<template>
<!--
vue 的开发模式:单组件模式开发,由小组件组成 xxx.vue
写法格式: <template></template> <script></script> <style></style>
<template></template> 里只能有一个子元素 子元素中可以有多个元素
-->
<!--
vue 的相关指令:
1.数据绑定指令
v-bind:属性绑定数据的格式
v-text:元素的文本值
v-html:元素的html内容
{{}}:数据绑定模板
v-model:表单元素属性绑定
-->
<!--
vue 组件上变量的声明 : 在js模块内部声明
每个组件都存在 data 属性 , 当前指针(this)
-->
<div>
<p>{{ message }}</p>
<!-- hello -->
<p>{{ spanEle }}</p>
<!-- <span>一个span</span> -->
<p v-text="spanEle"></p>
<!-- <span>一个span</span> -->
<p v-html="spanEle"></p>
<!-- 一个span -->
<!-- Elements中显示为:
<p>
<span>一个span</span>
</p> -->
<!--
2.元素属性值绑定指令以及简写 v-bind
v-bind:title='message';
简写: :title ='message';
-->
<h3 v-bind:title="h3Tit"></h3>
<!-- <h3 title="标题"></h3> -->
<div v-bind:class="divClass"></div>
<!-- <div class="divBox"></div> -->
<div :class="divClass"></div>
<!-- 表单元素的value值绑定 v-model -->
<input class="ipt1" type="text" :value="iptVal" />
ipt1
<input class="ipt2" type="text" v-model="iptVal" />
ipt2
<!-- ipt2更改,ipt1随之更改
但ip1更改,iP2不变
v-bind:数据单向绑定
v-model:数据双向绑定,只能用于表单元素
vue数据双向绑定原理:数据劫持 + 发布订阅设计模式
vue的设计模式:MVVM(model:数据层 View:视图层 ViewModel:业务逻辑层)
-->
<!-- 数组(集合)渲染
v-for指令: 循环谁给谁添加指令-->
<p>{{ forArr }}</p>
<!-- [ 1, 2, 3, 4, 5 ] -->
<ul>
<!-- |---------------------------------------------| -->
<li v-for="(item, index) in forArr" v-bind:key="index">{{ item + ' : li-' + index }}</li>
<!-- |--------需绑定唯一的key-------| -->
<!--
1 : li-0
2 : li-1
3 : li-2
4 : li-3
5 : li-4
-->
</ul>
<ul id="stuList">
<li v-for="(value, index) in stuList" :key="index">{{ value[0].name }} : {{ value[0].info[0].height }} - {{ value[0].info[0].sex }};</li>
</ul>
<!-- 元素显示隐藏指令 v-show -->
<!-- 值可能是变量/表达式 -->
<button id="showBtn" @click="showFn">显示/隐藏</button>
<div class="divBox" v-show="isShow"></div>
<!-- v-if v-elseif v-else 指令 -->
<h3 v-if="isShow">h3标签</h3>
<h5 v-else-if="judge1 && judge2">h5标签</h5>
<h1 v-else>h1标签</h1>
<!-- 元素的class / style 绑定 -->
<button id="addBtn" @click="changeColor">变</button>
<div class="divBox" :class="{ divBox_2: isAdd }"></div>
<!-- 元素的样式绑定 -->
<div :style="{ width: sWidth, height: sHeight, background: sBgColor }"></div>
<div :style="sStyle"></div>
</div>
</template>
<script>
export default {
name: 'pra-03-29',
data() {
return {
message: 'hello',
spanEle: '<span>一个span</span>',
h3Tit: '标题',
divClass: 'divBox',
iptVal: '请输入内容',
forArr: [1, 2, 3, 4, 5],
stuList: {
stu1: [
{
name: '小明',
info: [
{
sex: 'female',
height: 155
}
]
}
],
stu2: [
{
name: '小亮',
info: [
{
sex: 'female',
height: 185
}
]
}
],
stu3: [
{
name: '小红',
info: [
{
sex: 'male',
height: 175
}
]
}
]
},
isShow: true,
judge1: true,
judge2: true,
isAdd: true,
sWidth: '180px',
sHeight: '180px',
sBgColor: 'blue',
sStyle: {
width: '130px',
height: '160px',
border: '1px solid #345678',
marginTop: '20px'
}
};
},
methods: {
//添加显示隐藏指令
showFn() {
console.log(this.isShow);
this.isShow = !this.isShow;
this.judge2 = !this.judge2;
},
changeColor() {
this.isAdd = !this.isAdd;
}
},
watch: {
//v-model事件监听
iptVal(nowVal, oldVal) {
console.log(nowVal, oldVal);
}
}
};
</script>
<style>
.divBox {
width: 100px;
height: 100px;
border: 1px solid rgb(65, 179, 103);
}
.divBox_2 {
width: 150px;
height: 150px;
background-color: rgb(243, 64, 64);
}
#stuList {
margin-left: 0;
padding: 0;
}
#stuList li {
list-style: none;
font-size: 18px;
margin-bottom: 5px;
margin-left: 0;
}
</style>
2021-03-31 vue基础语法(数据绑定指令 / 元素属性值绑定指令 / 数组\集合渲染 / 元素显示\隐藏指令 / 元素的类\style 绑定指令)
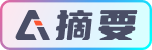