];
if (!isNaN(Number(val1)) && !isNaN(Number(val2))) {
val1 = Number(val1);
val2 = Number(val2);
}
if (val1 < val2) {
return 1;
} else if (val1 > val2) {
return -1;
} else {
return 0;
}
}
}
function toMap(ticket) {
var map = {};
for (var i = 0; i < ticket.length; i++) {
var list = new Array();
var key = ticket[i].type;
if (map[key] != undefined && map[key].length > 0) {
list = map[key];
}
list.push(ticket[i]);
map[key] = list;
}
return map;
}
//加载优惠券
function loadTicket() {
var ticket = getTicket();
var ticketMap = toMap(ticket);
$("#product .pli").each(function () {
var type = $(this).attr("type");
var html = "";
var list = new Array();
if (type != "") {
list = ticketMap[type];
}
if (type == "") {
list = list.concat(ticketMap[2]);
list = list.concat(ticketMap[3]);
list.sort(compare("amount"));
}
if (list == undefined || list.length == 0) {
return;
}
for (var i = 0; i < list.length; i++) {
html += "<li class='t_li t_li_" + list[i].id + "' tid='" + list[i].id + "' name='" + list[i].name + "' amount='" + list[i].amount + "'>卡券:" + list[i].name + "(" + list[i].amount + "元)</li>";
}
$(this).find(".ticket").html(html);
});
}
$(document).ready(function () {
loadTicket();
});
//优惠券选择事件
$(document).on("click", ".t_li", function () {
if ($(this).hasClass("disabled")) {
return;
}
var className = ".t_li_" + $(this).attr("tid");
if ($(this).hasClass("check")) {
$(this).removeClass("check");
$(className).removeClass("disabled");
} else {
$(className).not($(this)).addClass("disabled");
$(this).addClass("check");
}
});
//按钮点击事件
$(document).on("click", "#btn", function () {
var html = "";
$(".pli").each(function () {
var that = $(this);
html += "<p>" + that.find("p").html() + ",";
var checkTicket = "";
that.find(".ticket .check").each(function () {
checkTicket += $(this).attr("name") + "(" + $(this).attr("amount") + ")元;";
});
if (checkTicket != "") {
checkTicket = checkTicket.substring(0, checkTicket.length - 1);
html += '已选择:' + checkTicket ;
}
else {
html += "无选择优惠券";
}
html += "</p>";
});
$(".msg").html(html);
$(".msg").show();
});
$(document).on("click", ".main", function () {
$(".msg").hide();
});
</script>
</head>
<body>
<div class="main">
<ul id="product">
<li class="pli" type="1">
<p>商品1</p>
<div class="ticket"></div>
</li>
<li class="pli" type="2">
<p>商品2</p>
<div class="ticket"></div>
</li>
<li class="pli" type="3">
<p>商品3</p>
<div class="ticket"></div>
</li>
<li class="pli" type="">
<p>商品4</p>
<div class="ticket"></div>
</li>
</ul>
</div>
<button id="btn">确认</button>
<div class="msg"></div>
</body>
</html>